Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / MatrixCamera.cs / 1 / MatrixCamera.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class MatrixCamera : Camera { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new MatrixCamera Clone() { return (MatrixCamera)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new MatrixCamera CloneCurrentValue() { return (MatrixCamera)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ViewMatrixPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { MatrixCamera target = ((MatrixCamera) d); target.PropertyChanged(ViewMatrixProperty); } private static void ProjectionMatrixPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { MatrixCamera target = ((MatrixCamera) d); target.PropertyChanged(ProjectionMatrixProperty); } #region Public Properties ////// ViewMatrix - Matrix3D. Default value is Matrix3D.Identity. /// public Matrix3D ViewMatrix { get { return (Matrix3D) GetValue(ViewMatrixProperty); } set { SetValueInternal(ViewMatrixProperty, value); } } ////// ProjectionMatrix - Matrix3D. Default value is Matrix3D.Identity. /// public Matrix3D ProjectionMatrix { get { return (Matrix3D) GetValue(ProjectionMatrixProperty); } set { SetValueInternal(ProjectionMatrixProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new MatrixCamera(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform3D vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform3D.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Pack & send command packet DUCE.MILCMD_MATRIXCAMERA data; unsafe { data.Type = MILCMD.MilCmdMatrixCamera; data.Handle = _duceResource.GetHandle(channel); data.htransform = hTransform; data.viewMatrix = CompositionResourceManager.Matrix3DToD3DMATRIX(ViewMatrix); data.projectionMatrix = CompositionResourceManager.Matrix3DToD3DMATRIX(ProjectionMatrix); // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_MATRIXCAMERA)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_MATRIXCAMERA)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the MatrixCamera.ViewMatrix property. /// public static readonly DependencyProperty ViewMatrixProperty; ////// The DependencyProperty for the MatrixCamera.ProjectionMatrix property. /// public static readonly DependencyProperty ProjectionMatrixProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Matrix3D s_ViewMatrix = Matrix3D.Identity; internal static Matrix3D s_ProjectionMatrix = Matrix3D.Identity; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static MatrixCamera() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(MatrixCamera); ViewMatrixProperty = RegisterProperty("ViewMatrix", typeof(Matrix3D), typeofThis, Matrix3D.Identity, new PropertyChangedCallback(ViewMatrixPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ProjectionMatrixProperty = RegisterProperty("ProjectionMatrix", typeof(Matrix3D), typeofThis, Matrix3D.Identity, new PropertyChangedCallback(ProjectionMatrixPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class MatrixCamera : Camera { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new MatrixCamera Clone() { return (MatrixCamera)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new MatrixCamera CloneCurrentValue() { return (MatrixCamera)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ViewMatrixPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { MatrixCamera target = ((MatrixCamera) d); target.PropertyChanged(ViewMatrixProperty); } private static void ProjectionMatrixPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { MatrixCamera target = ((MatrixCamera) d); target.PropertyChanged(ProjectionMatrixProperty); } #region Public Properties ////// ViewMatrix - Matrix3D. Default value is Matrix3D.Identity. /// public Matrix3D ViewMatrix { get { return (Matrix3D) GetValue(ViewMatrixProperty); } set { SetValueInternal(ViewMatrixProperty, value); } } ////// ProjectionMatrix - Matrix3D. Default value is Matrix3D.Identity. /// public Matrix3D ProjectionMatrix { get { return (Matrix3D) GetValue(ProjectionMatrixProperty); } set { SetValueInternal(ProjectionMatrixProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new MatrixCamera(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform3D vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform3D.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Pack & send command packet DUCE.MILCMD_MATRIXCAMERA data; unsafe { data.Type = MILCMD.MilCmdMatrixCamera; data.Handle = _duceResource.GetHandle(channel); data.htransform = hTransform; data.viewMatrix = CompositionResourceManager.Matrix3DToD3DMATRIX(ViewMatrix); data.projectionMatrix = CompositionResourceManager.Matrix3DToD3DMATRIX(ProjectionMatrix); // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_MATRIXCAMERA)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_MATRIXCAMERA)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the MatrixCamera.ViewMatrix property. /// public static readonly DependencyProperty ViewMatrixProperty; ////// The DependencyProperty for the MatrixCamera.ProjectionMatrix property. /// public static readonly DependencyProperty ProjectionMatrixProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Matrix3D s_ViewMatrix = Matrix3D.Identity; internal static Matrix3D s_ProjectionMatrix = Matrix3D.Identity; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static MatrixCamera() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(MatrixCamera); ViewMatrixProperty = RegisterProperty("ViewMatrix", typeof(Matrix3D), typeofThis, Matrix3D.Identity, new PropertyChangedCallback(ViewMatrixPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ProjectionMatrixProperty = RegisterProperty("ProjectionMatrix", typeof(Matrix3D), typeofThis, Matrix3D.Identity, new PropertyChangedCallback(ProjectionMatrixPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
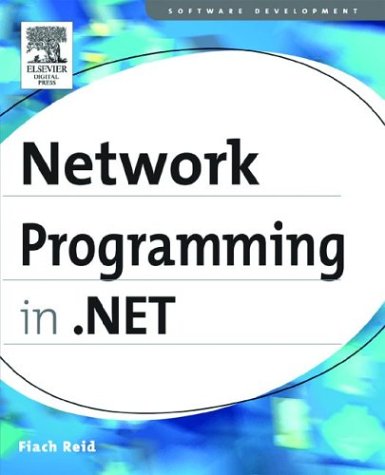
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbConnectionInternal.cs
- WebReferencesBuildProvider.cs
- DecoratedNameAttribute.cs
- PenThread.cs
- IndexExpression.cs
- SQLRoleProvider.cs
- ConfigurationSettings.cs
- AsnEncodedData.cs
- XamlStream.cs
- TdsRecordBufferSetter.cs
- ProfileInfo.cs
- InternalConfigSettingsFactory.cs
- unitconverter.cs
- IProvider.cs
- RoutedPropertyChangedEventArgs.cs
- CodeTypeReference.cs
- SignedPkcs7.cs
- DBCommand.cs
- SubclassTypeValidator.cs
- HtmlForm.cs
- ColorInterpolationModeValidation.cs
- tooltip.cs
- UInt16Storage.cs
- DataTableReader.cs
- ASCIIEncoding.cs
- CoreSwitches.cs
- DataGridLinkButton.cs
- PenCursorManager.cs
- NetworkInterface.cs
- GridViewColumnHeader.cs
- XmlObjectSerializerReadContext.cs
- InvalidOperationException.cs
- BinaryNode.cs
- FacetDescription.cs
- TreeNodeCollection.cs
- MetadataPropertyAttribute.cs
- EdmMember.cs
- ContextStaticAttribute.cs
- Types.cs
- MatrixTransform.cs
- EventListenerClientSide.cs
- MemoryStream.cs
- ReferencedType.cs
- ClassDataContract.cs
- MULTI_QI.cs
- DataGridCellsPresenter.cs
- Table.cs
- RegexMatch.cs
- CapabilitiesState.cs
- StatusBarDesigner.cs
- ProviderUtil.cs
- PageCatalogPart.cs
- Speller.cs
- TextSelectionProcessor.cs
- DataKeyArray.cs
- LinqDataSourceInsertEventArgs.cs
- SerialStream.cs
- XmlUtilWriter.cs
- MobileErrorInfo.cs
- TextBoxLine.cs
- CodeVariableDeclarationStatement.cs
- ObjectStateManager.cs
- NamespaceExpr.cs
- Automation.cs
- ByteStream.cs
- CacheMemory.cs
- Html32TextWriter.cs
- SoapSchemaExporter.cs
- CustomErrorsSectionWrapper.cs
- Binding.cs
- ListItem.cs
- ProxyGenerationError.cs
- BrushConverter.cs
- SmiSettersStream.cs
- AssociationSet.cs
- ExpressionBinding.cs
- HuffmanTree.cs
- ReservationNotFoundException.cs
- ListItemCollection.cs
- DbUpdateCommandTree.cs
- SecurityToken.cs
- DoubleAnimationClockResource.cs
- DataBoundControlAdapter.cs
- Helper.cs
- FilterElement.cs
- XmlWriterTraceListener.cs
- EllipticalNodeOperations.cs
- DataGridViewControlCollection.cs
- Grid.cs
- SafeNativeMethodsMilCoreApi.cs
- unsafenativemethodsother.cs
- Style.cs
- PreviewPageInfo.cs
- GCHandleCookieTable.cs
- ActivityDesignerResources.cs
- NativeCppClassAttribute.cs
- Dictionary.cs
- DataGridViewRowStateChangedEventArgs.cs
- ArrayElementGridEntry.cs
- CalendarButton.cs