Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / ListItemCollection.cs / 1 / ListItemCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.ListItemsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ListItemCollection : ICollection, IList, IStateManager { private ArrayList listItems; private bool marked; private bool saveAll; ///Encapsulates the items within a ///. /// This class cannot be inherited. /// Initializes a new instance of the /// public ListItemCollection() { listItems = new ArrayList(); marked = false; saveAll = false; } ///class. /// /// public ListItem this[int index] { get { return (ListItem)listItems[index]; } } ///Gets a ///referenced by the specified ordinal /// index value. object IList.this[int index] { get { return listItems[index]; } set { listItems[index] = (ListItem)value; } } public int Capacity { get { return listItems.Capacity; } set { listItems.Capacity = value; } } /// /// public int Count { get { return listItems.Count; } } ///Gets the item count of the collection. ////// public void Add(string item) { Add(new ListItem(item)); } ///Adds the specified item to the end of the collection. ////// public void Add(ListItem item) { listItems.Add(item); if (marked) { item.Dirty = true; } } ///Adds the specified ///to the end of the collection. int IList.Add(object item) { ListItem newItem = (ListItem) item; int returnValue = listItems.Add(newItem); if (marked) { newItem.Dirty = true; } return returnValue; } /// /// public void AddRange(ListItem[] items) { if (items == null) { throw new ArgumentNullException("items"); } foreach(ListItem item in items) { Add(item); } } ////// public void Clear() { listItems.Clear(); if (marked) saveAll = true; } ///Removes all ///controls from the collection. /// public bool Contains(ListItem item) { return listItems.Contains(item); } ///Returns a value indicating whether the /// collection contains the specified item. ///bool IList.Contains(object item) { return Contains((ListItem) item); } /// /// public void CopyTo(Array array, int index) { listItems.CopyTo(array,index); } public ListItem FindByText(string text) { int index = FindByTextInternal(text, true); if (index != -1) { return (ListItem)listItems[index]; } return null; } internal int FindByTextInternal(string text, bool includeDisabled) { int i = 0; foreach (ListItem item in listItems) { if (item.Text.Equals(text) && (includeDisabled || item.Enabled)) { return i; } i++; } return -1; } public ListItem FindByValue(string value) { int index = FindByValueInternal(value, true); if (index != -1) { return (ListItem)listItems[index]; } return null; } internal int FindByValueInternal(string value, bool includeDisabled) { int i = 0; foreach (ListItem item in listItems) { if (item.Value.Equals(value) && (includeDisabled || item.Enabled)) { return i; } i++; } return -1; } ///Copies contents from the collection to a specified ///with a /// specified starting index. /// public IEnumerator GetEnumerator() { return listItems.GetEnumerator(); } ///Returns an enumerator of all ///controls within the /// collection. /// public int IndexOf(ListItem item) { return listItems.IndexOf(item); } ///Returns an ordinal index value that represents the /// position of the specified ///within the collection. int IList.IndexOf(object item) { return IndexOf((ListItem) item); } /// /// public void Insert(int index,string item) { Insert(index,new ListItem(item)); } ///Adds the specified item to the collection at the specified index /// location. ////// public void Insert(int index,ListItem item) { listItems.Insert(index,item); if (marked) saveAll = true; } ///Inserts the specified ///to the collection at the specified /// index location. void IList.Insert(int index, object item) { Insert(index, (ListItem) item); } /// bool IList.IsFixedSize { get { return false; } } /// /// public bool IsReadOnly { get { return listItems.IsReadOnly; } } ///Gets a value indicating whether the collection is read-only. ////// public bool IsSynchronized { get { return listItems.IsSynchronized; } } ///Gets a value indicating whether access to the collection is synchronized /// (thread-safe). ////// public void RemoveAt(int index) { listItems.RemoveAt(index); if (marked) saveAll = true; } ///Removes the ///from the collection at the specified /// index location. /// public void Remove(string item) { int index = IndexOf(new ListItem(item)); if (index >= 0) { RemoveAt(index); } } ///Removes the specified item from the collection. ////// public void Remove(ListItem item) { int index = IndexOf(item); if (index >= 0) { RemoveAt(index); } } ///Removes the specified ///from the collection. void IList.Remove(object item) { Remove((ListItem) item); } /// /// public object SyncRoot { get { return this; } } ///Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. ////// /// Return true if tracking state changes. /// Method of private interface, IStateManager. /// bool IStateManager.IsTrackingViewState { get { return marked; } } ///void IStateManager.LoadViewState(object state) { LoadViewState(state); } internal void LoadViewState(object state) { if (state != null) { if (state is Pair) { // only changed items were saved Pair p = (Pair) state; ArrayList indices = (ArrayList) p.First; ArrayList items = (ArrayList) p.Second; for (int i=0; i void IStateManager.TrackViewState() { TrackViewState(); } internal void TrackViewState() { marked = true; for (int i=0; i < Count; i++) this[i].TrackViewState(); } /// object IStateManager.SaveViewState() { return SaveViewState(); } internal object SaveViewState() { if (saveAll == true) { // save all items int count = Count; object[] texts = new string[count]; object[] values = new string[count]; bool[] enableds = new bool[count]; for (int i=0; i < count; i++) { texts[i] = this[i].Text; values[i] = this[i].Value; enableds[i] = this[i].Enabled; } return new Triplet(texts, values, enableds); } else { // save only the changed items ArrayList indices = new ArrayList(4); ArrayList items = new ArrayList(4); for (int i=0; i < Count; i++) { object item = this[i].SaveViewState(); if (item != null) { indices.Add(i); items.Add(item); } } if (indices.Count > 0) return new Pair(indices, items); return null; } } } }
Link Menu
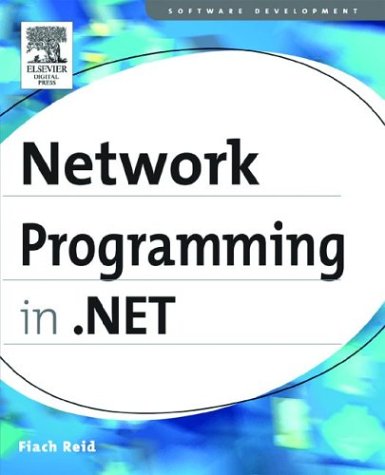
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HybridWebProxyFinder.cs
- DockAndAnchorLayout.cs
- ReliableChannelBinder.cs
- DefaultHttpHandler.cs
- FloaterParagraph.cs
- XmlSchemaAnnotation.cs
- ContextStack.cs
- BulletChrome.cs
- TransactionTraceIdentifier.cs
- SecurityKeyIdentifier.cs
- DirectionalLight.cs
- MetadataArtifactLoader.cs
- ObjectHandle.cs
- XmlSchemaInferenceException.cs
- BitmapVisualManager.cs
- HostedTransportConfigurationManager.cs
- StaticSiteMapProvider.cs
- UInt16Converter.cs
- DrawingAttributes.cs
- MessageSecurityOverHttpElement.cs
- SHA384Managed.cs
- RefreshPropertiesAttribute.cs
- FunctionCommandText.cs
- GeometryDrawing.cs
- ThicknessKeyFrameCollection.cs
- MiniAssembly.cs
- StatusBarItemAutomationPeer.cs
- HandlerBase.cs
- DeviceContexts.cs
- TextBoxBase.cs
- SettingsAttributeDictionary.cs
- WebPartZone.cs
- FlowDocumentView.cs
- KnownBoxes.cs
- DeliveryRequirementsAttribute.cs
- ActivityBuilder.cs
- Base64Stream.cs
- DSACryptoServiceProvider.cs
- ScrollEventArgs.cs
- NumberFormatter.cs
- TimeIntervalCollection.cs
- BoundPropertyEntry.cs
- SQLSingleStorage.cs
- XPathNode.cs
- DataGridDetailsPresenter.cs
- SchemaManager.cs
- ControlCommandSet.cs
- PointCollectionConverter.cs
- HelpEvent.cs
- PathSegmentCollection.cs
- PropertyAccessVisitor.cs
- GridViewRowEventArgs.cs
- List.cs
- VScrollProperties.cs
- ObjectDataSource.cs
- XmlAnyAttributeAttribute.cs
- TCEAdapterGenerator.cs
- CapacityStreamGeometryContext.cs
- BooleanExpr.cs
- HttpGetProtocolReflector.cs
- ContextInformation.cs
- EnumType.cs
- DLinqTableProvider.cs
- BlurBitmapEffect.cs
- CompositeCollection.cs
- TableRow.cs
- UndirectedGraph.cs
- PartialArray.cs
- PartDesigner.cs
- EventHandlerList.cs
- SelectionRange.cs
- FrameDimension.cs
- XsdDataContractExporter.cs
- ArgumentsParser.cs
- DiffuseMaterial.cs
- WebConfigurationFileMap.cs
- Expander.cs
- DataControlFieldCell.cs
- DesignerForm.cs
- PropertyGridCommands.cs
- AppDomainProtocolHandler.cs
- CacheEntry.cs
- CrossSiteScriptingValidation.cs
- BridgeDataRecord.cs
- Path.cs
- _AutoWebProxyScriptWrapper.cs
- TranslateTransform.cs
- ImageListUtils.cs
- ControlHelper.cs
- PropertyMetadata.cs
- BitmapMetadata.cs
- XmlBinaryReader.cs
- HtmlWindowCollection.cs
- DataGridViewCellPaintingEventArgs.cs
- XmlLanguageConverter.cs
- Automation.cs
- StatusBarDrawItemEvent.cs
- OdbcTransaction.cs
- FaultPropagationRecord.cs
- ToolBarTray.cs