Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / SqlClient / TdsRecordBufferSetter.cs / 4 / TdsRecordBufferSetter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using Microsoft.SqlServer.Server; using System; using System.Data; using System.Data.SqlClient; using System.Diagnostics; using System.Data.SqlTypes; // TdsRecordBufferSetter handles writing a structured value out to a TDS stream internal class TdsRecordBufferSetter : SmiRecordBuffer { #region Fields (private) private TdsValueSetter[] _fieldSetters; // setters for individual fields private TdsParserStateObject _stateObj; // target to write to private SmiMetaData _metaData; // metadata describing value #if DEBUG private const int ReadyForToken = -1; // must call new/end element next private const int EndElementsCalled = -2; // already called EndElements, can only call Close private const int Closed = -3; // closed (zombied) private int _currentField; // validate that caller sets columns in correct order. #endif #endregion #region Exposed Construct and control methods/properties internal TdsRecordBufferSetter(TdsParserStateObject stateObj, SmiMetaData md) { Debug.Assert(SqlDbType.Structured == md.SqlDbType, "Unsupported SqlDbType: " + md.SqlDbType); _fieldSetters = new TdsValueSetter[md.FieldMetaData.Count]; for(int i=0; i_currentField, "_currentField out of range for setting a column:" + _currentField); Debug.Assert(ordinal == _currentField, "Setter called out of order. Should be " + _currentField + ", but was " + ordinal); // Must not write to field with a DefaultFieldsProperty set to true Debug.Assert(!((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[ordinal], "Attempt to write to a default-valued field: " + ordinal); #endif } // Handle logic of skipping default columns [Conditional("DEBUG")] private void SkipPossibleDefaultedColumns(int targetColumn) { #if DEBUG Debug.Assert(targetColumn < _metaData.FieldMetaData.Count && targetColumn >= ReadyForToken, "TdsRecordBufferSetter.SkipPossibleDefaultedColumns: Invalid target column: " + targetColumn); // special setup for ReadyForToken as the target if (targetColumn == ReadyForToken) { if (ReadyForToken == _currentField) { return; } // Handle readyfortoken by using count of columns in the loop. targetColumn = _metaData.FieldMetaData.Count; } // Handle skipping default-valued fields while (targetColumn > _currentField) { // All intermediate fields must be default fields (i.e. have a "true" entry in SmiDefaultFieldsProperty Debug.Assert(((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[_currentField], "Skipping a field that was not default: " + _currentField); _currentField++; } if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } [Conditional("DEBUG")] internal void CheckSettingColumn(int ordinal) { #if DEBUG // Make sure target column can be written to. CheckWritingToColumn(ordinal); _currentField++; if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using Microsoft.SqlServer.Server; using System; using System.Data; using System.Data.SqlClient; using System.Diagnostics; using System.Data.SqlTypes; // TdsRecordBufferSetter handles writing a structured value out to a TDS stream internal class TdsRecordBufferSetter : SmiRecordBuffer { #region Fields (private) private TdsValueSetter[] _fieldSetters; // setters for individual fields private TdsParserStateObject _stateObj; // target to write to private SmiMetaData _metaData; // metadata describing value #if DEBUG private const int ReadyForToken = -1; // must call new/end element next private const int EndElementsCalled = -2; // already called EndElements, can only call Close private const int Closed = -3; // closed (zombied) private int _currentField; // validate that caller sets columns in correct order. #endif #endregion #region Exposed Construct and control methods/properties internal TdsRecordBufferSetter(TdsParserStateObject stateObj, SmiMetaData md) { Debug.Assert(SqlDbType.Structured == md.SqlDbType, "Unsupported SqlDbType: " + md.SqlDbType); _fieldSetters = new TdsValueSetter[md.FieldMetaData.Count]; for(int i=0; i_currentField, "_currentField out of range for setting a column:" + _currentField); Debug.Assert(ordinal == _currentField, "Setter called out of order. Should be " + _currentField + ", but was " + ordinal); // Must not write to field with a DefaultFieldsProperty set to true Debug.Assert(!((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[ordinal], "Attempt to write to a default-valued field: " + ordinal); #endif } // Handle logic of skipping default columns [Conditional("DEBUG")] private void SkipPossibleDefaultedColumns(int targetColumn) { #if DEBUG Debug.Assert(targetColumn < _metaData.FieldMetaData.Count && targetColumn >= ReadyForToken, "TdsRecordBufferSetter.SkipPossibleDefaultedColumns: Invalid target column: " + targetColumn); // special setup for ReadyForToken as the target if (targetColumn == ReadyForToken) { if (ReadyForToken == _currentField) { return; } // Handle readyfortoken by using count of columns in the loop. targetColumn = _metaData.FieldMetaData.Count; } // Handle skipping default-valued fields while (targetColumn > _currentField) { // All intermediate fields must be default fields (i.e. have a "true" entry in SmiDefaultFieldsProperty Debug.Assert(((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[_currentField], "Skipping a field that was not default: " + _currentField); _currentField++; } if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } [Conditional("DEBUG")] internal void CheckSettingColumn(int ordinal) { #if DEBUG // Make sure target column can be written to. CheckWritingToColumn(ordinal); _currentField++; if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
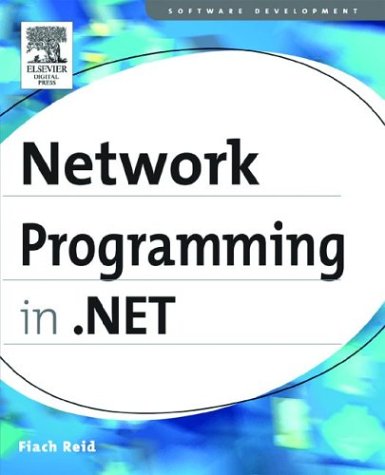
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyTab.cs
- FontInfo.cs
- XsdCachingReader.cs
- CodeTypeParameterCollection.cs
- HtmlWindowCollection.cs
- PeerCollaboration.cs
- CommonProperties.cs
- RepeaterItemCollection.cs
- DecimalAnimationUsingKeyFrames.cs
- Thread.cs
- ExtendedPropertyDescriptor.cs
- FlowNode.cs
- BitmapEffect.cs
- ResetableIterator.cs
- CompositeFontInfo.cs
- CounterSample.cs
- ObjectDataSourceMethodEditor.cs
- MimeTypePropertyAttribute.cs
- QilLoop.cs
- BamlMapTable.cs
- ListViewEditEventArgs.cs
- CanExpandCollapseAllConverter.cs
- DataTemplateKey.cs
- PngBitmapEncoder.cs
- _TLSstream.cs
- DbConnectionFactory.cs
- SkewTransform.cs
- DockPatternIdentifiers.cs
- SrgsRuleRef.cs
- Point3DIndependentAnimationStorage.cs
- FixedDocumentPaginator.cs
- CounterSampleCalculator.cs
- TraceSection.cs
- EntityKeyElement.cs
- TextReturnReader.cs
- IgnoreSectionHandler.cs
- XmlIterators.cs
- InfoCardBaseException.cs
- SqlServices.cs
- XmlILStorageConverter.cs
- SystemKeyConverter.cs
- MetadataSection.cs
- RepeatInfo.cs
- SqlConnection.cs
- Mouse.cs
- ChangeTracker.cs
- TextPointer.cs
- Page.cs
- ToolStripDropDownClosedEventArgs.cs
- MatrixCamera.cs
- ModuleElement.cs
- MasterPage.cs
- CodePropertyReferenceExpression.cs
- ClientConfigurationHost.cs
- NetDispatcherFaultException.cs
- PrincipalPermission.cs
- httpstaticobjectscollection.cs
- EvidenceTypeDescriptor.cs
- XsltQilFactory.cs
- OutputChannel.cs
- DataGridViewCellStyleConverter.cs
- Soap12FormatExtensions.cs
- ChildTable.cs
- DbConnectionPoolGroupProviderInfo.cs
- ConfigurationSection.cs
- Operator.cs
- ToolStripManager.cs
- WindowsEditBoxRange.cs
- PageRequestManager.cs
- PixelFormatConverter.cs
- UseAttributeSetsAction.cs
- TextTreeDeleteContentUndoUnit.cs
- Events.cs
- StreamReader.cs
- CodeEventReferenceExpression.cs
- BindingSource.cs
- EdmType.cs
- MetaModel.cs
- GridViewColumnCollection.cs
- DefaultTraceListener.cs
- DataError.cs
- WMIGenerator.cs
- EncryptedType.cs
- FlowchartSizeFeature.cs
- SocketInformation.cs
- ConnectorSelectionGlyph.cs
- PreloadedPackages.cs
- ApplicationHost.cs
- AdjustableArrowCap.cs
- PointHitTestResult.cs
- XmlSchemaExternal.cs
- CapabilitiesRule.cs
- TypeBuilder.cs
- ListView.cs
- MsmqDecodeHelper.cs
- Journal.cs
- AutoGeneratedFieldProperties.cs
- PeerApplicationLaunchInfo.cs
- HttpCookie.cs
- Method.cs