Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / PtsHost / SectionVisual.cs / 1305600 / SectionVisual.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SectionVisual.cs // // Description: Visual representing a section. // // History: // 05/20/2003 : [....] - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Threading; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Visual representing a section. // --------------------------------------------------------------------- internal class SectionVisual : DrawingVisual { // ------------------------------------------------------------------ // Constructor. // ----------------------------------------------------------------- internal SectionVisual() { } // ------------------------------------------------------------------ // Set information about column rules that are necessary for rendering // process. Draw column rules if necessary. // // arrayColumnDesc - column description including rectangle coordinates, etc // columnVStart - vertical start coordinate of column rule // columnHeight - height of column rule // columnProperties - column properties. // ------------------------------------------------------------------ internal void DrawColumnRules(ref PTS.FSTRACKDESCRIPTION[] arrayColumnDesc, double columnVStart, double columnHeight, ColumnPropertiesGroup columnProperties) { // Compute column rules data first. Point[] rulePositions = null; double ruleWidth = columnProperties.ColumnRuleWidth; if (arrayColumnDesc.Length > 1) { if (ruleWidth > 0) { int gapWidth = (arrayColumnDesc[1].fsrc.u - (arrayColumnDesc[0].fsrc.u + arrayColumnDesc[0].fsrc.du)) / 2; rulePositions = new Point[(arrayColumnDesc.Length - 1)*2]; for (int index = 1; index < arrayColumnDesc.Length; index++) { double u = TextDpi.FromTextDpi(arrayColumnDesc[index].fsrc.u - gapWidth); double v = columnVStart; double dv = columnHeight; rulePositions[(index-1)*2].X = u; rulePositions[(index-1)*2].Y = v; rulePositions[(index-1)*2+1].X = u; rulePositions[(index-1)*2+1].Y = v + dv; } } } // Check if update of the visual render data is needed. bool needsUpdate = _ruleWidth != ruleWidth; if (!needsUpdate && _rulePositions != rulePositions) { int prevSize = _rulePositions == null ? 0 : _rulePositions.Length; int newSize = rulePositions == null ? 0 : rulePositions.Length; if (prevSize == newSize) { for (int index = 0; index < rulePositions.Length; index++) { if (!DoubleUtil.AreClose(rulePositions[index].X, _rulePositions[index].X) || !DoubleUtil.AreClose(rulePositions[index].Y, _rulePositions[index].Y)) { needsUpdate = true; break; } } } else { needsUpdate = true; } } // Draw column rules if necessary if (needsUpdate) { _ruleWidth = ruleWidth; _rulePositions = rulePositions; // Open DrawingContext and draw background. // If background is not set, Open will clear the render data, but it // will preserve visual children. using (DrawingContext dc = RenderOpen()) { if (rulePositions != null) { // We do not want to cause the user's Brush to become frozen when we // freeze pen below, therefore we make our own copy of the Brush if // it is not already frozen. Brush columnRuleBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(columnProperties.ColumnRuleBrush); Pen pen = new Pen(columnRuleBrush, ruleWidth); // Freeze the pen if possible. Doing this avoids the overhead of // maintaining changed handlers. if (pen.CanFreeze) { pen.Freeze(); } for (int index = 0; index < rulePositions.Length; index += 2) { dc.DrawLine(pen, rulePositions[index], rulePositions[index + 1]); } } } } } // ----------------------------------------------------------------- // Column rules positions // ------------------------------------------------------------------ private Point[] _rulePositions; // ----------------------------------------------------------------- // Pen for column rule. // ----------------------------------------------------------------- private double _ruleWidth; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SectionVisual.cs // // Description: Visual representing a section. // // History: // 05/20/2003 : [....] - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Threading; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Visual representing a section. // --------------------------------------------------------------------- internal class SectionVisual : DrawingVisual { // ------------------------------------------------------------------ // Constructor. // ----------------------------------------------------------------- internal SectionVisual() { } // ------------------------------------------------------------------ // Set information about column rules that are necessary for rendering // process. Draw column rules if necessary. // // arrayColumnDesc - column description including rectangle coordinates, etc // columnVStart - vertical start coordinate of column rule // columnHeight - height of column rule // columnProperties - column properties. // ------------------------------------------------------------------ internal void DrawColumnRules(ref PTS.FSTRACKDESCRIPTION[] arrayColumnDesc, double columnVStart, double columnHeight, ColumnPropertiesGroup columnProperties) { // Compute column rules data first. Point[] rulePositions = null; double ruleWidth = columnProperties.ColumnRuleWidth; if (arrayColumnDesc.Length > 1) { if (ruleWidth > 0) { int gapWidth = (arrayColumnDesc[1].fsrc.u - (arrayColumnDesc[0].fsrc.u + arrayColumnDesc[0].fsrc.du)) / 2; rulePositions = new Point[(arrayColumnDesc.Length - 1)*2]; for (int index = 1; index < arrayColumnDesc.Length; index++) { double u = TextDpi.FromTextDpi(arrayColumnDesc[index].fsrc.u - gapWidth); double v = columnVStart; double dv = columnHeight; rulePositions[(index-1)*2].X = u; rulePositions[(index-1)*2].Y = v; rulePositions[(index-1)*2+1].X = u; rulePositions[(index-1)*2+1].Y = v + dv; } } } // Check if update of the visual render data is needed. bool needsUpdate = _ruleWidth != ruleWidth; if (!needsUpdate && _rulePositions != rulePositions) { int prevSize = _rulePositions == null ? 0 : _rulePositions.Length; int newSize = rulePositions == null ? 0 : rulePositions.Length; if (prevSize == newSize) { for (int index = 0; index < rulePositions.Length; index++) { if (!DoubleUtil.AreClose(rulePositions[index].X, _rulePositions[index].X) || !DoubleUtil.AreClose(rulePositions[index].Y, _rulePositions[index].Y)) { needsUpdate = true; break; } } } else { needsUpdate = true; } } // Draw column rules if necessary if (needsUpdate) { _ruleWidth = ruleWidth; _rulePositions = rulePositions; // Open DrawingContext and draw background. // If background is not set, Open will clear the render data, but it // will preserve visual children. using (DrawingContext dc = RenderOpen()) { if (rulePositions != null) { // We do not want to cause the user's Brush to become frozen when we // freeze pen below, therefore we make our own copy of the Brush if // it is not already frozen. Brush columnRuleBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(columnProperties.ColumnRuleBrush); Pen pen = new Pen(columnRuleBrush, ruleWidth); // Freeze the pen if possible. Doing this avoids the overhead of // maintaining changed handlers. if (pen.CanFreeze) { pen.Freeze(); } for (int index = 0; index < rulePositions.Length; index += 2) { dc.DrawLine(pen, rulePositions[index], rulePositions[index + 1]); } } } } } // ----------------------------------------------------------------- // Column rules positions // ------------------------------------------------------------------ private Point[] _rulePositions; // ----------------------------------------------------------------- // Pen for column rule. // ----------------------------------------------------------------- private double _ruleWidth; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
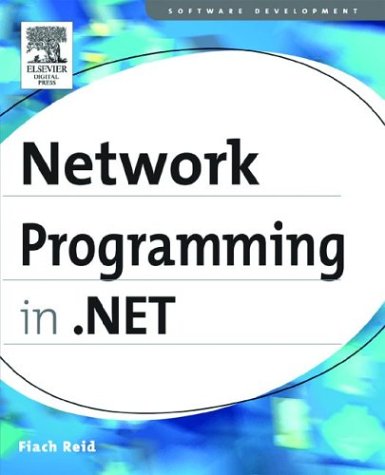
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IndexerReference.cs
- BindingExpressionUncommonField.cs
- OrCondition.cs
- XmlSchemaGroupRef.cs
- HatchBrush.cs
- Timer.cs
- HttpModuleAction.cs
- DataMemberListEditor.cs
- IPEndPoint.cs
- SatelliteContractVersionAttribute.cs
- PtsHost.cs
- NCryptSafeHandles.cs
- DocumentDesigner.cs
- InvokeMemberBinder.cs
- RadioButtonList.cs
- CacheModeValueSerializer.cs
- CompressStream.cs
- OdbcCommandBuilder.cs
- ComponentTray.cs
- GroupBoxAutomationPeer.cs
- XsdDateTime.cs
- ComponentEditorForm.cs
- OleDbPermission.cs
- DiscoveryMessageSequence11.cs
- OpCodes.cs
- TableCellAutomationPeer.cs
- BindStream.cs
- GenericUriParser.cs
- datacache.cs
- XmlSerializationGeneratedCode.cs
- XmlSchemas.cs
- TryCatch.cs
- Mutex.cs
- SqlDataRecord.cs
- GridViewRowPresenter.cs
- TableItemPattern.cs
- ReflectTypeDescriptionProvider.cs
- DesignerAutoFormatCollection.cs
- TextFormatterHost.cs
- AddInPipelineAttributes.cs
- KeyFrames.cs
- AppSettingsSection.cs
- Deserializer.cs
- SynchronizationHandlesCodeDomSerializer.cs
- ReturnEventArgs.cs
- LogArchiveSnapshot.cs
- Clipboard.cs
- ListControl.cs
- WorkflowItemsPresenter.cs
- SoapProtocolImporter.cs
- EmissiveMaterial.cs
- ButtonChrome.cs
- AdRotator.cs
- Parameter.cs
- GenerateTemporaryTargetAssembly.cs
- InputElement.cs
- CodeDomSerializationProvider.cs
- InputLanguageManager.cs
- XamlFigureLengthSerializer.cs
- DbDataRecord.cs
- PerformanceCounterPermissionEntryCollection.cs
- FontWeightConverter.cs
- OlePropertyStructs.cs
- SmiEventSink_Default.cs
- RegexGroupCollection.cs
- TreeNodeCollection.cs
- EndpointConfigContainer.cs
- WindowsButton.cs
- DbParameterCollection.cs
- StatusBarItemAutomationPeer.cs
- CorrelationManager.cs
- TextDecorationUnitValidation.cs
- RootBrowserWindow.cs
- EmissiveMaterial.cs
- DispatcherTimer.cs
- MarkedHighlightComponent.cs
- SoapHelper.cs
- BitmapEffectDrawingContextWalker.cs
- MobileCapabilities.cs
- DeviceContexts.cs
- AQNBuilder.cs
- SchemaTypeEmitter.cs
- TextElementEditingBehaviorAttribute.cs
- BooleanAnimationUsingKeyFrames.cs
- DataRecordInternal.cs
- CapacityStreamGeometryContext.cs
- MissingMethodException.cs
- ToolboxItem.cs
- SafeHandle.cs
- SecureUICommand.cs
- QuaternionAnimation.cs
- DateTimeFormatInfoScanner.cs
- ExtensionWindow.cs
- DbConnectionFactory.cs
- TraceUtils.cs
- RsaKeyIdentifierClause.cs
- DomainUpDown.cs
- NullableConverter.cs
- ModuleBuilderData.cs
- GeneratedCodeAttribute.cs