Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / FontWeightConverter.cs / 1 / FontWeightConverter.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontWeight type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontWeightConverter class parses a font weight string. /// public sealed class FontWeightConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontWeight from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontWeight. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontWeight fontWeight = new FontWeight(); if (!FontWeights.FontWeightStringToKnownWeight(s, ci, ref fontWeight)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontWeight; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontWeight, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontWeight, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontWeight) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(FontWeight).GetMethod("FromOpenTypeWeight", new Type[]{typeof(int)}); FontWeight c = (FontWeight)value; return new InstanceDescriptor(mi, new object[]{c.ToOpenTypeWeight()}); } else if (destinationType == typeof(string)) { FontWeight c = (FontWeight)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontWeight type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontWeightConverter class parses a font weight string. /// public sealed class FontWeightConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontWeight from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontWeight. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontWeight fontWeight = new FontWeight(); if (!FontWeights.FontWeightStringToKnownWeight(s, ci, ref fontWeight)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontWeight; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontWeight, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontWeight, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontWeight) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(FontWeight).GetMethod("FromOpenTypeWeight", new Type[]{typeof(int)}); FontWeight c = (FontWeight)value; return new InstanceDescriptor(mi, new object[]{c.ToOpenTypeWeight()}); } else if (destinationType == typeof(string)) { FontWeight c = (FontWeight)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
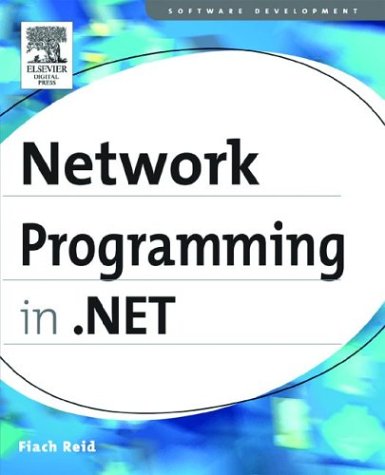
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LocalizationParserHooks.cs
- ObservableDictionary.cs
- CodeMethodReturnStatement.cs
- MethodBuilderInstantiation.cs
- TreeViewEvent.cs
- DetailsViewDeletedEventArgs.cs
- MenuAdapter.cs
- HtmlControlPersistable.cs
- ViewManager.cs
- PrinterUnitConvert.cs
- XmlSchemaIdentityConstraint.cs
- StylusButton.cs
- NavigationProgressEventArgs.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- __FastResourceComparer.cs
- CreateUserWizardStep.cs
- MgmtConfigurationRecord.cs
- EventProviderWriter.cs
- XmlDeclaration.cs
- OutputCacheSettings.cs
- ConfigurationLocation.cs
- EdmScalarPropertyAttribute.cs
- SoapFormatter.cs
- NeutralResourcesLanguageAttribute.cs
- ExceptionRoutedEventArgs.cs
- DynamicResourceExtensionConverter.cs
- JsonXmlDataContract.cs
- Filter.cs
- SqlUDTStorage.cs
- cookie.cs
- CultureInfo.cs
- ManagedWndProcTracker.cs
- SqlWriter.cs
- EntityWrapperFactory.cs
- Solver.cs
- EntityRecordInfo.cs
- NativeMethods.cs
- PartialCachingControl.cs
- ClientTargetSection.cs
- LinqToSqlWrapper.cs
- Pipe.cs
- unsafenativemethodstextservices.cs
- ChangeInterceptorAttribute.cs
- DataListItem.cs
- ErrorWrapper.cs
- ResourceAssociationSet.cs
- HandoffBehavior.cs
- ConstructorNeedsTagAttribute.cs
- UserControlParser.cs
- CheckPair.cs
- PeerCredential.cs
- WindowsUpDown.cs
- HelpProvider.cs
- PageVisual.cs
- MimeMultiPart.cs
- NamespaceMapping.cs
- Base64Stream.cs
- SoapCodeExporter.cs
- CodeAccessPermission.cs
- ParallelActivityDesigner.cs
- AssertSection.cs
- PointHitTestParameters.cs
- XamlGridLengthSerializer.cs
- ServiceObjectContainer.cs
- BindingContext.cs
- AsymmetricSignatureDeformatter.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- DeferredTextReference.cs
- Menu.cs
- DistributedTransactionPermission.cs
- StringWriter.cs
- BehaviorEditorPart.cs
- ConnectionStringsSection.cs
- ContentOperations.cs
- CollectionChangeEventArgs.cs
- ActiveDocumentEvent.cs
- CodeDomSerializer.cs
- AssemblyHash.cs
- LateBoundBitmapDecoder.cs
- CultureTable.cs
- EntityParameterCollection.cs
- SqlBuffer.cs
- BaseParser.cs
- ModelTypeConverter.cs
- IdnMapping.cs
- EncoderParameters.cs
- LineBreak.cs
- ResolveCompletedEventArgs.cs
- XmlNodeChangedEventArgs.cs
- FormsAuthenticationModule.cs
- SoapEnvelopeProcessingElement.cs
- DataRow.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- mda.cs
- documentsequencetextcontainer.cs
- DbDataReader.cs
- CheckBox.cs
- DecoratedNameAttribute.cs
- ListViewCancelEventArgs.cs
- DataListCommandEventArgs.cs