Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / InputOutput / Ports.cs / 2 / Ports.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file implements utility functions relating to messaging using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.Globalization; using System.ServiceModel; using System.Security.Cryptography; using System.Text; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; using ServiceModelDiagnosticUtility = System.ServiceModel.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.InputOutput { static class Ports { public static Guid GetGuidFromTransactionId (string transactionId) { // E.g. "a01b8bc6781641c4b138c1acdcc940ef" const int minGuidLength = 32; int minTransactionIdLength = CoordinationContext.UuidScheme.Length + minGuidLength; if (transactionId.Length >= minTransactionIdLength && transactionId.StartsWith(CoordinationContext.UuidScheme, StringComparison.OrdinalIgnoreCase)) { Guid txIdGuid; if (ServiceModelDiagnosticUtility.Utility.TryCreateGuid( transactionId.Substring(CoordinationContext.UuidScheme.Length), out txIdGuid)) { return txIdGuid; } } // // Interop path // // Create a guid deterministically, using the transaction id as input // For obvious reasons, we need a 128-bit hash. // We can't use entropy because we want to be deterministic. Collisions are only fatal // if they occur often enough to coincide in a log. // // We do this to avoid deadlocks in RMs who might participant in the same // transaction through WS-AT and some other protocol that doesn't know about // the WS-AT specific id HashAlgorithm hash = SHA256.Create(); using (hash) { hash.Initialize(); byte[] buffer = Encoding.UTF8.GetBytes(transactionId); byte[] fullHash = hash.ComputeHash(buffer); // Grab the first 128 bits off the hash - see MB 40808 for more details byte[] hash128 = new byte[16]; Array.Copy(fullHash, hash128, hash128.Length); return new Guid(hash128); } } // // Addresses // public static string TryGetAddress(Proxy proxy) { if (proxy == null) { return "unknown"; } return proxy.To.Uri.AbsoluteUri; } public static string TryGetFromAddress(Message message) { string address; EndpointAddress replyTo = Library.GetReplyToHeader(message.Headers); if (replyTo != null) { address = replyTo.Uri.AbsoluteUri; if (address != null) { return address; } } return "unknown"; } public static bool TryGetEnlistment(Message message, out Guid enlistmentId, out ControlProtocol protocol) { enlistmentId = Guid.Empty; protocol = ControlProtocol.None; try { if (!EnlistmentHeader.ReadFrom(message, out enlistmentId, out protocol)) { return false; } } catch (InvalidEnlistmentHeaderException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); return false; } return true; } public static bool TryGetEnlistment(Message message, out Guid enlistmentId) { ControlProtocol dontCare; return TryGetEnlistment(message, out enlistmentId, out dontCare); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
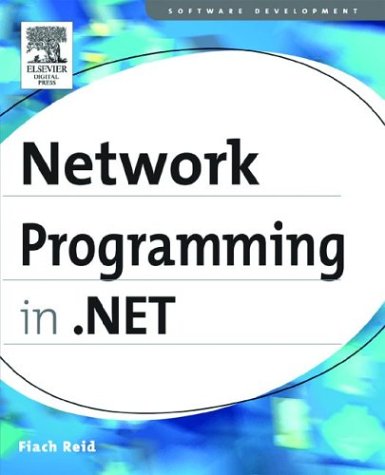
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlTypeMapping.cs
- DataSysAttribute.cs
- SimplePropertyEntry.cs
- RegularExpressionValidator.cs
- GradientStopCollection.cs
- SafeBitVector32.cs
- PrePrepareMethodAttribute.cs
- ConfigXmlComment.cs
- TextSelectionProcessor.cs
- CanExecuteRoutedEventArgs.cs
- WebPartConnection.cs
- SHA512Cng.cs
- AccessedThroughPropertyAttribute.cs
- TagPrefixAttribute.cs
- StaticContext.cs
- ToolBarDesigner.cs
- IItemProperties.cs
- NodeLabelEditEvent.cs
- AliasExpr.cs
- ExpressionBuilderCollection.cs
- StaticResourceExtension.cs
- RTLAwareMessageBox.cs
- HtmlTextBoxAdapter.cs
- ReliableMessagingVersionConverter.cs
- SQLInt16Storage.cs
- FileChangesMonitor.cs
- StorageMappingItemLoader.cs
- CommonObjectSecurity.cs
- MarkupExtensionReturnTypeAttribute.cs
- FileCodeGroup.cs
- SqlParameter.cs
- DateTimeFormatInfoScanner.cs
- ADMembershipProvider.cs
- TypeNameHelper.cs
- RemoteWebConfigurationHost.cs
- TagPrefixCollection.cs
- ResetableIterator.cs
- ControlCollection.cs
- InfoCardTraceRecord.cs
- HttpRuntimeSection.cs
- TraceContextRecord.cs
- Bold.cs
- AttributeUsageAttribute.cs
- DesignerVerbCollection.cs
- HttpRuntime.cs
- XmlSchemaDocumentation.cs
- PseudoWebRequest.cs
- Emitter.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- CacheVirtualItemsEvent.cs
- MimeReturn.cs
- OutOfProcStateClientManager.cs
- PluralizationService.cs
- _Win32.cs
- ModelServiceImpl.cs
- filewebresponse.cs
- ICspAsymmetricAlgorithm.cs
- DataGridViewRowPrePaintEventArgs.cs
- Helpers.cs
- SQLBinary.cs
- FontInfo.cs
- DoubleLinkList.cs
- SubstitutionList.cs
- oledbmetadatacollectionnames.cs
- HashJoinQueryOperatorEnumerator.cs
- DataListItemCollection.cs
- WebPartZone.cs
- FileDialog_Vista_Interop.cs
- Propagator.Evaluator.cs
- SafeBitVector32.cs
- SoapSchemaImporter.cs
- SoapSchemaExporter.cs
- RadioButtonFlatAdapter.cs
- SequenceDesigner.cs
- ValidationHelper.cs
- GetImportedCardRequest.cs
- SafeSystemMetrics.cs
- DataControlField.cs
- DataGridRowEventArgs.cs
- SoundPlayer.cs
- UniformGrid.cs
- SQLBinary.cs
- safelinkcollection.cs
- ThousandthOfEmRealDoubles.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ListenerElementsCollection.cs
- TemplateParser.cs
- CodeGeneratorOptions.cs
- XmlObjectSerializerReadContextComplexJson.cs
- CompilerGeneratedAttribute.cs
- _ChunkParse.cs
- JumpPath.cs
- WebControlParameterProxy.cs
- SubpageParagraph.cs
- PageAsyncTaskManager.cs
- SmiSettersStream.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- Automation.cs
- CompilerGeneratedAttribute.cs
- SpellerError.cs