Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / HttpNamespaceReservationInstallComponent.cs / 1 / HttpNamespaceReservationInstallComponent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using System.ComponentModel; using System.Runtime.InteropServices; class HttpNamespaceReservationInstallComponent : ServiceModelInstallComponent { internal override string DisplayName { get {return SR.GetString(SR.HttpNamespaceReservationsName); } } protected override string InstallActionMessage { get {return SR.GetString(SR.HttpNamespaceReservationsInstall); } } internal override string[] InstalledVersions { get { string[] result; if (this.IsInstalled) { result = new string[] {ServiceModelInstallStrings.ReservedNamespace}; } else { result = new string[] {}; } return result; } } internal override bool IsInstalled { get { bool result; try { int retVal = NativeMethods.HttpInitialize(NativeMethods.HTTPAPI_VERSION, NativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); if (retVal != ErrorCodes.NoError) { #pragma warning suppress 56503 // [....]; not a publicly accessible API throw new Win32Exception(retVal); } HTTP_SERVICE_CONFIG_URLACL_QUERY query = new HTTP_SERVICE_CONFIG_URLACL_QUERY(); query.QueryDesc = HTTP_SERVICE_CONFIG_QUERY_TYPE.HttpServiceConfigQueryExact; query.KeyDesc = new HTTP_SERVICE_CONFIG_URLACL_KEY(ServiceModelInstallStrings.ReservedNamespace); int returnLength; retVal = NativeMethods.HttpQueryServiceConfiguration(IntPtr.Zero, HTTP_SERVICE_CONFIG_ID.HttpServiceConfigUrlAclInfo, ref query, Marshal.SizeOf(query), IntPtr.Zero, 0, out returnLength, IntPtr.Zero); switch (retVal) { case ErrorCodes.NoError: case ErrorCodes.ErrorInsufficientBuffer: result = true; break; case ErrorCodes.ErrorFileNotFound: result = false; break; default: #pragma warning suppress 56503 // [....]; not a publicly accessible API throw new Win32Exception(retVal); } } finally { #pragma warning disable 56523 //[....]; Win32 errors tracked through method return value #pragma warning disable 56031 //[....]; No need to check return value since shutting down NativeMethods.HttpTerminate(NativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); #pragma restore } return result; } } protected override string ReinstallActionMessage { get {return SR.GetString(SR.HttpNamespaceReservationsReinstall); } } protected override string UninstallActionMessage { get {return SR.GetString(SR.HttpNamespaceReservationsUninstall); } } static void FreeURL(string networkURL) { int retVal = ErrorCodes.NoError; try { #pragma warning suppress 56523 //[....]; Win32 errors tracked through method return value retVal = NativeMethods.HttpInitialize(NativeMethods.HTTPAPI_VERSION, NativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); if (ErrorCodes.NoError == retVal) { HTTP_SERVICE_CONFIG_URLACL_KEY urlAclKey = new HTTP_SERVICE_CONFIG_URLACL_KEY(networkURL); HTTP_SERVICE_CONFIG_URLACL_SET configInformation = new HTTP_SERVICE_CONFIG_URLACL_SET(); configInformation.KeyDesc = urlAclKey; int configInformationSize = Marshal.SizeOf(configInformation); #pragma warning suppress 56523 //[....]; Win32 errors tracked through method return value retVal = NativeMethods.HttpDeleteServiceConfiguration(IntPtr.Zero, HTTP_SERVICE_CONFIG_ID.HttpServiceConfigUrlAclInfo, ref configInformation, configInformationSize, IntPtr.Zero); } } finally { #pragma warning disable 56523 //[....]; Win32 errors tracked through method return value #pragma warning disable 56031 //[....]; No need to check return value since shutting down NativeMethods.HttpTerminate(NativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); #pragma restore } if (ErrorCodes.NoError != retVal) { throw new Win32Exception(retVal); } } internal override void Install(OutputLevel outputLevel) { if (this.IsInstalled) { EventLogger.LogWarning(SR.GetString(SR.HttpNamespaceReservationsAlreadyExist), (OutputLevel.Verbose == outputLevel)); } else { bool throwing = true; try { ReserveURL(ServiceModelInstallStrings.ReservedNamespace, ServiceModelInstallStrings.ReservedNamespaceAcl); throwing = false; } finally { if (throwing) { FreeURL(ServiceModelInstallStrings.ReservedNamespace); } } } } static void ReserveURL(string networkURL, string securityDescriptor) { int retVal = ErrorCodes.NoError; try { #pragma warning suppress 56523 //[....]; Win32 errors tracked through method return value retVal = NativeMethods.HttpInitialize(NativeMethods.HTTPAPI_VERSION, NativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); if (ErrorCodes.NoError == retVal) { HTTP_SERVICE_CONFIG_URLACL_KEY keyDesc = new HTTP_SERVICE_CONFIG_URLACL_KEY(networkURL); HTTP_SERVICE_CONFIG_URLACL_PARAM paramDesc = new HTTP_SERVICE_CONFIG_URLACL_PARAM(securityDescriptor); HTTP_SERVICE_CONFIG_URLACL_SET configInformation = new HTTP_SERVICE_CONFIG_URLACL_SET(); configInformation.KeyDesc = keyDesc; configInformation.ParamDesc = paramDesc; int configInformationLength = Marshal.SizeOf(configInformation); #pragma warning suppress 56523 //[....]; Win32 errors tracked through method return value retVal = NativeMethods.HttpSetServiceConfiguration(IntPtr.Zero, HTTP_SERVICE_CONFIG_ID.HttpServiceConfigUrlAclInfo, ref configInformation, configInformationLength, IntPtr.Zero); if (ErrorCodes.ErrorAlreadyExists == retVal) { #pragma warning suppress 56523 //[....]; Win32 errors tracked through method return value retVal = NativeMethods.HttpDeleteServiceConfiguration(IntPtr.Zero, HTTP_SERVICE_CONFIG_ID.HttpServiceConfigUrlAclInfo, ref configInformation, configInformationLength, IntPtr.Zero); if (ErrorCodes.NoError == retVal) { #pragma warning suppress 56523 //[....]; Win32 errors tracked through method return value retVal = NativeMethods.HttpSetServiceConfiguration(IntPtr.Zero, HTTP_SERVICE_CONFIG_ID.HttpServiceConfigUrlAclInfo, ref configInformation, configInformationLength, IntPtr.Zero); } } } } finally { #pragma warning disable 56523 //[....]; Win32 errors tracked through method return value #pragma warning disable 56031 //[....]; No need to check return value since shutting down NativeMethods.HttpTerminate(NativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); #pragma restore } if (ErrorCodes.NoError != retVal) { throw new Win32Exception(retVal); } } internal override void Uninstall(OutputLevel outputLevel) { if (!this.IsInstalled) { EventLogger.LogWarning(SR.GetString(SR.HttpNamespaceReservationsNotInstalled), (OutputLevel.Verbose == outputLevel)); } else { try { FreeURL(ServiceModelInstallStrings.ReservedNamespace); } catch (Win32Exception exception) { EventLogger.LogWarning(SR.GetString(SR.FreeHttpNamespaceFailed, exception), (OutputLevel.Quiet < outputLevel)); } } } internal override InstallationState VerifyInstall() { return this.IsInstalled ? InstallationState.InstalledDefaults : InstallationState.NotInstalled; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
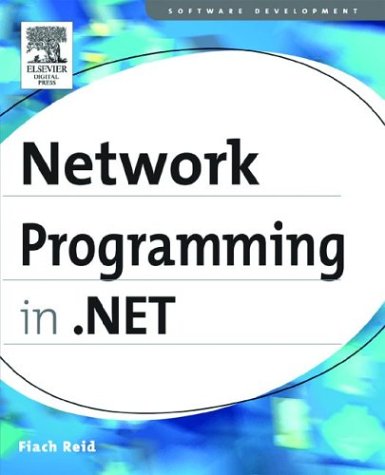
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FacetEnabledSchemaElement.cs
- InvalidContentTypeException.cs
- MetadataPropertyvalue.cs
- ContextBase.cs
- GlyphRunDrawing.cs
- PropertyValidationContext.cs
- SQLDateTimeStorage.cs
- DataGridViewDataConnection.cs
- AccessedThroughPropertyAttribute.cs
- DoubleAnimationBase.cs
- DataBoundControlHelper.cs
- SecurityKeyType.cs
- MenuItemStyleCollection.cs
- OleDbTransaction.cs
- ListControlBoundActionList.cs
- XmlnsPrefixAttribute.cs
- MDIControlStrip.cs
- FolderBrowserDialogDesigner.cs
- BinHexEncoder.cs
- KnownColorTable.cs
- SystemWebExtensionsSectionGroup.cs
- IisTraceWebEventProvider.cs
- QueryPageSettingsEventArgs.cs
- XmlTextEncoder.cs
- StateDesigner.cs
- XmlSchema.cs
- AuthorizationRuleCollection.cs
- ConnectionInterfaceCollection.cs
- CatalogZoneBase.cs
- ArrayList.cs
- _BufferOffsetSize.cs
- WebPartConnectionsCancelEventArgs.cs
- HtmlInputSubmit.cs
- FromReply.cs
- SystemResourceHost.cs
- DataObjectMethodAttribute.cs
- LambdaCompiler.Generated.cs
- DesignerSerializationOptionsAttribute.cs
- FrameworkContentElement.cs
- Rect.cs
- CompilerTypeWithParams.cs
- CursorConverter.cs
- SourceFileInfo.cs
- SqlNodeAnnotation.cs
- TreeNodeEventArgs.cs
- HostExecutionContextManager.cs
- XmlSchemas.cs
- DtdParser.cs
- BinaryParser.cs
- ActivityDesigner.cs
- SoundPlayer.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs
- ControllableStoryboardAction.cs
- SizeAnimationUsingKeyFrames.cs
- SchemaAttDef.cs
- PaintValueEventArgs.cs
- X509Chain.cs
- ExtendedPropertyDescriptor.cs
- EpmCustomContentDeSerializer.cs
- WindowHideOrCloseTracker.cs
- ReadOnlyPermissionSet.cs
- SpAudioStreamWrapper.cs
- StructuredTypeEmitter.cs
- Graph.cs
- StackBuilderSink.cs
- BitmapMetadata.cs
- FamilyMap.cs
- SqlCharStream.cs
- XmlHelper.cs
- DeobfuscatingStream.cs
- controlskin.cs
- EdmEntityTypeAttribute.cs
- HttpFileCollection.cs
- EdmMember.cs
- EditorZone.cs
- bindurihelper.cs
- PersonalizationStateQuery.cs
- _SafeNetHandles.cs
- FileLoadException.cs
- BitmapVisualManager.cs
- StringBuilder.cs
- FlowDocumentReader.cs
- ProfileService.cs
- ZeroOpNode.cs
- DefaultDiscoveryServiceExtension.cs
- InsufficientMemoryException.cs
- Logging.cs
- GlobalProxySelection.cs
- SwitchLevelAttribute.cs
- DesignBindingConverter.cs
- VectorKeyFrameCollection.cs
- FrameworkContextData.cs
- Point3D.cs
- GridViewColumnHeaderAutomationPeer.cs
- DataServiceStreamResponse.cs
- SafeMILHandleMemoryPressure.cs
- prefixendpointaddressmessagefiltertable.cs
- InternalRelationshipCollection.cs
- ConstrainedDataObject.cs
- ImageInfo.cs