Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Media / Animation / ControllableStoryboardAction.cs / 1305600 / ControllableStoryboardAction.cs
/****************************************************************************\ * * File: ControllableStoryboardAction.cs * * This object includes a named Storyboard references. When triggered, the * name is resolved and passed to a derived class method to perform the * actual action. * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.ComponentModel; // DefaultValueAttribute using System.Diagnostics; // Debug.Assert using System.Windows.Documents; // TableTemplate using System.Windows.Markup; // INameScope namespace System.Windows.Media.Animation { ////// A controllable storyboard action associates a trigger action with a /// Storyboard. The association from this object is a string that is the name /// of the Storyboard in a resource dictionary. /// public abstract class ControllableStoryboardAction : TriggerAction { ////// Internal constructor - nobody is supposed to ever create an instance /// of this class. Use a derived class instead. /// internal ControllableStoryboardAction() { } ////// Name to use for resolving the storyboard reference needed. This /// points to a BeginStoryboard instance, and we're controlling that one. /// [DefaultValue(null)] public string BeginStoryboardName { get { return _beginStoryboardName; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "ControllableStoryboardAction")); } // Null is allowed to wipe out existing name - as long as another // valid name is set before Invoke time. _beginStoryboardName = value; } } internal sealed override void Invoke( FrameworkElement fe, FrameworkContentElement fce, Style targetStyle, FrameworkTemplate frameworkTemplate, Int64 layer ) { Debug.Assert( fe != null || fce != null, "Caller of internal function failed to verify that we have a FE or FCE - we have neither." ); Debug.Assert( targetStyle != null || frameworkTemplate != null, "This function expects to be called when the associated action is inside a Style/Template. But it was not given a reference to anything." ); INameScope nameScope = null; if( targetStyle != null ) { nameScope = targetStyle; } else { Debug.Assert( frameworkTemplate != null ); nameScope = frameworkTemplate; } Invoke( fe, fce, GetStoryboard( fe, fce, nameScope ) ); } internal sealed override void Invoke( FrameworkElement fe ) { Debug.Assert( fe != null, "Invoke needs an object as starting point"); Invoke( fe, null, GetStoryboard( fe, null, null ) ); } internal virtual void Invoke( FrameworkElement containingFE, FrameworkContentElement containingFCE, Storyboard storyboard ) { } // Find a Storyboard object for this StoryboardAction to act on, using the // given BeginStoryboardName to find a BeginStoryboard instance and use // its Storyboard object reference. private Storyboard GetStoryboard( FrameworkElement fe, FrameworkContentElement fce, INameScope nameScope ) { if( BeginStoryboardName == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNameRequired)); } BeginStoryboard keyedBeginStoryboard = Storyboard.ResolveBeginStoryboardName( BeginStoryboardName, nameScope, fe, fce ); Storyboard storyboard = keyedBeginStoryboard.Storyboard; if( storyboard == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNoStoryboard, BeginStoryboardName)); } return storyboard; } private string _beginStoryboardName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: ControllableStoryboardAction.cs * * This object includes a named Storyboard references. When triggered, the * name is resolved and passed to a derived class method to perform the * actual action. * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.ComponentModel; // DefaultValueAttribute using System.Diagnostics; // Debug.Assert using System.Windows.Documents; // TableTemplate using System.Windows.Markup; // INameScope namespace System.Windows.Media.Animation { ////// A controllable storyboard action associates a trigger action with a /// Storyboard. The association from this object is a string that is the name /// of the Storyboard in a resource dictionary. /// public abstract class ControllableStoryboardAction : TriggerAction { ////// Internal constructor - nobody is supposed to ever create an instance /// of this class. Use a derived class instead. /// internal ControllableStoryboardAction() { } ////// Name to use for resolving the storyboard reference needed. This /// points to a BeginStoryboard instance, and we're controlling that one. /// [DefaultValue(null)] public string BeginStoryboardName { get { return _beginStoryboardName; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "ControllableStoryboardAction")); } // Null is allowed to wipe out existing name - as long as another // valid name is set before Invoke time. _beginStoryboardName = value; } } internal sealed override void Invoke( FrameworkElement fe, FrameworkContentElement fce, Style targetStyle, FrameworkTemplate frameworkTemplate, Int64 layer ) { Debug.Assert( fe != null || fce != null, "Caller of internal function failed to verify that we have a FE or FCE - we have neither." ); Debug.Assert( targetStyle != null || frameworkTemplate != null, "This function expects to be called when the associated action is inside a Style/Template. But it was not given a reference to anything." ); INameScope nameScope = null; if( targetStyle != null ) { nameScope = targetStyle; } else { Debug.Assert( frameworkTemplate != null ); nameScope = frameworkTemplate; } Invoke( fe, fce, GetStoryboard( fe, fce, nameScope ) ); } internal sealed override void Invoke( FrameworkElement fe ) { Debug.Assert( fe != null, "Invoke needs an object as starting point"); Invoke( fe, null, GetStoryboard( fe, null, null ) ); } internal virtual void Invoke( FrameworkElement containingFE, FrameworkContentElement containingFCE, Storyboard storyboard ) { } // Find a Storyboard object for this StoryboardAction to act on, using the // given BeginStoryboardName to find a BeginStoryboard instance and use // its Storyboard object reference. private Storyboard GetStoryboard( FrameworkElement fe, FrameworkContentElement fce, INameScope nameScope ) { if( BeginStoryboardName == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNameRequired)); } BeginStoryboard keyedBeginStoryboard = Storyboard.ResolveBeginStoryboardName( BeginStoryboardName, nameScope, fe, fce ); Storyboard storyboard = keyedBeginStoryboard.Storyboard; if( storyboard == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNoStoryboard, BeginStoryboardName)); } return storyboard; } private string _beginStoryboardName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
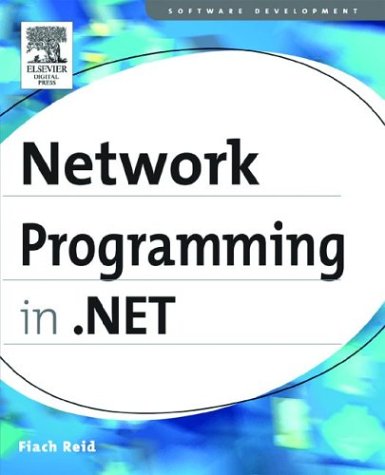
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeHandles.cs
- TemplateManager.cs
- CodeCatchClauseCollection.cs
- ValidationErrorCollection.cs
- HiddenFieldPageStatePersister.cs
- XmlAtomicValue.cs
- UnknownWrapper.cs
- AsyncStreamReader.cs
- TextContainerHelper.cs
- path.cs
- LookupNode.cs
- TextCollapsingProperties.cs
- DescendantOverDescendantQuery.cs
- CustomAttributeFormatException.cs
- DataRelationCollection.cs
- EntityObject.cs
- ProfilePropertySettings.cs
- SafeThreadHandle.cs
- Utils.cs
- GCHandleCookieTable.cs
- securitymgrsite.cs
- MetabaseSettings.cs
- NGCPageContentCollectionSerializerAsync.cs
- PinnedBufferMemoryStream.cs
- AddingNewEventArgs.cs
- BaseDataListComponentEditor.cs
- SqlDataSourceFilteringEventArgs.cs
- ShaderEffect.cs
- XmlWrappingReader.cs
- TableCellAutomationPeer.cs
- ProxyAssemblyNotLoadedException.cs
- FlagPanel.cs
- HttpCookie.cs
- SocketPermission.cs
- FunctionImportMapping.cs
- ConstraintEnumerator.cs
- LZCodec.cs
- OleDbMetaDataFactory.cs
- ImageField.cs
- CodeMemberField.cs
- DataControlPagerLinkButton.cs
- TransformConverter.cs
- PackageFilter.cs
- AutomationIdentifierGuids.cs
- XAMLParseException.cs
- SchemaNotation.cs
- FieldMetadata.cs
- ConfigurationStrings.cs
- Command.cs
- SmiContextFactory.cs
- ShapingWorkspace.cs
- ExpressionParser.cs
- CompensationParticipant.cs
- ContentType.cs
- FormConverter.cs
- TableLayoutCellPaintEventArgs.cs
- SqlExpander.cs
- CompilerLocalReference.cs
- FamilyMapCollection.cs
- ComponentResourceManager.cs
- HiddenField.cs
- TextElementCollectionHelper.cs
- XPathAncestorQuery.cs
- SerialErrors.cs
- LookupNode.cs
- ObjectDataProvider.cs
- _SecureChannel.cs
- RealizationDrawingContextWalker.cs
- storagemappingitemcollection.viewdictionary.cs
- CryptoApi.cs
- WeakReference.cs
- Point3DAnimationUsingKeyFrames.cs
- GAC.cs
- GridViewColumnCollectionChangedEventArgs.cs
- BlurBitmapEffect.cs
- NameTable.cs
- TogglePattern.cs
- EventWaitHandleSecurity.cs
- TextRange.cs
- EntityCollection.cs
- SqlCacheDependency.cs
- BreadCrumbTextConverter.cs
- SqlTrackingService.cs
- GraphicsState.cs
- GenericUriParser.cs
- Context.cs
- UIAgentAsyncBeginRequest.cs
- TreeNodeEventArgs.cs
- XmlValueConverter.cs
- CombinedGeometry.cs
- SignedXml.cs
- SoapMessage.cs
- QilBinary.cs
- EncodingTable.cs
- CustomMenuItemCollection.cs
- EventArgs.cs
- ImportDesigner.xaml.cs
- Attributes.cs
- RequestCacheEntry.cs
- MouseCaptureWithinProperty.cs