Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / Common / DBAsyncResult.cs / 1 / DBAsyncResult.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Data.ProviderBase; using System.Diagnostics; using System.Threading; internal sealed class DbAsyncResult : IAsyncResult { private readonly AsyncCallback _callback = null; private bool _fCompleted = false; private bool _fCompletedSynchronously = false; private readonly ManualResetEvent _manualResetEvent = null; private object _owner = null; private readonly object _stateObject = null; private readonly string _endMethodName; private ExecutionContext _execContext = null; static private ContextCallback _contextCallback = new ContextCallback(AsyncCallback_Context); // Used for SqlClient Open async private DbConnectionInternal _connectionInternal = null; internal DbAsyncResult(object owner, string endMethodName, AsyncCallback callback, object stateObject, ExecutionContext execContext) { _owner = owner; _endMethodName = endMethodName; _callback = callback; _stateObject = stateObject; _manualResetEvent = new ManualResetEvent(false); _execContext = execContext; } object IAsyncResult.AsyncState { get { return _stateObject; } } WaitHandle IAsyncResult.AsyncWaitHandle { get { return _manualResetEvent; } } bool IAsyncResult.CompletedSynchronously { get { return _fCompletedSynchronously; } } internal DbConnectionInternal ConnectionInternal { get { return _connectionInternal; } set { _connectionInternal = value; } } bool IAsyncResult.IsCompleted { get { return _fCompleted; } } internal string EndMethodName { get { return _endMethodName; } } internal void CompareExchangeOwner(object owner, string method) { object prior = Interlocked.CompareExchange(ref _owner, null, owner); if (prior != owner) { if (null != prior) { throw ADP.IncorrectAsyncResult(); } throw ADP.MethodCalledTwice(method); } } internal void Reset() { _fCompleted = false; _fCompletedSynchronously = false; _manualResetEvent.Reset(); } internal void SetCompleted() { _fCompleted = true; _manualResetEvent.Set(); if (_callback != null) { // QueueUserWorkItem only accepts WaitCallback - which requires a signature of Foo(object state). // Must call function on this object with that signature - and then call user AsyncCallback. // AsyncCallback signature is Foo(IAsyncResult result). ThreadPool.QueueUserWorkItem(new WaitCallback(ExecuteCallback), this); } } internal void SetCompletedSynchronously() { _fCompletedSynchronously = true; } static private void AsyncCallback_Context(Object state) { DbAsyncResult result = (DbAsyncResult) state; if (result._callback != null) { result._callback(result); } } private void ExecuteCallback(object asyncResult) { DbAsyncResult result = (DbAsyncResult) asyncResult; if (null != result._callback) { if (result._execContext != null) { ExecutionContext.Run(result._execContext, DbAsyncResult._contextCallback, result); } else { result._callback(this); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Data.ProviderBase; using System.Diagnostics; using System.Threading; internal sealed class DbAsyncResult : IAsyncResult { private readonly AsyncCallback _callback = null; private bool _fCompleted = false; private bool _fCompletedSynchronously = false; private readonly ManualResetEvent _manualResetEvent = null; private object _owner = null; private readonly object _stateObject = null; private readonly string _endMethodName; private ExecutionContext _execContext = null; static private ContextCallback _contextCallback = new ContextCallback(AsyncCallback_Context); // Used for SqlClient Open async private DbConnectionInternal _connectionInternal = null; internal DbAsyncResult(object owner, string endMethodName, AsyncCallback callback, object stateObject, ExecutionContext execContext) { _owner = owner; _endMethodName = endMethodName; _callback = callback; _stateObject = stateObject; _manualResetEvent = new ManualResetEvent(false); _execContext = execContext; } object IAsyncResult.AsyncState { get { return _stateObject; } } WaitHandle IAsyncResult.AsyncWaitHandle { get { return _manualResetEvent; } } bool IAsyncResult.CompletedSynchronously { get { return _fCompletedSynchronously; } } internal DbConnectionInternal ConnectionInternal { get { return _connectionInternal; } set { _connectionInternal = value; } } bool IAsyncResult.IsCompleted { get { return _fCompleted; } } internal string EndMethodName { get { return _endMethodName; } } internal void CompareExchangeOwner(object owner, string method) { object prior = Interlocked.CompareExchange(ref _owner, null, owner); if (prior != owner) { if (null != prior) { throw ADP.IncorrectAsyncResult(); } throw ADP.MethodCalledTwice(method); } } internal void Reset() { _fCompleted = false; _fCompletedSynchronously = false; _manualResetEvent.Reset(); } internal void SetCompleted() { _fCompleted = true; _manualResetEvent.Set(); if (_callback != null) { // QueueUserWorkItem only accepts WaitCallback - which requires a signature of Foo(object state). // Must call function on this object with that signature - and then call user AsyncCallback. // AsyncCallback signature is Foo(IAsyncResult result). ThreadPool.QueueUserWorkItem(new WaitCallback(ExecuteCallback), this); } } internal void SetCompletedSynchronously() { _fCompletedSynchronously = true; } static private void AsyncCallback_Context(Object state) { DbAsyncResult result = (DbAsyncResult) state; if (result._callback != null) { result._callback(result); } } private void ExecuteCallback(object asyncResult) { DbAsyncResult result = (DbAsyncResult) asyncResult; if (null != result._callback) { if (result._execContext != null) { ExecutionContext.Run(result._execContext, DbAsyncResult._contextCallback, result); } else { result._callback(this); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
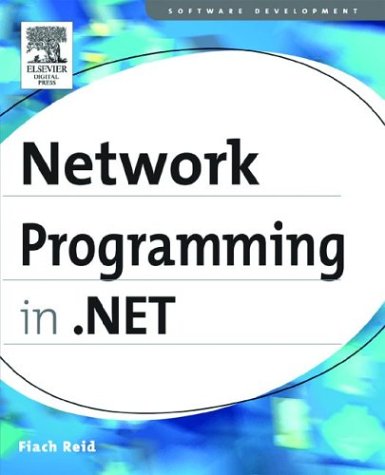
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CopyAction.cs
- SecureEnvironment.cs
- AutomationPropertyInfo.cs
- ConfigurationPropertyAttribute.cs
- ActionItem.cs
- SatelliteContractVersionAttribute.cs
- DataGridHeaderBorder.cs
- RadioButton.cs
- DatagridviewDisplayedBandsData.cs
- ToolStripArrowRenderEventArgs.cs
- PageParserFilter.cs
- Substitution.cs
- ControlAdapter.cs
- SafeLibraryHandle.cs
- DocumentXmlWriter.cs
- SqlNodeTypeOperators.cs
- SapiRecognizer.cs
- EncryptedReference.cs
- FileSystemEventArgs.cs
- XmlEnumAttribute.cs
- CompModSwitches.cs
- CloudCollection.cs
- DataGridViewTopLeftHeaderCell.cs
- ErrorEventArgs.cs
- EditingMode.cs
- AccessViolationException.cs
- SqlConnectionString.cs
- wgx_render.cs
- XmlSchemaChoice.cs
- FixedSOMSemanticBox.cs
- MediaElement.cs
- AnimatedTypeHelpers.cs
- FixedSOMContainer.cs
- WebProxyScriptElement.cs
- RegisteredArrayDeclaration.cs
- InvalidComObjectException.cs
- PageRanges.cs
- SerializationObjectManager.cs
- Number.cs
- XmlSchema.cs
- ElementMarkupObject.cs
- AssemblyNameProxy.cs
- FullTextBreakpoint.cs
- XmlChildNodes.cs
- HierarchicalDataSourceControl.cs
- _Rfc2616CacheValidators.cs
- XsltLibrary.cs
- Int32CAMarshaler.cs
- PersonalizationEntry.cs
- EmptyControlCollection.cs
- LinqDataSourceContextData.cs
- NetworkInterface.cs
- NullEntityWrapper.cs
- CodeStatementCollection.cs
- ColorPalette.cs
- ConfigurationStrings.cs
- HierarchicalDataTemplate.cs
- StrongTypingException.cs
- TagMapCollection.cs
- FormattedText.cs
- MdImport.cs
- DataStreams.cs
- GridViewColumnCollection.cs
- ProxySimple.cs
- SqlDeflator.cs
- MappingModelBuildProvider.cs
- GridSplitterAutomationPeer.cs
- TdsEnums.cs
- DataGridViewRowCollection.cs
- Char.cs
- DataGridRow.cs
- ReadOnlyCollection.cs
- TextSchema.cs
- HostingEnvironment.cs
- ChangePasswordDesigner.cs
- TransformedBitmap.cs
- RegexStringValidatorAttribute.cs
- DetailsViewRowCollection.cs
- ProfileService.cs
- MILUtilities.cs
- DispatchChannelSink.cs
- StyleSheetDesigner.cs
- SessionIDManager.cs
- SecurityElement.cs
- InstanceCreationEditor.cs
- TemplateKey.cs
- ScriptingProfileServiceSection.cs
- RuntimeHandles.cs
- DoubleAnimationClockResource.cs
- VoiceObjectToken.cs
- FixedTextPointer.cs
- EntityDataSourceQueryBuilder.cs
- IsolatedStorageException.cs
- XmlSchemaCompilationSettings.cs
- AttributeData.cs
- IPeerNeighbor.cs
- DecoderReplacementFallback.cs
- X509Certificate2Collection.cs
- Unit.cs
- EntityDataSourceDataSelection.cs