Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / Internal / NullEntityWrapper.cs / 1305376 / NullEntityWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections; using System.Data.Objects.DataClasses; using System.Data.Entity; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Objects.Internal { ////// Defines an entity wrapper that wraps an entity with a null value. /// This is a singleton class for which the same instance is always returned /// any time a wrapper around a null entity is requested. Objects of this /// type are immutable and mutable to allow this behavior to work correctly. /// internal class NullEntityWrapper : IEntityWrapper { private static IEntityWrapper s_nullWrapper = new NullEntityWrapper(); // Private constructor prevents anyone else from creating an instance private NullEntityWrapper() { } ////// The single instance of this class. /// internal static IEntityWrapper NullWrapper { get { return s_nullWrapper; } } public RelationshipManager RelationshipManager { get { Debug.Fail("Cannot access RelationshipManager from null wrapper."); return null; } } public bool OwnsRelationshipManager { get { Debug.Fail("Cannot access RelationshipManager from null wrapper."); return false; } } public object Entity { get { return null; } } public EntityEntry ObjectStateEntry { get { return null; } set { } } public void CollectionAdd(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot modify collection from null wrapper."); } public bool CollectionRemove(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot modify collection from null wrapper."); return false; } public EntityKey EntityKey { get { Debug.Fail("Cannot access EntityKey from null wrapper."); return null; } set { Debug.Fail("Cannot access EntityKey from null wrapper."); } } public EntityKey GetEntityKeyFromEntity() { Debug.Assert(false, "Method on NullEntityWrapper should not be called"); return null; } public ObjectContext Context { get { Debug.Fail("Cannot access Context from null wrapper."); return null; } set { Debug.Fail("Cannot access Context from null wrapper."); } } public MergeOption MergeOption { get { Debug.Fail("Cannot access MergeOption from null wrapper."); return MergeOption.NoTracking; } } public void AttachContext(ObjectContext context, EntitySet entitySet, MergeOption mergeOption) { Debug.Fail("Cannot access Context from null wrapper."); } public void ResetContext(ObjectContext context, EntitySet entitySet, MergeOption mergeOption) { Debug.Fail("Cannot access Context from null wrapper."); } public void DetachContext() { Debug.Fail("Cannot access Context from null wrapper."); } public void SetChangeTracker(IEntityChangeTracker changeTracker) { Debug.Fail("Cannot access ChangeTracker from null wrapper."); } public void TakeSnapshot(EntityEntry entry) { Debug.Fail("Cannot take snapshot of using null wrapper."); } public void TakeSnapshotOfRelationships(EntityEntry entry) { Debug.Fail("Cannot take snapshot using null wrapper."); } public Type IdentityType { get { Debug.Fail("Cannot access IdentityType from null wrapper."); return null; } } public void EnsureCollectionNotNull(RelatedEnd relatedEnd) { Debug.Fail("Cannot modify collection from null wrapper."); } public object GetNavigationPropertyValue(RelatedEnd relatedEnd) { Debug.Fail("Cannot access property using null wrapper."); return null; } public void SetNavigationPropertyValue(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot access property using null wrapper."); } public void RemoveNavigationPropertyValue(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot access property using null wrapper."); } public void SetCurrentValue(EntityEntry entry, StateManagerMemberMetadata member, int ordinal, object target, object value) { Debug.Fail("Cannot set a value onto a null entity."); } public bool InitializingProxyRelatedEnds { get { Debug.Fail("Cannot access flag on null wrapper."); return false; } set { Debug.Fail("Cannot access flag on null wrapper."); } } public void UpdateCurrentValueRecord(object value, EntityEntry entry) { Debug.Fail("Cannot UpdateCurrentValueRecord on a null entity."); } public bool RequiresRelationshipChangeTracking { get { return false; } } public bool RequiresComplexChangeTracking { get { return false; } } public bool RequiresScalarChangeTracking { get { return false; } } public bool RequiresAnyChangeTracking { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections; using System.Data.Objects.DataClasses; using System.Data.Entity; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Objects.Internal { ////// Defines an entity wrapper that wraps an entity with a null value. /// This is a singleton class for which the same instance is always returned /// any time a wrapper around a null entity is requested. Objects of this /// type are immutable and mutable to allow this behavior to work correctly. /// internal class NullEntityWrapper : IEntityWrapper { private static IEntityWrapper s_nullWrapper = new NullEntityWrapper(); // Private constructor prevents anyone else from creating an instance private NullEntityWrapper() { } ////// The single instance of this class. /// internal static IEntityWrapper NullWrapper { get { return s_nullWrapper; } } public RelationshipManager RelationshipManager { get { Debug.Fail("Cannot access RelationshipManager from null wrapper."); return null; } } public bool OwnsRelationshipManager { get { Debug.Fail("Cannot access RelationshipManager from null wrapper."); return false; } } public object Entity { get { return null; } } public EntityEntry ObjectStateEntry { get { return null; } set { } } public void CollectionAdd(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot modify collection from null wrapper."); } public bool CollectionRemove(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot modify collection from null wrapper."); return false; } public EntityKey EntityKey { get { Debug.Fail("Cannot access EntityKey from null wrapper."); return null; } set { Debug.Fail("Cannot access EntityKey from null wrapper."); } } public EntityKey GetEntityKeyFromEntity() { Debug.Assert(false, "Method on NullEntityWrapper should not be called"); return null; } public ObjectContext Context { get { Debug.Fail("Cannot access Context from null wrapper."); return null; } set { Debug.Fail("Cannot access Context from null wrapper."); } } public MergeOption MergeOption { get { Debug.Fail("Cannot access MergeOption from null wrapper."); return MergeOption.NoTracking; } } public void AttachContext(ObjectContext context, EntitySet entitySet, MergeOption mergeOption) { Debug.Fail("Cannot access Context from null wrapper."); } public void ResetContext(ObjectContext context, EntitySet entitySet, MergeOption mergeOption) { Debug.Fail("Cannot access Context from null wrapper."); } public void DetachContext() { Debug.Fail("Cannot access Context from null wrapper."); } public void SetChangeTracker(IEntityChangeTracker changeTracker) { Debug.Fail("Cannot access ChangeTracker from null wrapper."); } public void TakeSnapshot(EntityEntry entry) { Debug.Fail("Cannot take snapshot of using null wrapper."); } public void TakeSnapshotOfRelationships(EntityEntry entry) { Debug.Fail("Cannot take snapshot using null wrapper."); } public Type IdentityType { get { Debug.Fail("Cannot access IdentityType from null wrapper."); return null; } } public void EnsureCollectionNotNull(RelatedEnd relatedEnd) { Debug.Fail("Cannot modify collection from null wrapper."); } public object GetNavigationPropertyValue(RelatedEnd relatedEnd) { Debug.Fail("Cannot access property using null wrapper."); return null; } public void SetNavigationPropertyValue(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot access property using null wrapper."); } public void RemoveNavigationPropertyValue(RelatedEnd relatedEnd, object value) { Debug.Fail("Cannot access property using null wrapper."); } public void SetCurrentValue(EntityEntry entry, StateManagerMemberMetadata member, int ordinal, object target, object value) { Debug.Fail("Cannot set a value onto a null entity."); } public bool InitializingProxyRelatedEnds { get { Debug.Fail("Cannot access flag on null wrapper."); return false; } set { Debug.Fail("Cannot access flag on null wrapper."); } } public void UpdateCurrentValueRecord(object value, EntityEntry entry) { Debug.Fail("Cannot UpdateCurrentValueRecord on a null entity."); } public bool RequiresRelationshipChangeTracking { get { return false; } } public bool RequiresComplexChangeTracking { get { return false; } } public bool RequiresScalarChangeTracking { get { return false; } } public bool RequiresAnyChangeTracking { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
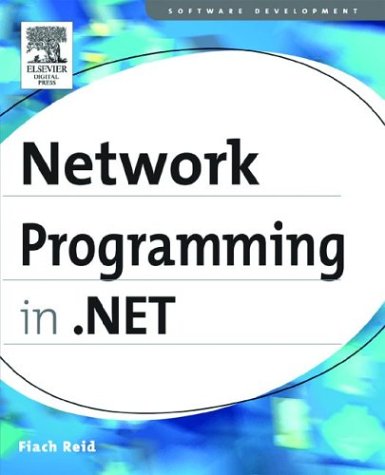
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PingOptions.cs
- ExpressionHelper.cs
- DbConnectionPoolGroupProviderInfo.cs
- WithParamAction.cs
- FreezableOperations.cs
- Type.cs
- HtmlInputImage.cs
- SafeUserTokenHandle.cs
- FrameworkRichTextComposition.cs
- AssemblyBuilderData.cs
- FormsAuthenticationUser.cs
- ArgIterator.cs
- Transform3DGroup.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- EntityCollection.cs
- SingleKeyFrameCollection.cs
- Panel.cs
- HwndTarget.cs
- ProcessRequestAsyncResult.cs
- HostedBindingBehavior.cs
- ResourcesChangeInfo.cs
- DefaultValueTypeConverter.cs
- NamespaceMapping.cs
- Thread.cs
- SwitchElementsCollection.cs
- DataGridHeaderBorder.cs
- PersonalizablePropertyEntry.cs
- _LazyAsyncResult.cs
- SemaphoreSecurity.cs
- Drawing.cs
- storepermissionattribute.cs
- HtmlLink.cs
- FieldDescriptor.cs
- CustomCategoryAttribute.cs
- PageContentAsyncResult.cs
- ScrollViewerAutomationPeer.cs
- UnrecognizedPolicyAssertionElement.cs
- InfoCardProofToken.cs
- Set.cs
- MediaContext.cs
- Registry.cs
- PatternMatcher.cs
- DuplicateDetector.cs
- FrameworkElement.cs
- StringAnimationUsingKeyFrames.cs
- WebPartManager.cs
- ObjectRef.cs
- ColumnMapProcessor.cs
- StreamReader.cs
- ModelVisual3D.cs
- UndoManager.cs
- WebPartChrome.cs
- Command.cs
- _ListenerAsyncResult.cs
- MailMessageEventArgs.cs
- rsa.cs
- SecurityDescriptor.cs
- SqlBooleanizer.cs
- URLString.cs
- SoapRpcServiceAttribute.cs
- ContractBase.cs
- XmlSerializer.cs
- AsyncPostBackErrorEventArgs.cs
- TextProperties.cs
- BehaviorEditorPart.cs
- SqlDataSourceCache.cs
- BevelBitmapEffect.cs
- TokenBasedSet.cs
- AudioException.cs
- StringUtil.cs
- DataServiceExpressionVisitor.cs
- HtmlInputRadioButton.cs
- PersianCalendar.cs
- ResourceExpressionBuilder.cs
- safemediahandle.cs
- EditorAttribute.cs
- IImplicitResourceProvider.cs
- AppendHelper.cs
- Rights.cs
- WindowsListViewItemCheckBox.cs
- Item.cs
- WaitForChangedResult.cs
- ModelTreeManager.cs
- StringDictionary.cs
- WindowsFormsHost.cs
- DataSpaceManager.cs
- UnmanagedMemoryStreamWrapper.cs
- Button.cs
- ProcessHost.cs
- MessageAction.cs
- SemaphoreFullException.cs
- JumpTask.cs
- RC2.cs
- ControlHelper.cs
- SqlTriggerAttribute.cs
- PolicyManager.cs
- PropertyReferenceSerializer.cs
- DynamicControlParameter.cs
- WorkflowViewStateService.cs
- WebPartZone.cs