Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / XamlBuildTask / Microsoft / Build / Tasks / Xaml / AttributeData.cs / 1305376 / AttributeData.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Xaml; using System.ComponentModel; using System.Runtime; namespace Microsoft.Build.Tasks.Xaml { sealed class AttributeData : Attribute { IListparameters; IList properties; public XamlType Type { get; set; } public IList Parameters { get { if (parameters == null) { parameters = new List (); } return parameters; } set { parameters = value; } } public IList Properties { get { if (properties == null) { properties = new List (); } return properties; } set { properties = value; } } // We get here when we are inside x:ClassAttributes or x:Property.Attributes. We expect the first element to be the Attribute SO. public static AttributeData LoadAttributeData(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { AttributeData attributeData = null; reader.Read(); if (reader.NodeType == XamlNodeType.StartObject) { attributeData = new AttributeData { Type = reader.Type }; bool readNext = false; while (readNext || reader.Read()) { namespaceTable.ManageNamespace(reader); readNext = false; if (reader.NodeType == XamlNodeType.StartMember) { if (reader.Member == XamlLanguage.Arguments) { attributeData.Parameters = ReadParameters(reader.ReadSubtree(), namespaceTable, rootNamespace); readNext = true; } else if (!reader.Member.IsDirective) { AttributeParameterInfo propertyInfo = ReadAttributeProperty(reader.ReadSubtree(), namespaceTable, rootNamespace); attributeData.Properties.Add(propertyInfo); readNext = true; } } } } return attributeData; } // Read the Property on the attribute. private static AttributeParameterInfo ReadAttributeProperty(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { reader.Read(); Fx.Assert(reader.Member != null, "Member element should not be null"); XamlMember member = reader.Member; AttributeParameterInfo propertyInfo = new AttributeParameterInfo(); propertyInfo.Name = member.Name; if (member.Type != null && !member.Type.IsUnknown) { propertyInfo.Type = member.Type.UnderlyingType; } ReadParamInfo(reader, namespaceTable, rootNamespace, propertyInfo); return propertyInfo; } // Read the parameters on the Attribute. We expect the parameters to be in the order in which they are supposed to appear in the output code. // Here we are inside x:Arguments and we expect a list of parameters. private static IList ReadParameters(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { IList parameters = new List (); bool readNext = false; while (readNext || reader.Read()) { readNext = false; if (reader.NodeType == XamlNodeType.StartObject) { AttributeParameterInfo paramInfo = new AttributeParameterInfo(); ReadParamInfo(reader.ReadSubtree(), namespaceTable, rootNamespace, paramInfo); parameters.Add(paramInfo); readNext = true; } } return parameters; } // Read the actual parameter info, i.e. the type of the paramter and its value. // The first element could be a V or an SO. private static void ReadParamInfo(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace, AttributeParameterInfo paramInfo) { reader.Read(); bool readNext = false; do { readNext = false; if (reader.NodeType == XamlNodeType.StartObject && reader.Type == XamlLanguage.Array) { paramInfo.IsArray = true; XamlReader xamlArrayReader = reader.ReadSubtree(); xamlArrayReader.Read(); while (readNext || xamlArrayReader.Read()) { readNext = false; if (xamlArrayReader.NodeType == XamlNodeType.StartMember && xamlArrayReader.Member.Name == "Type") { xamlArrayReader.Read(); if (xamlArrayReader.NodeType == XamlNodeType.Value) { XamlType arrayType = XamlBuildTaskServices.GetXamlTypeFromString(xamlArrayReader.Value as string, namespaceTable, xamlArrayReader.SchemaContext); paramInfo.Type = arrayType.UnderlyingType; } } else if (xamlArrayReader.NodeType == XamlNodeType.StartObject) { AttributeParameterInfo arrayEntry = new AttributeParameterInfo(); ReadParamInfo(xamlArrayReader.ReadSubtree(), namespaceTable, rootNamespace, arrayEntry); paramInfo.ArrayContents.Add(arrayEntry); readNext = true; } } } else if(reader.NodeType == XamlNodeType.StartObject || reader.NodeType == XamlNodeType.Value) { paramInfo.IsArray = false; string paramVal; Type paramType; GetParamValueType(reader.ReadSubtree(), paramInfo.Type, namespaceTable, rootNamespace, out paramVal, out paramType); paramInfo.Value = paramVal; paramInfo.Type = paramType; } } while (readNext || reader.Read()); } // Get the paramter value. If the value is enclosed inside nodes of the type, then get the parameter type as well. // Else infer the type from the type of the property. private static void GetParamValueType(XamlReader reader, Type type, NamespaceTable namespaceTable, string rootNamespace, out string paramValue, out Type paramType) { paramValue = String.Empty; paramType = type; while (reader.Read()) { if (reader.NodeType == XamlNodeType.Value) { if (paramType != null) { paramValue = GetParamValue(reader.Value as string, paramType, reader, namespaceTable, rootNamespace); } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.AttributeParameterTypeUnknown(reader.Value as string))); } } else if (reader.NodeType == XamlNodeType.StartObject) { if (reader.Type == XamlLanguage.Null) { paramValue = null; paramType = null; } else if (reader.Type == XamlLanguage.Type) { paramType = typeof(Type); } else { paramType = reader.Type.UnderlyingType; } } } } // Get the parameter value that is to be put in the generated code as is. private static string GetParamValue(string value, Type type, XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { string paramValue; if (type.IsEnum) { paramValue = type.FullName + "." + value; } else if (type.IsAssignableFrom(typeof(System.Type))) { XamlType typeName = XamlBuildTaskServices.GetXamlTypeFromString(value, namespaceTable, reader.SchemaContext); string clrTypeName; if (!XamlBuildTaskServices.TryGetClrTypeName(typeName, rootNamespace, out clrTypeName)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.TypeNameUnknown(XamlBuildTaskServices.GetFullTypeName(typeName)))); } paramValue = clrTypeName; } else if (type == typeof(String)) { paramValue = value; } else if (type.IsPrimitive) { value = value.TrimStart('"'); value = value.TrimEnd('"'); if (type == typeof(bool)) { if (string.Compare(value, "true", StringComparison.OrdinalIgnoreCase) == 0) { paramValue = "true"; } else if (string.Compare(value, "false", StringComparison.OrdinalIgnoreCase) == 0) { paramValue = "false"; } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.UnknownBooleanValue(value))); } } else { paramValue = value; } } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.AttributeParamTypeNotSupported(type.FullName))); } return paramValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
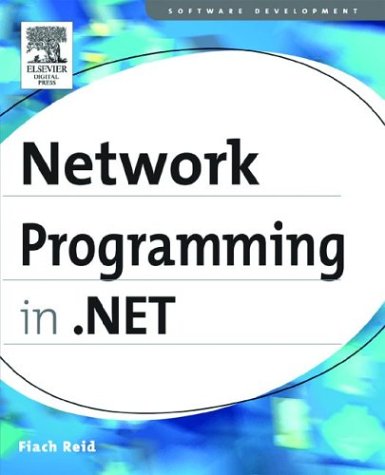
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JumpTask.cs
- TextDecoration.cs
- ContentElement.cs
- FontStretch.cs
- AppDomainManager.cs
- TextEditorSpelling.cs
- HttpServerUtilityWrapper.cs
- TabletDeviceInfo.cs
- StorageInfo.cs
- UICuesEvent.cs
- IdentifierCollection.cs
- _HelperAsyncResults.cs
- UTF32Encoding.cs
- Query.cs
- ConstantCheck.cs
- FileSystemEventArgs.cs
- SiblingIterators.cs
- ComplexObject.cs
- DataSvcMapFile.cs
- MimeBasePart.cs
- CryptoProvider.cs
- InfiniteIntConverter.cs
- CheckBox.cs
- ByteConverter.cs
- MessagePartDescription.cs
- TemplateKeyConverter.cs
- IIS7WorkerRequest.cs
- ClientConvert.cs
- TransportSecurityProtocolFactory.cs
- TrackingProfileSerializer.cs
- HttpCachePolicyBase.cs
- DocumentViewerHelper.cs
- XmlSchemaSimpleTypeRestriction.cs
- HealthMonitoringSection.cs
- Model3D.cs
- ConfigurationValue.cs
- FaultDesigner.cs
- ExtensibleClassFactory.cs
- ExpressionDumper.cs
- SafeNativeMethods.cs
- SpinLock.cs
- TypeDescriptionProvider.cs
- DependencyObjectType.cs
- UiaCoreTypesApi.cs
- RuntimeConfigLKG.cs
- WorkflowServiceAttributes.cs
- EntitySqlQueryState.cs
- SctClaimDictionary.cs
- XmlDocument.cs
- ResourceExpressionEditor.cs
- CodeEventReferenceExpression.cs
- TypeReference.cs
- Select.cs
- KeysConverter.cs
- EUCJPEncoding.cs
- RegexGroup.cs
- DrawListViewSubItemEventArgs.cs
- TypedElement.cs
- Cell.cs
- CompressStream.cs
- CommandExpr.cs
- SerializationTrace.cs
- Choices.cs
- _RequestCacheProtocol.cs
- COAUTHINFO.cs
- SqlUserDefinedAggregateAttribute.cs
- CodeBlockBuilder.cs
- Stacktrace.cs
- NGCSerializationManagerAsync.cs
- KerberosSecurityTokenProvider.cs
- LocalizableResourceBuilder.cs
- TextFragmentEngine.cs
- DoubleLink.cs
- Point3DCollection.cs
- UnknownBitmapEncoder.cs
- InternalsVisibleToAttribute.cs
- ToolStripEditorManager.cs
- CustomValidator.cs
- CodeAccessSecurityEngine.cs
- GenericAuthenticationEventArgs.cs
- RichTextBoxAutomationPeer.cs
- IERequestCache.cs
- DocumentPageView.cs
- LockRecursionException.cs
- HtmlTable.cs
- CqlBlock.cs
- odbcmetadatacollectionnames.cs
- XmlSchemaAnnotated.cs
- XmlSchemaAttributeGroup.cs
- GregorianCalendarHelper.cs
- Size3DValueSerializer.cs
- EntityReference.cs
- Win32PrintDialog.cs
- WebPartDisplayModeCancelEventArgs.cs
- Misc.cs
- HelpHtmlBuilder.cs
- shaperfactory.cs
- ApplicationActivator.cs
- FontFamily.cs
- DirectoryRootQuery.cs