Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media3D / GeneralTransform3DTo2D.cs / 1 / GeneralTransform3DTo2D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Declaration of the GeneralTransform3DTo2D class. // //--------------------------------------------------------------------------- using System.Windows.Media; using System.Windows.Media.Animation; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// GeneralTransform3DTo2D class provides services to transform points and rects in 3D to 2D /// public class GeneralTransform3DTo2D : Freezable { internal GeneralTransform3DTo2D() { _transformBetween2D = null; } internal GeneralTransform3DTo2D(Matrix3D projectionTransform, GeneralTransform transformBetween2D) { _projectionTransform = projectionTransform; _transformBetween2D = (GeneralTransform)transformBetween2D.GetAsFrozen(); } ////// Transform a point /// /// Input point /// Output point ///True if the point was transformed successfuly, false otherwise public bool TryTransform(Point3D inPoint, out Point result) { bool success = false; result = new Point(); // project the point if (_projectionTransform != null) { Point3D projectedPoint = _projectionTransform.Transform(inPoint); if (_transformBetween2D != null) { result = _transformBetween2D.Transform(new Point(projectedPoint.X, projectedPoint.Y)); success = true; } } return success; } ////// Transform a point from 3D in to 2D /// /// If the transformation does not succeed, this will throw an InvalidOperationException. /// If you don't want to try/catch, call TryTransform instead and check the boolean it /// returns. /// /// /// Input point ///The transformed point public Point Transform(Point3D point) { Point transformedPoint; if (!TryTransform(point, out transformedPoint)) { throw new InvalidOperationException(SR.Get(SRID.GeneralTransform_TransformFailed, null)); } return transformedPoint; } ////// Transform a Rect3D to a Rect. If this transformation cannot be completed Rect.Empty is returned. /// /// Input 3D bounding box ///The 2D bounding box of the projection of these points public Rect TransformBounds(Rect3D rect3D) { if (_transformBetween2D != null) { return _transformBetween2D.TransformBounds(MILUtilities.ProjectBounds(ref _projectionTransform, ref rect3D)); } else { return Rect.Empty; } } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GeneralTransform3DTo2D(); } ////// Implementation of /// protected override void CloneCore(Freezable sourceFreezable) { GeneralTransform3DTo2D transform = (GeneralTransform3DTo2D)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCore . ////// Implementation of /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { GeneralTransform3DTo2D transform = (GeneralTransform3DTo2D)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCurrentValueCore . ////// Implementation of /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { GeneralTransform3DTo2D transform = (GeneralTransform3DTo2D)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetAsFrozenCore . ////// Implementation of /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { GeneralTransform3DTo2D transform = (GeneralTransform3DTo2D)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Clones values that do not have corresponding DPs /// /// private void CopyCommon(GeneralTransform3DTo2D transform) { _projectionTransform = transform._projectionTransform; _transformBetween2D = transform._transformBetween2D; } private Matrix3D _projectionTransform; private GeneralTransform _transformBetween2D; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
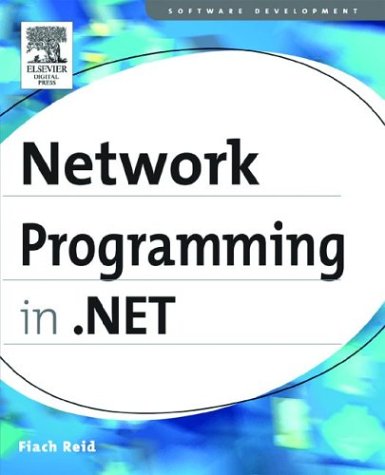
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MultiBinding.cs
- HttpWebRequest.cs
- DeviceContexts.cs
- StubHelpers.cs
- ValidatedControlConverter.cs
- NonBatchDirectoryCompiler.cs
- MetadataPropertyAttribute.cs
- UpdateTracker.cs
- ReadOnlyNameValueCollection.cs
- Itemizer.cs
- StateBag.cs
- PathParser.cs
- EntityViewContainer.cs
- DynamicRendererThreadManager.cs
- IFormattable.cs
- StopRoutingHandler.cs
- WebBrowserSiteBase.cs
- MetafileHeader.cs
- MediaTimeline.cs
- FocusWithinProperty.cs
- BinaryQueryOperator.cs
- XmlSchemaObjectTable.cs
- ColumnTypeConverter.cs
- VisualProxy.cs
- GridViewEditEventArgs.cs
- HttpPostServerProtocol.cs
- Attribute.cs
- Instrumentation.cs
- TextFormatterImp.cs
- XamlToRtfParser.cs
- processwaithandle.cs
- Point3DIndependentAnimationStorage.cs
- WebControlsSection.cs
- CodeDelegateCreateExpression.cs
- EUCJPEncoding.cs
- ToolBarDesigner.cs
- HGlobalSafeHandle.cs
- ProcessDesigner.cs
- XmlDictionaryReaderQuotas.cs
- DataList.cs
- BindingExpressionUncommonField.cs
- XmlReflectionImporter.cs
- ElementFactory.cs
- SafeCertificateContext.cs
- shaperfactory.cs
- EventDescriptorCollection.cs
- OrderedDictionary.cs
- Byte.cs
- VoiceSynthesis.cs
- DataControlLinkButton.cs
- NumberFunctions.cs
- ToolStripItemEventArgs.cs
- TextSearch.cs
- ToolStripDropDownClosingEventArgs.cs
- QuestionEventArgs.cs
- ObjectListField.cs
- EventListenerClientSide.cs
- CharEnumerator.cs
- XmlSiteMapProvider.cs
- ScaleTransform3D.cs
- IsolatedStorageFile.cs
- CreateUserWizardDesigner.cs
- VoiceChangeEventArgs.cs
- ParseElementCollection.cs
- SqlException.cs
- ObjectStateEntryDbDataRecord.cs
- DynamicRouteExpression.cs
- SafeFindHandle.cs
- ResolveNameEventArgs.cs
- SiteMapNode.cs
- RequestBringIntoViewEventArgs.cs
- XPathMessageContext.cs
- InsufficientMemoryException.cs
- FileDialog_Vista.cs
- TrustLevelCollection.cs
- SplitterPanel.cs
- DocumentGridPage.cs
- TextEndOfSegment.cs
- OrElse.cs
- Label.cs
- MultiTouchSystemGestureLogic.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ScrollPatternIdentifiers.cs
- PerspectiveCamera.cs
- ResourceManager.cs
- DatatypeImplementation.cs
- XmlILTrace.cs
- XmlIlTypeHelper.cs
- HtmlGenericControl.cs
- HtmlTextViewAdapter.cs
- ModifyActivitiesPropertyDescriptor.cs
- WebConfigurationManager.cs
- EmissiveMaterial.cs
- Win32KeyboardDevice.cs
- PermissionToken.cs
- ManagementScope.cs
- VisualTransition.cs
- StrokeNodeOperations2.cs
- DataSourceControlBuilder.cs
- PixelShader.cs