Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / Canonicalizers.cs / 1 / Canonicalizers.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Globalization; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // Manages the Different canonicalization forms and routines // we require for use during indexing. // internal sealed class Canonicalizers { delegate byte[] CanonicalizeObjectCallback( object dataToHash ); static ICanonicalizer s_binary; static ICanonicalizer s_caseInsensitiveWithHashing; static ICanonicalizer s_caseSensitiveWithHashing; static ICanonicalizer s_binaryWithHashing; private Canonicalizers() { } // // Summary // Returns the global instance of a CaseSensitive Canonicalizer. This instance // Will hash the value, allowing the entire string value to be matched against. // // Remarks // This should be used with string based indexes only. // public static ICanonicalizer CaseInsensitiveWithHashing { get { if( null == s_caseInsensitiveWithHashing ) { s_caseInsensitiveWithHashing = new CaseInsensitiveCanonicalizer( true, Encoding.Unicode, CultureInfo.InvariantCulture ); } return s_caseInsensitiveWithHashing; } } // // Summary // Returns the global instance of a CaseSensitive Canonicalizer. This instance // Will hash the value, allowing the entire string value to be matched against. // // Remarks // This should be used with string based indexes only. // public static ICanonicalizer CaseSensitiveWithHashing { get { if( null == s_caseSensitiveWithHashing ) { s_caseSensitiveWithHashing = new CaseSensitiveCanonicalizer( true, Encoding.Unicode, CultureInfo.InvariantCulture ); } return s_caseSensitiveWithHashing; } } // // Summary // Returns the global instance of a Binary Canonicalizer. This instance will use // the raw binary form of the input. If the data is larger than the size of the // index, it will be truncated. // // Remarks // This should be used with string based indexes only. // This Canonicalizer supports the following datatypes: // Int16 // UInt16 // Int32 // UInt32 // Int64 // UInt64 // Byte // Byte[] // String // Uri // Guid // GlobalId // public static ICanonicalizer Binary { get { if( null == s_binary ) { s_binary = new BinaryCanonicalizer( false ); } return s_binary; } } // // Summary // Returns the global instance of a Binary Canonicalizer. This instance will use // the raw binary form of the input. The data is hashed before inserted into an index, // so the value can exceed the size of the index. // // Remarks // This should be used with string based indexes only. // This Canonicalizer supports the following datatypes: // Int16 // UInt16 // Int32 // UInt32 // Int64 // UInt64 // Byte // Byte[] // String // Uri // Guid // GlobalId // public static ICanonicalizer BinaryWithHashing { get { if( null == s_binaryWithHashing ) { s_binaryWithHashing = new BinaryCanonicalizer( true ); } return s_binaryWithHashing; } } abstract class CanonicalizerBase : ICanonicalizer { bool m_hashValue; public CanonicalizerBase( bool hashValue ) { m_hashValue = hashValue; } public byte[] Canonicalize( object obj ) { if( null == obj ) { throw IDT.ThrowHelperArgumentNull( "obj" ); } byte[] raw = GetRawForm( obj ); if( !m_hashValue ) { return raw; } byte[] hashValue = new byte[ HashUtility.HashBufferLength ]; HashUtility.SetHashValue( hashValue, 0, raw ); return hashValue; } public abstract bool CanCanonicalize( object obj ); protected abstract byte[] GetRawForm( object obj ); } class BinaryCanonicalizer : CanonicalizerBase { Dictionarym_canonicalizers; public BinaryCanonicalizer( bool hashValue ) : base( hashValue ) { m_canonicalizers = new Dictionary (); CreateCanonicalizers(); } public override bool CanCanonicalize( object obj ) { if( null == obj ) { throw IDT.ThrowHelperArgumentNull( "obj" ); } return m_canonicalizers.ContainsKey( obj.GetType() ); } void CreateCanonicalizers() { m_canonicalizers.Add( typeof( Int16 ), new CanonicalizeObjectCallback( CanonicalizeInt16 ) ); m_canonicalizers.Add( typeof( UInt16 ), new CanonicalizeObjectCallback( CanonicalizeUInt16 ) ); m_canonicalizers.Add( typeof( Int32 ), new CanonicalizeObjectCallback( CanonicalizeInt32 ) ); m_canonicalizers.Add( typeof( UInt32 ), new CanonicalizeObjectCallback( CanonicalizeUInt32 ) ); m_canonicalizers.Add( typeof( Int64 ), new CanonicalizeObjectCallback( CanonicalizeInt64 ) ); m_canonicalizers.Add( typeof( UInt64 ), new CanonicalizeObjectCallback( CanonicalizeUInt64 ) ); m_canonicalizers.Add( typeof( Byte ), new CanonicalizeObjectCallback( CanonicalizeByte ) ); m_canonicalizers.Add( typeof( Byte[] ), new CanonicalizeObjectCallback( CanonicalizeByteArray ) ); m_canonicalizers.Add( typeof( String ), new CanonicalizeObjectCallback( CanonicalizeString ) ); m_canonicalizers.Add( typeof( Guid ), new CanonicalizeObjectCallback( CanonicalizeGuid ) ); m_canonicalizers.Add( typeof( GlobalId ), new CanonicalizeObjectCallback( CanonicalizeGlobalId ) ); } protected override byte[] GetRawForm( object obj ) { return m_canonicalizers[ obj.GetType() ]( obj ); } unsafe byte[] CanonicalizeGlobalId( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } Guid value = (Guid)((GlobalId)data); return value.ToByteArray(); } unsafe byte[] CanonicalizeGuid( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } Guid value = (Guid)data; return value.ToByteArray(); } unsafe byte[] CanonicalizeInt16( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } Int16 value = (Int16)data; byte[] buffer = new byte[ sizeof( Int16 ) ]; fixed( byte* pBuffer = &buffer[ 0 ] ) { *( (Int16*)pBuffer ) = value; } return buffer; } unsafe byte[] CanonicalizeUInt16( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } UInt16 value = (UInt16)data; byte[] buffer = new byte[ sizeof( UInt16 ) ]; fixed( byte* pBuffer = &buffer[ 0 ] ) { *( (UInt16*)pBuffer ) = value; } return buffer; } unsafe byte[] CanonicalizeInt32( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } Int32 value = (Int32)data; byte[] buffer = new byte[ sizeof( Int32 ) ]; fixed( byte* pBuffer = &buffer[ 0 ] ) { *( (Int32*)pBuffer ) = value; } return buffer; } unsafe byte[] CanonicalizeUInt32( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } UInt32 value = (UInt32)data; byte[] buffer = new byte[ sizeof( UInt32 ) ]; fixed( byte* pBuffer = &buffer[ 0 ] ) { *( (UInt32*)pBuffer ) = value; } return buffer; } unsafe byte[] CanonicalizeInt64( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } Int64 value = (Int64)data; byte[] buffer = new byte[ sizeof( Int64 ) ]; fixed( byte* pBuffer = &buffer[ 0 ] ) { *( (Int64*)pBuffer ) = value; } return buffer; } unsafe byte[] CanonicalizeUInt64( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } UInt64 value = (UInt64)data; byte[] buffer = new byte[ sizeof( UInt64 ) ]; fixed( byte* pBuffer = &buffer[ 0 ] ) { *( (UInt64*)pBuffer ) = value; } return buffer; } byte[] CanonicalizeString( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } string value = (string)data; if( String.IsNullOrEmpty(value) ) { throw IDT.ThrowHelperArgumentNull( "data" ); } return Encoding.Unicode.GetBytes( value ); } byte[] CanonicalizeByte( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } byte value = (byte)data; return new byte[]{value}; } byte[] CanonicalizeByteArray( object data ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } byte[] value = (byte[])data; return value; } } // // Base class for String canononicalization. // abstract class StringCanonicalizerBase : CanonicalizerBase { static readonly Type[] s_validTypes = { typeof( string ), typeof( Uri ) }; Encoding m_encoding; CultureInfo m_culture; protected StringCanonicalizerBase( bool hashValue, Encoding encoding, CultureInfo culture ) : base( hashValue ) { if( null == encoding ) { throw IDT.ThrowHelperArgumentNull( "encoding" ); } if( null == culture ) { m_culture = CultureInfo.InvariantCulture; } else { m_culture = culture; } m_encoding = encoding; } protected virtual Type[] SupportedTypes { get { return s_validTypes; } } public CultureInfo Culture { get{ return m_culture; } } public Encoding Encoding { get{ return m_encoding; } } public override bool CanCanonicalize( object obj ) { if( null == obj ) { throw IDT.ThrowHelperArgumentNull( "obj" ); } if( null == SupportedTypes ) return false; return -1 != Array.IndexOf( SupportedTypes, obj.GetType() ); } protected override byte[] GetRawForm( object obj ) { string data = obj.ToString(); return GetBytesFromString( data ); } protected abstract byte[] GetBytesFromString( string data ); } // // Default String Canonicalizer. // class CaseSensitiveCanonicalizer : StringCanonicalizerBase { public CaseSensitiveCanonicalizer( bool hashValue, Encoding encoding,CultureInfo culture ) : base( hashValue, encoding,culture ) { } protected override byte[] GetBytesFromString( string data ) { return Encoding.GetBytes( data ); } } // // Culture Sensitive/Case Sensitive string canonicalizer. // class CaseInsensitiveCanonicalizer : StringCanonicalizerBase { public CaseInsensitiveCanonicalizer( bool hashValue, Encoding encoding,CultureInfo culture ) : base( hashValue, encoding, culture ) { } protected override byte[] GetBytesFromString( string obj ) { return Encoding.GetBytes( Culture.TextInfo.ToUpper( obj ) ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
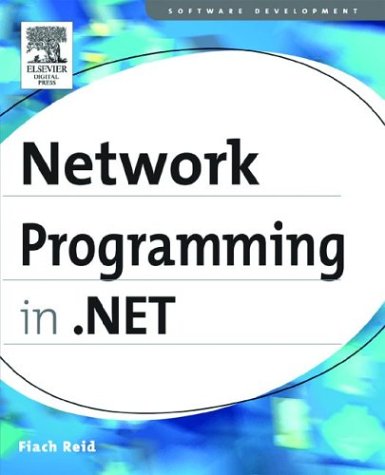
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Column.cs
- Base64Decoder.cs
- CodeStatement.cs
- DataSvcMapFile.cs
- StreamingContext.cs
- NoClickablePointException.cs
- ProfileSettingsCollection.cs
- SQLBytesStorage.cs
- Metafile.cs
- LateBoundBitmapDecoder.cs
- LifetimeServices.cs
- CancelAsyncOperationRequest.cs
- HMACSHA1.cs
- PriorityBinding.cs
- Int32CollectionValueSerializer.cs
- HostingEnvironmentSection.cs
- HtmlInputImage.cs
- EtwProvider.cs
- RichTextBox.cs
- SystemBrushes.cs
- Tracer.cs
- SignerInfo.cs
- ConsoleKeyInfo.cs
- DataException.cs
- MissingMemberException.cs
- CreateSequenceResponse.cs
- Activation.cs
- FileLoadException.cs
- FastEncoderWindow.cs
- ExpressionBuilder.cs
- DbgCompiler.cs
- GridViewColumnHeader.cs
- LogLogRecordEnumerator.cs
- SuppressIldasmAttribute.cs
- DataBinding.cs
- FreezableDefaultValueFactory.cs
- RelationshipManager.cs
- WinEventQueueItem.cs
- userdatakeys.cs
- GlyphInfoList.cs
- ResolveCompletedEventArgs.cs
- TextParagraphCache.cs
- WebServiceTypeData.cs
- ExpandableObjectConverter.cs
- UIElementParagraph.cs
- log.cs
- iisPickupDirectory.cs
- Property.cs
- LabelDesigner.cs
- SessionEndedEventArgs.cs
- DataGridViewCellValueEventArgs.cs
- GeneralTransformGroup.cs
- SessionParameter.cs
- DrawingCollection.cs
- PreviewPageInfo.cs
- OracleRowUpdatingEventArgs.cs
- StrokeCollectionDefaultValueFactory.cs
- Slider.cs
- ClientScriptManager.cs
- FormViewDeleteEventArgs.cs
- NativeRecognizer.cs
- WorkflowMarkupSerializationProvider.cs
- Condition.cs
- BitmapFrameDecode.cs
- BrowserInteropHelper.cs
- DataViewSettingCollection.cs
- NetworkInformationException.cs
- DotExpr.cs
- SQLMembershipProvider.cs
- MasterPageParser.cs
- CacheAxisQuery.cs
- OutOfMemoryException.cs
- EventHandlersStore.cs
- ServiceReflector.cs
- SessionEndingCancelEventArgs.cs
- HotSpotCollection.cs
- IdleTimeoutMonitor.cs
- GenericUriParser.cs
- relpropertyhelper.cs
- DataGridRelationshipRow.cs
- IPHostEntry.cs
- ApplicationDirectory.cs
- FontStretchConverter.cs
- PackageRelationship.cs
- UrlMappingsSection.cs
- CompiledQuery.cs
- SqlExpander.cs
- HMACSHA512.cs
- TabItemAutomationPeer.cs
- ProtocolsConfigurationHandler.cs
- BaseCodePageEncoding.cs
- Header.cs
- Dump.cs
- ConnectorEditor.cs
- FormsAuthenticationModule.cs
- ExceptionWrapper.cs
- BufferedStream.cs
- Form.cs
- IndicShape.cs
- WorkflowItemsPresenter.cs