Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // if (HostingEnvironment.BusyCount != 0) return; // if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
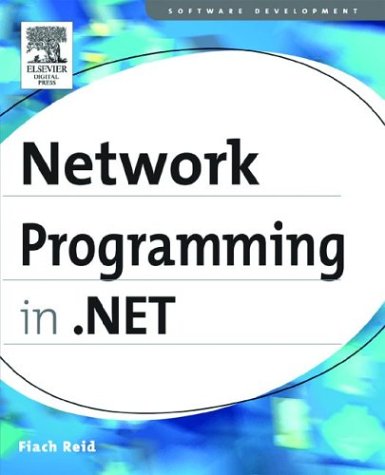
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EdmScalarPropertyAttribute.cs
- EntityContainerRelationshipSet.cs
- ResourcesBuildProvider.cs
- _BaseOverlappedAsyncResult.cs
- EventLogPropertySelector.cs
- DataExpression.cs
- SchemaImporter.cs
- PermissionSetEnumerator.cs
- WebPartRestoreVerb.cs
- Attribute.cs
- VisualStates.cs
- SmiMetaDataProperty.cs
- WeakEventTable.cs
- TransportElement.cs
- InnerItemCollectionView.cs
- GetPageCompletedEventArgs.cs
- MouseBinding.cs
- URL.cs
- SelectorAutomationPeer.cs
- AutoFocusStyle.xaml.cs
- TypeConverterAttribute.cs
- RegexCode.cs
- SoapAttributeAttribute.cs
- ProfileGroupSettingsCollection.cs
- DataGridViewHeaderCell.cs
- ExitEventArgs.cs
- ClassicBorderDecorator.cs
- StrokeFIndices.cs
- SystemBrushes.cs
- PropertyCondition.cs
- XamlPoint3DCollectionSerializer.cs
- PointAnimation.cs
- BindingBase.cs
- RSAPKCS1SignatureDeformatter.cs
- UiaCoreTypesApi.cs
- TextMarkerSource.cs
- StoreContentChangedEventArgs.cs
- Axis.cs
- ResourcePart.cs
- ContentFilePart.cs
- SelfIssuedAuthProofToken.cs
- SqlRowUpdatedEvent.cs
- PrintDialog.cs
- Simplifier.cs
- JsonFormatGeneratorStatics.cs
- HeaderUtility.cs
- AffineTransform3D.cs
- SafeNativeMethods.cs
- UnionCodeGroup.cs
- MostlySingletonList.cs
- ScriptControlDescriptor.cs
- SchemaTableOptionalColumn.cs
- RuleConditionDialog.Designer.cs
- TabItemAutomationPeer.cs
- QuestionEventArgs.cs
- SqlRecordBuffer.cs
- DocumentGrid.cs
- _TransmitFileOverlappedAsyncResult.cs
- EventSourceCreationData.cs
- OracleCommand.cs
- DataServiceConfiguration.cs
- NamespaceCollection.cs
- MenuItemAutomationPeer.cs
- SqlCommandBuilder.cs
- RetrieveVirtualItemEventArgs.cs
- ZoneLinkButton.cs
- UserInitiatedRoutedEventPermission.cs
- PropertyFilterAttribute.cs
- AliasedExpr.cs
- peernodestatemanager.cs
- TemplateManager.cs
- TreeNodeConverter.cs
- XmlChildNodes.cs
- ValueConversionAttribute.cs
- WsatTransactionFormatter.cs
- Registry.cs
- PreservationFileWriter.cs
- ProjectionPlanCompiler.cs
- Mapping.cs
- ForwardPositionQuery.cs
- XmlSchemaAttribute.cs
- ObjectPersistData.cs
- SoapElementAttribute.cs
- ValueType.cs
- ToolStripItemRenderEventArgs.cs
- FileDialog_Vista.cs
- TransactionInformation.cs
- ImageCodecInfo.cs
- Tile.cs
- MarshalDirectiveException.cs
- CompilationUtil.cs
- CapabilitiesPattern.cs
- MetaTableHelper.cs
- EventRecordWrittenEventArgs.cs
- FileSystemWatcher.cs
- TextTreeTextElementNode.cs
- InputMethodStateChangeEventArgs.cs
- Accessors.cs
- BamlWriter.cs
- NativeMethods.cs