Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / PageRanges.cs / 1 / PageRanges.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation. All rights reserved. Module Name: PageRanges.cs Abstract: This file contains the implementation of the PageRange class and the PageRangeSelection enum for page range support in the dialog. Author: Robert Anderson (robertan) 9-May-2005 --*/ using System; using System.Globalization; using System.Windows; namespace System.Windows.Controls { ////// Enumeration of values for page range options. /// public enum PageRangeSelection { ////// All pages are printed. /// AllPages, ////// A set of user defined pages are printed. /// UserPages } ////// This class defines one single page range from /// a start page to an end page. /// public struct PageRange { #region Constructors ////// Constructs an instance of PageRange with one specified page. /// /// /// Single page of this page range. /// public PageRange( int page ) { _pageFrom = page; _pageTo = page; } ////// Constructs an instance of PageRange with specified values. /// /// /// Starting page of this range. /// /// /// Ending page of this range. /// public PageRange( int pageFrom, int pageTo ) { _pageFrom = pageFrom; _pageTo = pageTo; } #endregion Constructors #region Public properties ////// Gets or sets the start page of the page range. /// public int PageFrom { get { return _pageFrom; } set { _pageFrom = value; } } ////// Gets of sets the end page of the page range. /// public int PageTo { get { return _pageTo; } set { _pageTo = value; } } #endregion Public properties #region Private data private int _pageFrom; private int _pageTo; #endregion Private data #region Override methods ////// Converts this PageRange structure to its string representation. /// ////// A string value containing the range. /// public override string ToString( ) { string rangeText; if (_pageTo != _pageFrom) { rangeText = String.Format(CultureInfo.InvariantCulture, SR.Get(SRID.PrintDialogPageRange), _pageFrom, _pageTo); } else { rangeText = _pageFrom.ToString(CultureInfo.InvariantCulture); } return rangeText; } ////// Tests equality between this instance and the specified object. /// /// /// The object to compare this instance to. /// ////// True if obj is equal to this object, else false. /// Returns false if obj is not of type PageRange. /// public override bool Equals( object obj ) { if (obj == null || obj.GetType() != typeof(PageRange)) { return false; } return Equals((PageRange) obj); } ////// Tests equality between this instance and the specified page range. /// /// /// The page range to compare this instance to. /// ////// True if the page range is equal to this object, else false. /// public bool Equals( PageRange pageRange ) { return (pageRange.PageFrom == this.PageFrom) && (pageRange.PageTo == this.PageTo); } ////// Calculates a hash code for this PageRange. /// ////// Returns an integer hashcode for this instance. /// public override int GetHashCode() { return base.GetHashCode(); } ////// Test for equality. /// public static bool operator ==( PageRange pr1, PageRange pr2 ) { return pr1.Equals(pr2); } ////// Test for inequality. /// public static bool operator !=( PageRange pr1, PageRange pr2 ) { return !(pr1.Equals(pr2)); } #endregion Override methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /*++ Copyright (C) 2004 - 2005 Microsoft Corporation. All rights reserved. Module Name: PageRanges.cs Abstract: This file contains the implementation of the PageRange class and the PageRangeSelection enum for page range support in the dialog. Author: Robert Anderson (robertan) 9-May-2005 --*/ using System; using System.Globalization; using System.Windows; namespace System.Windows.Controls { ////// Enumeration of values for page range options. /// public enum PageRangeSelection { ////// All pages are printed. /// AllPages, ////// A set of user defined pages are printed. /// UserPages } ////// This class defines one single page range from /// a start page to an end page. /// public struct PageRange { #region Constructors ////// Constructs an instance of PageRange with one specified page. /// /// /// Single page of this page range. /// public PageRange( int page ) { _pageFrom = page; _pageTo = page; } ////// Constructs an instance of PageRange with specified values. /// /// /// Starting page of this range. /// /// /// Ending page of this range. /// public PageRange( int pageFrom, int pageTo ) { _pageFrom = pageFrom; _pageTo = pageTo; } #endregion Constructors #region Public properties ////// Gets or sets the start page of the page range. /// public int PageFrom { get { return _pageFrom; } set { _pageFrom = value; } } ////// Gets of sets the end page of the page range. /// public int PageTo { get { return _pageTo; } set { _pageTo = value; } } #endregion Public properties #region Private data private int _pageFrom; private int _pageTo; #endregion Private data #region Override methods ////// Converts this PageRange structure to its string representation. /// ////// A string value containing the range. /// public override string ToString( ) { string rangeText; if (_pageTo != _pageFrom) { rangeText = String.Format(CultureInfo.InvariantCulture, SR.Get(SRID.PrintDialogPageRange), _pageFrom, _pageTo); } else { rangeText = _pageFrom.ToString(CultureInfo.InvariantCulture); } return rangeText; } ////// Tests equality between this instance and the specified object. /// /// /// The object to compare this instance to. /// ////// True if obj is equal to this object, else false. /// Returns false if obj is not of type PageRange. /// public override bool Equals( object obj ) { if (obj == null || obj.GetType() != typeof(PageRange)) { return false; } return Equals((PageRange) obj); } ////// Tests equality between this instance and the specified page range. /// /// /// The page range to compare this instance to. /// ////// True if the page range is equal to this object, else false. /// public bool Equals( PageRange pageRange ) { return (pageRange.PageFrom == this.PageFrom) && (pageRange.PageTo == this.PageTo); } ////// Calculates a hash code for this PageRange. /// ////// Returns an integer hashcode for this instance. /// public override int GetHashCode() { return base.GetHashCode(); } ////// Test for equality. /// public static bool operator ==( PageRange pr1, PageRange pr2 ) { return pr1.Equals(pr2); } ////// Test for inequality. /// public static bool operator !=( PageRange pr1, PageRange pr2 ) { return !(pr1.Equals(pr2)); } #endregion Override methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
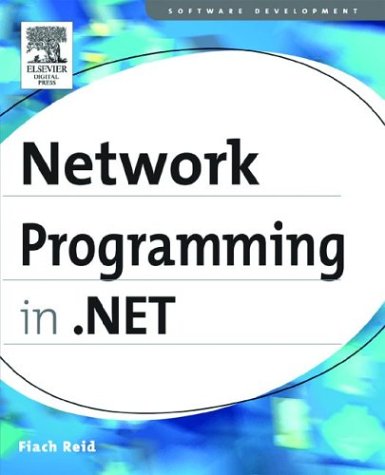
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartEditVerb.cs
- DatePickerTextBox.cs
- CheckBoxList.cs
- WebServiceTypeData.cs
- HScrollBar.cs
- DesignerActionVerbItem.cs
- EFDataModelProvider.cs
- DesignTimeTemplateParser.cs
- EntityKeyElement.cs
- OperationAbortedException.cs
- LinkArea.cs
- LogStream.cs
- WindowsTab.cs
- SchemaTypeEmitter.cs
- CodeObject.cs
- CompileLiteralTextParser.cs
- EntitySetBase.cs
- AsymmetricKeyExchangeFormatter.cs
- InProcStateClientManager.cs
- MiniModule.cs
- OperationParameterInfoCollection.cs
- OdbcConnectionPoolProviderInfo.cs
- TiffBitmapEncoder.cs
- HwndPanningFeedback.cs
- XsdDuration.cs
- ProtocolsSection.cs
- BinaryParser.cs
- FormsAuthenticationEventArgs.cs
- Drawing.cs
- FaultCode.cs
- IdentityHolder.cs
- PageAdapter.cs
- MultiPageTextView.cs
- DataControlField.cs
- TraceHandlerErrorFormatter.cs
- FrameworkContentElement.cs
- MemoryFailPoint.cs
- InlinedLocationReference.cs
- NativeBuffer.cs
- UnsafeNativeMethods.cs
- ResourceAttributes.cs
- TextDpi.cs
- ShaderRenderModeValidation.cs
- TypeElementCollection.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- MD5HashHelper.cs
- InputManager.cs
- ReversePositionQuery.cs
- BaseTemplateBuildProvider.cs
- InputEventArgs.cs
- ToolStripCustomTypeDescriptor.cs
- ViewBox.cs
- ExceptionUtil.cs
- HttpResponseHeader.cs
- UnmanagedMemoryStream.cs
- ListControl.cs
- TextElementEditingBehaviorAttribute.cs
- DispatcherTimer.cs
- AuthenticationServiceManager.cs
- RemotingSurrogateSelector.cs
- WebPartConnectionsDisconnectVerb.cs
- ValidationResult.cs
- PhysicalOps.cs
- Pkcs9Attribute.cs
- BitmapMetadataEnumerator.cs
- ObjectCloneHelper.cs
- GridItemProviderWrapper.cs
- EventItfInfo.cs
- BuildResultCache.cs
- ApplicationTrust.cs
- FtpWebResponse.cs
- RegexReplacement.cs
- DataServiceProcessingPipeline.cs
- WebProxyScriptElement.cs
- CompilationPass2Task.cs
- VectorCollection.cs
- SecurityListenerSettingsLifetimeManager.cs
- TerminatorSinks.cs
- ProgressBar.cs
- GetPageCompletedEventArgs.cs
- SymbolResolver.cs
- ToolStripItemImageRenderEventArgs.cs
- NotifyIcon.cs
- BuildProvider.cs
- PartitionerStatic.cs
- DynamicValidator.cs
- ServiceNameCollection.cs
- PermissionToken.cs
- HTTPNotFoundHandler.cs
- AttachmentCollection.cs
- InteropBitmapSource.cs
- WebServiceResponseDesigner.cs
- SiteMapHierarchicalDataSourceView.cs
- ListenUriMode.cs
- IResourceProvider.cs
- TextTabProperties.cs
- ColorConvertedBitmap.cs
- BitSet.cs
- DBCommandBuilder.cs
- ContextActivityUtils.cs