Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EntitySetBase.cs / 1 / EntitySetBase.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class for representing a entity set /// public abstract class EntitySetBase : MetadataItem { //--------------------------------------------------------------------------------------------- // Possible Future Enhancement: revisit factoring of EntitySetBase and delta between C constructs and S constructs // // Currently, we need to have a way to map an entityset or a relationship set in S space // to the appropriate structures in the store. In order to address this we said we would // add new ItemAttributes (tableName, schemaName and catalogName to the EntitySetBase)... // problem with this is that we are bleading a leaf-level, store specific set of constructs // into the object model for things that may exist at either C or S. // // We need to do this for now to push forward on enabling the conversion but we need to re-examine // whether we should have separate C and S space constructs or some other mechanism for // maintaining this metadata. //--------------------------------------------------------------------------------------------- #region Constructors ////// The constructor for constructing the EntitySet with a given name and an entity type /// /// The name of the EntitySet /// The db schema /// The db table /// The provider specific query that should be used to retrieve the EntitySet /// The entity type of the entities that this entity set type contains ///Thrown if the name or entityType argument is null internal EntitySetBase(string name, string schema, string table, string definingQuery, EntityTypeBase entityType) { EntityUtil.GenericCheckArgumentNull(entityType, "entityType"); EntityUtil.CheckStringArgument(name, "name"); // SQLBU 480236: catalogName, schemaName & tableName are allowed to be null, empty & non-empty _name = name; //---- name of the 'schema' //---- this is used by the SQL Gen utility to support generation of the correct name in the store _schema = schema; //---- name of the 'table' //---- this is used by the SQL Gen utility to support generation of the correct name in the store _table = table; //---- the Provider specific query to use to retrieve the EntitySet data _definingQuery = definingQuery; this.ElementType = entityType; } #endregion #region Fields private EntityContainer _entityContainer; private string _name; private EntityTypeBase _elementType; private string _table; private string _schema; private string _definingQuery; private string _cachedProviderSql; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.EntitySetBase; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return this.Name; } } ////// Gets or sets escaped SQL describing this entity set. /// [MetadataProperty(PrimitiveTypeKind.String, false)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // referenced by System.Data.Entity.Design.dll internal string DefiningQuery { get { return _definingQuery; } set { _definingQuery = value; } } ////// Get and set by the provider only as a convientent place to /// store the created sql fragment that represetnts this entity set /// internal string CachedProviderSql { get { return _cachedProviderSql; } set { _cachedProviderSql = value; } } ////// Gets/Sets the name of this entity set /// ///Thrown if value passed into setter is null ///Thrown if the setter is called when EntitySetBase instance is in ReadOnly state [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } } ////// Returns the entity container of the entity set /// ///Thrown if value passed into setter is null ///Thrown if the setter is called when the EntitySetBase instance or the EntityContainer passed into the setter is in ReadOnly state public EntityContainer EntityContainer { get { return _entityContainer; } } ////// Gets/Sets the entity type of this entity set /// ///if value passed into setter is null ///Thrown if the setter is called when EntitySetBase instance is in ReadOnly state [MetadataProperty(BuiltInTypeKind.EntityTypeBase, false)] public EntityTypeBase ElementType { get { return _elementType; } internal set { EntityUtil.GenericCheckArgumentNull(value, "value"); Util.ThrowIfReadOnly(this); _elementType = value; } } [MetadataProperty(PrimitiveTypeKind.String, false)] internal string Table { get { return _table; } } [MetadataProperty(PrimitiveTypeKind.String, false)] internal string Schema { get { return _schema; } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return Name; } ////// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!this.IsReadOnly) { base.SetReadOnly(); EntityTypeBase elementType = ElementType; if (elementType != null) { elementType.SetReadOnly(); } } } ////// Change the entity container without doing fixup in the entity set collection /// internal void ChangeEntityContainerWithoutCollectionFixup(EntityContainer newEntityContainer) { _entityContainer = newEntityContainer; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class for representing a entity set /// public abstract class EntitySetBase : MetadataItem { //--------------------------------------------------------------------------------------------- // Possible Future Enhancement: revisit factoring of EntitySetBase and delta between C constructs and S constructs // // Currently, we need to have a way to map an entityset or a relationship set in S space // to the appropriate structures in the store. In order to address this we said we would // add new ItemAttributes (tableName, schemaName and catalogName to the EntitySetBase)... // problem with this is that we are bleading a leaf-level, store specific set of constructs // into the object model for things that may exist at either C or S. // // We need to do this for now to push forward on enabling the conversion but we need to re-examine // whether we should have separate C and S space constructs or some other mechanism for // maintaining this metadata. //--------------------------------------------------------------------------------------------- #region Constructors ////// The constructor for constructing the EntitySet with a given name and an entity type /// /// The name of the EntitySet /// The db schema /// The db table /// The provider specific query that should be used to retrieve the EntitySet /// The entity type of the entities that this entity set type contains ///Thrown if the name or entityType argument is null internal EntitySetBase(string name, string schema, string table, string definingQuery, EntityTypeBase entityType) { EntityUtil.GenericCheckArgumentNull(entityType, "entityType"); EntityUtil.CheckStringArgument(name, "name"); // SQLBU 480236: catalogName, schemaName & tableName are allowed to be null, empty & non-empty _name = name; //---- name of the 'schema' //---- this is used by the SQL Gen utility to support generation of the correct name in the store _schema = schema; //---- name of the 'table' //---- this is used by the SQL Gen utility to support generation of the correct name in the store _table = table; //---- the Provider specific query to use to retrieve the EntitySet data _definingQuery = definingQuery; this.ElementType = entityType; } #endregion #region Fields private EntityContainer _entityContainer; private string _name; private EntityTypeBase _elementType; private string _table; private string _schema; private string _definingQuery; private string _cachedProviderSql; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.EntitySetBase; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return this.Name; } } ////// Gets or sets escaped SQL describing this entity set. /// [MetadataProperty(PrimitiveTypeKind.String, false)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // referenced by System.Data.Entity.Design.dll internal string DefiningQuery { get { return _definingQuery; } set { _definingQuery = value; } } ////// Get and set by the provider only as a convientent place to /// store the created sql fragment that represetnts this entity set /// internal string CachedProviderSql { get { return _cachedProviderSql; } set { _cachedProviderSql = value; } } ////// Gets/Sets the name of this entity set /// ///Thrown if value passed into setter is null ///Thrown if the setter is called when EntitySetBase instance is in ReadOnly state [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } } ////// Returns the entity container of the entity set /// ///Thrown if value passed into setter is null ///Thrown if the setter is called when the EntitySetBase instance or the EntityContainer passed into the setter is in ReadOnly state public EntityContainer EntityContainer { get { return _entityContainer; } } ////// Gets/Sets the entity type of this entity set /// ///if value passed into setter is null ///Thrown if the setter is called when EntitySetBase instance is in ReadOnly state [MetadataProperty(BuiltInTypeKind.EntityTypeBase, false)] public EntityTypeBase ElementType { get { return _elementType; } internal set { EntityUtil.GenericCheckArgumentNull(value, "value"); Util.ThrowIfReadOnly(this); _elementType = value; } } [MetadataProperty(PrimitiveTypeKind.String, false)] internal string Table { get { return _table; } } [MetadataProperty(PrimitiveTypeKind.String, false)] internal string Schema { get { return _schema; } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return Name; } ////// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!this.IsReadOnly) { base.SetReadOnly(); EntityTypeBase elementType = ElementType; if (elementType != null) { elementType.SetReadOnly(); } } } ////// Change the entity container without doing fixup in the entity set collection /// internal void ChangeEntityContainerWithoutCollectionFixup(EntityContainer newEntityContainer) { _entityContainer = newEntityContainer; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
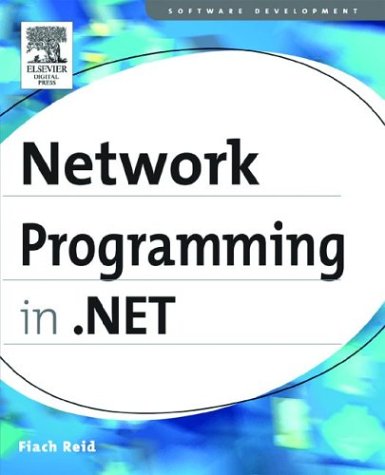
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JoinCqlBlock.cs
- FileSystemInfo.cs
- SafeEventLogReadHandle.cs
- DescendantQuery.cs
- AutomationPatternInfo.cs
- mda.cs
- BaseCodePageEncoding.cs
- WebScriptEndpointElement.cs
- OrthographicCamera.cs
- CursorConverter.cs
- WebPartCloseVerb.cs
- ImportCatalogPart.cs
- CreateUserErrorEventArgs.cs
- ObjectManager.cs
- ElementHostPropertyMap.cs
- BamlWriter.cs
- DBSqlParserTableCollection.cs
- Encoder.cs
- DrawListViewColumnHeaderEventArgs.cs
- UnsafeNativeMethods.cs
- listviewsubitemcollectioneditor.cs
- StrongName.cs
- SizeAnimation.cs
- DiscoveryUtility.cs
- Point3D.cs
- SectionXmlInfo.cs
- ConnectionStringsSection.cs
- ACL.cs
- NavigationProgressEventArgs.cs
- RowUpdatingEventArgs.cs
- DataGridViewColumnConverter.cs
- XamlFigureLengthSerializer.cs
- TextParaLineResult.cs
- Expression.cs
- ClientConvert.cs
- MetadataResolver.cs
- TextParagraph.cs
- PeerContact.cs
- NodeFunctions.cs
- GridViewSelectEventArgs.cs
- TableProviderWrapper.cs
- TypeConvertions.cs
- WriteableBitmap.cs
- SqlUserDefinedAggregateAttribute.cs
- AppearanceEditorPart.cs
- Subtree.cs
- altserialization.cs
- JsonObjectDataContract.cs
- DataGridViewSelectedCellCollection.cs
- SQLMembershipProvider.cs
- RegexGroupCollection.cs
- RequestCacheManager.cs
- Win32.cs
- RoleProviderPrincipal.cs
- DefaultParameterValueAttribute.cs
- elementinformation.cs
- StringTraceRecord.cs
- ReversePositionQuery.cs
- FormViewInsertEventArgs.cs
- XmlRawWriter.cs
- CopyAction.cs
- PrintDialogException.cs
- WebPartAddingEventArgs.cs
- StructuralObject.cs
- Attributes.cs
- XmlLanguageConverter.cs
- Assert.cs
- ComponentResourceKey.cs
- XmlNotation.cs
- DrawingImage.cs
- ColumnMap.cs
- CodeEntryPointMethod.cs
- _ConnectionGroup.cs
- pingexception.cs
- mactripleDES.cs
- XmlUtil.cs
- ScriptingJsonSerializationSection.cs
- HttpCapabilitiesBase.cs
- VirtualPathUtility.cs
- EdgeModeValidation.cs
- ByteStorage.cs
- MediaContextNotificationWindow.cs
- ComponentSerializationService.cs
- ExceptionRoutedEventArgs.cs
- CatalogZone.cs
- MsmqTransportBindingElement.cs
- ProviderException.cs
- ImportCatalogPart.cs
- Matrix3D.cs
- ArrayList.cs
- StaticSiteMapProvider.cs
- FrameworkObject.cs
- GridViewSortEventArgs.cs
- InfoCardBaseException.cs
- BitStack.cs
- DataGridViewCellValueEventArgs.cs
- OleDbRowUpdatedEvent.cs
- NullableDoubleSumAggregationOperator.cs
- IisTraceWebEventProvider.cs
- SystemInfo.cs