Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / ConfigUtil.cs / 1305376 / ConfigUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Threading; using System.Configuration; using System.Xml; using System.Web.Compilation; using System.Web.Util; internal class ConfigUtil { private ConfigUtil() { } internal static void CheckBaseType(Type expectedBaseType, Type userBaseType, string propertyName, ConfigurationElement configElement) { // Make sure the base type is valid if (!expectedBaseType.IsAssignableFrom(userBaseType)) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_type_to_inherit_from, userBaseType.FullName, expectedBaseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, XmlNode node, bool checkAptcaBit, bool ignoreCase) { // We should get either a propertyName/configElement or node, but not both. // They are used only for error reporting. Debug.Assert((propertyName != null) != (node != null)); Type val; try { val = BuildManager.GetType(typeName, true /*throwOnError*/, ignoreCase); } catch (Exception e) { if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (node != null) { throw new ConfigurationErrorsException(e.Message, e, node); } else { if (configElement != null) { throw new ConfigurationErrorsException(e.Message, e, configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } else { throw new ConfigurationErrorsException(e.Message, e); } } } // If we're not in full trust, only allow types that have the APTCA bit (ASURT 139687), // unless the checkAptcaBit flag is false if (checkAptcaBit) { if (node != null) { HttpRuntime.FailIfNoAPTCABit(val, node); } else { HttpRuntime.FailIfNoAPTCABit(val, configElement != null ? configElement.ElementInformation : null, propertyName); } } return val; } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement) { return GetType(typeName, propertyName, configElement, true /*checkAptcaBit*/); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit) { return GetType(typeName, propertyName, configElement, checkAptcaBit, false); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit, bool ignoreCase) { return GetType(typeName, propertyName, configElement, null /*node*/, checkAptcaBit, ignoreCase); } internal static Type GetType(string typeName, XmlNode node) { return GetType(typeName, node, false /*ignoreCase*/); } internal static Type GetType(string typeName, XmlNode node, bool ignoreCase) { return GetType(typeName, null, null, node, true /*checkAptcaBit*/, ignoreCase); } internal static void CheckAssignableType(Type baseType, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static void CheckAssignableType(Type baseType, Type baseType2, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type) && !baseType2.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static bool IsTypeHandlerOrFactory(Type t) { return typeof(IHttpHandler).IsAssignableFrom(t) || typeof(IHttpHandlerFactory).IsAssignableFrom(t); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
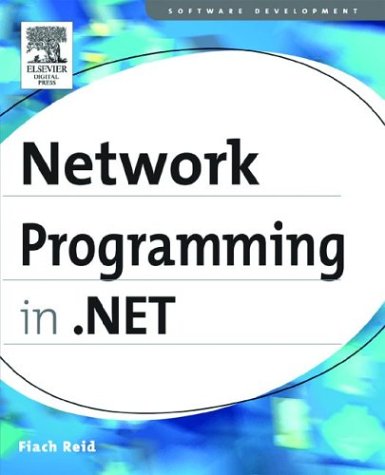
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataBoundControlDesigner.cs
- MaterialGroup.cs
- RemotingAttributes.cs
- RSAPKCS1SignatureFormatter.cs
- QilScopedVisitor.cs
- DodSequenceMerge.cs
- MetadataCollection.cs
- BitmapMetadataEnumerator.cs
- HtmlTernaryTree.cs
- DrawingState.cs
- ObjectListShowCommandsEventArgs.cs
- AnonymousIdentificationModule.cs
- SystemTcpConnection.cs
- ToolStripSystemRenderer.cs
- CommittableTransaction.cs
- SafeSecurityHelper.cs
- CodeConstructor.cs
- DataTableNewRowEvent.cs
- Listen.cs
- ConfigXmlCDataSection.cs
- ProfilePropertySettingsCollection.cs
- HtmlElementCollection.cs
- CodeGotoStatement.cs
- PointHitTestResult.cs
- EventData.cs
- MergePropertyDescriptor.cs
- PenLineCapValidation.cs
- CommandEventArgs.cs
- SecurityElement.cs
- DrawingImage.cs
- HitTestParameters3D.cs
- RequestCachingSection.cs
- CapabilitiesState.cs
- DocumentViewerBaseAutomationPeer.cs
- SemanticAnalyzer.cs
- Registry.cs
- SystemKeyConverter.cs
- ProgressBar.cs
- TableCellCollection.cs
- FormatConvertedBitmap.cs
- StorageConditionPropertyMapping.cs
- PropertyFilterAttribute.cs
- TreePrinter.cs
- IUnknownConstantAttribute.cs
- SqlBulkCopyColumnMappingCollection.cs
- PlatformCulture.cs
- AutomationElementCollection.cs
- HashStream.cs
- TypeValidationEventArgs.cs
- SimpleMailWebEventProvider.cs
- PenThreadWorker.cs
- ObjectStateManagerMetadata.cs
- XmlMemberMapping.cs
- TreeViewCancelEvent.cs
- ValidationSummary.cs
- TabletCollection.cs
- EventProvider.cs
- DispatcherEventArgs.cs
- PropertyGridCommands.cs
- NetDataContractSerializer.cs
- RequestNavigateEventArgs.cs
- SplitterPanel.cs
- HtmlDocument.cs
- Vector3DAnimationUsingKeyFrames.cs
- ValueProviderWrapper.cs
- WebPartsPersonalizationAuthorization.cs
- LocatorGroup.cs
- StateItem.cs
- CanonicalFormWriter.cs
- BaseTemplateParser.cs
- List.cs
- DataRow.cs
- CodeMemberField.cs
- FileUtil.cs
- Selector.cs
- FactoryGenerator.cs
- WeakReferenceEnumerator.cs
- XmlnsDictionary.cs
- QueryAccessibilityHelpEvent.cs
- VirtualPathProvider.cs
- ThicknessKeyFrameCollection.cs
- StyleXamlTreeBuilder.cs
- _SecureChannel.cs
- ProfileService.cs
- AssociationSetMetadata.cs
- TraceData.cs
- Task.cs
- PageCatalogPartDesigner.cs
- UxThemeWrapper.cs
- Menu.cs
- XmlResolver.cs
- ListenerTraceUtility.cs
- TagNameToTypeMapper.cs
- fixedPageContentExtractor.cs
- SerialErrors.cs
- DesignDataSource.cs
- TextTreeDeleteContentUndoUnit.cs
- LinkArea.cs
- AudioDeviceOut.cs
- EmptyEnumerable.cs