Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Remoting / Channels / TCP / CombinedTcpChannel.cs / 1305376 / CombinedTcpChannel.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //========================================================================== // File: CombinedTcpChannel.cs // // Summary: Merges the client and server TCP channels // // Classes: public TcpChannel // //========================================================================= using System; using System.Collections; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; using System.Globalization; using System.Security.Permissions; namespace System.Runtime.Remoting.Channels.Tcp { public class TcpChannel : IChannelReceiver, IChannelSender, ISecurableChannel { private TcpClientChannel _clientChannel = null; // client channel private TcpServerChannel _serverChannel = null; // server channel private int _channelPriority = 1; // channel priority private String _channelName = "tcp"; // channel name public TcpChannel() { _clientChannel = new TcpClientChannel(); // server channel will not be activated. } // TcpChannel public TcpChannel(int port) : this() { _serverChannel = new TcpServerChannel(port); } // TcpChannel public TcpChannel(IDictionary properties, IClientChannelSinkProvider clientSinkProvider, IServerChannelSinkProvider serverSinkProvider) { Hashtable clientData = new Hashtable(); Hashtable serverData = new Hashtable(); bool portFound = false; // divide properties up for respective channels if (properties != null) { foreach (DictionaryEntry entry in properties) { switch ((String)entry.Key) { // general channel properties case "name": _channelName = (String)entry.Value; break; case "priority": _channelPriority = Convert.ToInt32((String)entry.Value, CultureInfo.InvariantCulture); break; case "port": { serverData["port"] = entry.Value; portFound = true; break; } default: clientData[entry.Key] = entry.Value; serverData[entry.Key] = entry.Value; break; } } } _clientChannel = new TcpClientChannel(clientData, clientSinkProvider); if (portFound) _serverChannel = new TcpServerChannel(serverData, serverSinkProvider); } // TcpChannel // // ISecurableChannel implementation // public bool IsSecured { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { if (_clientChannel != null) return _clientChannel.IsSecured; if (_serverChannel != null) return _serverChannel.IsSecured; return false; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] set { if (((IList)ChannelServices.RegisteredChannels).Contains(this)) throw new InvalidOperationException(CoreChannel.GetResourceString("Remoting_InvalidOperation_IsSecuredCannotBeChangedOnRegisteredChannels")); if (_clientChannel != null) _clientChannel.IsSecured = value; if (_serverChannel != null) _serverChannel.IsSecured = value; } } // // IChannel implementation // public int ChannelPriority { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { return _channelPriority; } } // ChannelPriority public String ChannelName { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { return _channelName; } } // ChannelName // returns channelURI and places object uri into out parameter [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public String Parse(String url, out String objectURI) { return TcpChannelHelper.ParseURL(url, out objectURI); } // Parse // // end of IChannel implementation // // // IChannelSender implementation // [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public IMessageSink CreateMessageSink(String url, Object remoteChannelData, out String objectURI) { return _clientChannel.CreateMessageSink(url, remoteChannelData, out objectURI); } // CreateMessageSink // // end of IChannelSender implementation // // // IChannelReceiver implementation // public Object ChannelData { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { if (_serverChannel != null) return _serverChannel.ChannelData; else return null; } } // ChannelData [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public String[] GetUrlsForUri(String objectURI) { if (_serverChannel != null) return _serverChannel.GetUrlsForUri(objectURI); else return null; } // GetUrlsforURI [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public void StartListening(Object data) { if (_serverChannel != null) _serverChannel.StartListening(data); } // StartListening [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public void StopListening(Object data) { if (_serverChannel != null) _serverChannel.StopListening(data); } // StopListening // // IChannelReceiver implementation // } // class TcpChannel } // namespace System.Runtime.Remoting.Channels.Tcp // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //========================================================================== // File: CombinedTcpChannel.cs // // Summary: Merges the client and server TCP channels // // Classes: public TcpChannel // //========================================================================= using System; using System.Collections; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; using System.Globalization; using System.Security.Permissions; namespace System.Runtime.Remoting.Channels.Tcp { public class TcpChannel : IChannelReceiver, IChannelSender, ISecurableChannel { private TcpClientChannel _clientChannel = null; // client channel private TcpServerChannel _serverChannel = null; // server channel private int _channelPriority = 1; // channel priority private String _channelName = "tcp"; // channel name public TcpChannel() { _clientChannel = new TcpClientChannel(); // server channel will not be activated. } // TcpChannel public TcpChannel(int port) : this() { _serverChannel = new TcpServerChannel(port); } // TcpChannel public TcpChannel(IDictionary properties, IClientChannelSinkProvider clientSinkProvider, IServerChannelSinkProvider serverSinkProvider) { Hashtable clientData = new Hashtable(); Hashtable serverData = new Hashtable(); bool portFound = false; // divide properties up for respective channels if (properties != null) { foreach (DictionaryEntry entry in properties) { switch ((String)entry.Key) { // general channel properties case "name": _channelName = (String)entry.Value; break; case "priority": _channelPriority = Convert.ToInt32((String)entry.Value, CultureInfo.InvariantCulture); break; case "port": { serverData["port"] = entry.Value; portFound = true; break; } default: clientData[entry.Key] = entry.Value; serverData[entry.Key] = entry.Value; break; } } } _clientChannel = new TcpClientChannel(clientData, clientSinkProvider); if (portFound) _serverChannel = new TcpServerChannel(serverData, serverSinkProvider); } // TcpChannel // // ISecurableChannel implementation // public bool IsSecured { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { if (_clientChannel != null) return _clientChannel.IsSecured; if (_serverChannel != null) return _serverChannel.IsSecured; return false; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] set { if (((IList)ChannelServices.RegisteredChannels).Contains(this)) throw new InvalidOperationException(CoreChannel.GetResourceString("Remoting_InvalidOperation_IsSecuredCannotBeChangedOnRegisteredChannels")); if (_clientChannel != null) _clientChannel.IsSecured = value; if (_serverChannel != null) _serverChannel.IsSecured = value; } } // // IChannel implementation // public int ChannelPriority { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { return _channelPriority; } } // ChannelPriority public String ChannelName { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { return _channelName; } } // ChannelName // returns channelURI and places object uri into out parameter [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public String Parse(String url, out String objectURI) { return TcpChannelHelper.ParseURL(url, out objectURI); } // Parse // // end of IChannel implementation // // // IChannelSender implementation // [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public IMessageSink CreateMessageSink(String url, Object remoteChannelData, out String objectURI) { return _clientChannel.CreateMessageSink(url, remoteChannelData, out objectURI); } // CreateMessageSink // // end of IChannelSender implementation // // // IChannelReceiver implementation // public Object ChannelData { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] get { if (_serverChannel != null) return _serverChannel.ChannelData; else return null; } } // ChannelData [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public String[] GetUrlsForUri(String objectURI) { if (_serverChannel != null) return _serverChannel.GetUrlsForUri(objectURI); else return null; } // GetUrlsforURI [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public void StartListening(Object data) { if (_serverChannel != null) _serverChannel.StartListening(data); } // StartListening [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure, Infrastructure=true)] public void StopListening(Object data) { if (_serverChannel != null) _serverChannel.StopListening(data); } // StopListening // // IChannelReceiver implementation // } // class TcpChannel } // namespace System.Runtime.Remoting.Channels.Tcp // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
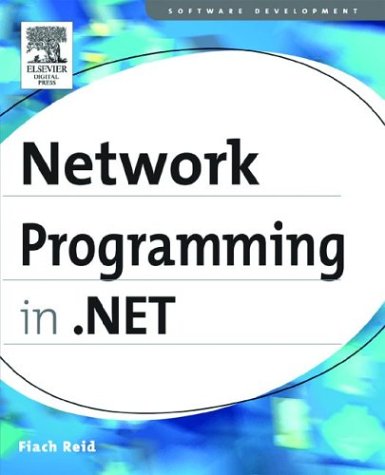
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceContainerNameItem.cs
- ButtonRenderer.cs
- WorkflowOwnershipException.cs
- Tokenizer.cs
- SymmetricAlgorithm.cs
- RangeValidator.cs
- SerializationHelper.cs
- TraceListener.cs
- SqlCacheDependencyDatabase.cs
- SchemaSetCompiler.cs
- SessionIDManager.cs
- ReferencedCollectionType.cs
- BitmapSizeOptions.cs
- SubpageParagraph.cs
- MonitoringDescriptionAttribute.cs
- WeakReadOnlyCollection.cs
- TraceRecords.cs
- SizeChangedEventArgs.cs
- BookmarkScopeManager.cs
- EntityParameter.cs
- ObjectSecurity.cs
- RuleSet.cs
- DESCryptoServiceProvider.cs
- CellRelation.cs
- ResXResourceSet.cs
- IndividualDeviceConfig.cs
- ExpressionBuilder.cs
- StructuredType.cs
- ScriptControlManager.cs
- FixedPageAutomationPeer.cs
- SchemaTypeEmitter.cs
- ExpandCollapsePattern.cs
- CodeEntryPointMethod.cs
- ExtendLockAsyncResult.cs
- Light.cs
- Part.cs
- AdornerHitTestResult.cs
- XmlLoader.cs
- _KerberosClient.cs
- MarshalDirectiveException.cs
- DataGridViewRowsRemovedEventArgs.cs
- GPStream.cs
- InstalledFontCollection.cs
- ContentType.cs
- TextEditorMouse.cs
- __ConsoleStream.cs
- AnchoredBlock.cs
- Rect3D.cs
- CodeGotoStatement.cs
- ConfigurationSettings.cs
- Solver.cs
- WebService.cs
- VarRemapper.cs
- QuotedPairReader.cs
- MulticastDelegate.cs
- HandoffBehavior.cs
- CodeDOMProvider.cs
- BitmapEffect.cs
- MetricEntry.cs
- StringValidator.cs
- followingquery.cs
- VectorCollectionConverter.cs
- TableLayout.cs
- DispatcherSynchronizationContext.cs
- ListenerSessionConnection.cs
- SQLConvert.cs
- TrustSection.cs
- ProcessThread.cs
- CheckStoreFileValidityRequest.cs
- IUnknownConstantAttribute.cs
- ComponentDispatcher.cs
- PassportIdentity.cs
- SqlRowUpdatingEvent.cs
- XmlDataLoader.cs
- ClientSettingsSection.cs
- DecoderReplacementFallback.cs
- SyndicationSerializer.cs
- XmlSerializationGeneratedCode.cs
- ArrayList.cs
- DetailsViewUpdateEventArgs.cs
- LocatorBase.cs
- Stroke.cs
- WithParamAction.cs
- CustomAttributeSerializer.cs
- ReferenceEqualityComparer.cs
- DockAndAnchorLayout.cs
- Vector3DCollectionValueSerializer.cs
- CommandCollectionEditor.cs
- MemberDomainMap.cs
- ReflectionUtil.cs
- TextTreeDeleteContentUndoUnit.cs
- TextEditorCopyPaste.cs
- WebMessageBodyStyleHelper.cs
- ApplicationSecurityInfo.cs
- ToolStripDropDownMenu.cs
- ModifierKeysConverter.cs
- Opcode.cs
- Helper.cs
- CommonGetThemePartSize.cs
- OdbcConnectionPoolProviderInfo.cs