Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Rules / RuleSet.cs / 1305376 / RuleSet.cs
// ---------------------------------------------------------------------------- // Copyright (C) 2005 Microsoft Corporation All Rights Reserved // --------------------------------------------------------------------------- using System.Collections.Generic; using System.ComponentModel; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Compiler; using System.Workflow.Activities.Common; namespace System.Workflow.Activities.Rules { public enum RuleChainingBehavior { None, UpdateOnly, Full }; [Serializable] public class RuleSet { internal const string RuleSetTrackingKey = "RuleSet."; internal string name; internal string description; internal Listrules; internal RuleChainingBehavior behavior = RuleChainingBehavior.Full; private bool runtimeInitialized; private object syncLock = new object(); // keep track of cached data [NonSerialized] private RuleEngine cachedEngine; [NonSerialized] private RuleValidation cachedValidation; public RuleSet() { this.rules = new List (); } public RuleSet(string name) : this() { this.name = name; } public RuleSet(string name, string description) : this(name) { this.description = description; } public string Name { get { return name; } set { if (runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); name = value; } } public string Description { get { return description; } set { if (runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); description = value; } } public RuleChainingBehavior ChainingBehavior { get { return behavior; } set { if (runtimeInitialized) throw new InvalidOperationException(SR.GetString(SR.Error_CanNotChangeAtRuntime)); behavior = value; } } [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public ICollection Rules { get { return rules; } } public bool Validate(RuleValidation validation) { if (validation == null) throw new ArgumentNullException("validation"); // Validate each rule. Dictionary ruleNames = new Dictionary (); foreach (Rule r in rules) { if (!string.IsNullOrEmpty(r.Name)) // invalid names caught when validating the rule { if (ruleNames.ContainsKey(r.Name)) { // Duplicate rule name found. ValidationError error = new ValidationError(Messages.Error_DuplicateRuleName, ErrorNumbers.Error_DuplicateConditions); error.UserData[RuleUserDataKeys.ErrorObject] = r; validation.AddError(error); } else { ruleNames.Add(r.Name, null); } } r.Validate(validation); } if (validation.Errors == null || validation.Errors.Count == 0) return true; return false; } public void Execute(RuleExecution ruleExecution) { // we have no way of knowing if the ruleset has been changed, so no caching done if (ruleExecution == null) throw new ArgumentNullException("ruleExecution"); if (ruleExecution.Validation == null) throw new ArgumentException(SR.GetString(SR.Error_MissingValidationProperty), "ruleExecution"); RuleEngine engine = new RuleEngine(this, ruleExecution.Validation, ruleExecution.ActivityExecutionContext); engine.Execute(ruleExecution); } internal void Execute(Activity activity, ActivityExecutionContext executionContext) { // this can be called from multiple threads if multiple workflows are // running at the same time (only a single workflow is single-threaded) // we want to only lock around the validation and preprocessing, so that // execution can run in parallel. if (activity == null) throw new ArgumentNullException("activity"); Type activityType = activity.GetType(); RuleEngine engine = null; lock (syncLock) { // do we have something useable cached? if ((cachedEngine == null) || (cachedValidation == null) || (cachedValidation.ThisType != activityType)) { // no cache (or its invalid) RuleValidation validation = new RuleValidation(activityType, null); engine = new RuleEngine(this, validation, executionContext); cachedValidation = validation; cachedEngine = engine; } else { // this will happen if the ruleset has already been processed // we can simply use the previously processed engine engine = cachedEngine; } } // when we get here, we have a local RuleEngine all ready to go // we are outside the lock, so these can run in parallel engine.Execute(activity, executionContext); } public RuleSet Clone() { RuleSet newRuleSet = (RuleSet)this.MemberwiseClone(); newRuleSet.runtimeInitialized = false; if (this.rules != null) { newRuleSet.rules = new List (); foreach (Rule r in this.rules) newRuleSet.rules.Add(r.Clone()); } return newRuleSet; } public override bool Equals(object obj) { RuleSet other = obj as RuleSet; if (other == null) return false; if ((this.Name != other.Name) || (this.Description != other.Description) || (this.ChainingBehavior != other.ChainingBehavior) || (this.Rules.Count != other.Rules.Count)) return false; // look similar, compare each rule for (int i = 0; i < this.rules.Count; ++i) { if (!this.rules[i].Equals(other.rules[i])) return false; } return true; } public override int GetHashCode() { return base.GetHashCode(); } internal void OnRuntimeInitialized() { lock (syncLock) { if (runtimeInitialized) return; foreach (Rule rule in rules) { rule.OnRuntimeInitialized(); } runtimeInitialized = true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
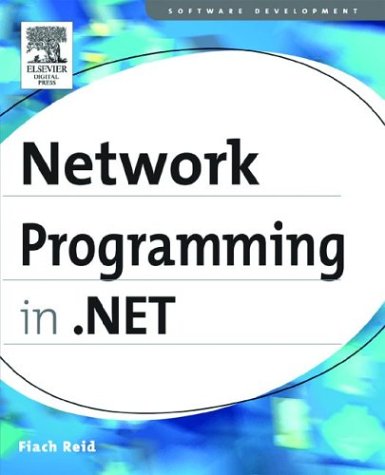
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditorAttribute.cs
- PolicyStatement.cs
- TokenizerHelper.cs
- OutputCacheProfileCollection.cs
- __Error.cs
- ConvertTextFrag.cs
- TextContainer.cs
- RelationshipManager.cs
- FlowLayoutPanel.cs
- COSERVERINFO.cs
- ISFClipboardData.cs
- LOSFormatter.cs
- AttachedAnnotationChangedEventArgs.cs
- ResourcesGenerator.cs
- XMLDiffLoader.cs
- NavigationProperty.cs
- VerificationAttribute.cs
- DynamicRenderer.cs
- EditBehavior.cs
- EntityDataSourceSelectingEventArgs.cs
- SimpleBitVector32.cs
- InstalledVoice.cs
- RowTypeElement.cs
- XmlSchemaElement.cs
- MediaElement.cs
- RawStylusInput.cs
- RootBrowserWindow.cs
- SafeEventLogWriteHandle.cs
- PropertyGrid.cs
- DataGridColumn.cs
- DataControlFieldCollection.cs
- RangeBaseAutomationPeer.cs
- GridViewAutomationPeer.cs
- HttpException.cs
- WhitespaceSignificantCollectionAttribute.cs
- MediaSystem.cs
- BaseAsyncResult.cs
- TextEffectCollection.cs
- ServiceMemoryGates.cs
- PropertyCondition.cs
- XmlAnyElementAttributes.cs
- SqlDataSourceView.cs
- SQLMoney.cs
- BuildManager.cs
- HtmlUtf8RawTextWriter.cs
- CultureInfo.cs
- BinaryCommonClasses.cs
- DbConnectionPoolGroupProviderInfo.cs
- TraceListener.cs
- SqlFunctionAttribute.cs
- SQLDateTimeStorage.cs
- MessageBox.cs
- HexParser.cs
- Walker.cs
- CmsInterop.cs
- DateTimeConverter2.cs
- Grid.cs
- ExtensibleClassFactory.cs
- ACL.cs
- TextElementCollection.cs
- ProvidersHelper.cs
- ResourceBinder.cs
- login.cs
- MenuItemCollection.cs
- AccessedThroughPropertyAttribute.cs
- UIPermission.cs
- TextureBrush.cs
- FilteredDataSetHelper.cs
- ObjectQuery_EntitySqlExtensions.cs
- AttachmentCollection.cs
- DynamicMethod.cs
- SQLConvert.cs
- ResXResourceWriter.cs
- TypeReference.cs
- HitTestWithGeometryDrawingContextWalker.cs
- OLEDB_Util.cs
- RightsManagementInformation.cs
- CodeRemoveEventStatement.cs
- MultiPropertyDescriptorGridEntry.cs
- TrackBarRenderer.cs
- SqlDependency.cs
- DoubleIndependentAnimationStorage.cs
- LingerOption.cs
- ScriptingProfileServiceSection.cs
- XamlRtfConverter.cs
- MetadataConversionError.cs
- DeferredElementTreeState.cs
- DocumentOrderQuery.cs
- StorageConditionPropertyMapping.cs
- SqlWebEventProvider.cs
- ADMembershipProvider.cs
- ConfigViewGenerator.cs
- IPEndPoint.cs
- Win32.cs
- ObfuscationAttribute.cs
- SystemInfo.cs
- ApplicationSettingsBase.cs
- MemberRelationshipService.cs
- VectorKeyFrameCollection.cs
- EntityDataSourceWrapperCollection.cs