Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Policy / PolicyStatement.cs / 1 / PolicyStatement.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // PolicyStatement.cs // // Represents the policy associated with some piece of evidence // namespace System.Security.Policy { using System; using System.Security; using System.Security.Util; using Math = System.Math; using System.Collections; using System.Security.Permissions; using System.Text; using System.Runtime.Remoting.Activation; using System.Globalization; [Flags, Serializable] [System.Runtime.InteropServices.ComVisible(true)] public enum PolicyStatementAttribute { Nothing = 0x0, Exclusive = 0x01, LevelFinal = 0x02, All = 0x03, } [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class PolicyStatement : ISecurityEncodable, ISecurityPolicyEncodable { // The PermissionSet associated with this policy internal PermissionSet m_permSet; // The bitfield of inheritance properties associated with this policy internal PolicyStatementAttribute m_attributes; internal PolicyStatement() { m_permSet = null; m_attributes = PolicyStatementAttribute.Nothing; } public PolicyStatement( PermissionSet permSet ) : this( permSet, PolicyStatementAttribute.Nothing ) { } public PolicyStatement( PermissionSet permSet, PolicyStatementAttribute attributes ) { if (permSet == null) { m_permSet = new PermissionSet( false ); } else { m_permSet = permSet.Copy(); } if (ValidProperties( attributes )) { m_attributes = attributes; } } private PolicyStatement( PermissionSet permSet, PolicyStatementAttribute attributes, bool copy ) { if (permSet != null) { if (copy) m_permSet = permSet.Copy(); else m_permSet = permSet; } else { m_permSet = new PermissionSet( false ); } m_attributes = attributes; } public PermissionSet PermissionSet { get { lock (this) { return m_permSet.Copy(); } } set { lock (this) { if (value == null) { m_permSet = new PermissionSet( false ); } else { m_permSet = value.Copy(); } } } } internal void SetPermissionSetNoCopy( PermissionSet permSet ) { m_permSet = permSet; } internal PermissionSet GetPermissionSetNoCopy() { lock (this) { return m_permSet; } } public PolicyStatementAttribute Attributes { get { return m_attributes; } set { if (ValidProperties( value )) { m_attributes = value; } } } public PolicyStatement Copy() { return new PolicyStatement( m_permSet, m_attributes, true ); } public String AttributeString { get { StringBuilder sb = new StringBuilder(); bool first = true; if (GetFlag((int) PolicyStatementAttribute.Exclusive )) { sb.Append( "Exclusive" ); first = false; } if (GetFlag((int) PolicyStatementAttribute.LevelFinal )) { if (!first) sb.Append( " " ); sb.Append( "LevelFinal" ); } return sb.ToString(); } } private static bool ValidProperties( PolicyStatementAttribute attributes ) { if ((attributes & ~(PolicyStatementAttribute.All)) == 0) { return true; } else { throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidFlag" ) ); } } private bool GetFlag( int flag ) { return (flag & (int)m_attributes) != 0; } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement et ) { FromXml( et, null ); } public SecurityElement ToXml( PolicyLevel level ) { return ToXml( level, false ); } internal SecurityElement ToXml( PolicyLevel level, bool useInternal ) { SecurityElement e = new SecurityElement( "PolicyStatement" ); e.AddAttribute( "version", "1" ); if (m_attributes != PolicyStatementAttribute.Nothing) e.AddAttribute( "Attributes", XMLUtil.BitFieldEnumToString( typeof( PolicyStatementAttribute ), m_attributes ) ); lock (this) { if (m_permSet != null) { if (m_permSet is NamedPermissionSet) { // If the named permission set exists in the parent level of this // policy struct, then just save the name of the permission set. // Otherwise, serialize it like normal. NamedPermissionSet namedPermSet = (NamedPermissionSet)m_permSet; if (level != null && level.GetNamedPermissionSet( namedPermSet.Name ) != null) { e.AddAttribute( "PermissionSetName", namedPermSet.Name ); } else { if (useInternal) e.AddChild( namedPermSet.InternalToXml() ); else e.AddChild( namedPermSet.ToXml() ); } } else { if (useInternal) e.AddChild( m_permSet.InternalToXml() ); else e.AddChild( m_permSet.ToXml() ); } } } return e; } public void FromXml( SecurityElement et, PolicyLevel level ) { FromXml( et, level, false ); } internal void FromXml( SecurityElement et, PolicyLevel level, bool allowInternalOnly ) { if (et == null) throw new ArgumentNullException( "et" ); if (!et.Tag.Equals( "PolicyStatement" )) throw new ArgumentException( String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Argument_InvalidXMLElement" ), "PolicyStatement", this.GetType().FullName ) ); m_attributes = (PolicyStatementAttribute) 0; String strAttributes = et.Attribute( "Attributes" ); if (strAttributes != null) m_attributes = (PolicyStatementAttribute)Enum.Parse( typeof( PolicyStatementAttribute ), strAttributes ); lock (this) { m_permSet = null; if (level != null) { String permSetName = et.Attribute( "PermissionSetName" ); if (permSetName != null) { m_permSet = level.GetNamedPermissionSetInternal( permSetName ); if (m_permSet == null) m_permSet = new PermissionSet( PermissionState.None ); } } if (m_permSet == null) { // There is no provided level, it is not a named permission set, or // the named permission set doesn't exist in the provided level, // so just create the class through reflection and decode normally. SecurityElement e = et.SearchForChildByTag( "PermissionSet" ); if (e != null) { String className = e.Attribute( "class" ); if (className != null && (className.Equals( "NamedPermissionSet" ) || className.Equals( "System.Security.NamedPermissionSet" ))) m_permSet = new NamedPermissionSet( "DefaultName", PermissionState.None ); else m_permSet = new PermissionSet( PermissionState.None ); try { m_permSet.FromXml( e, allowInternalOnly, true ); } catch { // ignore any exceptions from the decode process. // Note: we go ahead and use the permission set anyway. This should be safe since // the decode process should never give permission beyond what a proper decode would have // given. } } else { throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidXML" ) ); } } if (m_permSet == null) m_permSet = new PermissionSet( PermissionState.None ); } } internal void FromXml( SecurityDocument doc, int position, PolicyLevel level, bool allowInternalOnly ) { if (doc == null) throw new ArgumentNullException( "doc" ); if (!doc.GetTagForElement( position ).Equals( "PolicyStatement" )) throw new ArgumentException( String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Argument_InvalidXMLElement" ), "PolicyStatement", this.GetType().FullName ) ); m_attributes = (PolicyStatementAttribute) 0; String strAttributes = doc.GetAttributeForElement( position, "Attributes" ); if (strAttributes != null) m_attributes = (PolicyStatementAttribute)Enum.Parse( typeof( PolicyStatementAttribute ), strAttributes ); lock (this) { m_permSet = null; if (level != null) { String permSetName = doc.GetAttributeForElement( position, "PermissionSetName" ); if (permSetName != null) { m_permSet = level.GetNamedPermissionSetInternal( permSetName ); if (m_permSet == null) m_permSet = new PermissionSet( PermissionState.None ); } } if (m_permSet == null) { // There is no provided level, it is not a named permission set, or // the named permission set doesn't exist in the provided level, // so just create the class through reflection and decode normally. ArrayList childPositions = doc.GetChildrenPositionForElement( position ); int positionPermissionSet = -1; for (int i = 0; i < childPositions.Count; ++i) { if (doc.GetTagForElement( (int)childPositions[i] ).Equals( "PermissionSet" )) { positionPermissionSet = (int)childPositions[i]; } } if (positionPermissionSet != -1) { String className = doc.GetAttributeForElement( positionPermissionSet, "class" ); if (className != null && (className.Equals( "NamedPermissionSet" ) || className.Equals( "System.Security.NamedPermissionSet" ))) m_permSet = new NamedPermissionSet( "DefaultName", PermissionState.None ); else m_permSet = new PermissionSet( PermissionState.None ); m_permSet.FromXml( doc, positionPermissionSet, allowInternalOnly ); } else { throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidXML" ) ); } } if (m_permSet == null) m_permSet = new PermissionSet( PermissionState.None ); } } [System.Runtime.InteropServices.ComVisible(false)] public override bool Equals( Object obj ) { PolicyStatement other = obj as PolicyStatement; if (other == null) return false; if (this.m_attributes != other.m_attributes) return false; if (!Object.Equals( this.m_permSet, other.m_permSet )) return false; return true; } [System.Runtime.InteropServices.ComVisible(false)] public override int GetHashCode() { int accumulator = (int)this.m_attributes; if (m_permSet != null) accumulator = accumulator ^ m_permSet.GetHashCode(); return accumulator; } } }
Link Menu
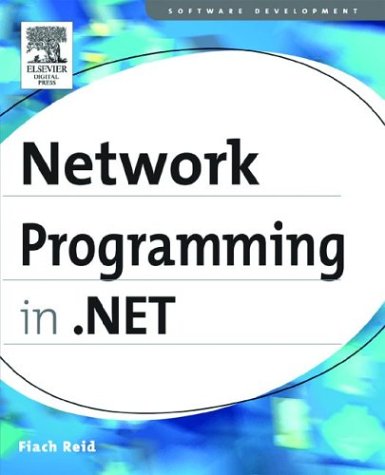
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MergePropertyDescriptor.cs
- TextRange.cs
- ManagementObjectCollection.cs
- WorkflowServiceHostFactory.cs
- PagerStyle.cs
- DefaultProxySection.cs
- ReadOnlyHierarchicalDataSource.cs
- DataGridItemEventArgs.cs
- ToolboxItemAttribute.cs
- ProcessModelInfo.cs
- XmlSchemaSequence.cs
- BitmapVisualManager.cs
- InvokeProviderWrapper.cs
- StatusBarDesigner.cs
- PeerName.cs
- GenericArgumentsUpdater.cs
- WindowsGraphics2.cs
- SatelliteContractVersionAttribute.cs
- CompositeFontFamily.cs
- RefreshEventArgs.cs
- IPGlobalProperties.cs
- WebPart.cs
- InternalControlCollection.cs
- FloaterParaClient.cs
- BindingMAnagerBase.cs
- CommonBehaviorsSection.cs
- WeakReference.cs
- DPCustomTypeDescriptor.cs
- ToolStripGripRenderEventArgs.cs
- XmlTextReaderImplHelpers.cs
- HandleRef.cs
- DecimalConstantAttribute.cs
- AssemblyResourceLoader.cs
- BindingContext.cs
- PlatformCulture.cs
- EncoderBestFitFallback.cs
- XPathDocumentNavigator.cs
- BindingSource.cs
- RegexCode.cs
- SchemaDeclBase.cs
- SessionEndedEventArgs.cs
- COM2PropertyDescriptor.cs
- DESCryptoServiceProvider.cs
- EdgeProfileValidation.cs
- XmlQueryStaticData.cs
- DocumentReferenceCollection.cs
- StrongNamePublicKeyBlob.cs
- CaseInsensitiveComparer.cs
- TextBoxDesigner.cs
- QilTargetType.cs
- CqlIdentifiers.cs
- PageContentAsyncResult.cs
- DataService.cs
- OdbcConnectionPoolProviderInfo.cs
- ContainerVisual.cs
- ToolStripSplitButton.cs
- SafeFileHandle.cs
- Padding.cs
- BindingRestrictions.cs
- RowTypePropertyElement.cs
- MethodToken.cs
- KeyboardEventArgs.cs
- DiagnosticsConfigurationHandler.cs
- AuthorizationContext.cs
- ComPlusSynchronizationContext.cs
- ProgressiveCrcCalculatingStream.cs
- RemoteWebConfigurationHost.cs
- AutomationAttributeInfo.cs
- IdentityModelDictionary.cs
- CodeAssignStatement.cs
- DependencyObjectValidator.cs
- StateManagedCollection.cs
- XamlSerializationHelper.cs
- UnitySerializationHolder.cs
- CLRBindingWorker.cs
- RequestTimeoutManager.cs
- HttpFileCollection.cs
- TraceSection.cs
- CommandID.cs
- RadioButtonBaseAdapter.cs
- Int32Storage.cs
- EnumerableRowCollectionExtensions.cs
- XmlSchemaObject.cs
- DiscoveryReferences.cs
- SpanIndex.cs
- ByteAnimationUsingKeyFrames.cs
- TableLayoutRowStyleCollection.cs
- Bold.cs
- ToolStrip.cs
- StringAnimationBase.cs
- CommentEmitter.cs
- NullExtension.cs
- RightsManagementPermission.cs
- graph.cs
- MoveSizeWinEventHandler.cs
- DesignerWebPartChrome.cs
- MultilineStringConverter.cs
- Validator.cs
- EmptyEnumerator.cs
- SmtpClient.cs