Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activities / Activation / WorkflowServiceHostFactory.cs / 1305376 / WorkflowServiceHostFactory.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activities.Activation { using System.Activities; using System.Activities.XamlIntegration; using System.IO; using System.Globalization; using System.Reflection; using System.Runtime; using System.Runtime.DurableInstancing; using System.Security; using System.Security.Permissions; using System.ServiceModel.Activation; using System.Web; using System.Web.Compilation; using System.Web.Hosting; using System.Xaml; using System.Xml; using System.Xml.Linq; public class WorkflowServiceHostFactory : ServiceHostFactoryBase { public override ServiceHostBase CreateServiceHost(string constructorString, Uri[] baseAddresses) { WorkflowServiceHost serviceHost = null; if (string.IsNullOrEmpty(constructorString)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.WorkflowServiceHostFactoryConstructorStringNotProvided)); } if (baseAddresses == null) { throw FxTrace.Exception.ArgumentNull("baseAddresses"); } if (baseAddresses.Length == 0) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.BaseAddressesNotProvided)); } if (!HostingEnvironment.IsHosted) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.Hosting_ProcessNotExecutingUnderHostedContext("WorkflowServiceHostFactory.CreateServiceHost"))); } // We expect most users will use .xamlx file instead of precompiled assembly // Samples of xamlVirtualPath under all scenarios // constructorString XamlFileBaseLocation xamlVirtualPath // 1. Xamlx direct ~/sub/a.xamlx ~/sub/ ~/sub/a.xamlx // 2. CBA with precompiled servicetypeinfo ~/sub/servicetypeinfo ~/sub/servicetypeinfo * no file will be found // 3. CBA with xamlx sub/a.xamlx ~/ ~/sub/a.xamlx // 4. Svc with precompiled servicetypeinfo ~/sub/servicetypeinfo ~/sub/servicetypeinfo * no file will be found // 5. Svc with Xamlx ../a.xamlx ~/sub/ ~/a.xamlx string xamlVirtualPath = VirtualPathUtility.Combine(AspNetEnvironment.Current.XamlFileBaseLocation, constructorString); Stream activityStream; string compiledCustomString; if (GetServiceFileStreamOrCompiledCustomString(xamlVirtualPath, baseAddresses, out activityStream, out compiledCustomString)) { object serviceObject; using (activityStream) { // BuildManager.GetReferencedAssemblies(); //XmlnsMappingHelper.EnsureMappingPassed(); XamlXmlReaderSettings xamlXmlReaderSettings = new XamlXmlReaderSettings(); xamlXmlReaderSettings.ProvideLineInfo = true; XamlReader wrappedReader = ActivityXamlServices.CreateReader(new XamlXmlReader(XmlReader.Create(activityStream), xamlXmlReaderSettings)); if (TD.XamlServicesLoadStartIsEnabled()) { TD.XamlServicesLoadStart(); } serviceObject = XamlServices.Load(wrappedReader); if (TD.XamlServicesLoadStopIsEnabled()) { TD.XamlServicesLoadStop(); } } WorkflowService service = null; if (serviceObject is Activity) { service = new WorkflowService { Body = (Activity)serviceObject }; } else if (serviceObject is WorkflowService) { service = (WorkflowService)serviceObject; } // If name of the service is not set // service name = xaml file name with extension // service namespace = IIS virtual path // service config name = Activity.DisplayName if (service != null) { if (service.Name == null) { string serviceName = VirtualPathUtility.GetFileName(xamlVirtualPath); string serviceNamespace = String.Format(CultureInfo.InvariantCulture, "/{0}{1}", ServiceHostingEnvironment.SiteName, VirtualPathUtility.GetDirectory(ServiceHostingEnvironment.FullVirtualPath)); service.Name = XName.Get(XmlConvert.EncodeLocalName(serviceName), serviceNamespace); if (service.ConfigurationName == null && service.Body != null) { service.ConfigurationName = XmlConvert.EncodeLocalName(service.Body.DisplayName); } } serviceHost = CreateWorkflowServiceHost(service, baseAddresses); } } else { Type activityType = this.GetTypeFromAssembliesInCurrentDomain(constructorString); if (null == activityType) { activityType = GetTypeFromCompileCustomString(compiledCustomString, constructorString); } if (null == activityType) { //for file-less cases, try in referenced assemblies as CompileCustomString assemblies are empty. BuildManager.GetReferencedAssemblies(); activityType = this.GetTypeFromAssembliesInCurrentDomain(constructorString); } if (null != activityType) { if (!TypeHelper.AreTypesCompatible(activityType, typeof(Activity))) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.TypeNotActivity(activityType.FullName))); } Activity activity = (Activity)Activator.CreateInstance(activityType); serviceHost = CreateWorkflowServiceHost(activity, baseAddresses); } } if (serviceHost == null) { throw FxTrace.Exception.AsError( new InvalidOperationException(SR.CannotResolveConstructorStringToWorkflowType(constructorString))); } //The Description.Name and Description.NameSpace aren't included intentionally - because //in farm scenarios the sole and unique identifier is the service deployment URL ((IDurableInstancingOptions)serviceHost.DurableInstancingOptions).SetScopeName( XName.Get(XmlConvert.EncodeLocalName(VirtualPathUtility.GetFileName(ServiceHostingEnvironment.FullVirtualPath)), String.Format(CultureInfo.InvariantCulture, "/{0}{1}", ServiceHostingEnvironment.SiteName, VirtualPathUtility.GetDirectory(ServiceHostingEnvironment.FullVirtualPath)))); return serviceHost; } Type GetTypeFromAssembliesInCurrentDomain(string typeString) { Type activityType = Type.GetType(typeString, false); if (null == activityType) { foreach (Assembly assembly in AppDomain.CurrentDomain.GetAssemblies()) { activityType = assembly.GetType(typeString, false); if (null != activityType) { break; } } } return activityType; } Type GetTypeFromCompileCustomString(string compileCustomString, string typeString) { if (string.IsNullOrEmpty(compileCustomString)) { return null; } string[] components = compileCustomString.Split('|'); if (components.Length < 3) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidCompiledString(compileCustomString))); } Type activityType = null; for (int i = 3; i < components.Length; i++) { Assembly assembly = Assembly.Load(components[i]); activityType = assembly.GetType(typeString, false); if (activityType != null) { break; } } return activityType; } protected virtual WorkflowServiceHost CreateWorkflowServiceHost(Activity activity, Uri[] baseAddresses) { return new WorkflowServiceHost(activity, baseAddresses); } protected virtual WorkflowServiceHost CreateWorkflowServiceHost(WorkflowService service, Uri[] baseAddresses) { return new WorkflowServiceHost(service, baseAddresses); } // The code is optimized for reducing impersonation // true means serviceFileStream has been set; false means CompiledCustomString has been set [Fx.Tag.SecurityNote(Critical = "Calls SecurityCritical method HostingEnvironmentWrapper.UnsafeImpersonate().", Safe = "Demands unmanaged code permission, disposes impersonation context in a finally, and tightly scopes the usage of the umpersonation token.")] [SecurityPermission(SecurityAction.Demand, UnmanagedCode = true)] [SecuritySafeCritical] bool GetServiceFileStreamOrCompiledCustomString(string virtualPath, Uri[] baseAddresses, out Stream serviceFileStream, out string compiledCustomString) { IDisposable unsafeImpersonate = null; compiledCustomString = null; serviceFileStream = null; try { try { try { } finally { unsafeImpersonate = HostingEnvironmentWrapper.UnsafeImpersonate(); } if (HostingEnvironment.VirtualPathProvider.FileExists(virtualPath)) { serviceFileStream = HostingEnvironment.VirtualPathProvider.GetFile(virtualPath).Open(); return true; } else { if (!AspNetEnvironment.Current.IsConfigurationBased) { compiledCustomString = BuildManager.GetCompiledCustomString(baseAddresses[0].AbsolutePath); } return false; } } finally { if (null != unsafeImpersonate) { unsafeImpersonate.Dispose(); } } } catch { throw; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
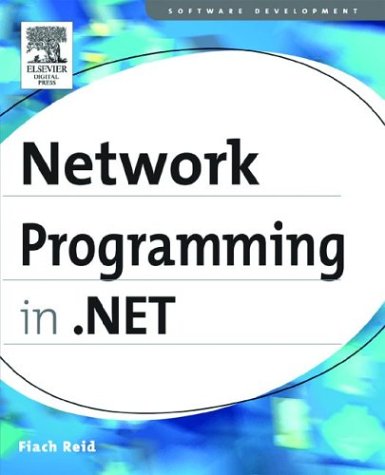
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesigntimeLicenseContextSerializer.cs
- TagPrefixCollection.cs
- XmlUtf8RawTextWriter.cs
- SqlNotificationRequest.cs
- Compilation.cs
- infer.cs
- _NativeSSPI.cs
- DecoratedNameAttribute.cs
- DataGridRelationshipRow.cs
- COSERVERINFO.cs
- LayoutUtils.cs
- MemberInfoSerializationHolder.cs
- CheckBoxBaseAdapter.cs
- Focus.cs
- Size3DConverter.cs
- XmlChildEnumerator.cs
- DesignBinding.cs
- TextDocumentView.cs
- FileNotFoundException.cs
- OutputCacheProfileCollection.cs
- RequiredFieldValidator.cs
- StopStoryboard.cs
- BitmapEffectvisualstate.cs
- PersonalizationAdministration.cs
- FilterQuery.cs
- XmlCodeExporter.cs
- XPathSelectionIterator.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- PropertyInformationCollection.cs
- RepeaterItemEventArgs.cs
- SrgsDocumentParser.cs
- ParameterToken.cs
- SqlNode.cs
- UpdateManifestForBrowserApplication.cs
- ByteStorage.cs
- Win32SafeHandles.cs
- WebPartCollection.cs
- TemplateBindingExpression.cs
- DataBinder.cs
- ExtendedPropertyDescriptor.cs
- WebPart.cs
- ContentControl.cs
- ComplexType.cs
- ProcessHostFactoryHelper.cs
- ConfigUtil.cs
- CodeGenHelper.cs
- HtmlListAdapter.cs
- keycontainerpermission.cs
- HwndKeyboardInputProvider.cs
- BindValidationContext.cs
- CheckBoxStandardAdapter.cs
- FixUpCollection.cs
- DesignerTransaction.cs
- keycontainerpermission.cs
- ChtmlTextWriter.cs
- CodePropertyReferenceExpression.cs
- processwaithandle.cs
- EventWaitHandle.cs
- HttpBufferlessInputStream.cs
- PropertyNames.cs
- ConfigurationSectionGroupCollection.cs
- BufferAllocator.cs
- QilTernary.cs
- ProjectionAnalyzer.cs
- VariantWrapper.cs
- SqlProviderManifest.cs
- MultiPropertyDescriptorGridEntry.cs
- RoleGroupCollection.cs
- DataTableNameHandler.cs
- TextRangeBase.cs
- PageAsyncTaskManager.cs
- BuildProviderCollection.cs
- LinearQuaternionKeyFrame.cs
- GeometryDrawing.cs
- HttpDebugHandler.cs
- exports.cs
- TcpAppDomainProtocolHandler.cs
- WebServiceClientProxyGenerator.cs
- FolderBrowserDialog.cs
- WrappedKeySecurityToken.cs
- unsafenativemethodsother.cs
- Pool.cs
- Tablet.cs
- TerminateWorkflow.cs
- AttributeAction.cs
- InputElement.cs
- RegexCode.cs
- DocumentScope.cs
- CompositeFontParser.cs
- DataObjectMethodAttribute.cs
- XmlSchemaAnnotation.cs
- LinkArea.cs
- XmlDataDocument.cs
- ValueUtilsSmi.cs
- PropertyGridEditorPart.cs
- SequentialUshortCollection.cs
- Char.cs
- oledbmetadatacollectionnames.cs
- DelegateSerializationHolder.cs
- DispatcherExceptionEventArgs.cs