Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / ComplexType.cs / 2 / ComplexType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represent the Edm Complex Type /// public class ComplexType : StructuralType { #region Constructors ////// Initializes a new instance of Complex Type with the given properties /// /// The name of the complex type /// The namespace name of the type /// The version of this type /// dataSpace in which this ComplexType belongs to ///If either name, namespace or version arguments are null internal ComplexType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } ////// Initializes a new instance of Complex Type - required for bootstraping code /// internal ComplexType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } #endregion #region Fields private ReadOnlyMetadataCollection_properties; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.ComplexType; } } ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } #endregion #region Methods /// /// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { if (!Helper.IsEdmProperty(member) && !Helper.IsNavigationProperty(member)) { throw EntityUtil.ComplexTypeInvalidMembers(); } } #endregion } internal sealed class ClrComplexType : ComplexType { ///cached CLR type handle, allowing the Type reference to be GC'd private readonly System.RuntimeTypeHandle _type; ///cached dynamic method to construct a CLR instance private Delegate _constructor; private readonly string _cspaceTypeName; ////// Initializes a new instance of Complex Type with properties from the type. /// /// The CLR type to construct from internal ClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) : base(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace ?? string.Empty, DataSpace.OSpace) { System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(cspaceNamespaceName) && !String.IsNullOrEmpty(cspaceTypeName), "Mapping information must never be null"); _type = clrType.TypeHandle; _cspaceTypeName = cspaceNamespaceName + "." + cspaceTypeName; this.Abstract = clrType.IsAbstract; } internal static ClrComplexType CreateReadonlyClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) { ClrComplexType type = new ClrComplexType(clrType, cspaceNamespaceName, cspaceTypeName); type.SetReadOnly(); return type; } ///cached dynamic method to construct a CLR instance internal Delegate Constructor { get { return _constructor; } set { // It doesn't matter which delegate wins, but only one should be jitted Interlocked.CompareExchange(ref _constructor, value, null); } } ////// internal override System.Type ClrType { get { return Type.GetTypeFromHandle(_type); } } internal string CSpaceTypeName { get { return _cspaceTypeName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represent the Edm Complex Type /// public class ComplexType : StructuralType { #region Constructors ////// Initializes a new instance of Complex Type with the given properties /// /// The name of the complex type /// The namespace name of the type /// The version of this type /// dataSpace in which this ComplexType belongs to ///If either name, namespace or version arguments are null internal ComplexType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } ////// Initializes a new instance of Complex Type - required for bootstraping code /// internal ComplexType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } #endregion #region Fields private ReadOnlyMetadataCollection_properties; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.ComplexType; } } ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } #endregion #region Methods /// /// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { if (!Helper.IsEdmProperty(member) && !Helper.IsNavigationProperty(member)) { throw EntityUtil.ComplexTypeInvalidMembers(); } } #endregion } internal sealed class ClrComplexType : ComplexType { ///cached CLR type handle, allowing the Type reference to be GC'd private readonly System.RuntimeTypeHandle _type; ///cached dynamic method to construct a CLR instance private Delegate _constructor; private readonly string _cspaceTypeName; ////// Initializes a new instance of Complex Type with properties from the type. /// /// The CLR type to construct from internal ClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) : base(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace ?? string.Empty, DataSpace.OSpace) { System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(cspaceNamespaceName) && !String.IsNullOrEmpty(cspaceTypeName), "Mapping information must never be null"); _type = clrType.TypeHandle; _cspaceTypeName = cspaceNamespaceName + "." + cspaceTypeName; this.Abstract = clrType.IsAbstract; } internal static ClrComplexType CreateReadonlyClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) { ClrComplexType type = new ClrComplexType(clrType, cspaceNamespaceName, cspaceTypeName); type.SetReadOnly(); return type; } ///cached dynamic method to construct a CLR instance internal Delegate Constructor { get { return _constructor; } set { // It doesn't matter which delegate wins, but only one should be jitted Interlocked.CompareExchange(ref _constructor, value, null); } } ////// internal override System.Type ClrType { get { return Type.GetTypeFromHandle(_type); } } internal string CSpaceTypeName { get { return _cspaceTypeName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
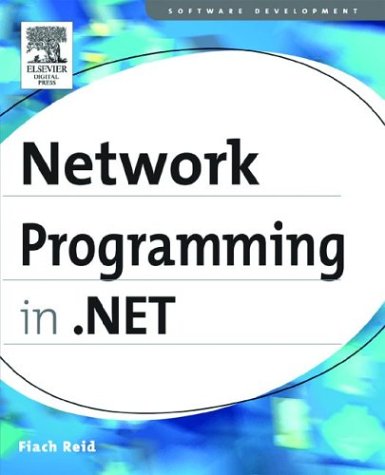
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZoomPercentageConverter.cs
- ValueConversionAttribute.cs
- EnvironmentPermission.cs
- RemotingSurrogateSelector.cs
- IFormattable.cs
- MediaCommands.cs
- AsyncOperationContext.cs
- Itemizer.cs
- AppDomainCompilerProxy.cs
- ListControl.cs
- WizardPanel.cs
- SHA512.cs
- WebPartMenuStyle.cs
- ColumnTypeConverter.cs
- ZipIOCentralDirectoryFileHeader.cs
- CodeDomComponentSerializationService.cs
- QilGenerator.cs
- ContextDataSourceView.cs
- ExpandedProjectionNode.cs
- Comparer.cs
- XmlObjectSerializerReadContextComplex.cs
- InputReport.cs
- MatrixCamera.cs
- TypeValidationEventArgs.cs
- BaseComponentEditor.cs
- FileUtil.cs
- TypeNameHelper.cs
- HandleRef.cs
- MouseEvent.cs
- PopOutPanel.cs
- FixedFindEngine.cs
- FastEncoderStatics.cs
- __ConsoleStream.cs
- UrlAuthFailureHandler.cs
- FixedPageStructure.cs
- NativeMethods.cs
- LinqDataSourceDisposeEventArgs.cs
- TextDecorationUnitValidation.cs
- RemoteWebConfigurationHostServer.cs
- HatchBrush.cs
- MarkupExtensionParser.cs
- IISUnsafeMethods.cs
- MessageFormatterConverter.cs
- SchemaSetCompiler.cs
- loginstatus.cs
- Listen.cs
- Token.cs
- EncodingFallbackAwareXmlTextWriter.cs
- TimersDescriptionAttribute.cs
- HttpCookiesSection.cs
- XAMLParseException.cs
- XmlUTF8TextWriter.cs
- Funcletizer.cs
- Transform3DGroup.cs
- FormsAuthentication.cs
- ClrProviderManifest.cs
- TextureBrush.cs
- DiscoveryMessageSequence.cs
- TreeNodeBinding.cs
- XmlSerializationReader.cs
- TimerElapsedEvenArgs.cs
- Attributes.cs
- XamlStyleSerializer.cs
- KoreanCalendar.cs
- Stackframe.cs
- TemplateAction.cs
- OpacityConverter.cs
- ExpressionEvaluator.cs
- ObjectDataSourceMethodEditor.cs
- FixedDSBuilder.cs
- AsymmetricSecurityProtocol.cs
- ArithmeticException.cs
- OleDbException.cs
- SourceFilter.cs
- FontStretchConverter.cs
- Calendar.cs
- ConcurrentDictionary.cs
- PseudoWebRequest.cs
- XmlNamespaceManager.cs
- DrawItemEvent.cs
- HotSpotCollection.cs
- ListSourceHelper.cs
- OdbcStatementHandle.cs
- PropertyHelper.cs
- StateBag.cs
- processwaithandle.cs
- Activator.cs
- PerCallInstanceContextProvider.cs
- IPEndPoint.cs
- ControlLocalizer.cs
- HwndKeyboardInputProvider.cs
- Vector3DAnimation.cs
- ApplicationException.cs
- IndexerNameAttribute.cs
- PartialTrustVisibleAssembly.cs
- WaitHandleCannotBeOpenedException.cs
- StatusBarPanelClickEvent.cs
- LateBoundBitmapDecoder.cs
- CommandLibraryHelper.cs
- ChannelFactory.cs