Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / TabPage.cs / 1305376 / TabPage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Drawing.Design; using System.ComponentModel.Design; using System.Text; using System.Windows.Forms; using System.Security.Permissions; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; using System.Runtime.InteropServices; using System.Globalization; using System.Windows.Forms.Layout; ////// /// TabPage implements a single page of a tab control. It is essentially /// a Panel with TabItem properties. /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), Designer("System.Windows.Forms.Design.TabPageDesigner, " + AssemblyRef.SystemDesign), ToolboxItem (false), DesignTimeVisible (false), DefaultEvent ("Click"), DefaultProperty ("Text") ] public class TabPage : Panel { private ImageList.Indexer imageIndexer; private string toolTipText = ""; private bool enterFired = false; private bool leaveFired = false; private bool useVisualStyleBackColor = false; ////// /// Constructs an empty TabPage. /// public TabPage () : base() { SetStyle (ControlStyles.CacheText, true); Text = null; } ////// Allows the control to optionally shrink when AutoSize is true. /// [ EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Browsable(false), Localizable(false) ] public override AutoSizeMode AutoSizeMode { get { return AutoSizeMode.GrowOnly; } set { } } ////// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public override bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ///Hide AutoSize: it doesn't make sense for this control ///[SRCategory(SR.CatPropertyChanged), SRDescription(SR.ControlOnAutoSizeChangedDescr)] [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// /// The background color of this control. This is an ambient property and /// will always return a non-null value. /// [ SRCategory(SR.CatAppearance), SRDescription(SR.ControlBackColorDescr) ] public override Color BackColor { get { Color color = base.BackColor; // If some color is Set by the user return that... if (color != DefaultBackColor) { return color; } // If user has not set a color and if XP theming ON and Parent's appearance is Normal, then return the Transparent Color.... TabControl parent = ParentInternal as TabControl; if (Application.RenderWithVisualStyles && UseVisualStyleBackColor && (parent != null && parent.Appearance == TabAppearance.Normal)) { return Color.Transparent; } // return base.Color by default... return color; } set { if (DesignMode) { if (value != Color.Empty) { PropertyDescriptor pd = TypeDescriptor.GetProperties(this)["UseVisualStyleBackColor"]; Debug.Assert(pd != null); if (pd != null) { pd.SetValue(this, false); } } } else { UseVisualStyleBackColor = false; } base.BackColor = value; } } ////// /// Constructs the new instance of the Controls collection objects. Subclasses /// should not call base.CreateControlsInstance. Our version creates a control /// collection that does not support /// protected override Control.ControlCollection CreateControlsInstance () { return new TabPageControlCollection (this); } ////// internal ImageList.Indexer ImageIndexer { get { if (imageIndexer == null) { imageIndexer = new ImageList.Indexer (); } return imageIndexer; } } /// /// /// Returns the imageIndex for the tabPage. This should point to an image /// in the TabControl's associated imageList that will appear on the tab, or be -1. /// [ TypeConverterAttribute (typeof(ImageIndexConverter)), Editor ("System.Windows.Forms.Design.ImageIndexEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Localizable (true), RefreshProperties(RefreshProperties.Repaint), DefaultValue (-1), SRDescription (SR.TabItemImageIndexDescr) ] public int ImageIndex { get { return ImageIndexer.Index; } set { if (value < -1) { throw new ArgumentOutOfRangeException ("ImageIndex", SR.GetString (SR.InvalidLowBoundArgumentEx, "imageIndex", (value).ToString (CultureInfo.CurrentCulture), (-1).ToString(CultureInfo.CurrentCulture))); } TabControl parent = ParentInternal as TabControl; if (parent != null) { this.ImageIndexer.ImageList = parent.ImageList; } this.ImageIndexer.Index = value; UpdateParent (); } } ////// /// Returns the imageIndex for the tabPage. This should point to an image /// in the TabControl's associated imageList that will appear on the tab, or be -1. /// [ TypeConverterAttribute (typeof(ImageKeyConverter)), Editor ("System.Windows.Forms.Design.ImageIndexEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Localizable (true), DefaultValue (""), RefreshProperties(RefreshProperties.Repaint), SRDescription(SR.TabItemImageIndexDescr) ] public string ImageKey { get { return ImageIndexer.Key; } set { this.ImageIndexer.Key = value; TabControl parent = ParentInternal as TabControl; if (parent != null) { this.ImageIndexer.ImageList = parent.ImageList; } UpdateParent (); } } ////// /// Constructs a TabPage with text for the tab. /// public TabPage (string text) : this() { Text = text; } ////// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] public override AnchorStyles Anchor { get { return base.Anchor; } set { base.Anchor = value; } } ///[To be supplied.] ////// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] public override DockStyle Dock { get { return base.Dock; } set { base.Dock = value; } } ///[To be supplied.] ////// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler DockChanged { add { base.DockChanged += value; } remove { base.DockChanged -= value; } } /// /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public bool Enabled { get { return base.Enabled; } set { base.Enabled = value; } } ///[To be supplied.] ////// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler EnabledChanged { add { base.EnabledChanged += value; } remove { base.EnabledChanged -= value; } } /// /// [ DefaultValue (false), SRCategory(SR.CatAppearance), SRDescription (SR.TabItemUseVisualStyleBackColorDescr) ] public bool UseVisualStyleBackColor { get { return useVisualStyleBackColor; } set { useVisualStyleBackColor = value; this.Invalidate(true); } } /// /// // VSWhidbey 94772: Make the Location property non-browsable for the TabPages. [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public Point Location { get { return base.Location; } set { base.Location = value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler LocationChanged { add { base.LocationChanged += value; } remove { base.LocationChanged -= value; } } /// [DefaultValue(typeof(Size), "0, 0")] [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] public override Size MaximumSize { get { return base.MaximumSize; } set { base.MaximumSize = value; } } /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] public override Size MinimumSize { get { return base.MinimumSize; } set { base.MinimumSize = value; } } /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] public new Size PreferredSize { get { return base.PreferredSize; } } /// /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public int TabIndex { get { return base.TabIndex; } set { base.TabIndex = value; } } ///[To be supplied.] ////// Refer VsWhidbey : 440669: This property is required by certain controls (TabPage) to render its transparency using theming API. /// We dont want all controls (that are have transparent BackColor) to use theming API to render its background because it has HUGE PERF cost. /// ///internal override bool RenderTransparencyWithVisualStyles { get { return true; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler TabIndexChanged { add { base.TabIndexChanged += value; } remove { base.TabIndexChanged -= value; } } /// /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public bool TabStop { get { return base.TabStop; } set { base.TabStop = value; } } ///[To be supplied.] ////// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler TabStopChanged { add { base.TabStopChanged += value; } remove { base.TabStopChanged -= value; } } /// /// /// [ Localizable (true), Browsable (true), EditorBrowsable (EditorBrowsableState.Always) ] public override string Text { get { return base.Text; } set { base.Text = value; UpdateParent (); } } ///[To be supplied.] ////// [Browsable (true), EditorBrowsable (EditorBrowsableState.Always)] new public event EventHandler TextChanged { add { base.TextChanged += value; } remove { base.TextChanged -= value; } } /// /// /// The toolTipText for the tab, that will appear when the mouse hovers /// over the tab and the TabControl's showToolTips property is true. /// [ DefaultValue (""), Localizable (true), SRDescription (SR.TabItemToolTipTextDescr) ] public string ToolTipText { get { return toolTipText; } set { if (value == null) { value = ""; } if (value == toolTipText) return; toolTipText = value; UpdateParent (); } } ////// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public bool Visible { get { return base.Visible; } set { base.Visible = value; } } ///[To be supplied.] ////// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler VisibleChanged { add { base.VisibleChanged += value; } remove { base.VisibleChanged -= value; } } /// /// Assigns a new parent control. Sends out the appropriate property change /// notifications for properties that are affected by the change of parent. /// internal override void AssignParent (Control value) { if (value != null && !(value is TabControl)) { throw new ArgumentException (SR.GetString (SR.TABCONTROLTabPageNotOnTabControl, value.GetType ().FullName)); } base.AssignParent (value); } ////// /// Given a component, this retrieves the tab page that it's parented to, or /// null if it's not parented to any tab page. /// public static TabPage GetTabPageOfComponent (Object comp) { if (!(comp is Control)) { return null; } Control c = (Control)comp; while (c != null && !(c is TabPage)) { c = c.ParentInternal; } return (TabPage)c; } ////// /// ///internal NativeMethods.TCITEM_T GetTCITEM () { NativeMethods.TCITEM_T tcitem = new NativeMethods.TCITEM_T (); tcitem.mask = 0; tcitem.pszText = null; tcitem.cchTextMax = 0; tcitem.lParam = IntPtr.Zero; string text = Text; PrefixAmpersands (ref text); if (text != null) { tcitem.mask |= NativeMethods.TCIF_TEXT; tcitem.pszText = text; tcitem.cchTextMax = text.Length; } int imageIndex = ImageIndex; tcitem.mask |= NativeMethods.TCIF_IMAGE; tcitem.iImage = ImageIndexer.ActualIndex; return tcitem; } private void PrefixAmpersands (ref string value) { // Due to a comctl32 problem, ampersands underline the next letter in the // text string, but the accelerators don't work. // So in this function, we prefix ampersands with another ampersand // so that they actually appear as ampersands. // // Sanity check parameter // if (value == null || value.Length == 0) { return; } // If there are no ampersands, we don't need to do anything here // if (value.IndexOf ('&') < 0) { return; } // Insert extra ampersands // StringBuilder newString = new StringBuilder (); for (int i = 0; i < value.Length; ++i) { if (value[i] == '&') { if (i < value.Length - 1 && value[i + 1] == '&') { ++i; // Skip the second ampersand } newString.Append ("&&"); } else { newString.Append (value[i]); } } value = newString.ToString (); } /// /// This is an internal method called by the TabControl to fire the Leave event when TabControl leave occurs. /// internal void FireLeave (EventArgs e) { leaveFired = true; OnLeave (e); } ////// This is an internal method called by the TabControl to fire the Enter event when TabControl leave occurs. /// internal void FireEnter (EventArgs e) { enterFired = true; OnEnter (e); } ////// /// Actually goes and fires the OnEnter event. Inheriting controls /// should use this to know when the event is fired [this is preferable to /// adding an event handler on yourself for this event]. They should, /// however, remember to call base.OnEnter(e); to ensure the event is /// still fired to external listeners /// This listener is overidden so that we can fire SAME ENTER and LEAVE /// events on the TabPage. /// TabPage should fire enter when the focus is on the TABPAGE and not when the control /// within the TabPage gets Focused. /// protected override void OnEnter (EventArgs e) { TabControl parent = ParentInternal as TabControl; if (parent != null) { if (enterFired) { base.OnEnter (e); } enterFired = false; } } ////// /// Actually goes and fires the OnLeave event. Inheriting controls /// should use this to know when the event is fired [this is preferable to /// adding an event handler on yourself for this event]. They should, /// however, remember to call base.OnLeave(e); to ensure the event is /// still fired to external listeners /// This listener is overidden so that we can fire SAME ENTER and LEAVE /// events on the TabPage. /// TabPage should fire enter when the focus is on the TABPAGE and not when the control /// within the TabPage gets Focused. /// Similary the Leave should fire when the TabControl (and hence the TabPage) looses /// Focus. /// protected override void OnLeave (EventArgs e) { TabControl parent = ParentInternal as TabControl; if (parent != null) { if (leaveFired) { base.OnLeave (e); } leaveFired = false; } } ///protected override void OnPaintBackground (PaintEventArgs e) { // VSWhidbey 94759: Utilize the TabRenderer new to Whidbey to draw the tab pages so that the // panels are drawn using the correct visual styles when the application supports using visual // styles. // Does this application utilize Visual Styles? // VSWhidbey 167291: Utilize the UseVisualStyleBackColor property to determine whether or // not the themed background should be utilized. TabControl parent = ParentInternal as TabControl; if (Application.RenderWithVisualStyles && UseVisualStyleBackColor && (parent != null && parent.Appearance == TabAppearance.Normal)) { Color bkcolor = UseVisualStyleBackColor ? Color.Transparent : this.BackColor; Rectangle inflateRect = LayoutUtils.InflateRect(DisplayRectangle, Padding); //HACK: to ensure that the tabpage draws correctly (the border will get clipped and // and gradient fill will match correctly with the tabcontrol). Unfortunately, there is no good way to determine // the padding used on the tabpage. // I would like to use the following below, but GetMargins is busted in the theming API: //VisualStyleRenderer visualStyleRenderer = new VisualStyleRenderer(VisualStyleElement.Tab.Pane.Normal); //Padding themePadding = visualStyleRenderer.GetMargins(e.Graphics, MarginProperty.ContentMargins); //Rectangle rectWithBorder = new Rectangle(inflateRect.X - themePadding.Left, // inflateRect.Y - themePadding.Top, // inflateRect.Width + themePadding.Right + themePadding.Left, // inflateRect.Height + themePadding.Bottom + themePadding.Top); Rectangle rectWithBorder = new Rectangle(inflateRect.X - 4, inflateRect.Y - 2, inflateRect.Width + 8, inflateRect.Height + 6); TabRenderer.DrawTabPage(e.Graphics, rectWithBorder); // Is there a background image to paint? The TabRenderer does not currently support // painting the background image on the panel, so we need to draw it ourselves. if (this.BackgroundImage != null) { ControlPaint.DrawBackgroundImage(e.Graphics, BackgroundImage, bkcolor, BackgroundImageLayout, inflateRect, inflateRect, DisplayRectangle.Location); } } else { base.OnPaintBackground (e); } } /// /// /// overrides main setting of our bounds so that we can control our size and that of our /// TabPages... /// ///protected override void SetBoundsCore (int x, int y, int width, int height, BoundsSpecified specified) { Control parent = ParentInternal; if (parent is TabControl && parent.IsHandleCreated) { Rectangle r = parent.DisplayRectangle; // LayoutEngines send BoundsSpecified.None so they can know they are the ones causing the size change // in the subsequent InitLayout. We need to be careful preserve a None. base.SetBoundsCore (r.X, r.Y, r.Width, r.Height, specified == BoundsSpecified.None ? BoundsSpecified.None : BoundsSpecified.All); } else { base.SetBoundsCore (x, y, width, height, specified); } } /// /// Determines if the Location property needs to be persisted. /// [EditorBrowsable (EditorBrowsableState.Never)] private bool ShouldSerializeLocation () { return Left != 0 || Top != 0; } ////// /// The text property is what is returned for the TabPages default printing. /// public override string ToString () { return "TabPage: {" + Text + "}"; } ////// /// ///internal void UpdateParent () { TabControl parent = ParentInternal as TabControl; if (parent != null) { parent.UpdateTab (this); } } /// /// /// Our control collection will throw an exception if you try to add other tab pages. /// [ComVisible(false)] public class TabPageControlCollection : Control.ControlCollection { ////// /// /// Creates a new TabPageControlCollection. /// public TabPageControlCollection (TabPage owner) : base(owner) { } ////// /// Adds a child control to this control. The control becomes the last control /// in the child control list. If the control is already a child of another /// control it is first removed from that control. The tab page overrides /// this method to ensure that child tab pages are not added to it, as these /// are illegal. /// public override void Add (Control value) { if (value is TabPage) { throw new ArgumentException (SR.GetString (SR.TABCONTROLTabPageOnTabPage)); } base.Add (value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
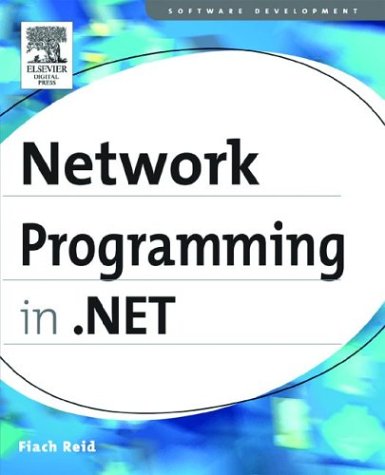
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItemMouseHoverEvent.cs
- DataRowView.cs
- NavigatingCancelEventArgs.cs
- FormsIdentity.cs
- FunctionUpdateCommand.cs
- ChineseLunisolarCalendar.cs
- TdsParserSafeHandles.cs
- VirtualizingPanel.cs
- DataPagerFieldCommandEventArgs.cs
- CodeDOMUtility.cs
- infer.cs
- ErrorFormatter.cs
- CodeAttachEventStatement.cs
- DesignerValidatorAdapter.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- PseudoWebRequest.cs
- FixedDocumentPaginator.cs
- MouseEvent.cs
- AlternateView.cs
- ProfileModule.cs
- CodeDesigner.cs
- WorkflowDesignerMessageFilter.cs
- CleanUpVirtualizedItemEventArgs.cs
- CompiledXpathExpr.cs
- RepeaterItemCollection.cs
- ApplicationInterop.cs
- BorderGapMaskConverter.cs
- DataGridColumnCollection.cs
- UpdateException.cs
- CssClassPropertyAttribute.cs
- SeparatorAutomationPeer.cs
- PropertyNames.cs
- MonitoringDescriptionAttribute.cs
- FrameworkContextData.cs
- Material.cs
- SamlSerializer.cs
- Route.cs
- TypeNameHelper.cs
- SQLConvert.cs
- TextEditorSpelling.cs
- XamlToRtfWriter.cs
- ProgressPage.cs
- Panel.cs
- BitmapEffectState.cs
- NullReferenceException.cs
- ByteFacetDescriptionElement.cs
- PolicyStatement.cs
- CalendarDay.cs
- SQLInt64Storage.cs
- FixedTextView.cs
- DPCustomTypeDescriptor.cs
- GeometryGroup.cs
- MailDefinitionBodyFileNameEditor.cs
- RtfToXamlReader.cs
- PropertyCollection.cs
- elementinformation.cs
- MetricEntry.cs
- Dispatcher.cs
- AuthenticationModuleElement.cs
- Permission.cs
- RelationshipManager.cs
- EventRoute.cs
- MouseEvent.cs
- DefaultValueAttribute.cs
- WebPartDisplayModeCancelEventArgs.cs
- ResourceExpression.cs
- sqlinternaltransaction.cs
- DataGridViewColumnConverter.cs
- DetailsViewUpdatedEventArgs.cs
- XamlFilter.cs
- CryptoConfig.cs
- DataObject.cs
- HandleCollector.cs
- InstanceNotReadyException.cs
- SingleStorage.cs
- MembershipUser.cs
- BaseTemplateParser.cs
- EventToken.cs
- FrameworkRichTextComposition.cs
- ThreadStartException.cs
- QueryExecutionOption.cs
- StringSource.cs
- DesignerAutoFormatCollection.cs
- SuppressMergeCheckAttribute.cs
- ColorAnimationBase.cs
- ConfigurationPropertyAttribute.cs
- ComPlusServiceHost.cs
- StateDesigner.Layouts.cs
- DefaultParameterValueAttribute.cs
- ResourceDescriptionAttribute.cs
- DbModificationCommandTree.cs
- PropertyEntry.cs
- XmlnsDictionary.cs
- ReaderWriterLock.cs
- UIElement3DAutomationPeer.cs
- GradientBrush.cs
- UrlPath.cs
- XmlAnyElementAttributes.cs
- SocketConnection.cs
- ComboBoxAutomationPeer.cs