Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / Emitters / NamespaceEmitter.cs / 1 / NamespaceEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// This class is responsible for Emitting the code to create the CLR namespace container and assembly level attributes /// internal sealed class NamespaceEmitter : Emitter { #region Static Fields private static Pair[] EmitterCreators = new Pair [] { (new Pair (typeof(EntityType), delegate (ClientApiGenerator generator1, GlobalItem element) { return new EntityTypeEmitter(generator1,(EntityType)element); })), (new Pair (typeof(ComplexType), delegate (ClientApiGenerator generator2, GlobalItem element) { return new ComplexTypeEmitter(generator2,(ComplexType)element); })), (new Pair (typeof(EntityContainer), delegate (ClientApiGenerator generator3, GlobalItem element) { return new EntityContainerEmitter(generator3,(EntityContainer)element); })), (new Pair (typeof(AssociationType), delegate (ClientApiGenerator generator4, GlobalItem element) { return new AssociationTypeEmitter(generator4,(AssociationType)element); })), }; #endregion #region Private Fields private string _targetFilePath; private string _namespacePrefix; #endregion #region Public Methods /// /// /// /// public NamespaceEmitter(ClientApiGenerator generator, string namespacePrefix, string targetFilePath) : base(generator) { _targetFilePath = targetFilePath != null ? targetFilePath : string.Empty; _namespacePrefix = namespacePrefix; } ////// Creates the CodeTypeDeclarations necessary to generate the code /// public void Emit() { DictionaryusedClassName = new Dictionary (StringComparer.Ordinal); HashSet used = new HashSet (); used.Add("Edm"); EntityContainer defaultContainer = this.Generator.EdmItemCollection .GetItems () .FirstOrDefault(c => IsDefaultContainer(c)); this.Generator.DefaultContainerNamespace = this.Generator.GetContainerNamespace(defaultContainer); this.BuildNamespaceMap(this.Generator.DefaultContainerNamespace); foreach (EntityContainer container in this.Generator.EdmItemCollection.GetItems ()) { string namespaceName = this.Generator.GetContainerNamespace(container); used.Add(namespaceName); List items = new List (); items.Add(container); foreach (GlobalItem element in Generator.GetSourceTypes()) { EdmType edmType = (element as EdmType); if ((null != edmType) && (edmType.NamespaceName == namespaceName)) { items.Add(edmType); } } if (!string.IsNullOrEmpty(_namespacePrefix)) { if (string.IsNullOrEmpty(namespaceName) || IsDefaultContainer(container)) { namespaceName = _namespacePrefix; } else { namespaceName = _namespacePrefix + "." + namespaceName; } } Emit(usedClassName, namespaceName, items); } foreach (string namespaceName in (from x in this.Generator.EdmItemCollection.GetItems () select x.NamespaceName).Distinct()) { if (used.Add(namespaceName)) { List items = new List (); foreach (GlobalItem element in Generator.GetSourceTypes()) { EdmType edmType = (element as EdmType); if ((null != edmType) && (edmType.NamespaceName == namespaceName)) { items.Add(edmType); } } if (0 < items.Count) { string clientNamespace = namespaceName; if (_namespacePrefix != null) { clientNamespace = this.Generator.GetClientTypeNamespace(namespaceName); } this.Emit(usedClassName, clientNamespace, items); } } } } private void Emit(Dictionary usedClassName, string namespaceName, List items) { // it is a valid scenario for namespaceName to be empty //string namespaceName = Generator.SourceObjectNamespaceName; Generator.SourceEdmNamespaceName = namespaceName; // emit the namespace definition CodeNamespace codeNamespace = new CodeNamespace(namespaceName); // output some boiler plate comments string comments = Strings.NamespaceComments( System.IO.Path.GetFileName(_targetFilePath), DateTime.Now.ToString(System.Globalization.CultureInfo.CurrentCulture)); CommentEmitter.EmitComments(CommentEmitter.GetFormattedLines(comments, false), codeNamespace.Comments, false); CompileUnit.Namespaces.Add(codeNamespace); // Emit the classes in the schema foreach (GlobalItem element in items) { if (AddElementNameToCache(element, usedClassName)) { SchemaTypeEmitter emitter = CreateElementEmitter(element); CodeTypeDeclarationCollection typeDeclaration = emitter.EmitApiClass(); if (typeDeclaration.Count > 0) { codeNamespace.Types.AddRange(typeDeclaration); } } } Generator.SourceEdmNamespaceName = null; } #endregion #region Private Properties /// /// Gets the compile unit (top level codedom object) /// ///private CodeCompileUnit CompileUnit { get { return Generator.CompileUnit; } } #endregion private bool AddElementNameToCache(GlobalItem element, Dictionary cache) { if (element.BuiltInTypeKind == BuiltInTypeKind.EntityContainer) { return TryAddNameToCache((element as EntityContainer).Name, element.BuiltInTypeKind.ToString(), cache); } else if (element.BuiltInTypeKind == BuiltInTypeKind.EntityType || element.BuiltInTypeKind == BuiltInTypeKind.ComplexType || element.BuiltInTypeKind == BuiltInTypeKind.AssociationType) { return TryAddNameToCache((element as StructuralType).Name, element.BuiltInTypeKind.ToString(), cache); } return true; } private bool TryAddNameToCache(string name, string type, Dictionary cache) { if (!cache.ContainsKey(name)) { cache.Add(name, type); } else { this.Generator.AddError(Strings.DuplicateClassName(type, name, cache[name]), ModelBuilderErrorCode.DuplicateClassName, EdmSchemaErrorSeverity.Error); return false; } return true; } /// /// Create an Emitter for a schema type element /// /// ///private SchemaTypeEmitter CreateElementEmitter(GlobalItem element) { Type typeOfElement = element.GetType(); foreach (Pair pair in EmitterCreators) { if (pair.First.IsAssignableFrom(typeOfElement)) return pair.Second(Generator, element); } return null; } private delegate SchemaTypeEmitter CreateEmitter(ClientApiGenerator generator, GlobalItem item); /// /// Reponsible for relating two objects together into a pair /// ////// private class Pair { public T1 First; public T2 Second; internal Pair(T1 first, T2 second) { First = first; Second = second; } } private bool IsDefaultContainer(EntityContainer container) { if (container == null) return false; MetadataProperty prop; if (container.MetadataProperties.TryGetValue("http://schemas.microsoft.com/ado/2007/08/dataservices/metadata:IsDefaultEntityContainer", false, out prop)) { return prop != null && prop.Value != null && string.Equals(prop.Value.ToString(), "true", StringComparison.OrdinalIgnoreCase); } return false; } private void BuildNamespaceMap(string defaultContainerNamespace) { if (!string.IsNullOrEmpty(this.Generator.NamespacePrefix)) { var namespaceMap = this.Generator.GetSourceTypes() .OfType () .Select(et => et.NamespaceName) .Distinct() .Select(ns => new { ServiceNamespace = ns, ClientNamespace = ((ns == defaultContainerNamespace) ? _namespacePrefix : _namespacePrefix + "." + ns) }); foreach (var i in namespaceMap) { this.Generator.NamespaceMap.Add(i.ServiceNamespace, i.ClientNamespace); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// This class is responsible for Emitting the code to create the CLR namespace container and assembly level attributes /// internal sealed class NamespaceEmitter : Emitter { #region Static Fields private static Pair[] EmitterCreators = new Pair [] { (new Pair (typeof(EntityType), delegate (ClientApiGenerator generator1, GlobalItem element) { return new EntityTypeEmitter(generator1,(EntityType)element); })), (new Pair (typeof(ComplexType), delegate (ClientApiGenerator generator2, GlobalItem element) { return new ComplexTypeEmitter(generator2,(ComplexType)element); })), (new Pair (typeof(EntityContainer), delegate (ClientApiGenerator generator3, GlobalItem element) { return new EntityContainerEmitter(generator3,(EntityContainer)element); })), (new Pair (typeof(AssociationType), delegate (ClientApiGenerator generator4, GlobalItem element) { return new AssociationTypeEmitter(generator4,(AssociationType)element); })), }; #endregion #region Private Fields private string _targetFilePath; private string _namespacePrefix; #endregion #region Public Methods /// /// /// /// public NamespaceEmitter(ClientApiGenerator generator, string namespacePrefix, string targetFilePath) : base(generator) { _targetFilePath = targetFilePath != null ? targetFilePath : string.Empty; _namespacePrefix = namespacePrefix; } ////// Creates the CodeTypeDeclarations necessary to generate the code /// public void Emit() { DictionaryusedClassName = new Dictionary (StringComparer.Ordinal); HashSet used = new HashSet (); used.Add("Edm"); EntityContainer defaultContainer = this.Generator.EdmItemCollection .GetItems () .FirstOrDefault(c => IsDefaultContainer(c)); this.Generator.DefaultContainerNamespace = this.Generator.GetContainerNamespace(defaultContainer); this.BuildNamespaceMap(this.Generator.DefaultContainerNamespace); foreach (EntityContainer container in this.Generator.EdmItemCollection.GetItems ()) { string namespaceName = this.Generator.GetContainerNamespace(container); used.Add(namespaceName); List items = new List (); items.Add(container); foreach (GlobalItem element in Generator.GetSourceTypes()) { EdmType edmType = (element as EdmType); if ((null != edmType) && (edmType.NamespaceName == namespaceName)) { items.Add(edmType); } } if (!string.IsNullOrEmpty(_namespacePrefix)) { if (string.IsNullOrEmpty(namespaceName) || IsDefaultContainer(container)) { namespaceName = _namespacePrefix; } else { namespaceName = _namespacePrefix + "." + namespaceName; } } Emit(usedClassName, namespaceName, items); } foreach (string namespaceName in (from x in this.Generator.EdmItemCollection.GetItems () select x.NamespaceName).Distinct()) { if (used.Add(namespaceName)) { List items = new List (); foreach (GlobalItem element in Generator.GetSourceTypes()) { EdmType edmType = (element as EdmType); if ((null != edmType) && (edmType.NamespaceName == namespaceName)) { items.Add(edmType); } } if (0 < items.Count) { string clientNamespace = namespaceName; if (_namespacePrefix != null) { clientNamespace = this.Generator.GetClientTypeNamespace(namespaceName); } this.Emit(usedClassName, clientNamespace, items); } } } } private void Emit(Dictionary usedClassName, string namespaceName, List items) { // it is a valid scenario for namespaceName to be empty //string namespaceName = Generator.SourceObjectNamespaceName; Generator.SourceEdmNamespaceName = namespaceName; // emit the namespace definition CodeNamespace codeNamespace = new CodeNamespace(namespaceName); // output some boiler plate comments string comments = Strings.NamespaceComments( System.IO.Path.GetFileName(_targetFilePath), DateTime.Now.ToString(System.Globalization.CultureInfo.CurrentCulture)); CommentEmitter.EmitComments(CommentEmitter.GetFormattedLines(comments, false), codeNamespace.Comments, false); CompileUnit.Namespaces.Add(codeNamespace); // Emit the classes in the schema foreach (GlobalItem element in items) { if (AddElementNameToCache(element, usedClassName)) { SchemaTypeEmitter emitter = CreateElementEmitter(element); CodeTypeDeclarationCollection typeDeclaration = emitter.EmitApiClass(); if (typeDeclaration.Count > 0) { codeNamespace.Types.AddRange(typeDeclaration); } } } Generator.SourceEdmNamespaceName = null; } #endregion #region Private Properties /// /// Gets the compile unit (top level codedom object) /// ///private CodeCompileUnit CompileUnit { get { return Generator.CompileUnit; } } #endregion private bool AddElementNameToCache(GlobalItem element, Dictionary cache) { if (element.BuiltInTypeKind == BuiltInTypeKind.EntityContainer) { return TryAddNameToCache((element as EntityContainer).Name, element.BuiltInTypeKind.ToString(), cache); } else if (element.BuiltInTypeKind == BuiltInTypeKind.EntityType || element.BuiltInTypeKind == BuiltInTypeKind.ComplexType || element.BuiltInTypeKind == BuiltInTypeKind.AssociationType) { return TryAddNameToCache((element as StructuralType).Name, element.BuiltInTypeKind.ToString(), cache); } return true; } private bool TryAddNameToCache(string name, string type, Dictionary cache) { if (!cache.ContainsKey(name)) { cache.Add(name, type); } else { this.Generator.AddError(Strings.DuplicateClassName(type, name, cache[name]), ModelBuilderErrorCode.DuplicateClassName, EdmSchemaErrorSeverity.Error); return false; } return true; } /// /// Create an Emitter for a schema type element /// /// ///private SchemaTypeEmitter CreateElementEmitter(GlobalItem element) { Type typeOfElement = element.GetType(); foreach (Pair pair in EmitterCreators) { if (pair.First.IsAssignableFrom(typeOfElement)) return pair.Second(Generator, element); } return null; } private delegate SchemaTypeEmitter CreateEmitter(ClientApiGenerator generator, GlobalItem item); /// /// Reponsible for relating two objects together into a pair /// ////// private class Pair { public T1 First; public T2 Second; internal Pair(T1 first, T2 second) { First = first; Second = second; } } private bool IsDefaultContainer(EntityContainer container) { if (container == null) return false; MetadataProperty prop; if (container.MetadataProperties.TryGetValue("http://schemas.microsoft.com/ado/2007/08/dataservices/metadata:IsDefaultEntityContainer", false, out prop)) { return prop != null && prop.Value != null && string.Equals(prop.Value.ToString(), "true", StringComparison.OrdinalIgnoreCase); } return false; } private void BuildNamespaceMap(string defaultContainerNamespace) { if (!string.IsNullOrEmpty(this.Generator.NamespacePrefix)) { var namespaceMap = this.Generator.GetSourceTypes() .OfType () .Select(et => et.NamespaceName) .Distinct() .Select(ns => new { ServiceNamespace = ns, ClientNamespace = ((ns == defaultContainerNamespace) ? _namespacePrefix : _namespacePrefix + "." + ns) }); foreach (var i in namespaceMap) { this.Generator.NamespaceMap.Add(i.ServiceNamespace, i.ClientNamespace); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
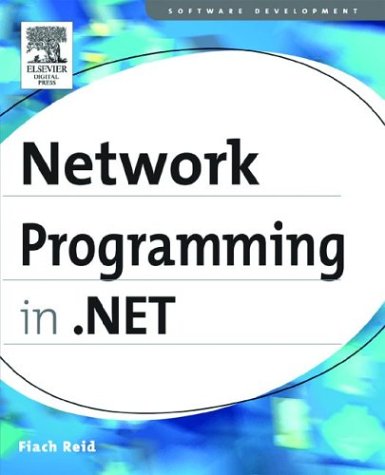
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusCaptureWithinProperty.cs
- EtwProvider.cs
- StatusBarDrawItemEvent.cs
- UIntPtr.cs
- AudioStateChangedEventArgs.cs
- IISUnsafeMethods.cs
- PropertyPathConverter.cs
- ProxyHelper.cs
- DbParameterHelper.cs
- XmlSchemaCollection.cs
- InheritanceContextChangedEventManager.cs
- ContactManager.cs
- InteropDesigner.xaml.cs
- MemberInfoSerializationHolder.cs
- PassportAuthentication.cs
- ComponentEvent.cs
- DataRowComparer.cs
- BufferAllocator.cs
- ReadOnlyCollection.cs
- UnicastIPAddressInformationCollection.cs
- RijndaelCryptoServiceProvider.cs
- SBCSCodePageEncoding.cs
- Facet.cs
- DataListItemEventArgs.cs
- AddressAccessDeniedException.cs
- TransactionFlowAttribute.cs
- SkinBuilder.cs
- bindurihelper.cs
- ResourceManager.cs
- ResourcesGenerator.cs
- GetWinFXPath.cs
- Thread.cs
- LazyInitializer.cs
- PrintPreviewDialog.cs
- ToolStripDropDown.cs
- Hash.cs
- DataGridDesigner.cs
- RemotingConfigParser.cs
- ConditionalAttribute.cs
- FolderBrowserDialogDesigner.cs
- SimpleMailWebEventProvider.cs
- XmlSerializerSection.cs
- SizeFConverter.cs
- NonBatchDirectoryCompiler.cs
- WizardPanel.cs
- Sequence.cs
- IIS7WorkerRequest.cs
- SystemTcpConnection.cs
- PrintPageEvent.cs
- DocumentXmlWriter.cs
- ModelEditingScope.cs
- LinqDataSourceSelectEventArgs.cs
- Compiler.cs
- ObjectViewFactory.cs
- CssStyleCollection.cs
- ToolStripSettings.cs
- TextServicesCompartmentEventSink.cs
- AuthenticationService.cs
- AccessViolationException.cs
- AncestorChangedEventArgs.cs
- ServiceControllerDesigner.cs
- PersonalizationState.cs
- QueryTask.cs
- KeyInfo.cs
- SQLInt16.cs
- FunctionUpdateCommand.cs
- CalendarData.cs
- ACE.cs
- BatchStream.cs
- ValidationEventArgs.cs
- CompoundFileIOPermission.cs
- PointConverter.cs
- DataServiceRequestOfT.cs
- DataViewSetting.cs
- RoutedEvent.cs
- DesignConnection.cs
- SectionVisual.cs
- KernelTypeValidation.cs
- ProfilePropertyMetadata.cs
- IPAddressCollection.cs
- UIElementHelper.cs
- RouteParameter.cs
- Compiler.cs
- ContextToken.cs
- DataObject.cs
- Nodes.cs
- StyleTypedPropertyAttribute.cs
- AxImporter.cs
- DataShape.cs
- TableLayout.cs
- TemplateFactory.cs
- Classification.cs
- TypeBuilder.cs
- ArgumentFixer.cs
- TextTreeTextNode.cs
- CodeTypeParameterCollection.cs
- GroupBoxAutomationPeer.cs
- RecognizerStateChangedEventArgs.cs
- BaseValidator.cs
- IdentityHolder.cs