Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Util / ProviderUtil.cs / 1 / ProviderUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Sec * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.Util { using System.Collections.Specialized; using System.Web.Mail; using System.Configuration; using System.Globalization; internal static class ProviderUtil { internal const int Infinite = Int32.MaxValue; internal static void GetAndRemoveStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { val = config.Get(attrib); config.Remove(attrib); } internal static void GetAndRemovePositiveAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { GetPositiveAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetPositiveAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { string s = config.Get(attrib); int t; if (s == null) { return; } try { t = Convert.ToInt32(s, CultureInfo.InvariantCulture); } catch (Exception e){ if (e is ArgumentException || e is FormatException || e is OverflowException) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } else { throw; } } if (t < 0) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } val = t; } internal static void GetAndRemovePositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { GetPositiveOrInfiniteAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetPositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { string s = config.Get(attrib); int t; if (s == null) { return; } if (s == "Infinite") { t = ProviderUtil.Infinite; } else { try { t = Convert.ToInt32(s, CultureInfo.InvariantCulture); } catch (Exception e){ if (e is ArgumentException || e is FormatException || e is OverflowException) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } else { throw; } } if (t < 0) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } } val = t; } internal static void GetAndRemoveNonZeroPositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { GetNonZeroPositiveOrInfiniteAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetNonZeroPositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { string s = config.Get(attrib); int t; if (s == null) { return; } if (s == "Infinite") { t = ProviderUtil.Infinite; } else { try { t = Convert.ToInt32(s, CultureInfo.InvariantCulture); } catch (Exception e){ if (e is ArgumentException || e is FormatException || e is OverflowException) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_non_zero_positive_attributes, attrib, providerName)); } else { throw; } } if (t <= 0) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_non_zero_positive_attributes, attrib, providerName)); } } val = t; } internal static void GetAndRemoveBooleanAttribute(NameValueCollection config, string attrib, string providerName, ref bool val) { GetBooleanAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetBooleanAttribute(NameValueCollection config, string attrib, string providerName, ref bool val) { string s = config.Get(attrib); if (s == null) { return; } if (s == "true") { val = true; } else if (s == "false") { val = false; } else { throw new ConfigurationErrorsException(SR.GetString(SR.Invalid_provider_attribute, attrib, providerName, s)); } } internal static void GetAndRemoveRequiredNonEmptyStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { GetRequiredNonEmptyStringAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetRequiredNonEmptyStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { GetNonEmptyStringAttributeInternal(config, attrib, providerName, ref val, true); } #if UNUSED_CODE internal static void GetAndRemoveNonEmptyStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { GetNonEmptyStringAttributeInternal(config, attrib, providerName, ref val, false); config.Remove(attrib); } #endif private static void GetNonEmptyStringAttributeInternal(NameValueCollection config, string attrib, string providerName, ref string val, bool required) { string s = config.Get(attrib); // If it's (null and required) -OR- (empty string) we throw if ((s == null && required) || (s.Length == 0)) { throw new ConfigurationErrorsException( SR.GetString(SR.Provider_missing_attribute, attrib, providerName)); } val = s; } internal static void CheckUnrecognizedAttributes(NameValueCollection config, string providerName) { if (config.Count > 0) { string attribUnrecognized = config.GetKey(0); if (!String.IsNullOrEmpty(attribUnrecognized)) throw new ConfigurationErrorsException( SR.GetString(SR.Unexpected_provider_attribute, attribUnrecognized, providerName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Sec * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.Util { using System.Collections.Specialized; using System.Web.Mail; using System.Configuration; using System.Globalization; internal static class ProviderUtil { internal const int Infinite = Int32.MaxValue; internal static void GetAndRemoveStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { val = config.Get(attrib); config.Remove(attrib); } internal static void GetAndRemovePositiveAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { GetPositiveAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetPositiveAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { string s = config.Get(attrib); int t; if (s == null) { return; } try { t = Convert.ToInt32(s, CultureInfo.InvariantCulture); } catch (Exception e){ if (e is ArgumentException || e is FormatException || e is OverflowException) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } else { throw; } } if (t < 0) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } val = t; } internal static void GetAndRemovePositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { GetPositiveOrInfiniteAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetPositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { string s = config.Get(attrib); int t; if (s == null) { return; } if (s == "Infinite") { t = ProviderUtil.Infinite; } else { try { t = Convert.ToInt32(s, CultureInfo.InvariantCulture); } catch (Exception e){ if (e is ArgumentException || e is FormatException || e is OverflowException) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } else { throw; } } if (t < 0) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_positive_attributes, attrib, providerName)); } } val = t; } internal static void GetAndRemoveNonZeroPositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { GetNonZeroPositiveOrInfiniteAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetNonZeroPositiveOrInfiniteAttribute(NameValueCollection config, string attrib, string providerName, ref int val) { string s = config.Get(attrib); int t; if (s == null) { return; } if (s == "Infinite") { t = ProviderUtil.Infinite; } else { try { t = Convert.ToInt32(s, CultureInfo.InvariantCulture); } catch (Exception e){ if (e is ArgumentException || e is FormatException || e is OverflowException) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_non_zero_positive_attributes, attrib, providerName)); } else { throw; } } if (t <= 0) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_provider_non_zero_positive_attributes, attrib, providerName)); } } val = t; } internal static void GetAndRemoveBooleanAttribute(NameValueCollection config, string attrib, string providerName, ref bool val) { GetBooleanAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetBooleanAttribute(NameValueCollection config, string attrib, string providerName, ref bool val) { string s = config.Get(attrib); if (s == null) { return; } if (s == "true") { val = true; } else if (s == "false") { val = false; } else { throw new ConfigurationErrorsException(SR.GetString(SR.Invalid_provider_attribute, attrib, providerName, s)); } } internal static void GetAndRemoveRequiredNonEmptyStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { GetRequiredNonEmptyStringAttribute(config, attrib, providerName, ref val); config.Remove(attrib); } internal static void GetRequiredNonEmptyStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { GetNonEmptyStringAttributeInternal(config, attrib, providerName, ref val, true); } #if UNUSED_CODE internal static void GetAndRemoveNonEmptyStringAttribute(NameValueCollection config, string attrib, string providerName, ref string val) { GetNonEmptyStringAttributeInternal(config, attrib, providerName, ref val, false); config.Remove(attrib); } #endif private static void GetNonEmptyStringAttributeInternal(NameValueCollection config, string attrib, string providerName, ref string val, bool required) { string s = config.Get(attrib); // If it's (null and required) -OR- (empty string) we throw if ((s == null && required) || (s.Length == 0)) { throw new ConfigurationErrorsException( SR.GetString(SR.Provider_missing_attribute, attrib, providerName)); } val = s; } internal static void CheckUnrecognizedAttributes(NameValueCollection config, string providerName) { if (config.Count > 0) { string attribUnrecognized = config.GetKey(0); if (!String.IsNullOrEmpty(attribUnrecognized)) throw new ConfigurationErrorsException( SR.GetString(SR.Unexpected_provider_attribute, attribUnrecognized, providerName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
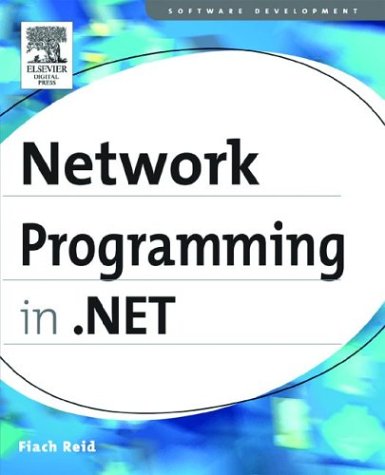
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AspNetSynchronizationContext.cs
- ArrayList.cs
- TimerEventSubscriptionCollection.cs
- SvcFileManager.cs
- CaseInsensitiveComparer.cs
- unsafeIndexingFilterStream.cs
- ProfilePropertyMetadata.cs
- CdpEqualityComparer.cs
- RotateTransform.cs
- WindowsAuthenticationEventArgs.cs
- DynamicDocumentPaginator.cs
- CompiledELinqQueryState.cs
- FormConverter.cs
- OdbcStatementHandle.cs
- Point3DCollection.cs
- StylusPointProperties.cs
- Latin1Encoding.cs
- SqlRemoveConstantOrderBy.cs
- IPipelineRuntime.cs
- ToolStripContentPanel.cs
- Msec.cs
- PieceNameHelper.cs
- Operand.cs
- BinaryWriter.cs
- DecimalStorage.cs
- ModelUIElement3D.cs
- Int16Converter.cs
- HttpCapabilitiesSectionHandler.cs
- DesignTimeVisibleAttribute.cs
- NativeMethods.cs
- CurrentChangingEventManager.cs
- Vector3DCollection.cs
- LinkLabel.cs
- Cursor.cs
- CounterCreationDataCollection.cs
- Int32Converter.cs
- FileRegion.cs
- ShaderEffect.cs
- RenderingEventArgs.cs
- ReverseQueryOperator.cs
- TextDecorations.cs
- UIElementParagraph.cs
- ApplicationSecurityInfo.cs
- SqlConnection.cs
- Animatable.cs
- LicFileLicenseProvider.cs
- ImportOptions.cs
- SR.cs
- FunctionMappingTranslator.cs
- WindowsTreeView.cs
- XomlCompilerError.cs
- EventProviderBase.cs
- ArrangedElement.cs
- ToolStripSeparatorRenderEventArgs.cs
- AsymmetricKeyExchangeFormatter.cs
- InputReferenceExpression.cs
- _FtpControlStream.cs
- InvalidProgramException.cs
- FontInfo.cs
- LifetimeServices.cs
- RowTypePropertyElement.cs
- DataViewListener.cs
- MetadataCache.cs
- LineSegment.cs
- XPathAncestorQuery.cs
- XPathNodeList.cs
- EmptyEnumerable.cs
- PropertyConverter.cs
- BamlMapTable.cs
- TargetFrameworkUtil.cs
- ToolStripControlHost.cs
- WorkflowPrinting.cs
- XMLDiffLoader.cs
- RC2.cs
- StaticExtension.cs
- Sorting.cs
- CodeExpressionStatement.cs
- GeneralTransform3DGroup.cs
- TickBar.cs
- PersonalizationProviderCollection.cs
- TextServicesCompartmentEventSink.cs
- Int16Converter.cs
- NativeCompoundFileAPIs.cs
- GlobalizationAssembly.cs
- MultilineStringConverter.cs
- CompositeFontFamily.cs
- ColorConverter.cs
- GridSplitter.cs
- WebDisplayNameAttribute.cs
- Events.cs
- AdRotatorDesigner.cs
- PackWebRequestFactory.cs
- NullableDoubleMinMaxAggregationOperator.cs
- EntityClientCacheEntry.cs
- CircleEase.cs
- ProgressChangedEventArgs.cs
- CodeGroup.cs
- PersistencePipeline.cs
- Binding.cs
- Helper.cs