Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / FontStretches.cs / 1305600 / FontStretches.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Predefined FontStretch structures that correspond to common font stretches. // // History: // 01/25/2005 mleonov - Created constants from FontStretch enum values. // //--------------------------------------------------------------------------- using System; using System.Globalization; namespace System.Windows { ////// FontStretches contains predefined font stretch structures for common font stretches. /// public static class FontStretches { ////// Predefined font stretch : Ultra-condensed. /// public static FontStretch UltraCondensed { get { return new FontStretch(1); } } ////// Predefined font stretch : Extra-condensed. /// public static FontStretch ExtraCondensed { get { return new FontStretch(2); } } ////// Predefined font stretch : Condensed. /// public static FontStretch Condensed { get { return new FontStretch(3); } } ////// Predefined font stretch : Semi-condensed. /// public static FontStretch SemiCondensed { get { return new FontStretch(4); } } ////// Predefined font stretch : Normal. /// public static FontStretch Normal { get { return new FontStretch(5); } } ////// Predefined font stretch : Medium. /// public static FontStretch Medium { get { return new FontStretch(5); } } ////// Predefined font stretch : Semi-expanded. /// public static FontStretch SemiExpanded { get { return new FontStretch(6); } } ////// Predefined font stretch : Expanded. /// public static FontStretch Expanded { get { return new FontStretch(7); } } ////// Predefined font stretch : Extra-expanded. /// public static FontStretch ExtraExpanded { get { return new FontStretch(8); } } ////// Predefined font stretch : Ultra-expanded. /// public static FontStretch UltraExpanded { get { return new FontStretch(9); } } internal static bool FontStretchStringToKnownStretch(string s, IFormatProvider provider, ref FontStretch fontStretch) { switch (s.Length) { case 6: if (s.Equals("Normal", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Normal; return true; } if (s.Equals("Medium", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Medium; return true; } break; case 8: if (s.Equals("Expanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Expanded; return true; } break; case 9: if (s.Equals("Condensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Condensed; return true; } break; case 12: if (s.Equals("SemiExpanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.SemiExpanded; return true; } break; case 13: if (s.Equals("SemiCondensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.SemiCondensed; return true; } if (s.Equals("ExtraExpanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.ExtraExpanded; return true; } if (s.Equals("UltraExpanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.UltraExpanded; return true; } break; case 14: if (s.Equals("UltraCondensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.UltraCondensed; return true; } if (s.Equals("ExtraCondensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.ExtraCondensed; return true; } break; } int stretchValue; if (int.TryParse(s, NumberStyles.Integer, provider, out stretchValue)) { fontStretch = FontStretch.FromOpenTypeStretch(stretchValue); return true; } return false; } internal static bool FontStretchToString(int stretch, out string convertedValue) { switch (stretch) { case 1: convertedValue = "UltraCondensed"; return true; case 2: convertedValue = "ExtraCondensed"; return true; case 3: convertedValue = "Condensed"; return true; case 4: convertedValue = "SemiCondensed"; return true; case 5: convertedValue = "Normal"; return true; case 6: convertedValue = "SemiExpanded"; return true; case 7: convertedValue = "Expanded"; return true; case 8: convertedValue = "ExtraExpanded"; return true; case 9: convertedValue = "UltraExpanded"; return true; } convertedValue = null; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Predefined FontStretch structures that correspond to common font stretches. // // History: // 01/25/2005 mleonov - Created constants from FontStretch enum values. // //--------------------------------------------------------------------------- using System; using System.Globalization; namespace System.Windows { ////// FontStretches contains predefined font stretch structures for common font stretches. /// public static class FontStretches { ////// Predefined font stretch : Ultra-condensed. /// public static FontStretch UltraCondensed { get { return new FontStretch(1); } } ////// Predefined font stretch : Extra-condensed. /// public static FontStretch ExtraCondensed { get { return new FontStretch(2); } } ////// Predefined font stretch : Condensed. /// public static FontStretch Condensed { get { return new FontStretch(3); } } ////// Predefined font stretch : Semi-condensed. /// public static FontStretch SemiCondensed { get { return new FontStretch(4); } } ////// Predefined font stretch : Normal. /// public static FontStretch Normal { get { return new FontStretch(5); } } ////// Predefined font stretch : Medium. /// public static FontStretch Medium { get { return new FontStretch(5); } } ////// Predefined font stretch : Semi-expanded. /// public static FontStretch SemiExpanded { get { return new FontStretch(6); } } ////// Predefined font stretch : Expanded. /// public static FontStretch Expanded { get { return new FontStretch(7); } } ////// Predefined font stretch : Extra-expanded. /// public static FontStretch ExtraExpanded { get { return new FontStretch(8); } } ////// Predefined font stretch : Ultra-expanded. /// public static FontStretch UltraExpanded { get { return new FontStretch(9); } } internal static bool FontStretchStringToKnownStretch(string s, IFormatProvider provider, ref FontStretch fontStretch) { switch (s.Length) { case 6: if (s.Equals("Normal", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Normal; return true; } if (s.Equals("Medium", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Medium; return true; } break; case 8: if (s.Equals("Expanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Expanded; return true; } break; case 9: if (s.Equals("Condensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.Condensed; return true; } break; case 12: if (s.Equals("SemiExpanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.SemiExpanded; return true; } break; case 13: if (s.Equals("SemiCondensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.SemiCondensed; return true; } if (s.Equals("ExtraExpanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.ExtraExpanded; return true; } if (s.Equals("UltraExpanded", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.UltraExpanded; return true; } break; case 14: if (s.Equals("UltraCondensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.UltraCondensed; return true; } if (s.Equals("ExtraCondensed", StringComparison.OrdinalIgnoreCase)) { fontStretch = FontStretches.ExtraCondensed; return true; } break; } int stretchValue; if (int.TryParse(s, NumberStyles.Integer, provider, out stretchValue)) { fontStretch = FontStretch.FromOpenTypeStretch(stretchValue); return true; } return false; } internal static bool FontStretchToString(int stretch, out string convertedValue) { switch (stretch) { case 1: convertedValue = "UltraCondensed"; return true; case 2: convertedValue = "ExtraCondensed"; return true; case 3: convertedValue = "Condensed"; return true; case 4: convertedValue = "SemiCondensed"; return true; case 5: convertedValue = "Normal"; return true; case 6: convertedValue = "SemiExpanded"; return true; case 7: convertedValue = "Expanded"; return true; case 8: convertedValue = "ExtraExpanded"; return true; case 9: convertedValue = "UltraExpanded"; return true; } convertedValue = null; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
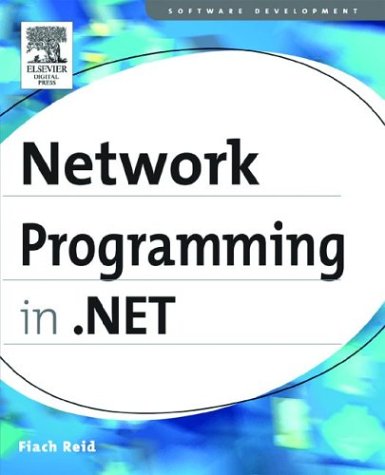
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MD5CryptoServiceProvider.cs
- FrameDimension.cs
- DataColumnChangeEvent.cs
- WebSysDisplayNameAttribute.cs
- PagePropertiesChangingEventArgs.cs
- StateMachineExecutionState.cs
- ReadOnlyPropertyMetadata.cs
- NumberSubstitution.cs
- ProgressBarRenderer.cs
- BindingGroup.cs
- RegexMatch.cs
- EmptyStringExpandableObjectConverter.cs
- StorageRoot.cs
- ConsoleTraceListener.cs
- Int32EqualityComparer.cs
- TextBreakpoint.cs
- StateMachineDesignerPaint.cs
- WFItemsToSpacerVisibility.cs
- SimpleLine.cs
- CounterCreationData.cs
- DbDataSourceEnumerator.cs
- DataGridViewCellValueEventArgs.cs
- ListDictionaryInternal.cs
- AssemblyCache.cs
- DelegatingChannelListener.cs
- GenericRootAutomationPeer.cs
- HealthMonitoringSection.cs
- ObjectManager.cs
- altserialization.cs
- TextSimpleMarkerProperties.cs
- SslStreamSecurityElement.cs
- ILGenerator.cs
- EqualityComparer.cs
- DesignerPainter.cs
- GridViewItemAutomationPeer.cs
- QilNode.cs
- MenuItem.cs
- Color.cs
- AuthenticodeSignatureInformation.cs
- EarlyBoundInfo.cs
- CounterCreationData.cs
- _AutoWebProxyScriptWrapper.cs
- SaveFileDialog.cs
- ChangeToolStripParentVerb.cs
- FastEncoderWindow.cs
- Partitioner.cs
- ComNativeDescriptor.cs
- DecimalConstantAttribute.cs
- InternalUserCancelledException.cs
- WinCategoryAttribute.cs
- ExtenderProvidedPropertyAttribute.cs
- WebPartUtil.cs
- PositiveTimeSpanValidatorAttribute.cs
- ProtocolsInstallComponent.cs
- Schema.cs
- FacetValueContainer.cs
- ClientCultureInfo.cs
- CacheAxisQuery.cs
- SystemWebCachingSectionGroup.cs
- DetailsViewPagerRow.cs
- FrameworkRichTextComposition.cs
- SingleSelectRootGridEntry.cs
- DoubleKeyFrameCollection.cs
- SevenBitStream.cs
- ByteAnimationBase.cs
- NotFiniteNumberException.cs
- SimpleType.cs
- StringFunctions.cs
- ConditionedDesigner.cs
- Vector3DAnimation.cs
- BamlTreeMap.cs
- Encoder.cs
- XmlSchemaNotation.cs
- IncomingWebRequestContext.cs
- ReadOnlyDataSourceView.cs
- Int32AnimationUsingKeyFrames.cs
- MissingMethodException.cs
- SqlRecordBuffer.cs
- ServiceMetadataExtension.cs
- Range.cs
- DataGridTablesFactory.cs
- TemplatePagerField.cs
- Bezier.cs
- EntityDataSourceChangedEventArgs.cs
- XmlSchemaProviderAttribute.cs
- NotImplementedException.cs
- VariableQuery.cs
- CommandBindingCollection.cs
- DependentList.cs
- Point3DCollection.cs
- HtmlDocument.cs
- ItemDragEvent.cs
- RelationshipConverter.cs
- DataSpaceManager.cs
- TableRow.cs
- LoginView.cs
- XmlIncludeAttribute.cs
- ScriptRegistrationManager.cs
- RichTextBox.cs
- StrongNameIdentityPermission.cs