Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / X509Certificates / AuthenticodeSignatureInformation.cs / 1305376 / AuthenticodeSignatureInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the Authenticode signature of a manifest /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class AuthenticodeSignatureInformation { private string m_description; private Uri m_descriptionUrl; private CapiNative.AlgorithmId m_hashAlgorithmId; private X509Chain m_signatureChain; private TimestampInformation m_timestamp; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_signingCertificate; internal AuthenticodeSignatureInformation(X509Native.AXL_AUTHENTICODE_SIGNER_INFO signer, X509Chain signatureChain, TimestampInformation timestamp) { m_verificationResult = (SignatureVerificationResult)signer.dwError; m_hashAlgorithmId = signer.algHash; if (signer.pwszDescription != IntPtr.Zero) { m_description = Marshal.PtrToStringUni(signer.pwszDescription); } if (signer.pwszDescriptionUrl != IntPtr.Zero) { string descriptionUrl = Marshal.PtrToStringUni(signer.pwszDescriptionUrl); Uri.TryCreate(descriptionUrl, UriKind.RelativeOrAbsolute, out m_descriptionUrl); } m_signatureChain = signatureChain; // If there was a timestamp, and it was not valid we need to invalidate the entire Authenticode // signature as well, since we cannot assume that the signature would have verified without // the timestamp. if (timestamp != null && timestamp.VerificationResult != SignatureVerificationResult.MissingSignature) { if (timestamp.IsValid) { m_timestamp = timestamp; } else { m_verificationResult = SignatureVerificationResult.InvalidTimestamp; } } else { m_timestamp = null; } } ////// Create an Authenticode signature information for a signature which is not valid /// internal AuthenticodeSignatureInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid); m_verificationResult = error; } ////// Description of the signing certificate /// public string Description { get { return m_description; } } ////// Description URL of the signing certificate /// public Uri DescriptionUrl { get { return m_descriptionUrl; } } ////// Hash algorithm the signature was computed with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the signature /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// X509 chain used to verify the Authenticode signature /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_signatureChain; } } ////// Certificate the manifest was signed with /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_signingCertificate == null && SignatureChain != null) { Debug.Assert(SignatureChain.ChainElements.Count > 0, "SignatureChain.ChainElements.Count > 0"); m_signingCertificate = SignatureChain.ChainElements[0].Certificate; } return m_signingCertificate; } } ////// Timestamp, if any, applied to the Authenticode signature /// ////// Note that this is only available in the trusted publisher case /// public TimestampInformation Timestamp { get { return m_timestamp; } } ////// Trustworthiness of the Authenticode signature /// public TrustStatus TrustStatus { get { switch (VerificationResult) { case SignatureVerificationResult.Valid: return TrustStatus.Trusted; case SignatureVerificationResult.CertificateNotExplicitlyTrusted: return TrustStatus.KnownIdentity; case SignatureVerificationResult.CertificateExplicitlyDistrusted: return TrustStatus.Untrusted; default: return TrustStatus.UnknownIdentity; } } } ////// Result of verifying the Authenticode signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the Authenticode signature of a manifest /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class AuthenticodeSignatureInformation { private string m_description; private Uri m_descriptionUrl; private CapiNative.AlgorithmId m_hashAlgorithmId; private X509Chain m_signatureChain; private TimestampInformation m_timestamp; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_signingCertificate; internal AuthenticodeSignatureInformation(X509Native.AXL_AUTHENTICODE_SIGNER_INFO signer, X509Chain signatureChain, TimestampInformation timestamp) { m_verificationResult = (SignatureVerificationResult)signer.dwError; m_hashAlgorithmId = signer.algHash; if (signer.pwszDescription != IntPtr.Zero) { m_description = Marshal.PtrToStringUni(signer.pwszDescription); } if (signer.pwszDescriptionUrl != IntPtr.Zero) { string descriptionUrl = Marshal.PtrToStringUni(signer.pwszDescriptionUrl); Uri.TryCreate(descriptionUrl, UriKind.RelativeOrAbsolute, out m_descriptionUrl); } m_signatureChain = signatureChain; // If there was a timestamp, and it was not valid we need to invalidate the entire Authenticode // signature as well, since we cannot assume that the signature would have verified without // the timestamp. if (timestamp != null && timestamp.VerificationResult != SignatureVerificationResult.MissingSignature) { if (timestamp.IsValid) { m_timestamp = timestamp; } else { m_verificationResult = SignatureVerificationResult.InvalidTimestamp; } } else { m_timestamp = null; } } ////// Create an Authenticode signature information for a signature which is not valid /// internal AuthenticodeSignatureInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid); m_verificationResult = error; } ////// Description of the signing certificate /// public string Description { get { return m_description; } } ////// Description URL of the signing certificate /// public Uri DescriptionUrl { get { return m_descriptionUrl; } } ////// Hash algorithm the signature was computed with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the signature /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// X509 chain used to verify the Authenticode signature /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_signatureChain; } } ////// Certificate the manifest was signed with /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_signingCertificate == null && SignatureChain != null) { Debug.Assert(SignatureChain.ChainElements.Count > 0, "SignatureChain.ChainElements.Count > 0"); m_signingCertificate = SignatureChain.ChainElements[0].Certificate; } return m_signingCertificate; } } ////// Timestamp, if any, applied to the Authenticode signature /// ////// Note that this is only available in the trusted publisher case /// public TimestampInformation Timestamp { get { return m_timestamp; } } ////// Trustworthiness of the Authenticode signature /// public TrustStatus TrustStatus { get { switch (VerificationResult) { case SignatureVerificationResult.Valid: return TrustStatus.Trusted; case SignatureVerificationResult.CertificateNotExplicitlyTrusted: return TrustStatus.KnownIdentity; case SignatureVerificationResult.CertificateExplicitlyDistrusted: return TrustStatus.Untrusted; default: return TrustStatus.UnknownIdentity; } } } ////// Result of verifying the Authenticode signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
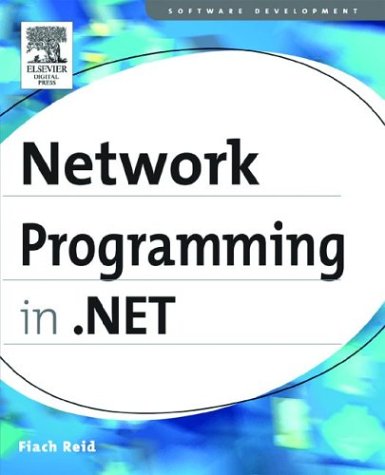
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfilePropertySettingsCollection.cs
- WindowsIPAddress.cs
- EncryptedData.cs
- Transform.cs
- DesignerTextWriter.cs
- OleStrCAMarshaler.cs
- TraceHandlerErrorFormatter.cs
- shaperfactoryquerycachekey.cs
- RSAOAEPKeyExchangeFormatter.cs
- ResourceDefaultValueAttribute.cs
- RegularExpressionValidator.cs
- UrlPropertyAttribute.cs
- SessionEndingEventArgs.cs
- SqlDependencyListener.cs
- ResourceReferenceExpression.cs
- TimeSpan.cs
- Overlapped.cs
- SafeHandle.cs
- Queue.cs
- TemplatedEditableDesignerRegion.cs
- IPAddress.cs
- DataGridBoolColumn.cs
- BlobPersonalizationState.cs
- Overlapped.cs
- WrappedIUnknown.cs
- FunctionDetailsReader.cs
- ControlAdapter.cs
- CompatibleComparer.cs
- MdiWindowListItemConverter.cs
- WebScriptServiceHost.cs
- SafeThreadHandle.cs
- MiniCustomAttributeInfo.cs
- PathFigureCollection.cs
- ImageSource.cs
- RoutedEventHandlerInfo.cs
- ViewgenContext.cs
- ConstantSlot.cs
- Decoder.cs
- ContextInformation.cs
- ProcessInfo.cs
- DeflateStream.cs
- DataGridViewCheckBoxColumn.cs
- CaseInsensitiveHashCodeProvider.cs
- DataServiceResponse.cs
- HttpChannelBindingToken.cs
- ChangeNode.cs
- InternalBufferOverflowException.cs
- BehaviorEditorPart.cs
- TextShapeableCharacters.cs
- DummyDataSource.cs
- WebPartCatalogCloseVerb.cs
- MenuEventArgs.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- CqlGenerator.cs
- HostedElements.cs
- HandlerFactoryCache.cs
- EncodingStreamWrapper.cs
- EmulateRecognizeCompletedEventArgs.cs
- DataViewManager.cs
- DataContractSerializer.cs
- ChildTable.cs
- ToolBar.cs
- ListenerAdapterBase.cs
- RedirectionProxy.cs
- XmlILOptimizerVisitor.cs
- ChangeBlockUndoRecord.cs
- ControlCollection.cs
- WindowsBrush.cs
- DrawToolTipEventArgs.cs
- EntityDataSourceDesignerHelper.cs
- SynchronizationLockException.cs
- WebScriptMetadataMessageEncoderFactory.cs
- Mappings.cs
- XamlRtfConverter.cs
- StickyNoteAnnotations.cs
- WebPartCollection.cs
- DataGridAutomationPeer.cs
- KnowledgeBase.cs
- RegexWriter.cs
- StaticContext.cs
- AutomationPropertyInfo.cs
- CreateUserWizardDesigner.cs
- RepeaterCommandEventArgs.cs
- StringComparer.cs
- UserControlAutomationPeer.cs
- Empty.cs
- ControlCommandSet.cs
- NullableIntAverageAggregationOperator.cs
- UriParserTemplates.cs
- AspNetSynchronizationContext.cs
- FreeFormDesigner.cs
- DomainUpDown.cs
- SqlParameterCollection.cs
- XmlBinaryReader.cs
- RadioButton.cs
- Vector3DAnimation.cs
- Size.cs
- Atom10FormatterFactory.cs
- _ListenerResponseStream.cs
- DbDataRecord.cs