Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / infocard / Client / System / IdentityModel / Selectors / PolicyChain.cs / 1305376 / PolicyChain.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; using System.Xml; // // For common // using Microsoft.InfoCards; // // Summary: // This class wraps and manages the lifetime of an array of PolicyElements that are to be Marshaled to // native memory. // internal class PolicyChain : IDisposable { HGlobalSafeHandle m_nativeChain; InternalPolicyElement[] m_chain; public int Length { get { return m_chain.Length; } } public PolicyChain( CardSpacePolicyElement[ ] elements ) { int length = elements.Length; m_chain = new InternalPolicyElement[ length ]; for( int i = 0; i < length; i++ ) { m_chain[ i ] = new InternalPolicyElement( elements[ i ] ); } } public SafeHandle DoMarshal() { if( null == m_nativeChain ) { int elementSize = InternalPolicyElement.Size; int chainLength = m_chain.Length; m_nativeChain = HGlobalSafeHandle.Construct( chainLength * elementSize ); IntPtr pos = m_nativeChain.DangerousGetHandle(); foreach( InternalPolicyElement element in m_chain ) { element.DoMarshal( pos ); unsafe { // // All this just to do pos += elementSize // pos = new IntPtr( (long)( ( (ulong) pos.ToPointer() ) + (ulong) elementSize ) ); } } } return m_nativeChain; } public void Dispose() { Dispose( true ); } ~PolicyChain() { Dispose( false ); } private void Dispose( bool disposing ) { if( disposing ) { GC.SuppressFinalize( this ); } if( null != m_chain ) { foreach( InternalPolicyElement element in m_chain ) { if( null != element ) { element.Dispose(); } } m_chain = null; } if( null != m_nativeChain ) { m_nativeChain.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; using System.Xml; // // For common // using Microsoft.InfoCards; // // Summary: // This class wraps and manages the lifetime of an array of PolicyElements that are to be Marshaled to // native memory. // internal class PolicyChain : IDisposable { HGlobalSafeHandle m_nativeChain; InternalPolicyElement[] m_chain; public int Length { get { return m_chain.Length; } } public PolicyChain( CardSpacePolicyElement[ ] elements ) { int length = elements.Length; m_chain = new InternalPolicyElement[ length ]; for( int i = 0; i < length; i++ ) { m_chain[ i ] = new InternalPolicyElement( elements[ i ] ); } } public SafeHandle DoMarshal() { if( null == m_nativeChain ) { int elementSize = InternalPolicyElement.Size; int chainLength = m_chain.Length; m_nativeChain = HGlobalSafeHandle.Construct( chainLength * elementSize ); IntPtr pos = m_nativeChain.DangerousGetHandle(); foreach( InternalPolicyElement element in m_chain ) { element.DoMarshal( pos ); unsafe { // // All this just to do pos += elementSize // pos = new IntPtr( (long)( ( (ulong) pos.ToPointer() ) + (ulong) elementSize ) ); } } } return m_nativeChain; } public void Dispose() { Dispose( true ); } ~PolicyChain() { Dispose( false ); } private void Dispose( bool disposing ) { if( disposing ) { GC.SuppressFinalize( this ); } if( null != m_chain ) { foreach( InternalPolicyElement element in m_chain ) { if( null != element ) { element.Dispose(); } } m_chain = null; } if( null != m_nativeChain ) { m_nativeChain.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
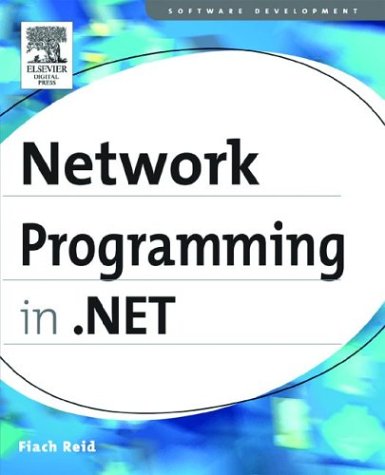
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KeyValueConfigurationCollection.cs
- DataBindingExpressionBuilder.cs
- WebExceptionStatus.cs
- DBDataPermissionAttribute.cs
- Soap.cs
- AutomationPeer.cs
- NegationPusher.cs
- IpcChannelHelper.cs
- ValidatingReaderNodeData.cs
- MetabaseServerConfig.cs
- PersistenceMetadataNamespace.cs
- DrawingContextWalker.cs
- HandleRef.cs
- Selection.cs
- NullRuntimeConfig.cs
- Models.cs
- OperationAbortedException.cs
- CuspData.cs
- SqlLiftWhereClauses.cs
- LineGeometry.cs
- HttpProfileBase.cs
- HtmlMeta.cs
- EventRoute.cs
- TreeNodeCollectionEditor.cs
- TreeNodeCollection.cs
- RowBinding.cs
- RuntimeConfig.cs
- CardSpaceException.cs
- Literal.cs
- CodeMethodInvokeExpression.cs
- DetailsViewAutoFormat.cs
- _NegotiateClient.cs
- UnsafeNativeMethodsMilCoreApi.cs
- DecoderReplacementFallback.cs
- ColumnHeaderConverter.cs
- Message.cs
- ChannelBuilder.cs
- XmlIlVisitor.cs
- BaseParaClient.cs
- SoapAttributeAttribute.cs
- DuplicateWaitObjectException.cs
- TextBox.cs
- ReadOnlyDictionary.cs
- ToolStripItemEventArgs.cs
- BitmapDecoder.cs
- Splitter.cs
- DataSourceControl.cs
- ProtectedProviderSettings.cs
- MetadataPropertyCollection.cs
- WebPartTracker.cs
- GlyphCollection.cs
- DataGridViewCellStyleConverter.cs
- HttpCapabilitiesEvaluator.cs
- CodeMemberProperty.cs
- MsmqBindingMonitor.cs
- WindowsSolidBrush.cs
- ControlValuePropertyAttribute.cs
- LinkArea.cs
- ResourceCategoryAttribute.cs
- SiteMembershipCondition.cs
- CancellableEnumerable.cs
- Int32Rect.cs
- CssTextWriter.cs
- Transform3D.cs
- DesignConnectionCollection.cs
- DocobjHost.cs
- PagedDataSource.cs
- ExecutionContext.cs
- InsufficientMemoryException.cs
- SchemaMerger.cs
- ButtonStandardAdapter.cs
- Drawing.cs
- ApplicationContext.cs
- TextRenderer.cs
- NavigationPropertyEmitter.cs
- IfJoinedCondition.cs
- AnonymousIdentificationSection.cs
- DigestTraceRecordHelper.cs
- SchemaCollectionPreprocessor.cs
- OleDbConnectionInternal.cs
- TemplateParser.cs
- TextBoxAutomationPeer.cs
- DataTableReaderListener.cs
- RuleSetDialog.Designer.cs
- SetIterators.cs
- UserControlBuildProvider.cs
- SrgsText.cs
- DummyDataSource.cs
- CollectionMarkupSerializer.cs
- SerializationIncompleteException.cs
- TypedDatasetGenerator.cs
- BookmarkNameHelper.cs
- MorphHelpers.cs
- HtmlSelect.cs
- DebugController.cs
- Invariant.cs
- SectionVisual.cs
- TextParentUndoUnit.cs
- IPAddressCollection.cs
- TextRangeEdit.cs