Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / NumericUpDownAccelerationCollection.cs / 1 / NumericUpDownAccelerationCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using System.ComponentModel; ////// Represents a SORTED collection of NumericUpDownAcceleration objects in the NumericUpDown Control. /// The elements in the collection are sorted by the NumericUpDownAcceleration.Seconds property. /// [ListBindable(false)] public class NumericUpDownAccelerationCollection : MarshalByRefObject, ICollection, IEnumerable { List items; /// ICollection implementation. /// /// Adds an item (NumericUpDownAcceleration object) to the ICollection. /// The item is added preserving the collection sorted. /// public void Add(NumericUpDownAcceleration acceleration) { if( acceleration == null ) { throw new ArgumentNullException("acceleration"); } // Keep the array sorted, insert in the right spot. int index = 0; while( index < this.items.Count ) { if( acceleration.Seconds < this.items[index].Seconds ) { break; } index++; } this.items.Insert(index, acceleration); } ////// Removes all items from the ICollection. /// public void Clear() { this.items.Clear(); } ////// Determines whether the IList contains a specific value. /// public bool Contains(NumericUpDownAcceleration acceleration) { return this.items.Contains(acceleration); } ////// Copies the elements of the ICollection to an Array, starting at a particular Array index. /// public void CopyTo(NumericUpDownAcceleration[] array, int index) { this.items.CopyTo(array, index); } ////// Gets the number of elements contained in the ICollection. /// public int Count { get {return this.items.Count;} } ////// Gets a value indicating whether the ICollection is read-only. /// This collection property returns false always. /// public bool IsReadOnly { get {return false;} } ////// Removes the specified item from the ICollection. /// public bool Remove(NumericUpDownAcceleration acceleration) { return this.items.Remove(acceleration); } /// IEnumerableimplementation. /// /// Returns an enumerator that can iterate through the collection. /// IEnumeratorIEnumerable .GetEnumerator() { return this.items.GetEnumerator(); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable)items).GetEnumerator(); } /// NumericUpDownAccelerationCollection methods. /// /// Class constructor. /// public NumericUpDownAccelerationCollection() { this.items = new List(); } /// /// Adds the elements of specified array to the collection, keeping the collection sorted. /// public void AddRange(params NumericUpDownAcceleration[] accelerations) { if (accelerations == null) { throw new ArgumentNullException("accelerations"); } // Accept the range only if ALL elements in the array are not null. foreach (NumericUpDownAcceleration acceleration in accelerations) { if (acceleration == null) { throw new ArgumentNullException(SR.GetString(SR.NumericUpDownAccelerationCollectionAtLeastOneEntryIsNull)); } } // The expected array size is typically small (5 items?), so we don't need to try to be smarter about the // way we add the elements to the collection, just call Add. foreach (NumericUpDownAcceleration acceleration in accelerations) { this.Add(acceleration); } } ////// Gets (ReadOnly) the element at the specified index. In C#, this property is the indexer for /// the IList class. /// public NumericUpDownAcceleration this[int index] { get { return this.items[index]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
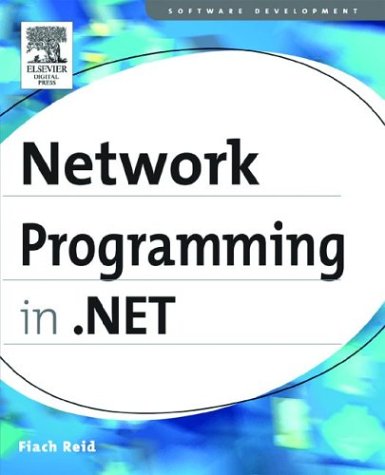
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Image.cs
- XmlAttributeAttribute.cs
- EnterpriseServicesHelper.cs
- TextEditorCopyPaste.cs
- CustomPopupPlacement.cs
- EncryptedKeyIdentifierClause.cs
- KeyboardEventArgs.cs
- MetadataPropertyAttribute.cs
- ToolStripArrowRenderEventArgs.cs
- TrailingSpaceComparer.cs
- FontCacheUtil.cs
- WSSecureConversation.cs
- PagedDataSource.cs
- Hashtable.cs
- QilLoop.cs
- UIElement3D.cs
- IncrementalReadDecoders.cs
- Rule.cs
- ObjectDataSourceChooseTypePanel.cs
- WebAdminConfigurationHelper.cs
- BamlMapTable.cs
- Queue.cs
- InputScopeNameConverter.cs
- IteratorFilter.cs
- CommunicationObjectAbortedException.cs
- EnumType.cs
- ImageSource.cs
- ToolStripItemCollection.cs
- FieldBuilder.cs
- NameValueSectionHandler.cs
- ProfileGroupSettings.cs
- XmlBinaryReaderSession.cs
- StringBlob.cs
- PasswordTextContainer.cs
- ApplicationServicesHostFactory.cs
- Encoder.cs
- DeferredTextReference.cs
- TraceUtility.cs
- WebPartDisplayModeEventArgs.cs
- XPathSelfQuery.cs
- InputLanguageSource.cs
- TaskHelper.cs
- BuildResult.cs
- XsltQilFactory.cs
- FileIOPermission.cs
- MultiBinding.cs
- FilteredReadOnlyMetadataCollection.cs
- categoryentry.cs
- RenderCapability.cs
- WebPartConnectionsCancelEventArgs.cs
- StreamAsIStream.cs
- RadioButton.cs
- Image.cs
- InstallerTypeAttribute.cs
- SafeFileHandle.cs
- Expression.cs
- DateBoldEvent.cs
- objectresult_tresulttype.cs
- DrawingContextWalker.cs
- WebPartZone.cs
- KeyBinding.cs
- SymmetricKey.cs
- VerificationAttribute.cs
- Axis.cs
- AutomationIdentifier.cs
- Image.cs
- DefaultAssemblyResolver.cs
- TextServicesContext.cs
- IpcServerChannel.cs
- SQLByte.cs
- FixedSOMImage.cs
- XmlTypeAttribute.cs
- XmlJsonWriter.cs
- XamlVector3DCollectionSerializer.cs
- PersonalizationDictionary.cs
- SessionPageStateSection.cs
- AssemblyInfo.cs
- QilFunction.cs
- SQLInt32.cs
- TextDecoration.cs
- XmlSiteMapProvider.cs
- XmlMembersMapping.cs
- StaticSiteMapProvider.cs
- ModelItemKeyValuePair.cs
- RuntimeHelpers.cs
- TemplateFactory.cs
- ExtensionFile.cs
- QilParameter.cs
- SafeFileHandle.cs
- Rfc2898DeriveBytes.cs
- ToolStripRenderEventArgs.cs
- DesignerAttributeInfo.cs
- CopyNodeSetAction.cs
- RuleDefinitions.cs
- HttpValueCollection.cs
- DocumentViewerBase.cs
- RequestCacheEntry.cs
- BaseParaClient.cs
- EdmSchemaError.cs
- WindowsPrincipal.cs