Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripRenderEventArgs.cs / 1 / ToolStripRenderEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Drawing; ////// /// ToolStripRenderEventArgs /// public class ToolStripRenderEventArgs : EventArgs { private ToolStrip toolStrip = null; private Graphics graphics = null; private Rectangle affectedBounds = Rectangle.Empty; private Color backColor = Color.Empty; ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip) { this.toolStrip = toolStrip; this.graphics = g; this.affectedBounds = new Rectangle(Point.Empty, toolStrip.Size); } ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip, Rectangle affectedBounds, Color backColor) { this.toolStrip = toolStrip; this.affectedBounds = affectedBounds; this.graphics = g; this.backColor = backColor; } ////// /// the bounds to draw in /// public Rectangle AffectedBounds { get { return affectedBounds; } } ////// /// the back color to draw with. /// public Color BackColor { get { if (backColor == Color.Empty) { // get the user specified color backColor = toolStrip.RawBackColor; if (backColor == Color.Empty) { if (toolStrip is ToolStripDropDown) { backColor = SystemColors.Menu; } else if (toolStrip is MenuStrip) { backColor = SystemColors.MenuBar; } else { backColor = SystemColors.Control; } } } return backColor; } } ////// /// the graphics object to draw with /// public Graphics Graphics { get { return graphics; } } ////// /// Represents which toolStrip was affected by the click /// public ToolStrip ToolStrip { get { return toolStrip; } } ///public Rectangle ConnectedArea { get { ToolStripDropDown dropDown = toolStrip as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem ownerItem = dropDown.OwnerItem as ToolStripDropDownItem; if (ownerItem is MdiControlStrip.SystemMenuItem) { // there's no connected rect between a system menu item and a dropdown. return Rectangle.Empty; } if (ownerItem !=null && ownerItem.ParentInternal != null && !ownerItem.IsOnDropDown) { // translate the item into our coordinate system. Rectangle itemBounds = new Rectangle(toolStrip.PointToClient(ownerItem.TranslatePoint(Point.Empty, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords)), ownerItem.Size); Rectangle bounds = ToolStrip.Bounds; Rectangle overlap = ToolStrip.ClientRectangle; overlap.Inflate(1,1); if (overlap.IntersectsWith(itemBounds)) { switch (ownerItem.DropDownDirection) { case ToolStripDropDownDirection.AboveLeft: case ToolStripDropDownDirection.AboveRight: // Consider... the shadow effect interferes with the connected area. return Rectangle.Empty; // return new Rectangle(itemBounds.X+1, bounds.Height -2, itemBounds.Width -2, 2); case ToolStripDropDownDirection.BelowRight: case ToolStripDropDownDirection.BelowLeft: overlap.Intersect(itemBounds); if (overlap.Height == 2) { return new Rectangle(itemBounds.X+1, 0, itemBounds.Width -2, 2); } // if its overlapping more than one pixel, this means we've pushed it to obscure // the menu item. in this case pretend it's not connected. return Rectangle.Empty; case ToolStripDropDownDirection.Right: case ToolStripDropDownDirection.Left: return Rectangle.Empty; // Consider... the shadow effect interferes with the connected area. // return new Rectangle(bounds.Width-2, 1, 2, itemBounds.Height-2); } } } } return Rectangle.Empty; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Drawing; ////// /// ToolStripRenderEventArgs /// public class ToolStripRenderEventArgs : EventArgs { private ToolStrip toolStrip = null; private Graphics graphics = null; private Rectangle affectedBounds = Rectangle.Empty; private Color backColor = Color.Empty; ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip) { this.toolStrip = toolStrip; this.graphics = g; this.affectedBounds = new Rectangle(Point.Empty, toolStrip.Size); } ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip, Rectangle affectedBounds, Color backColor) { this.toolStrip = toolStrip; this.affectedBounds = affectedBounds; this.graphics = g; this.backColor = backColor; } ////// /// the bounds to draw in /// public Rectangle AffectedBounds { get { return affectedBounds; } } ////// /// the back color to draw with. /// public Color BackColor { get { if (backColor == Color.Empty) { // get the user specified color backColor = toolStrip.RawBackColor; if (backColor == Color.Empty) { if (toolStrip is ToolStripDropDown) { backColor = SystemColors.Menu; } else if (toolStrip is MenuStrip) { backColor = SystemColors.MenuBar; } else { backColor = SystemColors.Control; } } } return backColor; } } ////// /// the graphics object to draw with /// public Graphics Graphics { get { return graphics; } } ////// /// Represents which toolStrip was affected by the click /// public ToolStrip ToolStrip { get { return toolStrip; } } ///public Rectangle ConnectedArea { get { ToolStripDropDown dropDown = toolStrip as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem ownerItem = dropDown.OwnerItem as ToolStripDropDownItem; if (ownerItem is MdiControlStrip.SystemMenuItem) { // there's no connected rect between a system menu item and a dropdown. return Rectangle.Empty; } if (ownerItem !=null && ownerItem.ParentInternal != null && !ownerItem.IsOnDropDown) { // translate the item into our coordinate system. Rectangle itemBounds = new Rectangle(toolStrip.PointToClient(ownerItem.TranslatePoint(Point.Empty, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords)), ownerItem.Size); Rectangle bounds = ToolStrip.Bounds; Rectangle overlap = ToolStrip.ClientRectangle; overlap.Inflate(1,1); if (overlap.IntersectsWith(itemBounds)) { switch (ownerItem.DropDownDirection) { case ToolStripDropDownDirection.AboveLeft: case ToolStripDropDownDirection.AboveRight: // Consider... the shadow effect interferes with the connected area. return Rectangle.Empty; // return new Rectangle(itemBounds.X+1, bounds.Height -2, itemBounds.Width -2, 2); case ToolStripDropDownDirection.BelowRight: case ToolStripDropDownDirection.BelowLeft: overlap.Intersect(itemBounds); if (overlap.Height == 2) { return new Rectangle(itemBounds.X+1, 0, itemBounds.Width -2, 2); } // if its overlapping more than one pixel, this means we've pushed it to obscure // the menu item. in this case pretend it's not connected. return Rectangle.Empty; case ToolStripDropDownDirection.Right: case ToolStripDropDownDirection.Left: return Rectangle.Empty; // Consider... the shadow effect interferes with the connected area. // return new Rectangle(bounds.Width-2, 1, 2, itemBounds.Height-2); } } } } return Rectangle.Empty; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
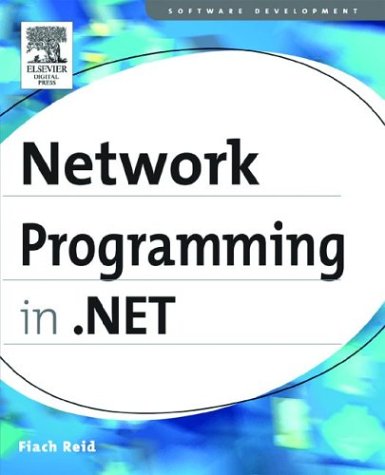
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NewItemsContextMenuStrip.cs
- RuntimeHandles.cs
- VSDExceptions.cs
- SQLMoneyStorage.cs
- MarshalByRefObject.cs
- OleDbStruct.cs
- Ray3DHitTestResult.cs
- DataGridViewTextBoxCell.cs
- PolygonHotSpot.cs
- ApplicationActivator.cs
- QilLiteral.cs
- SqlNodeAnnotations.cs
- EventManager.cs
- NullableDecimalMinMaxAggregationOperator.cs
- NetNamedPipeSecurityElement.cs
- WebBrowserUriTypeConverter.cs
- XmlWrappingWriter.cs
- ParseNumbers.cs
- PrintPreviewGraphics.cs
- ExeConfigurationFileMap.cs
- BooleanConverter.cs
- GiveFeedbackEvent.cs
- AccessText.cs
- TypeLibConverter.cs
- CloudCollection.cs
- XsltLoader.cs
- DataBindingCollectionConverter.cs
- EncryptedXml.cs
- ObjectDataSourceDesigner.cs
- AbandonedMutexException.cs
- WSDualHttpSecurityMode.cs
- PathFigureCollection.cs
- Brush.cs
- Enlistment.cs
- MobileControl.cs
- WeakReferenceKey.cs
- Win32.cs
- CacheDependency.cs
- WizardStepBase.cs
- wgx_commands.cs
- PageStatePersister.cs
- IResourceProvider.cs
- _ConnectOverlappedAsyncResult.cs
- XmlWrappingReader.cs
- EtwTrace.cs
- Assign.cs
- Size.cs
- ToolStripArrowRenderEventArgs.cs
- TailCallAnalyzer.cs
- RenderCapability.cs
- MenuItemBinding.cs
- GeneralTransform2DTo3D.cs
- StartUpEventArgs.cs
- DataRelation.cs
- TaiwanCalendar.cs
- CollectionViewProxy.cs
- UnknownWrapper.cs
- ServerIdentity.cs
- ClientTarget.cs
- TextEditorTyping.cs
- PopupEventArgs.cs
- Button.cs
- BufferModeSettings.cs
- SqlBuilder.cs
- WorkflowServiceHost.cs
- HostAdapter.cs
- WebServiceFaultDesigner.cs
- MessageBox.cs
- FlowLayout.cs
- SoapElementAttribute.cs
- EpmTargetPathSegment.cs
- DataSourceControl.cs
- TerminatorSinks.cs
- TextMessageEncodingElement.cs
- PassportPrincipal.cs
- SqlDataSourceCommandEventArgs.cs
- EntitySqlQueryState.cs
- URLBuilder.cs
- InteropEnvironment.cs
- Point4D.cs
- DecimalConstantAttribute.cs
- XmlElementCollection.cs
- ImageMap.cs
- _SSPISessionCache.cs
- BindingCollectionElement.cs
- PolicyManager.cs
- PropertyTab.cs
- Win32Exception.cs
- AncestorChangedEventArgs.cs
- NameTable.cs
- SerializationSectionGroup.cs
- PrintDialog.cs
- WebPartCloseVerb.cs
- HostingEnvironmentException.cs
- RefreshEventArgs.cs
- FloaterParaClient.cs
- ParserHooks.cs
- RecordBuilder.cs
- NavigationWindow.cs
- SelectionEditingBehavior.cs