Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / Internal / ExpressionLink.cs / 2 / ExpressionLink.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.Common.CommandTrees.Internal { internal delegate void ExpressionLinkConstraint(ExpressionLink link, DbExpression newValue); internal class ExpressionLink { private string _name; private DbCommandTree _tree; private DbExpression _expression; private TypeUsage _expectedType; ////// Creates a new ExpressionLink. The Expected Type and inital value are not set. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink internal ExpressionLink(string name, DbCommandTree commandTree) { _name = name; _tree = EntityUtil.CheckArgumentNull(commandTree, "commandTree"); } ////// Creates a new ExpressionLink with the specified initial value. The Expected Type of the ExpressionLink is fixed as the Type of the initial value /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// An expression that provides the initial value of the ExpressionLink internal ExpressionLink(string strName, DbCommandTree commandTree, DbExpression initialValue) : this(strName, commandTree) { // // Attempt to set the DbExpression property to the specified initial value // this.InitializeValue(initialValue); // // If initialization was successful then fix the type of the initial value as the type to which subsequent expressions values must be promotable // this.SetExpectedType(initialValue.ResultType); } ////// Creates a new ExpressionLink with the specified expected type. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// A description of the type to which the types of values assigned to this ExpressionLink must be promotable internal ExpressionLink(string name, DbCommandTree commandTree, TypeUsage expectedType) : this(name, commandTree) { // // Fix the specified type as the type to which future values must be promotable // this.SetExpectedType(expectedType); } ////// Creates a new ExpressionLink with the specified expected type and initial value. If the specified value is not promotable to the expected type an exception is thrown. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// A description of the type to which the types of values assigned to this ExpressionLink must be promotable /// A DbExpression that provides the initial value of the ExpressionLink ///If the Type of the inital value is not promotable to the expected type internal ExpressionLink(string name, DbCommandTree commandTree, TypeUsage expectedType, DbExpression initialValue) : this(name, commandTree) { // // Fix the specified type as the type to which future values must be promotable // this.SetExpectedType(expectedType); // // Attempt to set the DbExpression property to the specified initial value // this.InitializeValue(initialValue); } ////// Creates a new ExpressionLink with the specified expected primitive type and initial value. If the specified value is not promotable to the expected ModelType an exception is thrown. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// The primitive type to which the types of values assigned to this ExpressionLink must be promotable /// A DbExpression that provides the initial value of the ExpressionLink ///If the Type of the inital value is not promotable to the expected primitive type internal ExpressionLink(string name, DbCommandTree commandTree, PrimitiveTypeKind expectedPrimitiveType, DbExpression initialValue) : this(name, commandTree) { // initialValue cannot be null EntityUtil.CheckArgumentNull(initialValue, this.Name); // // Ensure initialValue is the expected primitive type // PrimitiveTypeKind valueTypeKind; bool valueIsPrimitive = TypeHelpers.TryGetPrimitiveTypeKind(initialValue.ResultType, out valueTypeKind); if( !valueIsPrimitive || valueTypeKind != expectedPrimitiveType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionLink_TypeMismatch(Enum.GetName(typeof(PrimitiveTypeKind), expectedPrimitiveType), valueIsPrimitive ? Enum.GetName(typeof(PrimitiveTypeKind), valueTypeKind) : TypeHelpers.GetFullName(initialValue.ResultType)), this.Name); } // // Fix the specified type as the type to which future values must be promotable // this.SetExpectedType(initialValue.ResultType); // // Attempt to set the DbExpression property to the specified initial value // this.InitializeValue(initialValue); } internal string Name { get { return _name; } } public DbExpression Expression { get { return _expression; } set { // // Verify that the expression satisifes the constraints of this ExpressionLink // if (this.CheckValue(value)) { // Update the DbExpression property and inform the owner tree that it should be considered 'modified' this.UpdateValue(value); _tree.SetModified(); } } } ////// Sets the DbExpression value of this ExpressionLink without instructing the owning command tree to consider itself 'modified'. /// Should only be used when setting the initial value of the ExpressionLink. /// /// internal void InitializeValue(DbExpression initialValue) { this.CheckValue(initialValue); this.UpdateValue(initialValue); } ////// Sets the type to which the types of values assigned to this ExpressionLink must be promotable. /// Can only be set once. Subsequent attempts to set the expected type will result in an exception. /// /// A TypeUsage instance that describes the expected type ///If the expected type has already been set internal void SetExpectedType(TypeUsage expectedType) { Debug.Assert(null == _expectedType, "ExpressionLink.SetExpectedType should only be called once"); Debug.Assert(expectedType.IsReadOnly, "Editable type used as ExpressionLink expected type"); _expectedType = expectedType; } internal event ExpressionLinkConstraint ValueChanging; #if EXPRESSIONLINK_FIRE_CHANGED internal event ExpressionLinkConstraint ValueChanged; #endif private bool CheckValue(DbExpression newValue) { // // Is the DbExpression null? // EntityUtil.CheckArgumentNull(newValue, this.Name); if (object.ReferenceEquals(newValue, _expression)) { return false; } // Does the DbExpression belong to the expected command tree? if (!object.ReferenceEquals(_tree, newValue.CommandTree)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, this.Name); } // Is the Type of the new value promotable to the Type of the old value? if (_expectedType != null) { if (!TypeSemantics.IsEquivalentOrPromotableTo(newValue.ResultType, _expectedType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionLink_TypeMismatch( TypeHelpers.GetFullName(_expectedType), TypeHelpers.GetFullName(newValue.ResultType) ), this.Name ); } } // If the Type of the new value is NullType, throw if NullType is not allowed if (TypeSemantics.IsNullType(newValue.ResultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionLink_NullTypeInvalid(this.Name), this.Name); } // // All invariant constraints have been satisfied, so raise the Changing event to allow dynamic constraints to be tested // if (this.ValueChanging != null) { this.ValueChanging(this, newValue); } return true; } private void UpdateValue(DbExpression newValue) { _expression = newValue; #if EXPRESSIONLINK_FIRECHANGED if (this.ValueChanged != null) { this.ValueChanged(this, newValue); } #endif } } // End of class ExpressionLink } // End of namespace System.Data.Common.CommandTrees.Internal // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.Common.CommandTrees.Internal { internal delegate void ExpressionLinkConstraint(ExpressionLink link, DbExpression newValue); internal class ExpressionLink { private string _name; private DbCommandTree _tree; private DbExpression _expression; private TypeUsage _expectedType; ////// Creates a new ExpressionLink. The Expected Type and inital value are not set. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink internal ExpressionLink(string name, DbCommandTree commandTree) { _name = name; _tree = EntityUtil.CheckArgumentNull(commandTree, "commandTree"); } ////// Creates a new ExpressionLink with the specified initial value. The Expected Type of the ExpressionLink is fixed as the Type of the initial value /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// An expression that provides the initial value of the ExpressionLink internal ExpressionLink(string strName, DbCommandTree commandTree, DbExpression initialValue) : this(strName, commandTree) { // // Attempt to set the DbExpression property to the specified initial value // this.InitializeValue(initialValue); // // If initialization was successful then fix the type of the initial value as the type to which subsequent expressions values must be promotable // this.SetExpectedType(initialValue.ResultType); } ////// Creates a new ExpressionLink with the specified expected type. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// A description of the type to which the types of values assigned to this ExpressionLink must be promotable internal ExpressionLink(string name, DbCommandTree commandTree, TypeUsage expectedType) : this(name, commandTree) { // // Fix the specified type as the type to which future values must be promotable // this.SetExpectedType(expectedType); } ////// Creates a new ExpressionLink with the specified expected type and initial value. If the specified value is not promotable to the expected type an exception is thrown. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// A description of the type to which the types of values assigned to this ExpressionLink must be promotable /// A DbExpression that provides the initial value of the ExpressionLink ///If the Type of the inital value is not promotable to the expected type internal ExpressionLink(string name, DbCommandTree commandTree, TypeUsage expectedType, DbExpression initialValue) : this(name, commandTree) { // // Fix the specified type as the type to which future values must be promotable // this.SetExpectedType(expectedType); // // Attempt to set the DbExpression property to the specified initial value // this.InitializeValue(initialValue); } ////// Creates a new ExpressionLink with the specified expected primitive type and initial value. If the specified value is not promotable to the expected ModelType an exception is thrown. /// /// The name of the DbExpression property that this ExpressionLink implements /// The command tree that owns this ExpressionLink /// The primitive type to which the types of values assigned to this ExpressionLink must be promotable /// A DbExpression that provides the initial value of the ExpressionLink ///If the Type of the inital value is not promotable to the expected primitive type internal ExpressionLink(string name, DbCommandTree commandTree, PrimitiveTypeKind expectedPrimitiveType, DbExpression initialValue) : this(name, commandTree) { // initialValue cannot be null EntityUtil.CheckArgumentNull(initialValue, this.Name); // // Ensure initialValue is the expected primitive type // PrimitiveTypeKind valueTypeKind; bool valueIsPrimitive = TypeHelpers.TryGetPrimitiveTypeKind(initialValue.ResultType, out valueTypeKind); if( !valueIsPrimitive || valueTypeKind != expectedPrimitiveType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionLink_TypeMismatch(Enum.GetName(typeof(PrimitiveTypeKind), expectedPrimitiveType), valueIsPrimitive ? Enum.GetName(typeof(PrimitiveTypeKind), valueTypeKind) : TypeHelpers.GetFullName(initialValue.ResultType)), this.Name); } // // Fix the specified type as the type to which future values must be promotable // this.SetExpectedType(initialValue.ResultType); // // Attempt to set the DbExpression property to the specified initial value // this.InitializeValue(initialValue); } internal string Name { get { return _name; } } public DbExpression Expression { get { return _expression; } set { // // Verify that the expression satisifes the constraints of this ExpressionLink // if (this.CheckValue(value)) { // Update the DbExpression property and inform the owner tree that it should be considered 'modified' this.UpdateValue(value); _tree.SetModified(); } } } ////// Sets the DbExpression value of this ExpressionLink without instructing the owning command tree to consider itself 'modified'. /// Should only be used when setting the initial value of the ExpressionLink. /// /// internal void InitializeValue(DbExpression initialValue) { this.CheckValue(initialValue); this.UpdateValue(initialValue); } ////// Sets the type to which the types of values assigned to this ExpressionLink must be promotable. /// Can only be set once. Subsequent attempts to set the expected type will result in an exception. /// /// A TypeUsage instance that describes the expected type ///If the expected type has already been set internal void SetExpectedType(TypeUsage expectedType) { Debug.Assert(null == _expectedType, "ExpressionLink.SetExpectedType should only be called once"); Debug.Assert(expectedType.IsReadOnly, "Editable type used as ExpressionLink expected type"); _expectedType = expectedType; } internal event ExpressionLinkConstraint ValueChanging; #if EXPRESSIONLINK_FIRE_CHANGED internal event ExpressionLinkConstraint ValueChanged; #endif private bool CheckValue(DbExpression newValue) { // // Is the DbExpression null? // EntityUtil.CheckArgumentNull(newValue, this.Name); if (object.ReferenceEquals(newValue, _expression)) { return false; } // Does the DbExpression belong to the expected command tree? if (!object.ReferenceEquals(_tree, newValue.CommandTree)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, this.Name); } // Is the Type of the new value promotable to the Type of the old value? if (_expectedType != null) { if (!TypeSemantics.IsEquivalentOrPromotableTo(newValue.ResultType, _expectedType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionLink_TypeMismatch( TypeHelpers.GetFullName(_expectedType), TypeHelpers.GetFullName(newValue.ResultType) ), this.Name ); } } // If the Type of the new value is NullType, throw if NullType is not allowed if (TypeSemantics.IsNullType(newValue.ResultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionLink_NullTypeInvalid(this.Name), this.Name); } // // All invariant constraints have been satisfied, so raise the Changing event to allow dynamic constraints to be tested // if (this.ValueChanging != null) { this.ValueChanging(this, newValue); } return true; } private void UpdateValue(DbExpression newValue) { _expression = newValue; #if EXPRESSIONLINK_FIRECHANGED if (this.ValueChanged != null) { this.ValueChanged(this, newValue); } #endif } } // End of class ExpressionLink } // End of namespace System.Data.Common.CommandTrees.Internal // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
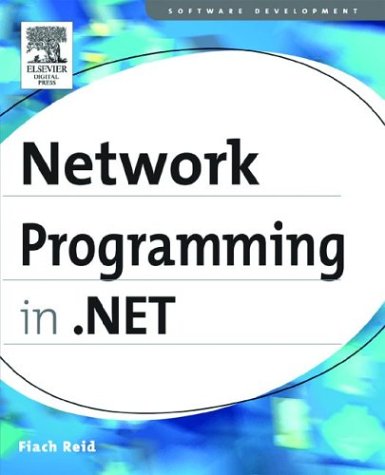
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeobfuscatingStream.cs
- XamlWriter.cs
- UserControl.cs
- StrokeNodeEnumerator.cs
- XmlBindingWorker.cs
- PeerCollaborationPermission.cs
- SByteConverter.cs
- SettingsPropertyIsReadOnlyException.cs
- DtrList.cs
- EntityType.cs
- SubpageParaClient.cs
- BulletDecorator.cs
- BorderSidesEditor.cs
- Propagator.Evaluator.cs
- SqlGenerator.cs
- CodeGenerator.cs
- OutputCacheSettingsSection.cs
- Vector3DCollectionValueSerializer.cs
- SqlRemoveConstantOrderBy.cs
- UInt32Storage.cs
- SingleObjectCollection.cs
- GlobalizationSection.cs
- HttpListenerRequest.cs
- CLRBindingWorker.cs
- messageonlyhwndwrapper.cs
- EventManager.cs
- Calendar.cs
- XmlCollation.cs
- indexingfiltermarshaler.cs
- EnumerableCollectionView.cs
- WinEventHandler.cs
- AppDomainFactory.cs
- MimeTypeMapper.cs
- WebServiceFault.cs
- DataGridViewCellValueEventArgs.cs
- RotateTransform3D.cs
- OperatingSystem.cs
- _NativeSSPI.cs
- SchemaNotation.cs
- Int32AnimationBase.cs
- TablePattern.cs
- StateInitializationDesigner.cs
- UnsafePeerToPeerMethods.cs
- NamespaceList.cs
- ClientCultureInfo.cs
- AutomationProperties.cs
- PresentationTraceSources.cs
- ResolveDuplex11AsyncResult.cs
- LicenseContext.cs
- TraceSection.cs
- ToolStripSystemRenderer.cs
- PropertyPathWorker.cs
- ProfessionalColorTable.cs
- BaseDataListActionList.cs
- GeneratedContractType.cs
- HttpWebRequestElement.cs
- CodeDirectoryCompiler.cs
- CodeTypeDeclaration.cs
- BufferedGraphicsManager.cs
- HighlightVisual.cs
- DateTimeConstantAttribute.cs
- CmsUtils.cs
- VisualStyleElement.cs
- WebHttpBinding.cs
- ExpressionParser.cs
- VectorCollectionValueSerializer.cs
- ToolStripEditorManager.cs
- HandlerMappingMemo.cs
- SpeechDetectedEventArgs.cs
- ListSortDescriptionCollection.cs
- RangeValuePatternIdentifiers.cs
- RoleService.cs
- Exception.cs
- CodeSubDirectoriesCollection.cs
- GPPOINT.cs
- BrowserDefinitionCollection.cs
- DockAndAnchorLayout.cs
- DataGridColumnCollection.cs
- OpenTypeLayout.cs
- ComAdminInterfaces.cs
- PersonalizationProviderHelper.cs
- SelectionEditor.cs
- GAC.cs
- DeviceContext.cs
- Byte.cs
- LogicalTreeHelper.cs
- ServiceCredentialsSecurityTokenManager.cs
- ContentDisposition.cs
- ImageSource.cs
- HtmlControl.cs
- ConnectionStringsExpressionBuilder.cs
- GridViewCommandEventArgs.cs
- LocalizationParserHooks.cs
- SynchronizationScope.cs
- HttpPostedFileBase.cs
- DesignTimeVisibleAttribute.cs
- ProfileSettings.cs
- UIHelper.cs
- ReflectionPermission.cs
- WebFormDesignerActionService.cs