Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / DataRowCollection.cs / 1 / DataRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; #if WINFSInternalOnly internal #else public #endif sealed class DataRowCollection : InternalDataCollectionBase { private sealed class DataRowTree : RBTree{ internal DataRowTree() : base(TreeAccessMethod.INDEX_ONLY) { } protected override int CompareNode (DataRow record1, DataRow record2) { throw ExceptionBuilder.InternalRBTreeError(RBTreeError.CompareNodeInDataRowTree); } protected override int CompareSateliteTreeNode (DataRow record1, DataRow record2) { throw ExceptionBuilder.InternalRBTreeError(RBTreeError.CompareSateliteTreeNodeInDataRowTree); } } private readonly DataTable table; private readonly DataRowTree list = new DataRowTree(); internal int nullInList = 0; /// /// Creates the DataRowCollection for the given table. /// internal DataRowCollection(DataTable table) { this.table = table; } public override int Count { get { return list.Count; } } ////// public DataRow this[int index] { get { return list[index]; } } ///Gets the row at the specified index. ////// public void Add(DataRow row) { table.AddRow(row, -1); } public void InsertAt(DataRow row, int pos) { if (pos < 0) throw ExceptionBuilder.RowInsertOutOfRange(pos); if (pos >= list.Count) table.AddRow(row, -1); else table.InsertRow(row, -1, pos); } internal void DiffInsertAt(DataRow row, int pos) { if ((pos < 0) || (pos == list.Count)) { table.AddRow(row, pos >-1? pos+1 : -1); return; } if (table.NestedParentRelations.Length > 0) { // get in this trouble only if table has a nested parent // get into trouble if table has JUST a nested parent? how about multi parent! if (pos < list.Count) { if (list[pos] != null) { throw ExceptionBuilder.RowInsertTwice(pos, table.TableName); } list.RemoveAt(pos); nullInList--; table.InsertRow(row, pos+1, pos); } else { while (pos>list.Count) { list.Add(null); nullInList++; } table.AddRow(row, pos+1); } } else { table.InsertRow(row, pos+1, pos > list.Count ? -1 : pos); } } public Int32 IndexOf(DataRow row) { if ((null == row) || (row.Table != this.table) || ((0 == row.RBTreeNodeId) && (row.RowState == DataRowState.Detached))) //Webdata 102857 return -1; return list.IndexOf(row.RBTreeNodeId, row); } ///Adds the specified ///to the object. /// internal DataRow AddWithColumnEvents(params object[] values) { DataRow row = table.NewRow(-1); row.ItemArray = values; table.AddRow(row, -1); return row; } public DataRow Add(params object[] values) { int record = table.NewRecordFromArray(values); DataRow row = table.NewRow(record); table.AddRow(row, -1); return row; } internal void ArrayAdd(DataRow row) { row.RBTreeNodeId = list.Add(row); } internal void ArrayInsert(DataRow row, int pos) { row.RBTreeNodeId = list.Insert(pos, row); } internal void ArrayClear() { list.Clear(); } internal void ArrayRemove(DataRow row) { if (row.RBTreeNodeId == 0) { throw ExceptionBuilder.InternalRBTreeError(RBTreeError.AttachedNodeWithZerorbTreeNodeId); } list.RBDelete(row.RBTreeNodeId); row.RBTreeNodeId = 0; } ///Creates a row using specified values and adds it to the /// ///. /// public DataRow Find(object key) { return table.FindByPrimaryKey(key); } ///Gets /// the row specified by the primary key value. /// ////// public DataRow Find(object[] keys) { return table.FindByPrimaryKey(keys); } ///Gets the row containing the specified primary key values. ////// public void Clear() { table.Clear(false); } ///Clears the collection of all rows. ////// public bool Contains(object key) { return(table.FindByPrimaryKey(key) != null); } ////// Gets a value indicating whether the primary key of any row in the /// collection contains the specified value. /// ////// public bool Contains(object[] keys) { return(table.FindByPrimaryKey(keys) != null); } public override void CopyTo(Array ar, int index) { list.CopyTo(ar, index); } public void CopyTo(DataRow[] array, int index) { list.CopyTo(array, index); } public override IEnumerator GetEnumerator() { return list.GetEnumerator(); } ////// Gets a value indicating if the ///with /// the specified primary key values exists. /// /// public void Remove(DataRow row) { if ((null == row) || (row.Table != table) || (-1 == row.rowID)) { throw ExceptionBuilder.RowOutOfRange(); } if ((row.RowState != DataRowState.Deleted) && (row.RowState != DataRowState.Detached)) row.Delete(); if (row.RowState != DataRowState.Detached) row.AcceptChanges(); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { Remove(this[index]); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Removes the row with the specified index from /// the collection. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; #if WINFSInternalOnly internal #else public #endif sealed class DataRowCollection : InternalDataCollectionBase { private sealed class DataRowTree : RBTree{ internal DataRowTree() : base(TreeAccessMethod.INDEX_ONLY) { } protected override int CompareNode (DataRow record1, DataRow record2) { throw ExceptionBuilder.InternalRBTreeError(RBTreeError.CompareNodeInDataRowTree); } protected override int CompareSateliteTreeNode (DataRow record1, DataRow record2) { throw ExceptionBuilder.InternalRBTreeError(RBTreeError.CompareSateliteTreeNodeInDataRowTree); } } private readonly DataTable table; private readonly DataRowTree list = new DataRowTree(); internal int nullInList = 0; /// /// Creates the DataRowCollection for the given table. /// internal DataRowCollection(DataTable table) { this.table = table; } public override int Count { get { return list.Count; } } ////// public DataRow this[int index] { get { return list[index]; } } ///Gets the row at the specified index. ////// public void Add(DataRow row) { table.AddRow(row, -1); } public void InsertAt(DataRow row, int pos) { if (pos < 0) throw ExceptionBuilder.RowInsertOutOfRange(pos); if (pos >= list.Count) table.AddRow(row, -1); else table.InsertRow(row, -1, pos); } internal void DiffInsertAt(DataRow row, int pos) { if ((pos < 0) || (pos == list.Count)) { table.AddRow(row, pos >-1? pos+1 : -1); return; } if (table.NestedParentRelations.Length > 0) { // get in this trouble only if table has a nested parent // get into trouble if table has JUST a nested parent? how about multi parent! if (pos < list.Count) { if (list[pos] != null) { throw ExceptionBuilder.RowInsertTwice(pos, table.TableName); } list.RemoveAt(pos); nullInList--; table.InsertRow(row, pos+1, pos); } else { while (pos>list.Count) { list.Add(null); nullInList++; } table.AddRow(row, pos+1); } } else { table.InsertRow(row, pos+1, pos > list.Count ? -1 : pos); } } public Int32 IndexOf(DataRow row) { if ((null == row) || (row.Table != this.table) || ((0 == row.RBTreeNodeId) && (row.RowState == DataRowState.Detached))) //Webdata 102857 return -1; return list.IndexOf(row.RBTreeNodeId, row); } ///Adds the specified ///to the object. /// internal DataRow AddWithColumnEvents(params object[] values) { DataRow row = table.NewRow(-1); row.ItemArray = values; table.AddRow(row, -1); return row; } public DataRow Add(params object[] values) { int record = table.NewRecordFromArray(values); DataRow row = table.NewRow(record); table.AddRow(row, -1); return row; } internal void ArrayAdd(DataRow row) { row.RBTreeNodeId = list.Add(row); } internal void ArrayInsert(DataRow row, int pos) { row.RBTreeNodeId = list.Insert(pos, row); } internal void ArrayClear() { list.Clear(); } internal void ArrayRemove(DataRow row) { if (row.RBTreeNodeId == 0) { throw ExceptionBuilder.InternalRBTreeError(RBTreeError.AttachedNodeWithZerorbTreeNodeId); } list.RBDelete(row.RBTreeNodeId); row.RBTreeNodeId = 0; } ///Creates a row using specified values and adds it to the /// ///. /// public DataRow Find(object key) { return table.FindByPrimaryKey(key); } ///Gets /// the row specified by the primary key value. /// ////// public DataRow Find(object[] keys) { return table.FindByPrimaryKey(keys); } ///Gets the row containing the specified primary key values. ////// public void Clear() { table.Clear(false); } ///Clears the collection of all rows. ////// public bool Contains(object key) { return(table.FindByPrimaryKey(key) != null); } ////// Gets a value indicating whether the primary key of any row in the /// collection contains the specified value. /// ////// public bool Contains(object[] keys) { return(table.FindByPrimaryKey(keys) != null); } public override void CopyTo(Array ar, int index) { list.CopyTo(ar, index); } public void CopyTo(DataRow[] array, int index) { list.CopyTo(array, index); } public override IEnumerator GetEnumerator() { return list.GetEnumerator(); } ////// Gets a value indicating if the ///with /// the specified primary key values exists. /// /// public void Remove(DataRow row) { if ((null == row) || (row.Table != table) || (-1 == row.rowID)) { throw ExceptionBuilder.RowOutOfRange(); } if ((row.RowState != DataRowState.Deleted) && (row.RowState != DataRowState.Detached)) row.Delete(); if (row.RowState != DataRowState.Detached) row.AcceptChanges(); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { Remove(this[index]); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Removes the row with the specified index from /// the collection. /// ///
Link Menu
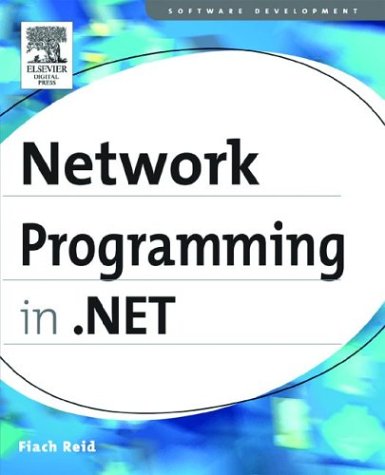
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DashStyle.cs
- SvcMapFile.cs
- List.cs
- ScaleTransform3D.cs
- EncodingDataItem.cs
- EntityEntry.cs
- ConsoleCancelEventArgs.cs
- ToolStripItemClickedEventArgs.cs
- _TimerThread.cs
- PointHitTestResult.cs
- ActivityXRefConverter.cs
- DbUpdateCommandTree.cs
- ObjectComplexPropertyMapping.cs
- XmlSchemaComplexType.cs
- Rotation3DAnimationBase.cs
- HtmlInputRadioButton.cs
- XmlMapping.cs
- AttributeCollection.cs
- FormCollection.cs
- ReadOnlyHierarchicalDataSource.cs
- Vector3DCollectionConverter.cs
- Wildcard.cs
- ToolStripTextBox.cs
- XmlSchemaDatatype.cs
- RepeatInfo.cs
- Storyboard.cs
- LongCountAggregationOperator.cs
- DataRelation.cs
- DecimalConstantAttribute.cs
- CompilerParameters.cs
- IpcChannel.cs
- xmlfixedPageInfo.cs
- DynamicMethod.cs
- BoolExpression.cs
- DictionaryCustomTypeDescriptor.cs
- TextChangedEventArgs.cs
- TargetParameterCountException.cs
- PersonalizationDictionary.cs
- ProfilePropertyMetadata.cs
- MetadataFile.cs
- WindowsEditBox.cs
- ParameterSubsegment.cs
- RelatedView.cs
- CorrelationManager.cs
- OdbcParameter.cs
- ImageIndexConverter.cs
- XmlnsPrefixAttribute.cs
- DataServiceRequestOfT.cs
- AssociationTypeEmitter.cs
- CellIdBoolean.cs
- NativeRightsManagementAPIsStructures.cs
- TreeView.cs
- ScriptReferenceEventArgs.cs
- MenuEventArgs.cs
- SqlClientPermission.cs
- BindingOperations.cs
- Int16Animation.cs
- DATA_BLOB.cs
- ScrollPattern.cs
- ScrollChrome.cs
- SimpleHandlerFactory.cs
- ImportContext.cs
- _Connection.cs
- ListViewItemMouseHoverEvent.cs
- MultipleViewPattern.cs
- XmlMapping.cs
- ServiceDiscoveryElement.cs
- SemanticResolver.cs
- TableCell.cs
- QilPatternVisitor.cs
- SplineKeyFrames.cs
- FocusChangedEventArgs.cs
- SendingRequestEventArgs.cs
- RectangleHotSpot.cs
- SaveFileDialog.cs
- CallbackWrapper.cs
- Win32Native.cs
- RequestTimeoutManager.cs
- ThrowHelper.cs
- ListenerConnectionModeReader.cs
- CLRBindingWorker.cs
- AppearanceEditorPart.cs
- Ref.cs
- SelectionEditingBehavior.cs
- Vector3D.cs
- Point.cs
- EventLogTraceListener.cs
- Border.cs
- InternalUserCancelledException.cs
- BrowsableAttribute.cs
- MailHeaderInfo.cs
- ModifierKeysConverter.cs
- StringOutput.cs
- DataSourceControl.cs
- WindowShowOrOpenTracker.cs
- CompiledIdentityConstraint.cs
- TreeViewTemplateSelector.cs
- Misc.cs
- WebBrowserPermission.cs
- InputScopeAttribute.cs