Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / Instrumentation / SchemaRegistration.cs / 1305376 / SchemaRegistration.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- // namespace System.Management.Instrumentation { using System; using System.Reflection; using System.Collections; using System.Collections.Specialized; using System.Configuration.Install; using System.Management; using Microsoft.Win32; using System.IO; using System.Text; using System.Runtime.InteropServices; using System.Globalization; using System.Runtime.Versioning; class SchemaNaming { class InstallLogWrapper { InstallContext context = null; public InstallLogWrapper(InstallContext context) { this.context = context; } public void LogMessage(string str) { if(null != context) context.LogMessage(str); } } public static SchemaNaming GetSchemaNaming(Assembly assembly) { InstrumentedAttribute attr = InstrumentedAttribute.GetAttribute(assembly); // See if this assembly provides instrumentation if(null == attr) return null; return new SchemaNaming(attr.NamespaceName, attr.SecurityDescriptor, assembly); } Assembly assembly; SchemaNaming(string namespaceName, string securityDescriptor, Assembly assembly) { this.assembly = assembly; assemblyInfo = new AssemblySpecificNaming(namespaceName, securityDescriptor, assembly); if ( DoesInstanceExist(RegistrationPath) == false ) { assemblyInfo.DecoupledProviderInstanceName = AssemblyNameUtility.UniqueToAssemblyMinorVersion(assembly); } } /////////////////////////////////////////// // string constants const string Win32ProviderClassName = "__Win32Provider"; const string EventProviderRegistrationClassName = "__EventProviderRegistration"; const string InstanceProviderRegistrationClassName = "__InstanceProviderRegistration"; const string DecoupledProviderClassName = "MSFT_DecoupledProvider"; const string ProviderClassName = "WMINET_ManagedAssemblyProvider"; const string InstrumentationClassName = "WMINET_Instrumentation"; const string InstrumentedAssembliesClassName = "WMINET_InstrumentedAssembly"; const string DecoupledProviderCLSID = "{54D8502C-527D-43f7-A506-A9DA075E229C}"; const string GlobalWmiNetNamespace = @"root\MicrosoftWmiNet"; const string InstrumentedNamespacesClassName = "WMINET_InstrumentedNamespaces"; const string NamingClassName = "WMINET_Naming"; /////////////////////////////////////////// // class that holds read only naming info // specific to an assembly private class AssemblySpecificNaming { [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public AssemblySpecificNaming(string namespaceName, string securityDescriptor, Assembly assembly) { this.namespaceName = namespaceName; this.securityDescriptor = securityDescriptor; this.decoupledProviderInstanceName = AssemblyNameUtility.UniqueToAssemblyFullVersion(assembly); this.assemblyUniqueIdentifier = AssemblyNameUtility.UniqueToAssemblyBuild(assembly); this.assemblyName = assembly.FullName; this.assemblyPath = assembly.Location; } string namespaceName; string securityDescriptor; string decoupledProviderInstanceName; string assemblyUniqueIdentifier; string assemblyName; string assemblyPath; public string NamespaceName {get {return namespaceName;} } public string SecurityDescriptor {get {return securityDescriptor;} } public string DecoupledProviderInstanceName {get {return decoupledProviderInstanceName;} set { decoupledProviderInstanceName=value;}} public string AssemblyUniqueIdentifier {get {return assemblyUniqueIdentifier;} } public string AssemblyName {get {return assemblyName;} } public string AssemblyPath {get {return assemblyPath;} } } /////////////////////////////////////////// // Accessors for name information // After these methods, there should be no // use of the lower case names AssemblySpecificNaming assemblyInfo; public string NamespaceName {get {return assemblyInfo.NamespaceName;} } public string SecurityDescriptor {get {return assemblyInfo.SecurityDescriptor;} } public string DecoupledProviderInstanceName {get {return assemblyInfo.DecoupledProviderInstanceName;} set {assemblyInfo.DecoupledProviderInstanceName=value;}} string AssemblyUniqueIdentifier {get {return assemblyInfo.AssemblyUniqueIdentifier;} } string AssemblyName {get {return assemblyInfo.AssemblyName;} } string AssemblyPath {get {return assemblyInfo.AssemblyPath;} } string Win32ProviderClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, Win32ProviderClassName);} } string DecoupledProviderClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, DecoupledProviderClassName);} } string InstrumentationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, InstrumentationClassName);} } string EventProviderRegistrationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, EventProviderRegistrationClassName);} } string EventProviderRegistrationPath {get {return AppendProperty(EventProviderRegistrationClassPath, "provider", @"\\\\.\\"+ProviderPath.Replace(@"\", @"\\").Replace(@"""", @"\"""));} } string InstanceProviderRegistrationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, InstanceProviderRegistrationClassName);} } string InstanceProviderRegistrationPath {get {return AppendProperty(InstanceProviderRegistrationClassPath, "provider", @"\\\\.\\"+ProviderPath.Replace(@"\", @"\\").Replace(@"""", @"\"""));} } string ProviderClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, ProviderClassName);} } string ProviderPath {get {return AppendProperty(ProviderClassPath, "Name", assemblyInfo.DecoupledProviderInstanceName);} } string RegistrationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, InstrumentedAssembliesClassName);} } string RegistrationPath {get {return AppendProperty(RegistrationClassPath, "Name", assemblyInfo.DecoupledProviderInstanceName);} } string GlobalRegistrationNamespace {get {return GlobalWmiNetNamespace;} } string GlobalInstrumentationClassPath {get {return MakeClassPath(GlobalWmiNetNamespace, InstrumentationClassName);} } string GlobalRegistrationClassPath {get {return MakeClassPath(GlobalWmiNetNamespace, InstrumentedNamespacesClassName);} } string GlobalRegistrationPath {get {return AppendProperty(GlobalRegistrationClassPath, "NamespaceName", assemblyInfo.NamespaceName.Replace(@"\", @"\\"));} } string GlobalNamingClassPath {get {return MakeClassPath(GlobalWmiNetNamespace, NamingClassName);} } string DataDirectory {get {return Path.Combine(WMICapabilities.FrameworkDirectory, NamespaceName);} } string MofPath {get {return Path.Combine(DataDirectory, DecoupledProviderInstanceName + ".mof");} } string CodePath {get {return Path.Combine(DataDirectory, DecoupledProviderInstanceName + ".cs");} } string PrecompiledAssemblyPath {get {return Path.Combine(DataDirectory, DecoupledProviderInstanceName + ".dll");} } static string MakeClassPath(string namespaceName, string className) { return namespaceName + ":" + className; } static string AppendProperty(string classPath, string propertyName, string propertyValue) { return classPath+'.'+propertyName+"=\""+propertyValue+'\"'; } public bool IsAssemblyRegistered() { if(DoesInstanceExist(RegistrationPath) ) { ManagementObject inst = new ManagementObject(RegistrationPath); // return (0==AssemblyUniqueIdentifier.ToLower(CultureInfo.InvariantCulture).CompareTo(inst["RegisteredBuild"].ToString().ToLower())); return (0==String.Compare(AssemblyUniqueIdentifier,inst["RegisteredBuild"].ToString(),StringComparison.OrdinalIgnoreCase)); } return false; } // Schema has to be compared only if both the below conditions are met // 1. Intance of WMI_InstrumentedAssembly is already present for the given name // 2. If the registeredBuild - which includes unique value for the provider assembly is different private bool IsSchemaToBeCompared() { bool bRet = false; if (DoesInstanceExist(RegistrationPath)) { ManagementObject obj = new ManagementObject(RegistrationPath); // return (0==AssemblyUniqueIdentifier.ToLower(CultureInfo.InvariantCulture).CompareTo(inst["RegisteredBuild"].ToString().ToLower())); bRet = (0 != String.Compare(AssemblyUniqueIdentifier, obj["RegisteredBuild"].ToString(), StringComparison.OrdinalIgnoreCase)); } return bRet; } ManagementObject registrationInstance = null; ManagementObject RegistrationInstance { get { if(null == registrationInstance) registrationInstance = new ManagementObject(RegistrationPath); return registrationInstance; } } public string Code { [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] get { using(StreamReader reader = new StreamReader(CodePath)) { return reader.ReadToEnd(); } } } public string Mof { [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] get { using(StreamReader reader = new StreamReader(MofPath)) { return reader.ReadToEnd(); } } } public Assembly PrecompiledAssembly { [ResourceExposure(ResourceScope.Machine),ResourceConsumption(ResourceScope.Machine)] get { if(File.Exists(PrecompiledAssemblyPath)) return Assembly.LoadFrom(PrecompiledAssemblyPath); return null; } } // function to check if the class to be added to MOF is already present in repository // [[....]] VSUQFE#2248 (VSWhidbey 231885) bool IsClassAlreadyPresentInRepository(ManagementObject obj) { bool bRet = false; string ClassPathInRepository = MakeClassPath(NamespaceName, (string)obj.SystemProperties["__CLASS"].Value); if (DoesClassExist(ClassPathInRepository)) { ManagementObject inst = new ManagementClass(ClassPathInRepository); bRet = inst.CompareTo(obj, ComparisonSettings.IgnoreCase | ComparisonSettings.IgnoreObjectSource); } return bRet; } string GenerateMof(string [] mofs) { return String.Concat( "//*************************************************************************\r\n", String.Format("//* {0}\r\n", DecoupledProviderInstanceName), String.Format("//* {0}\r\n", AssemblyUniqueIdentifier), "//**************************************************************************\r\n", "#pragma autorecover\r\n", EnsureNamespaceInMof(GlobalRegistrationNamespace), EnsureNamespaceInMof(NamespaceName), PragmaNamespace(GlobalRegistrationNamespace), GetMofFormat(new ManagementClass(GlobalInstrumentationClassPath)), GetMofFormat(new ManagementClass(GlobalRegistrationClassPath)), GetMofFormat(new ManagementClass(GlobalNamingClassPath)), GetMofFormat(new ManagementObject(GlobalRegistrationPath)), PragmaNamespace(NamespaceName), GetMofFormat(new ManagementClass(InstrumentationClassPath)), GetMofFormat(new ManagementClass(RegistrationClassPath)), GetMofFormat(new ManagementClass(DecoupledProviderClassPath)), GetMofFormat(new ManagementClass(ProviderClassPath)), GetMofFormat(new ManagementObject(ProviderPath)), // events.Count>0?GetMofFormat(new ManagementObject(EventProviderRegistrationPath)):"", GetMofFormat(new ManagementObject(EventProviderRegistrationPath)), GetMofFormat(new ManagementObject(InstanceProviderRegistrationPath)), String.Concat(mofs), GetMofFormat(new ManagementObject(RegistrationPath)) ); } public void RegisterNonAssemblySpecificSchema(InstallContext installContext) { SecurityHelper.UnmanagedCode.Demand(); // Bug#112640 - Close off any potential use from anything but fully trusted code // Make sure the 'Client' key has the correct permissions WmiNetUtilsHelper.VerifyClientKey_f(); InstallLogWrapper context = new InstallLogWrapper(installContext); EnsureNamespace(context, GlobalRegistrationNamespace); EnsureClassExists(context, GlobalInstrumentationClassPath, new ClassMaker(MakeGlobalInstrumentationClass)); EnsureClassExists(context, GlobalRegistrationClassPath, new ClassMaker(MakeNamespaceRegistrationClass)); EnsureClassExists(context, GlobalNamingClassPath, new ClassMaker(MakeNamingClass)); EnsureNamespace(context, NamespaceName); EnsureClassExists(context, InstrumentationClassPath, new ClassMaker(MakeInstrumentationClass)); EnsureClassExists(context, RegistrationClassPath, new ClassMaker(MakeRegistrationClass)); // Make sure Hosting model is set correctly by default. If not, we blow away the class definition try { ManagementClass cls = new ManagementClass(DecoupledProviderClassPath); if(cls["HostingModel"].ToString() != "Decoupled:Com") { cls.Delete(); } } catch(ManagementException e) { if(e.ErrorCode != ManagementStatus.NotFound) throw e; } EnsureClassExists(context, DecoupledProviderClassPath, new ClassMaker(MakeDecoupledProviderClass)); EnsureClassExists(context, ProviderClassPath, new ClassMaker(MakeProviderClass)); if(!DoesInstanceExist(GlobalRegistrationPath)) RegisterNamespaceAsInstrumented(); } [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public void RegisterAssemblySpecificSchema() { SecurityHelper.UnmanagedCode.Demand(); // Bug#112640 - Close off any potential use from anything but fully trusted code Type[] types = InstrumentedAttribute.GetInstrumentedTypes(assembly); StringCollection events = new StringCollection(); StringCollection instances = new StringCollection(); StringCollection abstracts = new StringCollection(); string[] mofs = new string[types.Length]; CodeWriter code = new CodeWriter(); ReferencesCollection references = new ReferencesCollection(); // Add the node with all the 'using' statements at the top code.AddChild(references.UsingCode); references.Add(typeof(Object)); references.Add(typeof(ManagementClass)); references.Add(typeof(Marshal)); references.Add(typeof(System.Security.SuppressUnmanagedCodeSecurityAttribute)); references.Add(typeof(System.Reflection.FieldInfo)); references.Add(typeof(Hashtable)); // Add a blank line code.Line(); // Add master converter class CodeWriter codeWMIConverter = code.AddChild("public class WMINET_Converter"); // Add master map of types to converters codeWMIConverter.Line("public static Hashtable mapTypeToConverter = new Hashtable();"); // Add master CCTOR CodeWriter codeCCTOR = codeWMIConverter.AddChild("static WMINET_Converter()"); // Make mapping of types to converter class names Hashtable mapTypeToConverterClassName = new Hashtable(); for(int i=0;i= 0) return String.Format(""{0:D4}{1:D2}{2:D2}{3:D2}{4:D2}{5:D2}.{6:D3}000+{7:D3}"", dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, dt.Millisecond, diffUTC); return String.Format(""{0:D4}{1:D2}{2:D2}{3:D2}{4:D2}{5:D2}.{6:D3}000-{7:D3}"", dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, dt.Millisecond, -diffUTC); } public static string WMITimeToString(TimeSpan ts) { return String.Format(""{0:D8}{1:D2}{2:D2}{3:D2}.{4:D3}000:000"", ts.Days, ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds); } public static string[] WMITimeArrayToStringArray(DateTime[] dates) { string[] strings = new string[dates.Length]; for(int i=0;i /// Given a class path, this function will return the ManagementClass /// if it exists, or null if it does not. /// /// WMI path to Class /// static ManagementClass SafeGetClass(string classPath) { ManagementClass theClass = null; try { ManagementClass existingClass = new ManagementClass(classPath); existingClass.Get(); theClass = existingClass; } catch(ManagementException e) { if(e.ErrorCode != ManagementStatus.NotFound) throw e; } return theClass; } /// /// Given a class path, and a ManagementClass class definition, this /// function will create the class if it does not exist, replace the /// class if it exists but is different, or do nothing if the class /// exists and is identical. This is useful for performance reasons /// since it can be expensive to delete an existing class and replace /// it. /// /// WMI path to class /// Class to create or replace static void ReplaceClassIfNecessary(string classPath, ManagementClass newClass) { try { ManagementClass oldClass = SafeGetClass(classPath); if(null == oldClass) newClass.Put(); else { // if(newClass.GetText(TextFormat.Mof) != oldClass.GetText(TextFormat.Mof)) { // oldClass.Delete(); newClass.Put(); } } } catch(ManagementException e) { string strformat = RC.GetString("CLASS_NOTREPLACED_EXCEPT") + "\r\n{0}\r\n{1}"; throw new ArgumentException(String.Format(strformat, classPath, newClass.GetText(TextFormat.Mof)), e); } } static string GetMofFormat(ManagementObject obj) { return obj.GetText(TextFormat.Mof).Replace("\n", "\r\n") + "\r\n"; } static string PragmaNamespace(string namespaceName) { return String.Format("#pragma namespace(\"\\\\\\\\.\\\\{0}\")\r\n\r\n", namespaceName.Replace("\\", "\\\\")); } static string EnsureNamespaceInMof(string baseNamespace, string childNamespaceName) { return String.Format("{0}instance of __Namespace\r\n{{\r\n Name = \"{1}\";\r\n}};\r\n\r\n", PragmaNamespace(baseNamespace), childNamespaceName); } static string EnsureNamespaceInMof(string namespaceName) { string mof=""; string fullNamespace = null; foreach(string name in namespaceName.Split(new char[] {'\\'})) { if(fullNamespace == null) { fullNamespace = name; continue; } mof+=EnsureNamespaceInMof(fullNamespace, name); fullNamespace += "\\" + name; } return mof; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- // namespace System.Management.Instrumentation { using System; using System.Reflection; using System.Collections; using System.Collections.Specialized; using System.Configuration.Install; using System.Management; using Microsoft.Win32; using System.IO; using System.Text; using System.Runtime.InteropServices; using System.Globalization; using System.Runtime.Versioning; class SchemaNaming { class InstallLogWrapper { InstallContext context = null; public InstallLogWrapper(InstallContext context) { this.context = context; } public void LogMessage(string str) { if(null != context) context.LogMessage(str); } } public static SchemaNaming GetSchemaNaming(Assembly assembly) { InstrumentedAttribute attr = InstrumentedAttribute.GetAttribute(assembly); // See if this assembly provides instrumentation if(null == attr) return null; return new SchemaNaming(attr.NamespaceName, attr.SecurityDescriptor, assembly); } Assembly assembly; SchemaNaming(string namespaceName, string securityDescriptor, Assembly assembly) { this.assembly = assembly; assemblyInfo = new AssemblySpecificNaming(namespaceName, securityDescriptor, assembly); if ( DoesInstanceExist(RegistrationPath) == false ) { assemblyInfo.DecoupledProviderInstanceName = AssemblyNameUtility.UniqueToAssemblyMinorVersion(assembly); } } /////////////////////////////////////////// // string constants const string Win32ProviderClassName = "__Win32Provider"; const string EventProviderRegistrationClassName = "__EventProviderRegistration"; const string InstanceProviderRegistrationClassName = "__InstanceProviderRegistration"; const string DecoupledProviderClassName = "MSFT_DecoupledProvider"; const string ProviderClassName = "WMINET_ManagedAssemblyProvider"; const string InstrumentationClassName = "WMINET_Instrumentation"; const string InstrumentedAssembliesClassName = "WMINET_InstrumentedAssembly"; const string DecoupledProviderCLSID = "{54D8502C-527D-43f7-A506-A9DA075E229C}"; const string GlobalWmiNetNamespace = @"root\MicrosoftWmiNet"; const string InstrumentedNamespacesClassName = "WMINET_InstrumentedNamespaces"; const string NamingClassName = "WMINET_Naming"; /////////////////////////////////////////// // class that holds read only naming info // specific to an assembly private class AssemblySpecificNaming { [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public AssemblySpecificNaming(string namespaceName, string securityDescriptor, Assembly assembly) { this.namespaceName = namespaceName; this.securityDescriptor = securityDescriptor; this.decoupledProviderInstanceName = AssemblyNameUtility.UniqueToAssemblyFullVersion(assembly); this.assemblyUniqueIdentifier = AssemblyNameUtility.UniqueToAssemblyBuild(assembly); this.assemblyName = assembly.FullName; this.assemblyPath = assembly.Location; } string namespaceName; string securityDescriptor; string decoupledProviderInstanceName; string assemblyUniqueIdentifier; string assemblyName; string assemblyPath; public string NamespaceName {get {return namespaceName;} } public string SecurityDescriptor {get {return securityDescriptor;} } public string DecoupledProviderInstanceName {get {return decoupledProviderInstanceName;} set { decoupledProviderInstanceName=value;}} public string AssemblyUniqueIdentifier {get {return assemblyUniqueIdentifier;} } public string AssemblyName {get {return assemblyName;} } public string AssemblyPath {get {return assemblyPath;} } } /////////////////////////////////////////// // Accessors for name information // After these methods, there should be no // use of the lower case names AssemblySpecificNaming assemblyInfo; public string NamespaceName {get {return assemblyInfo.NamespaceName;} } public string SecurityDescriptor {get {return assemblyInfo.SecurityDescriptor;} } public string DecoupledProviderInstanceName {get {return assemblyInfo.DecoupledProviderInstanceName;} set {assemblyInfo.DecoupledProviderInstanceName=value;}} string AssemblyUniqueIdentifier {get {return assemblyInfo.AssemblyUniqueIdentifier;} } string AssemblyName {get {return assemblyInfo.AssemblyName;} } string AssemblyPath {get {return assemblyInfo.AssemblyPath;} } string Win32ProviderClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, Win32ProviderClassName);} } string DecoupledProviderClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, DecoupledProviderClassName);} } string InstrumentationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, InstrumentationClassName);} } string EventProviderRegistrationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, EventProviderRegistrationClassName);} } string EventProviderRegistrationPath {get {return AppendProperty(EventProviderRegistrationClassPath, "provider", @"\\\\.\\"+ProviderPath.Replace(@"\", @"\\").Replace(@"""", @"\"""));} } string InstanceProviderRegistrationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, InstanceProviderRegistrationClassName);} } string InstanceProviderRegistrationPath {get {return AppendProperty(InstanceProviderRegistrationClassPath, "provider", @"\\\\.\\"+ProviderPath.Replace(@"\", @"\\").Replace(@"""", @"\"""));} } string ProviderClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, ProviderClassName);} } string ProviderPath {get {return AppendProperty(ProviderClassPath, "Name", assemblyInfo.DecoupledProviderInstanceName);} } string RegistrationClassPath {get {return MakeClassPath(assemblyInfo.NamespaceName, InstrumentedAssembliesClassName);} } string RegistrationPath {get {return AppendProperty(RegistrationClassPath, "Name", assemblyInfo.DecoupledProviderInstanceName);} } string GlobalRegistrationNamespace {get {return GlobalWmiNetNamespace;} } string GlobalInstrumentationClassPath {get {return MakeClassPath(GlobalWmiNetNamespace, InstrumentationClassName);} } string GlobalRegistrationClassPath {get {return MakeClassPath(GlobalWmiNetNamespace, InstrumentedNamespacesClassName);} } string GlobalRegistrationPath {get {return AppendProperty(GlobalRegistrationClassPath, "NamespaceName", assemblyInfo.NamespaceName.Replace(@"\", @"\\"));} } string GlobalNamingClassPath {get {return MakeClassPath(GlobalWmiNetNamespace, NamingClassName);} } string DataDirectory {get {return Path.Combine(WMICapabilities.FrameworkDirectory, NamespaceName);} } string MofPath {get {return Path.Combine(DataDirectory, DecoupledProviderInstanceName + ".mof");} } string CodePath {get {return Path.Combine(DataDirectory, DecoupledProviderInstanceName + ".cs");} } string PrecompiledAssemblyPath {get {return Path.Combine(DataDirectory, DecoupledProviderInstanceName + ".dll");} } static string MakeClassPath(string namespaceName, string className) { return namespaceName + ":" + className; } static string AppendProperty(string classPath, string propertyName, string propertyValue) { return classPath+'.'+propertyName+"=\""+propertyValue+'\"'; } public bool IsAssemblyRegistered() { if(DoesInstanceExist(RegistrationPath) ) { ManagementObject inst = new ManagementObject(RegistrationPath); // return (0==AssemblyUniqueIdentifier.ToLower(CultureInfo.InvariantCulture).CompareTo(inst["RegisteredBuild"].ToString().ToLower())); return (0==String.Compare(AssemblyUniqueIdentifier,inst["RegisteredBuild"].ToString(),StringComparison.OrdinalIgnoreCase)); } return false; } // Schema has to be compared only if both the below conditions are met // 1. Intance of WMI_InstrumentedAssembly is already present for the given name // 2. If the registeredBuild - which includes unique value for the provider assembly is different private bool IsSchemaToBeCompared() { bool bRet = false; if (DoesInstanceExist(RegistrationPath)) { ManagementObject obj = new ManagementObject(RegistrationPath); // return (0==AssemblyUniqueIdentifier.ToLower(CultureInfo.InvariantCulture).CompareTo(inst["RegisteredBuild"].ToString().ToLower())); bRet = (0 != String.Compare(AssemblyUniqueIdentifier, obj["RegisteredBuild"].ToString(), StringComparison.OrdinalIgnoreCase)); } return bRet; } ManagementObject registrationInstance = null; ManagementObject RegistrationInstance { get { if(null == registrationInstance) registrationInstance = new ManagementObject(RegistrationPath); return registrationInstance; } } public string Code { [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] get { using(StreamReader reader = new StreamReader(CodePath)) { return reader.ReadToEnd(); } } } public string Mof { [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] get { using(StreamReader reader = new StreamReader(MofPath)) { return reader.ReadToEnd(); } } } public Assembly PrecompiledAssembly { [ResourceExposure(ResourceScope.Machine),ResourceConsumption(ResourceScope.Machine)] get { if(File.Exists(PrecompiledAssemblyPath)) return Assembly.LoadFrom(PrecompiledAssemblyPath); return null; } } // function to check if the class to be added to MOF is already present in repository // [[....]] VSUQFE#2248 (VSWhidbey 231885) bool IsClassAlreadyPresentInRepository(ManagementObject obj) { bool bRet = false; string ClassPathInRepository = MakeClassPath(NamespaceName, (string)obj.SystemProperties["__CLASS"].Value); if (DoesClassExist(ClassPathInRepository)) { ManagementObject inst = new ManagementClass(ClassPathInRepository); bRet = inst.CompareTo(obj, ComparisonSettings.IgnoreCase | ComparisonSettings.IgnoreObjectSource); } return bRet; } string GenerateMof(string [] mofs) { return String.Concat( "//*************************************************************************\r\n", String.Format("//* {0}\r\n", DecoupledProviderInstanceName), String.Format("//* {0}\r\n", AssemblyUniqueIdentifier), "//**************************************************************************\r\n", "#pragma autorecover\r\n", EnsureNamespaceInMof(GlobalRegistrationNamespace), EnsureNamespaceInMof(NamespaceName), PragmaNamespace(GlobalRegistrationNamespace), GetMofFormat(new ManagementClass(GlobalInstrumentationClassPath)), GetMofFormat(new ManagementClass(GlobalRegistrationClassPath)), GetMofFormat(new ManagementClass(GlobalNamingClassPath)), GetMofFormat(new ManagementObject(GlobalRegistrationPath)), PragmaNamespace(NamespaceName), GetMofFormat(new ManagementClass(InstrumentationClassPath)), GetMofFormat(new ManagementClass(RegistrationClassPath)), GetMofFormat(new ManagementClass(DecoupledProviderClassPath)), GetMofFormat(new ManagementClass(ProviderClassPath)), GetMofFormat(new ManagementObject(ProviderPath)), // events.Count>0?GetMofFormat(new ManagementObject(EventProviderRegistrationPath)):"", GetMofFormat(new ManagementObject(EventProviderRegistrationPath)), GetMofFormat(new ManagementObject(InstanceProviderRegistrationPath)), String.Concat(mofs), GetMofFormat(new ManagementObject(RegistrationPath)) ); } public void RegisterNonAssemblySpecificSchema(InstallContext installContext) { SecurityHelper.UnmanagedCode.Demand(); // Bug#112640 - Close off any potential use from anything but fully trusted code // Make sure the 'Client' key has the correct permissions WmiNetUtilsHelper.VerifyClientKey_f(); InstallLogWrapper context = new InstallLogWrapper(installContext); EnsureNamespace(context, GlobalRegistrationNamespace); EnsureClassExists(context, GlobalInstrumentationClassPath, new ClassMaker(MakeGlobalInstrumentationClass)); EnsureClassExists(context, GlobalRegistrationClassPath, new ClassMaker(MakeNamespaceRegistrationClass)); EnsureClassExists(context, GlobalNamingClassPath, new ClassMaker(MakeNamingClass)); EnsureNamespace(context, NamespaceName); EnsureClassExists(context, InstrumentationClassPath, new ClassMaker(MakeInstrumentationClass)); EnsureClassExists(context, RegistrationClassPath, new ClassMaker(MakeRegistrationClass)); // Make sure Hosting model is set correctly by default. If not, we blow away the class definition try { ManagementClass cls = new ManagementClass(DecoupledProviderClassPath); if(cls["HostingModel"].ToString() != "Decoupled:Com") { cls.Delete(); } } catch(ManagementException e) { if(e.ErrorCode != ManagementStatus.NotFound) throw e; } EnsureClassExists(context, DecoupledProviderClassPath, new ClassMaker(MakeDecoupledProviderClass)); EnsureClassExists(context, ProviderClassPath, new ClassMaker(MakeProviderClass)); if(!DoesInstanceExist(GlobalRegistrationPath)) RegisterNamespaceAsInstrumented(); } [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public void RegisterAssemblySpecificSchema() { SecurityHelper.UnmanagedCode.Demand(); // Bug#112640 - Close off any potential use from anything but fully trusted code Type[] types = InstrumentedAttribute.GetInstrumentedTypes(assembly); StringCollection events = new StringCollection(); StringCollection instances = new StringCollection(); StringCollection abstracts = new StringCollection(); string[] mofs = new string[types.Length]; CodeWriter code = new CodeWriter(); ReferencesCollection references = new ReferencesCollection(); // Add the node with all the 'using' statements at the top code.AddChild(references.UsingCode); references.Add(typeof(Object)); references.Add(typeof(ManagementClass)); references.Add(typeof(Marshal)); references.Add(typeof(System.Security.SuppressUnmanagedCodeSecurityAttribute)); references.Add(typeof(System.Reflection.FieldInfo)); references.Add(typeof(Hashtable)); // Add a blank line code.Line(); // Add master converter class CodeWriter codeWMIConverter = code.AddChild("public class WMINET_Converter"); // Add master map of types to converters codeWMIConverter.Line("public static Hashtable mapTypeToConverter = new Hashtable();"); // Add master CCTOR CodeWriter codeCCTOR = codeWMIConverter.AddChild("static WMINET_Converter()"); // Make mapping of types to converter class names Hashtable mapTypeToConverterClassName = new Hashtable(); for(int i=0;i= 0) return String.Format(""{0:D4}{1:D2}{2:D2}{3:D2}{4:D2}{5:D2}.{6:D3}000+{7:D3}"", dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, dt.Millisecond, diffUTC); return String.Format(""{0:D4}{1:D2}{2:D2}{3:D2}{4:D2}{5:D2}.{6:D3}000-{7:D3}"", dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, dt.Millisecond, -diffUTC); } public static string WMITimeToString(TimeSpan ts) { return String.Format(""{0:D8}{1:D2}{2:D2}{3:D2}.{4:D3}000:000"", ts.Days, ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds); } public static string[] WMITimeArrayToStringArray(DateTime[] dates) { string[] strings = new string[dates.Length]; for(int i=0;i /// Given a class path, this function will return the ManagementClass /// if it exists, or null if it does not. /// /// WMI path to Class /// static ManagementClass SafeGetClass(string classPath) { ManagementClass theClass = null; try { ManagementClass existingClass = new ManagementClass(classPath); existingClass.Get(); theClass = existingClass; } catch(ManagementException e) { if(e.ErrorCode != ManagementStatus.NotFound) throw e; } return theClass; } /// /// Given a class path, and a ManagementClass class definition, this /// function will create the class if it does not exist, replace the /// class if it exists but is different, or do nothing if the class /// exists and is identical. This is useful for performance reasons /// since it can be expensive to delete an existing class and replace /// it. /// /// WMI path to class /// Class to create or replace static void ReplaceClassIfNecessary(string classPath, ManagementClass newClass) { try { ManagementClass oldClass = SafeGetClass(classPath); if(null == oldClass) newClass.Put(); else { // if(newClass.GetText(TextFormat.Mof) != oldClass.GetText(TextFormat.Mof)) { // oldClass.Delete(); newClass.Put(); } } } catch(ManagementException e) { string strformat = RC.GetString("CLASS_NOTREPLACED_EXCEPT") + "\r\n{0}\r\n{1}"; throw new ArgumentException(String.Format(strformat, classPath, newClass.GetText(TextFormat.Mof)), e); } } static string GetMofFormat(ManagementObject obj) { return obj.GetText(TextFormat.Mof).Replace("\n", "\r\n") + "\r\n"; } static string PragmaNamespace(string namespaceName) { return String.Format("#pragma namespace(\"\\\\\\\\.\\\\{0}\")\r\n\r\n", namespaceName.Replace("\\", "\\\\")); } static string EnsureNamespaceInMof(string baseNamespace, string childNamespaceName) { return String.Format("{0}instance of __Namespace\r\n{{\r\n Name = \"{1}\";\r\n}};\r\n\r\n", PragmaNamespace(baseNamespace), childNamespaceName); } static string EnsureNamespaceInMof(string namespaceName) { string mof=""; string fullNamespace = null; foreach(string name in namespaceName.Split(new char[] {'\\'})) { if(fullNamespace == null) { fullNamespace = name; continue; } mof+=EnsureNamespaceInMof(fullNamespace, name); fullNamespace += "\\" + name; } return mof; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
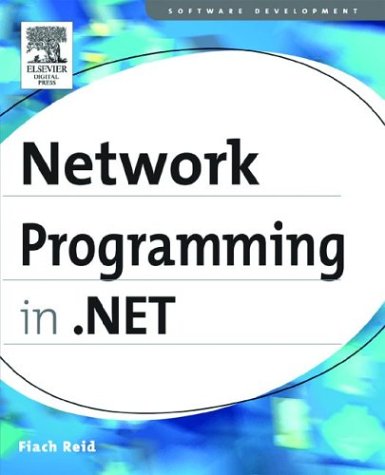
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KnownTypeDataContractResolver.cs
- FormViewRow.cs
- DynamicResourceExtensionConverter.cs
- TcpHostedTransportConfiguration.cs
- DataControlLinkButton.cs
- PartialList.cs
- NameHandler.cs
- BlurBitmapEffect.cs
- Page.cs
- StylusPointDescription.cs
- WinFormsSecurity.cs
- TagMapInfo.cs
- Substitution.cs
- StrokeFIndices.cs
- SqlCacheDependency.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- ServiceModelActivity.cs
- WebPartMenu.cs
- XmlLoader.cs
- BroadcastEventHelper.cs
- AuthenticationManager.cs
- SerializerProvider.cs
- SHA256Managed.cs
- DataPagerFieldItem.cs
- PagedDataSource.cs
- NullReferenceException.cs
- EncoderNLS.cs
- OleDbEnumerator.cs
- CodeCompiler.cs
- ImageClickEventArgs.cs
- TemplateField.cs
- ExtendedPropertyDescriptor.cs
- HwndSubclass.cs
- JsonCollectionDataContract.cs
- TemplateApplicationHelper.cs
- MemberCollection.cs
- ReadingWritingEntityEventArgs.cs
- MetabaseSettingsIis7.cs
- XamlVector3DCollectionSerializer.cs
- GiveFeedbackEventArgs.cs
- QueryContinueDragEvent.cs
- EntityDataSourceConfigureObjectContext.cs
- DataContext.cs
- PasswordBox.cs
- ExtensionElement.cs
- WebAdminConfigurationHelper.cs
- XD.cs
- PropertyDescriptor.cs
- DirectionalLight.cs
- Rect.cs
- FtpRequestCacheValidator.cs
- SHA384Managed.cs
- IconBitmapDecoder.cs
- CompilerScope.cs
- DecoderExceptionFallback.cs
- TripleDES.cs
- FormattedText.cs
- InboundActivityHelper.cs
- MailBnfHelper.cs
- DynamicMetaObject.cs
- ValidationHelpers.cs
- RuleSettingsCollection.cs
- SqlCommand.cs
- ConfigurationPropertyCollection.cs
- StaticFileHandler.cs
- StyleBamlRecordReader.cs
- CommandBinding.cs
- InkCanvasSelection.cs
- ActionItem.cs
- SiblingIterators.cs
- ParameterSubsegment.cs
- EventData.cs
- MaterialGroup.cs
- TextElementEnumerator.cs
- TraceHwndHost.cs
- FileLogRecord.cs
- BitmapDecoder.cs
- Int32CollectionConverter.cs
- DataGridViewTopRowAccessibleObject.cs
- Model3D.cs
- TiffBitmapEncoder.cs
- TraceContextRecord.cs
- SqlMethods.cs
- NavigationWindow.cs
- EmptyImpersonationContext.cs
- TrustManagerPromptUI.cs
- EditorAttributeInfo.cs
- FloaterParagraph.cs
- ContainerVisual.cs
- MachineKeyConverter.cs
- Timer.cs
- SQLInt16.cs
- ToolStripDropDownMenu.cs
- SqlDataSourceAdvancedOptionsForm.cs
- SortedList.cs
- XmlFormatExtensionAttribute.cs
- TextDecorationLocationValidation.cs
- HtmlMeta.cs
- Win32KeyboardDevice.cs
- MultipleViewProviderWrapper.cs