Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / Command / CommandBinding.cs / 1 / CommandBinding.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows; using System.Windows.Markup; using MS.Internal; using System.Security; using System.Security.Permissions; namespace System.Windows.Input { ////// CommandBinding - Command-EventHandlers map /// CommandBinding acts like a map for EventHandlers and Commands. /// PreviewExecute/Execute, PreviewCanExecute/CanExecute handlers /// can be added at CommandBinding which will exist at Element level /// in the form of a Collection and will be invoked when the system /// is routing the corresponding RoutedEvents. /// public class CommandBinding { #region Constructors ////// Default Constructor - required to allow creation from markup /// public CommandBinding() { } ////// Constructor /// /// Command associated with this binding. public CommandBinding(ICommand command) : this(command, null, null) { } ////// Constructor /// /// Command associated with this binding. /// Handler associated with executing the command. public CommandBinding(ICommand command, ExecutedRoutedEventHandler executed) : this(command, executed, null) { } ////// Constructor /// /// Command associated with this binding. /// Handler associated with executing the command. /// Handler associated with determining if the command can execute. public CommandBinding(ICommand command, ExecutedRoutedEventHandler executed, CanExecuteRoutedEventHandler canExecute) { if (command == null) { throw new ArgumentNullException("command"); } _command = command; if (executed != null) { Executed += executed; } if (canExecute != null) { CanExecute += canExecute; } } #endregion #region Public Properties ////// Command associated with this binding /// [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return _command; } set { if (value == null) { throw new ArgumentNullException("value"); } _command = value; } } #endregion #region Public Events ////// Called before the command is executed. /// public event ExecutedRoutedEventHandler PreviewExecuted; ////// Called when the command is executed. /// public event ExecutedRoutedEventHandler Executed; ////// Called before determining if the command can be executed. /// public event CanExecuteRoutedEventHandler PreviewCanExecute; ////// Called to determine if the command can be executed. /// public event CanExecuteRoutedEventHandler CanExecute; #endregion #region Implementation ////// Calls the CanExecute or PreviewCanExecute event based on the event argument's RoutedEvent. /// /// The sender of the event. /// Event arguments. internal void OnCanExecute(object sender, CanExecuteRoutedEventArgs e) { if (!e.Handled) { if (e.RoutedEvent == CommandManager.CanExecuteEvent) { if (CanExecute != null) { CanExecute(sender, e); if (e.CanExecute) { e.Handled = true; } } else if (!e.CanExecute) { // If there is an Executed handler, then the command can be executed. if (Executed != null) { e.CanExecute = true; e.Handled = true; } } } else // e.RoutedEvent == CommandManager.PreviewCanExecuteEvent { if (PreviewCanExecute != null) { PreviewCanExecute(sender, e); if (e.CanExecute) { e.Handled = true; } } } } } private bool CheckCanExecute(object sender, ExecutedRoutedEventArgs e) { CanExecuteRoutedEventArgs canExecuteArgs = new CanExecuteRoutedEventArgs(e.Command, e.Parameter); canExecuteArgs.RoutedEvent = CommandManager.CanExecuteEvent; // Since we don't actually raise this event, we have to explicitly set the source. canExecuteArgs.Source = e.OriginalSource; canExecuteArgs.OverrideSource(e.Source); OnCanExecute(sender, canExecuteArgs); return canExecuteArgs.CanExecute; } ////// Calls Executed or PreviewExecuted based on the event argument's RoutedEvent. /// /// The sender of the event. /// Event arguments. internal void OnExecuted(object sender, ExecutedRoutedEventArgs e) { if (!e.Handled) { if (e.RoutedEvent == CommandManager.ExecutedEvent) { if (Executed != null) { if (CheckCanExecute(sender, e)) { Executed(sender, e); e.Handled = true; } } } else // e.RoutedEvent == CommandManager.PreviewExecutedEvent { if (PreviewExecuted != null) { if (CheckCanExecute(sender, e)) { PreviewExecuted(sender, e); e.Handled = true; } } } } } #endregion #region Data private ICommand _command; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows; using System.Windows.Markup; using MS.Internal; using System.Security; using System.Security.Permissions; namespace System.Windows.Input { ////// CommandBinding - Command-EventHandlers map /// CommandBinding acts like a map for EventHandlers and Commands. /// PreviewExecute/Execute, PreviewCanExecute/CanExecute handlers /// can be added at CommandBinding which will exist at Element level /// in the form of a Collection and will be invoked when the system /// is routing the corresponding RoutedEvents. /// public class CommandBinding { #region Constructors ////// Default Constructor - required to allow creation from markup /// public CommandBinding() { } ////// Constructor /// /// Command associated with this binding. public CommandBinding(ICommand command) : this(command, null, null) { } ////// Constructor /// /// Command associated with this binding. /// Handler associated with executing the command. public CommandBinding(ICommand command, ExecutedRoutedEventHandler executed) : this(command, executed, null) { } ////// Constructor /// /// Command associated with this binding. /// Handler associated with executing the command. /// Handler associated with determining if the command can execute. public CommandBinding(ICommand command, ExecutedRoutedEventHandler executed, CanExecuteRoutedEventHandler canExecute) { if (command == null) { throw new ArgumentNullException("command"); } _command = command; if (executed != null) { Executed += executed; } if (canExecute != null) { CanExecute += canExecute; } } #endregion #region Public Properties ////// Command associated with this binding /// [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return _command; } set { if (value == null) { throw new ArgumentNullException("value"); } _command = value; } } #endregion #region Public Events ////// Called before the command is executed. /// public event ExecutedRoutedEventHandler PreviewExecuted; ////// Called when the command is executed. /// public event ExecutedRoutedEventHandler Executed; ////// Called before determining if the command can be executed. /// public event CanExecuteRoutedEventHandler PreviewCanExecute; ////// Called to determine if the command can be executed. /// public event CanExecuteRoutedEventHandler CanExecute; #endregion #region Implementation ////// Calls the CanExecute or PreviewCanExecute event based on the event argument's RoutedEvent. /// /// The sender of the event. /// Event arguments. internal void OnCanExecute(object sender, CanExecuteRoutedEventArgs e) { if (!e.Handled) { if (e.RoutedEvent == CommandManager.CanExecuteEvent) { if (CanExecute != null) { CanExecute(sender, e); if (e.CanExecute) { e.Handled = true; } } else if (!e.CanExecute) { // If there is an Executed handler, then the command can be executed. if (Executed != null) { e.CanExecute = true; e.Handled = true; } } } else // e.RoutedEvent == CommandManager.PreviewCanExecuteEvent { if (PreviewCanExecute != null) { PreviewCanExecute(sender, e); if (e.CanExecute) { e.Handled = true; } } } } } private bool CheckCanExecute(object sender, ExecutedRoutedEventArgs e) { CanExecuteRoutedEventArgs canExecuteArgs = new CanExecuteRoutedEventArgs(e.Command, e.Parameter); canExecuteArgs.RoutedEvent = CommandManager.CanExecuteEvent; // Since we don't actually raise this event, we have to explicitly set the source. canExecuteArgs.Source = e.OriginalSource; canExecuteArgs.OverrideSource(e.Source); OnCanExecute(sender, canExecuteArgs); return canExecuteArgs.CanExecute; } ////// Calls Executed or PreviewExecuted based on the event argument's RoutedEvent. /// /// The sender of the event. /// Event arguments. internal void OnExecuted(object sender, ExecutedRoutedEventArgs e) { if (!e.Handled) { if (e.RoutedEvent == CommandManager.ExecutedEvent) { if (Executed != null) { if (CheckCanExecute(sender, e)) { Executed(sender, e); e.Handled = true; } } } else // e.RoutedEvent == CommandManager.PreviewExecutedEvent { if (PreviewExecuted != null) { if (CheckCanExecute(sender, e)) { PreviewExecuted(sender, e); e.Handled = true; } } } } } #endregion #region Data private ICommand _command; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
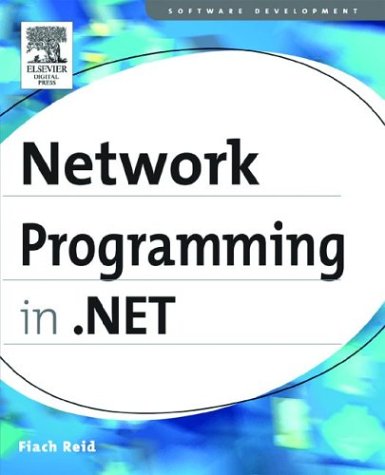
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ISessionStateStore.cs
- ChannelRequirements.cs
- CreateRefExpr.cs
- PeerConnector.cs
- MachineSettingsSection.cs
- HitTestWithGeometryDrawingContextWalker.cs
- AttributeProviderAttribute.cs
- SystemIPInterfaceStatistics.cs
- ObjectDataSourceFilteringEventArgs.cs
- CustomErrorCollection.cs
- VariantWrapper.cs
- QueryCursorEventArgs.cs
- DynamicILGenerator.cs
- PropertyTabChangedEvent.cs
- PropertyValueChangedEvent.cs
- SynchronizationHandlesCodeDomSerializer.cs
- XmlSchemaElement.cs
- MultiSelector.cs
- WebContext.cs
- TextSpanModifier.cs
- HMACSHA384.cs
- ReflectTypeDescriptionProvider.cs
- D3DImage.cs
- SerialPinChanges.cs
- MimePart.cs
- PropertyChangingEventArgs.cs
- DetailsViewRowCollection.cs
- DurationConverter.cs
- DrawListViewSubItemEventArgs.cs
- PreviewPrintController.cs
- ConnectionProviderAttribute.cs
- WorkflowInstanceQuery.cs
- ProcessHostFactoryHelper.cs
- FontWeightConverter.cs
- DSACryptoServiceProvider.cs
- Int32Storage.cs
- ViewLoader.cs
- XmlDataImplementation.cs
- QueryPageSettingsEventArgs.cs
- DataGridViewBindingCompleteEventArgs.cs
- DataSourceUtil.cs
- EmptyControlCollection.cs
- CheckBoxRenderer.cs
- QuerySetOp.cs
- AttributeQuery.cs
- OdbcErrorCollection.cs
- CompilationLock.cs
- GraphicsContainer.cs
- SignatureDescription.cs
- DataProtection.cs
- StateManagedCollection.cs
- BitStack.cs
- UrlParameterWriter.cs
- HybridDictionary.cs
- MultiTrigger.cs
- ToolStripLabel.cs
- TemplatedAdorner.cs
- mediaeventargs.cs
- SoapProtocolImporter.cs
- BinaryNode.cs
- ServiceMetadataBehavior.cs
- QuestionEventArgs.cs
- DependencySource.cs
- EndPoint.cs
- ISAPIWorkerRequest.cs
- SignatureDescription.cs
- LinkedDataMemberFieldEditor.cs
- InfoCardProofToken.cs
- EntityFunctions.cs
- ThreadExceptionEvent.cs
- SqlInternalConnectionSmi.cs
- NameValueConfigurationCollection.cs
- TabControlEvent.cs
- SessionStateItemCollection.cs
- DataBoundLiteralControl.cs
- QilPatternVisitor.cs
- OuterGlowBitmapEffect.cs
- DiscriminatorMap.cs
- ApplicationInfo.cs
- SurrogateSelector.cs
- HttpConfigurationContext.cs
- DataTableTypeConverter.cs
- WindowsGraphics2.cs
- SortQuery.cs
- listviewsubitemcollectioneditor.cs
- FixedSOMPageElement.cs
- RenderDataDrawingContext.cs
- SystemIPv6InterfaceProperties.cs
- Form.cs
- CheckBoxBaseAdapter.cs
- Internal.cs
- LocalizableResourceBuilder.cs
- SpotLight.cs
- COM2ColorConverter.cs
- DataServiceProcessingPipeline.cs
- BindingMemberInfo.cs
- StructuralCache.cs
- TableCellCollection.cs
- coordinatorfactory.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs