Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Cryptography / X509Certificates / safex509handles.cs / 1 / safex509handles.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // SafeX509Handles.cs // namespace System.Security.Cryptography.X509Certificates { using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using Microsoft.Win32.SafeHandles; // Since we need sometimes to delete the key container associated with a cert // context, the handle used in this class is actually a pointer // to a CERT_CTX unmanaged structure defined in COMX509Certificate.h internal sealed class SafeCertContextHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeCertContextHandle() : base (true) {} // 0 is an Invalid Handle internal SafeCertContextHandle(IntPtr handle) : base (true) { SetHandle(handle); } internal static SafeCertContextHandle InvalidHandle { get { return new SafeCertContextHandle(IntPtr.Zero); } } internal IntPtr pCertContext { get { if (handle == IntPtr.Zero) return IntPtr.Zero; return Marshal.ReadIntPtr(handle); } } // This method handles the case where pCert == NULL [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern void _FreePCertContext(IntPtr pCert); override protected bool ReleaseHandle() { _FreePCertContext(handle); return true; } } internal sealed class SafeCertStoreHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeCertStoreHandle() : base (true) {} // 0 is an Invalid Handle internal SafeCertStoreHandle(IntPtr handle) : base (true) { SetHandle(handle); } internal static SafeCertStoreHandle InvalidHandle { get { return new SafeCertStoreHandle(IntPtr.Zero); } } // This method handles the case where hCertStore == NULL [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern void _FreeCertStoreContext(IntPtr hCertStore); override protected bool ReleaseHandle() { _FreeCertStoreContext(handle); return true; } } }
Link Menu
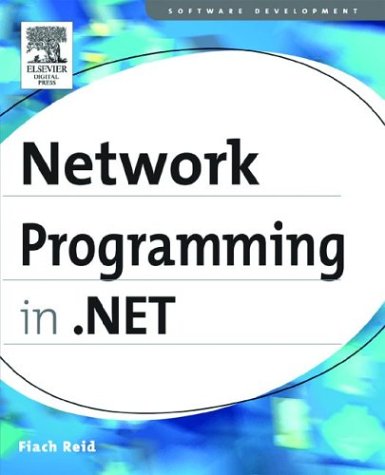
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InvokeMethod.cs
- Version.cs
- JournalEntryStack.cs
- DetailsViewInsertEventArgs.cs
- SubstitutionList.cs
- SiteMapDataSourceView.cs
- AttachmentService.cs
- ExtendedPropertyCollection.cs
- BrowserInteropHelper.cs
- SessionEndingCancelEventArgs.cs
- WebPartDeleteVerb.cs
- ClusterSafeNativeMethods.cs
- UrlPath.cs
- DataServiceQuery.cs
- SiteMapDataSource.cs
- ValueTable.cs
- DataGrid.cs
- XmlTextEncoder.cs
- RulePatternOps.cs
- EntitySetRetriever.cs
- OrderingQueryOperator.cs
- InternalControlCollection.cs
- CheckableControlBaseAdapter.cs
- CngKeyCreationParameters.cs
- FileEnumerator.cs
- PromptStyle.cs
- RtfToXamlReader.cs
- ThicknessKeyFrameCollection.cs
- BindingContext.cs
- List.cs
- UnsafeNativeMethods.cs
- UrlMappingCollection.cs
- QueryCacheKey.cs
- XmlSchemaAnnotated.cs
- MiniParameterInfo.cs
- PropertyMetadata.cs
- CryptographicAttribute.cs
- Substitution.cs
- XPathDocumentIterator.cs
- HttpHandlerActionCollection.cs
- BuilderPropertyEntry.cs
- CookieParameter.cs
- ElapsedEventArgs.cs
- CompositeClientFormatter.cs
- IEnumerable.cs
- TextStore.cs
- RsaSecurityKey.cs
- HttpListener.cs
- NumberFunctions.cs
- x509utils.cs
- SingleStorage.cs
- ContentElement.cs
- XmlSchemaComplexContent.cs
- MsmqTransportSecurity.cs
- RtfToken.cs
- StringReader.cs
- PlanCompiler.cs
- StyleSelector.cs
- SessionSwitchEventArgs.cs
- DataRecordObjectView.cs
- GenericEnumConverter.cs
- EncryptedKeyHashIdentifierClause.cs
- RoleManagerSection.cs
- TextEffect.cs
- DesignerActionUIStateChangeEventArgs.cs
- RowVisual.cs
- DBCommand.cs
- InnerItemCollectionView.cs
- BaseParser.cs
- BitmapCacheBrush.cs
- EventLogEntry.cs
- Mapping.cs
- CompiledELinqQueryState.cs
- AmbientValueAttribute.cs
- DataBindingList.cs
- CompositeControl.cs
- Annotation.cs
- TypeUtil.cs
- SupportingTokenAuthenticatorSpecification.cs
- ThicknessAnimation.cs
- BrushMappingModeValidation.cs
- CodeEventReferenceExpression.cs
- SoapMessage.cs
- PropertyIDSet.cs
- TableParagraph.cs
- GiveFeedbackEvent.cs
- XamlPathDataSerializer.cs
- SemaphoreFullException.cs
- ConfigurationStrings.cs
- ServiceAppDomainAssociationProvider.cs
- ExceptionRoutedEventArgs.cs
- HandlerWithFactory.cs
- PartialTrustHelpers.cs
- WorkflowViewStateService.cs
- SessionEndingEventArgs.cs
- WebExceptionStatus.cs
- ScriptIgnoreAttribute.cs
- XPathMultyIterator.cs
- EncryptedKeyIdentifierClause.cs
- SoapInteropTypes.cs