Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / IntPtr.cs / 1305376 / IntPtr.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: IntPtr ** ** ** Purpose: Platform independent integer ** ** ===========================================================*/ namespace System { using System; using System.Globalization; using System.Runtime; using System.Runtime.Serialization; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Diagnostics.Contracts; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct IntPtr : ISerializable { unsafe private void* m_value; // The compiler treats void* closest to uint hence explicit casts are required to preserve int behavior public static readonly IntPtr Zero; // fast way to compare IntPtr to (IntPtr)0 while IntPtr.Zero doesn't work due to slow statics access [System.Security.SecuritySafeCritical] // auto-generated [Pure] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal unsafe bool IsNull() { return (this.m_value == null); } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe IntPtr(int value) { #if WIN32 m_value = (void *)value; #else m_value = (void *)(long)value; #endif } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe IntPtr(long value) { #if WIN32 m_value = (void *)checked((int)value); #else m_value = (void *)value; #endif } [System.Security.SecurityCritical] // auto-generated [CLSCompliant(false)] [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] public unsafe IntPtr(void* value) { m_value = value; } [System.Security.SecurityCritical] // auto-generated private unsafe IntPtr(SerializationInfo info, StreamingContext context) { long l = info.GetInt64("value"); if (Size==4 && (l>Int32.MaxValue || l() != null); #if WIN32 return ((int)m_value).ToString(format, CultureInfo.InvariantCulture); #else return ((long)m_value).ToString(format, CultureInfo.InvariantCulture); #endif } [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static explicit operator IntPtr (int value) { return new IntPtr(value); } [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static explicit operator IntPtr (long value) { return new IntPtr(value); } [System.Security.SecurityCritical] // auto-generated [CLSCompliant(false), ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static unsafe explicit operator IntPtr (void* value) { return new IntPtr(value); } [System.Security.SecuritySafeCritical] // auto-generated [CLSCompliant(false)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static unsafe explicit operator void* (IntPtr value) { return value.ToPointer(); } [System.Security.SecuritySafeCritical] // auto-generated #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe static explicit operator int (IntPtr value) { #if WIN32 return (int)value.m_value; #else long l = (long)value.m_value; return checked((int)l); #endif } [System.Security.SecuritySafeCritical] // auto-generated #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe static explicit operator long (IntPtr value) { #if WIN32 return (long)(int)value.m_value; #else return (long)value.m_value; #endif } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe static bool operator == (IntPtr value1, IntPtr value2) { return value1.m_value == value2.m_value; } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe static bool operator != (IntPtr value1, IntPtr value2) { return value1.m_value != value2.m_value; } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static IntPtr Add(IntPtr pointer, int offset) { return pointer + offset; } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static IntPtr operator +(IntPtr pointer, int offset) { #if WIN32 return new IntPtr(pointer.ToInt32() + offset); #else return new IntPtr(pointer.ToInt64() + offset); #endif } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static IntPtr Subtract(IntPtr pointer, int offset) { return pointer - offset; } [System.Security.SecuritySafeCritical] // auto-generated [ReliabilityContract(Consistency.MayCorruptInstance, Cer.MayFail)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static IntPtr operator -(IntPtr pointer, int offset) { #if WIN32 return new IntPtr(pointer.ToInt32() - offset); #else return new IntPtr(pointer.ToInt64() - offset); #endif } public static int Size { [Pure] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif get { #if WIN32 return 4; #else return 8; #endif } } [System.Security.SecuritySafeCritical] // auto-generated [CLSCompliant(false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public unsafe void* ToPointer() { return m_value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
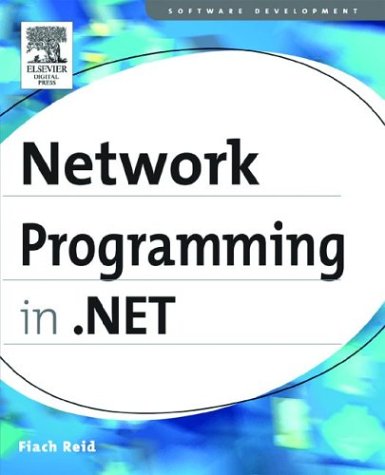
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataArtifactLoaderResource.cs
- WindowsSlider.cs
- CodeValidator.cs
- OneToOneMappingSerializer.cs
- UIElementAutomationPeer.cs
- DataPagerFieldItem.cs
- SecurityToken.cs
- CriticalExceptions.cs
- DLinqColumnProvider.cs
- WebServiceHost.cs
- DictionaryEntry.cs
- ToolStripDropDownItemDesigner.cs
- WebMessageEncodingBindingElement.cs
- FormsAuthentication.cs
- StoragePropertyMapping.cs
- LicenseProviderAttribute.cs
- TripleDESCryptoServiceProvider.cs
- ColorAnimationBase.cs
- NamespaceCollection.cs
- RangeBaseAutomationPeer.cs
- DiagnosticTraceRecords.cs
- TabControl.cs
- LocalClientSecuritySettingsElement.cs
- XsdCachingReader.cs
- HttpApplication.cs
- ColorMatrix.cs
- XmlSchemaElement.cs
- DynamicQueryableWrapper.cs
- LinkedList.cs
- FileCodeGroup.cs
- DataControlButton.cs
- CodeVariableDeclarationStatement.cs
- GradientStopCollection.cs
- HttpContext.cs
- DbParameterHelper.cs
- JavaScriptString.cs
- InteropBitmapSource.cs
- SchemaImporter.cs
- TypefaceMetricsCache.cs
- CodeIdentifiers.cs
- XhtmlBasicLiteralTextAdapter.cs
- OdbcParameter.cs
- HandleExceptionArgs.cs
- InstanceDataCollection.cs
- PointLight.cs
- DataStreamFromComStream.cs
- SchemaTableOptionalColumn.cs
- MouseActionValueSerializer.cs
- DataGrid.cs
- FrameDimension.cs
- PageParserFilter.cs
- WorkflowOperationErrorHandler.cs
- NopReturnReader.cs
- FixedSOMContainer.cs
- AbsoluteQuery.cs
- RegistryKey.cs
- InheritedPropertyChangedEventArgs.cs
- BaseTemplateParser.cs
- FormatSettings.cs
- SQLStringStorage.cs
- AssemblyBuilderData.cs
- SystemIPv4InterfaceProperties.cs
- WindowsButton.cs
- Wildcard.cs
- ResourceContainer.cs
- ContourSegment.cs
- MultilineStringConverter.cs
- Authorization.cs
- MemberInfoSerializationHolder.cs
- FormsIdentity.cs
- NameValueSectionHandler.cs
- RecognizerInfo.cs
- UserControl.cs
- TopClause.cs
- Span.cs
- CodeDomConfigurationHandler.cs
- MultiBinding.cs
- MD5HashHelper.cs
- ConnectionStringSettings.cs
- PasswordDeriveBytes.cs
- ChineseLunisolarCalendar.cs
- TemplatedEditableDesignerRegion.cs
- CompilationUtil.cs
- CreateUserErrorEventArgs.cs
- NestedContainer.cs
- MembershipValidatePasswordEventArgs.cs
- StagingAreaInputItem.cs
- DPCustomTypeDescriptor.cs
- SecureStringHasher.cs
- HitTestWithGeometryDrawingContextWalker.cs
- WindowsFormsHelpers.cs
- ISAPIApplicationHost.cs
- DesignBindingConverter.cs
- BinaryWriter.cs
- PhysicalOps.cs
- PolyLineSegment.cs
- GrammarBuilderWildcard.cs
- BinaryFormatterWriter.cs
- GridViewActionList.cs
- PropertyMetadata.cs