Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Generated / Size.cs / 1305600 / Size.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { [Serializable] [TypeConverter(typeof(SizeConverter))] [ValueSerializer(typeof(SizeValueSerializer))] // Used by MarkupWriter partial struct Size : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Size instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Size instances are exactly equal, false otherwise /// /// The first Size to compare /// The second Size to compare public static bool operator == (Size size1, Size size2) { return size1.Width == size2.Width && size1.Height == size2.Height; } ////// Compares two Size instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Size instances are exactly unequal, false otherwise /// /// The first Size to compare /// The second Size to compare public static bool operator != (Size size1, Size size2) { return !(size1 == size2); } ////// Compares two Size instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Size instances are exactly equal, false otherwise /// /// The first Size to compare /// The second Size to compare public static bool Equals (Size size1, Size size2) { if (size1.IsEmpty) { return size2.IsEmpty; } else { return size1.Width.Equals(size2.Width) && size1.Height.Equals(size2.Height); } } ////// Equals - compares this Size with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Size and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Size)) { return false; } Size value = (Size)o; return Size.Equals(this,value); } ////// Equals - compares this Size with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Size to compare to "this" public bool Equals(Size value) { return Size.Equals(this, value); } ////// Returns the HashCode for this Size /// ////// int - the HashCode for this Size /// public override int GetHashCode() { if (IsEmpty) { return 0; } else { // Perform field-by-field XOR of HashCodes return Width.GetHashCode() ^ Height.GetHashCode(); } } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Size data /// public static Size Parse(string source) { IFormatProvider formatProvider = System.Windows.Markup.TypeConverterHelper.InvariantEnglishUS; TokenizerHelper th = new TokenizerHelper(source, formatProvider); Size value; String firstToken = th.NextTokenRequired(); // The token will already have had whitespace trimmed so we can do a // simple string compare. if (firstToken == "Empty") { value = Empty; } else { value = new Size( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); } // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { if (IsEmpty) { return "Empty"; } // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}", separator, _width, _height); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _width; internal double _height; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { [Serializable] [TypeConverter(typeof(SizeConverter))] [ValueSerializer(typeof(SizeValueSerializer))] // Used by MarkupWriter partial struct Size : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Size instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Size instances are exactly equal, false otherwise /// /// The first Size to compare /// The second Size to compare public static bool operator == (Size size1, Size size2) { return size1.Width == size2.Width && size1.Height == size2.Height; } ////// Compares two Size instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Size instances are exactly unequal, false otherwise /// /// The first Size to compare /// The second Size to compare public static bool operator != (Size size1, Size size2) { return !(size1 == size2); } ////// Compares two Size instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Size instances are exactly equal, false otherwise /// /// The first Size to compare /// The second Size to compare public static bool Equals (Size size1, Size size2) { if (size1.IsEmpty) { return size2.IsEmpty; } else { return size1.Width.Equals(size2.Width) && size1.Height.Equals(size2.Height); } } ////// Equals - compares this Size with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Size and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Size)) { return false; } Size value = (Size)o; return Size.Equals(this,value); } ////// Equals - compares this Size with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Size to compare to "this" public bool Equals(Size value) { return Size.Equals(this, value); } ////// Returns the HashCode for this Size /// ////// int - the HashCode for this Size /// public override int GetHashCode() { if (IsEmpty) { return 0; } else { // Perform field-by-field XOR of HashCodes return Width.GetHashCode() ^ Height.GetHashCode(); } } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Size data /// public static Size Parse(string source) { IFormatProvider formatProvider = System.Windows.Markup.TypeConverterHelper.InvariantEnglishUS; TokenizerHelper th = new TokenizerHelper(source, formatProvider); Size value; String firstToken = th.NextTokenRequired(); // The token will already have had whitespace trimmed so we can do a // simple string compare. if (firstToken == "Empty") { value = Empty; } else { value = new Size( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); } // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { if (IsEmpty) { return "Empty"; } // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}", separator, _width, _height); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _width; internal double _height; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
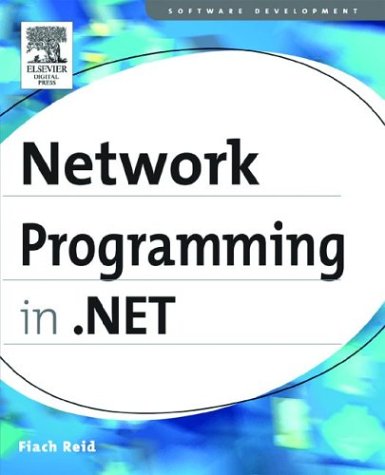
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImagingCache.cs
- Listen.cs
- EditingCommands.cs
- HealthMonitoringSection.cs
- ChildrenQuery.cs
- XmlSchemaAll.cs
- APCustomTypeDescriptor.cs
- GridViewEditEventArgs.cs
- ExtractedStateEntry.cs
- SafeEventHandle.cs
- TextSerializer.cs
- PathParser.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- DispatcherOperation.cs
- WebPartHeaderCloseVerb.cs
- Misc.cs
- ParameterToken.cs
- DeploymentSectionCache.cs
- SaveFileDialog.cs
- MultipartContentParser.cs
- InvalidAsynchronousStateException.cs
- HttpCapabilitiesBase.cs
- AuthenticationConfig.cs
- DesignerCapabilities.cs
- XmlDigitalSignatureProcessor.cs
- FileIOPermission.cs
- ActivationServices.cs
- UserControlCodeDomTreeGenerator.cs
- Timer.cs
- SqlServices.cs
- COM2Enum.cs
- TypeDescriptionProviderAttribute.cs
- ResourceBinder.cs
- XmlWrappingReader.cs
- WmlPageAdapter.cs
- OracleParameterBinding.cs
- BaseCollection.cs
- XmlSchemaExporter.cs
- ExpressionPrefixAttribute.cs
- FrameworkContentElement.cs
- XsdValidatingReader.cs
- CheckBox.cs
- ObjectStateEntryDbDataRecord.cs
- SqlDataSourceCommandParser.cs
- RbTree.cs
- ColumnWidthChangedEvent.cs
- WhiteSpaceTrimStringConverter.cs
- TextRange.cs
- PropertyMap.cs
- Permission.cs
- RegexMatchCollection.cs
- FaultDesigner.cs
- WizardDesigner.cs
- AdRotator.cs
- SqlMethodCallConverter.cs
- TextAdaptor.cs
- StatusBarAutomationPeer.cs
- OperandQuery.cs
- EpmContentDeSerializerBase.cs
- RuntimeConfigLKG.cs
- XamlUtilities.cs
- AudioBase.cs
- SoapProtocolReflector.cs
- SerializationFieldInfo.cs
- NullExtension.cs
- TypeUtil.cs
- BindingList.cs
- ISAPIRuntime.cs
- MethodToken.cs
- Profiler.cs
- PerformanceCountersElement.cs
- TextEditorMouse.cs
- Identity.cs
- RuleDefinitions.cs
- BinaryWriter.cs
- CngAlgorithm.cs
- RawKeyboardInputReport.cs
- Switch.cs
- MimeMapping.cs
- MostlySingletonList.cs
- HostingEnvironmentException.cs
- ActivationArguments.cs
- WindowsComboBox.cs
- DiscriminatorMap.cs
- CustomError.cs
- GridViewCellAutomationPeer.cs
- TrackingStringDictionary.cs
- LinqDataSourceHelper.cs
- EntitySetBase.cs
- XmlILIndex.cs
- GlyphInfoList.cs
- SqlSupersetValidator.cs
- ServiceHandle.cs
- SqlMetaData.cs
- DataBoundControlAdapter.cs
- BasicExpandProvider.cs
- ToolZone.cs
- Msec.cs
- WmlValidationSummaryAdapter.cs
- CodeFieldReferenceExpression.cs