Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / RawKeyboardInputReport.cs / 1305600 / RawKeyboardInputReport.cs
using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32; using System.Windows; namespace System.Windows.Input { ////// The RawKeyboardInputReport class encapsulates the raw input /// provided from a keyboard. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// internal class RawKeyboardInputReport : InputReport { ////// Constructs ad instance of the RawKeyboardInputReport class. /// /// /// The input source that provided this input. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The set of actions being reported. /// /// /// The scan code if a key is being reported. /// /// /// The true if a key is an extended key. /// /// /// The true if a key is a system key. /// /// /// The Win32 virtual key code if a key is being reported. /// /// /// Any extra information being provided along with the input. /// ////// Critical:This handles critical data in the form of PresentationSource and /// ExtraInformation /// TreatAsSafe:The data has demands on the property when someone tries to access it. /// [SecurityCritical,SecurityTreatAsSafe] public RawKeyboardInputReport( PresentationSource inputSource, InputMode mode, int timestamp, RawKeyboardActions actions, int scanCode, bool isExtendedKey, bool isSystemKey, int virtualKey, IntPtr extraInformation) : base(inputSource, InputType.Keyboard, mode, timestamp) { if (!IsValidRawKeyboardActions(actions)) throw new System.ComponentModel.InvalidEnumArgumentException("actions", (int)actions, typeof(RawKeyboardActions)); _actions = actions; _scanCode = scanCode; _isExtendedKey = isExtendedKey; _isSystemKey = isSystemKey; _virtualKey = virtualKey; _extraInformation = new SecurityCriticalData(extraInformation); } /// /// Read-only access to the set of actions that were reported. /// public RawKeyboardActions Actions {get {return _actions;}} ////// Read-only access to the scan code that was reported. /// public int ScanCode {get {return _scanCode;}} ////// Read-only access to the flag of an extended key. /// public bool IsExtendedKey {get {return _isExtendedKey;}} ////// Read-only access to the flag of a system key. /// public bool IsSystemKey {get {return _isSystemKey;}} ////// Read-only access to the virtual key that was reported. /// public int VirtualKey {get {return _virtualKey;}} ////// Read-only access to the extra information was provided along /// with the input. /// ////// Critical: This data was got under an elevation and is not safe to expose /// public IntPtr ExtraInformation { [SecurityCritical] get { return _extraInformation.Value; } } // IsValid Method for RawKeyboardActions. Relies on the enum being flags. internal static bool IsValidRawKeyboardActions(RawKeyboardActions actions) { if (((RawKeyboardActions.AttributesChanged | RawKeyboardActions.Activate | RawKeyboardActions.Deactivate | RawKeyboardActions.KeyDown | RawKeyboardActions.KeyUp) & actions) == actions) { if (!((((RawKeyboardActions.KeyUp | RawKeyboardActions.KeyDown) & actions) == (RawKeyboardActions.KeyUp | RawKeyboardActions.KeyDown)) || ((RawKeyboardActions.Deactivate & actions) == actions && RawKeyboardActions.Deactivate != actions))) { return true; } } return false; } private RawKeyboardActions _actions; private int _scanCode; private bool _isExtendedKey; private bool _isSystemKey; private int _virtualKey; ////// Critical: This information is got under an elevation and can latch onto /// any arbitrary data /// private SecurityCriticalData_extraInformation; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
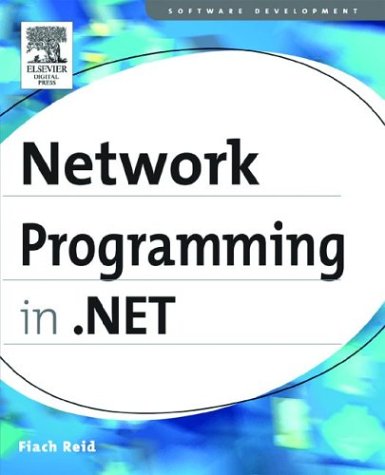
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringKeyFrameCollection.cs
- SectionRecord.cs
- FtpRequestCacheValidator.cs
- ParameterToken.cs
- WebBrowserHelper.cs
- TagPrefixCollection.cs
- AssociationSetEnd.cs
- DataGridViewCellMouseEventArgs.cs
- OleDbPropertySetGuid.cs
- DateTimeConverter.cs
- NamespaceList.cs
- EffectiveValueEntry.cs
- EditorZoneDesigner.cs
- BitmapEncoder.cs
- QilSortKey.cs
- Size3D.cs
- ResourceWriter.cs
- XmlSiteMapProvider.cs
- InstanceLockLostException.cs
- RequiredFieldValidator.cs
- BindableTemplateBuilder.cs
- DataGridViewCellCollection.cs
- XmlSchemaIdentityConstraint.cs
- AssertFilter.cs
- WindowsIPAddress.cs
- HttpApplication.cs
- SharedUtils.cs
- IxmlLineInfo.cs
- EdmItemCollection.OcAssemblyCache.cs
- MissingMemberException.cs
- DataServiceOperationContext.cs
- BindStream.cs
- PartialList.cs
- Effect.cs
- BookmarkInfo.cs
- StreamWriter.cs
- newinstructionaction.cs
- ComAwareEventInfo.cs
- PolicyStatement.cs
- AppDomainFactory.cs
- SqlConnection.cs
- HttpAsyncResult.cs
- _FtpDataStream.cs
- AppDomain.cs
- arclist.cs
- MetadataPropertyAttribute.cs
- PerfProviderCollection.cs
- ComContractElementCollection.cs
- DirectoryNotFoundException.cs
- SpanIndex.cs
- securitymgrsite.cs
- DesignerRegionCollection.cs
- TableParagraph.cs
- DescendantOverDescendantQuery.cs
- NewArrayExpression.cs
- DependencyProperty.cs
- SignatureResourcePool.cs
- Transform.cs
- Label.cs
- RegexWorker.cs
- XmlCharType.cs
- ByteAnimationBase.cs
- Tag.cs
- CellNormalizer.cs
- HighlightComponent.cs
- LazyInitializer.cs
- SmiTypedGetterSetter.cs
- FacetValueContainer.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- MailSettingsSection.cs
- HttpCapabilitiesEvaluator.cs
- WaitForChangedResult.cs
- DataServiceContext.cs
- ColumnTypeConverter.cs
- CssClassPropertyAttribute.cs
- ConfigXmlReader.cs
- HttpConfigurationContext.cs
- ProjectionPlanCompiler.cs
- PrintPreviewControl.cs
- InternalConfigEventArgs.cs
- WmpBitmapDecoder.cs
- RelationshipDetailsCollection.cs
- ReservationNotFoundException.cs
- MSAAWinEventWrap.cs
- BookmarkCallbackWrapper.cs
- DetailsViewDeletedEventArgs.cs
- WebCodeGenerator.cs
- SystemException.cs
- ClientTarget.cs
- ConnectionsZone.cs
- PersonalizationAdministration.cs
- Comparer.cs
- RoutedEventArgs.cs
- WinEventTracker.cs
- XNodeValidator.cs
- SettingsContext.cs
- _ShellExpression.cs
- SqlDesignerDataSourceView.cs
- Aggregates.cs
- DefaultEventAttribute.cs