Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / ComponentModel / DateTimeConverter.cs / 1 / DateTimeConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class DateTimeConverter : TypeConverter { ///Provides a type converter to convert ////// objects to and from various other representations. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to a ////// object using the /// specified context. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = ((string)value).Trim(); if (text.Length == 0) { return DateTime.MinValue; } try { // See if we have a culture info to parse with. If so, then use it. // DateTimeFormatInfo formatInfo = null; if (culture != null ) { formatInfo = (DateTimeFormatInfo)culture.GetFormat(typeof(DateTimeFormatInfo)); } if (formatInfo != null) { return DateTime.Parse(text, formatInfo); } else { return DateTime.Parse(text, culture); } } catch (FormatException e) { throw new FormatException(SR.GetString(SR.ConvertInvalidPrimitive, (string)value, "DateTime"), e); } } return base.ConvertFrom(context, culture, value); } ///Converts the given value object to a ////// object. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && value is DateTime) { DateTime dt = (DateTime) value; if (dt == DateTime.MinValue) { return string.Empty; } if (culture == null) { culture = CultureInfo.CurrentCulture; } DateTimeFormatInfo formatInfo = null; formatInfo = (DateTimeFormatInfo)culture.GetFormat(typeof(DateTimeFormatInfo)); string format; if (culture == CultureInfo.InvariantCulture) { if (dt.TimeOfDay.TotalSeconds == 0) { return dt.ToString("yyyy-MM-dd", culture); } else { return dt.ToString(culture); } } if (dt.TimeOfDay.TotalSeconds == 0) { format = formatInfo.ShortDatePattern; } else { format = formatInfo.ShortDatePattern + " " + formatInfo.ShortTimePattern; } return dt.ToString(format, CultureInfo.CurrentCulture); } if (destinationType == typeof(InstanceDescriptor) && value is DateTime) { DateTime dt = (DateTime)value; if (dt.Ticks == 0) { // Make a special case for the empty DateTime // ConstructorInfo ctr = typeof(DateTime).GetConstructor(new Type[] {typeof(Int64)}); if (ctr != null) { return new InstanceDescriptor(ctr, new object[] { dt.Ticks }); } } ConstructorInfo ctor = typeof(DateTime).GetConstructor(new Type[] { typeof(int), typeof(int), typeof(int), typeof(int), typeof(int), typeof(int), typeof(int)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, dt.Millisecond}); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Converts the given value object to a ////// object /// using the arguments. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class DateTimeConverter : TypeConverter { ///Provides a type converter to convert ////// objects to and from various other representations. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to a ////// object using the /// specified context. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = ((string)value).Trim(); if (text.Length == 0) { return DateTime.MinValue; } try { // See if we have a culture info to parse with. If so, then use it. // DateTimeFormatInfo formatInfo = null; if (culture != null ) { formatInfo = (DateTimeFormatInfo)culture.GetFormat(typeof(DateTimeFormatInfo)); } if (formatInfo != null) { return DateTime.Parse(text, formatInfo); } else { return DateTime.Parse(text, culture); } } catch (FormatException e) { throw new FormatException(SR.GetString(SR.ConvertInvalidPrimitive, (string)value, "DateTime"), e); } } return base.ConvertFrom(context, culture, value); } ///Converts the given value object to a ////// object. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && value is DateTime) { DateTime dt = (DateTime) value; if (dt == DateTime.MinValue) { return string.Empty; } if (culture == null) { culture = CultureInfo.CurrentCulture; } DateTimeFormatInfo formatInfo = null; formatInfo = (DateTimeFormatInfo)culture.GetFormat(typeof(DateTimeFormatInfo)); string format; if (culture == CultureInfo.InvariantCulture) { if (dt.TimeOfDay.TotalSeconds == 0) { return dt.ToString("yyyy-MM-dd", culture); } else { return dt.ToString(culture); } } if (dt.TimeOfDay.TotalSeconds == 0) { format = formatInfo.ShortDatePattern; } else { format = formatInfo.ShortDatePattern + " " + formatInfo.ShortTimePattern; } return dt.ToString(format, CultureInfo.CurrentCulture); } if (destinationType == typeof(InstanceDescriptor) && value is DateTime) { DateTime dt = (DateTime)value; if (dt.Ticks == 0) { // Make a special case for the empty DateTime // ConstructorInfo ctr = typeof(DateTime).GetConstructor(new Type[] {typeof(Int64)}); if (ctr != null) { return new InstanceDescriptor(ctr, new object[] { dt.Ticks }); } } ConstructorInfo ctor = typeof(DateTime).GetConstructor(new Type[] { typeof(int), typeof(int), typeof(int), typeof(int), typeof(int), typeof(int), typeof(int)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, dt.Millisecond}); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Converts the given value object to a ////// object /// using the arguments.
Link Menu
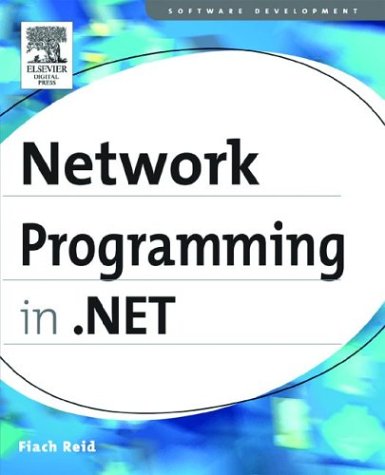
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckBox.cs
- TextComposition.cs
- TreeViewDataItemAutomationPeer.cs
- WebPartConnectionsConfigureVerb.cs
- Rule.cs
- ChannelServices.cs
- DataRow.cs
- ClassicBorderDecorator.cs
- FtpCachePolicyElement.cs
- HtmlForm.cs
- XmlReflectionMember.cs
- DictionarySectionHandler.cs
- MenuScrollingVisibilityConverter.cs
- UnknownWrapper.cs
- ColumnMapTranslator.cs
- StringReader.cs
- shaperfactory.cs
- IPEndPointCollection.cs
- BitmapEffectInputConnector.cs
- ConstructorNeedsTagAttribute.cs
- GridSplitter.cs
- StorageInfo.cs
- FlowDocument.cs
- DataRecordInternal.cs
- SiteMembershipCondition.cs
- EntityDesignerDataSourceView.cs
- AuthenticateEventArgs.cs
- SamlSerializer.cs
- AttributeQuery.cs
- AsymmetricSignatureFormatter.cs
- MetaModel.cs
- RoutingBehavior.cs
- QilStrConcatenator.cs
- CustomPopupPlacement.cs
- ButtonFlatAdapter.cs
- XmlEventCache.cs
- PageWrapper.cs
- StylusPointPropertyId.cs
- DropSource.cs
- __ComObject.cs
- AxImporter.cs
- ComponentDispatcher.cs
- ManipulationLogic.cs
- WeakHashtable.cs
- ValidationResult.cs
- TableLayoutPanelCellPosition.cs
- ManualResetEventSlim.cs
- SQLSingleStorage.cs
- WebZone.cs
- EncoderReplacementFallback.cs
- WebPartCatalogCloseVerb.cs
- IResourceProvider.cs
- XmlMemberMapping.cs
- ObjectViewQueryResultData.cs
- QilXmlReader.cs
- ProcessModelSection.cs
- ObjectQueryExecutionPlan.cs
- ColorConvertedBitmap.cs
- AudioSignalProblemOccurredEventArgs.cs
- MulticastOption.cs
- SingletonConnectionReader.cs
- RowCache.cs
- HelpKeywordAttribute.cs
- RowsCopiedEventArgs.cs
- XmlMessageFormatter.cs
- XmlAtomErrorReader.cs
- MetabaseServerConfig.cs
- NameValueCollection.cs
- CompoundFileReference.cs
- PixelShader.cs
- basecomparevalidator.cs
- TaskHelper.cs
- ListItemCollection.cs
- ContentDisposition.cs
- ClearTypeHintValidation.cs
- VerificationException.cs
- Clock.cs
- XsdDuration.cs
- DebugTraceHelper.cs
- WebServiceParameterData.cs
- AsyncCompletedEventArgs.cs
- Material.cs
- DataBoundControlActionList.cs
- BackgroundFormatInfo.cs
- Timer.cs
- OdbcTransaction.cs
- TextStore.cs
- ClientConfigurationSystem.cs
- StylusPointCollection.cs
- GeometryGroup.cs
- CategoryNameCollection.cs
- XslNumber.cs
- HtmlWindowCollection.cs
- ChannelFactoryBase.cs
- PriorityQueue.cs
- Vertex.cs
- SafeLibraryHandle.cs
- DefaultTextStore.cs
- ObjectListSelectEventArgs.cs
- PrintPreviewGraphics.cs