Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Hosting / AppDomainFactory.cs / 1 / AppDomainFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * AppDomain factory -- creates app domains on demand from ISAPI * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Hosting { using System.Collections; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.Web; using System.Web.Util; // // IAppDomainFactory / AppDomainFactory are obsolete and stay public // only to avoid breaking changes. // // The new code uses IAppManagerAppDomainFactory / AppAppManagerDomainFactory // ///[ComImport, Guid("e6e21054-a7dc-4378-877d-b7f4a2d7e8ba"), System.Runtime.InteropServices.InterfaceTypeAttribute(System.Runtime.InteropServices.ComInterfaceType.InterfaceIsIUnknown)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IAppDomainFactory { #if !FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Interface)] Object Create( [In, MarshalAs(UnmanagedType.BStr)] String module, [In, MarshalAs(UnmanagedType.BStr)] String typeName, [In, MarshalAs(UnmanagedType.BStr)] String appId, [In, MarshalAs(UnmanagedType.BStr)] String appPath, [In, MarshalAs(UnmanagedType.BStr)] String strUrlOfAppOrigin, [In, MarshalAs(UnmanagedType.I4)] int iZone); #else // !FEATURE_PAL [return: MarshalAs(UnmanagedType.Error)] Object Create( [In, MarshalAs(UnmanagedType.Error)] String module, [In, MarshalAs(UnmanagedType.Error)] String typeName, [In, MarshalAs(UnmanagedType.Error)] String appId, [In, MarshalAs(UnmanagedType.Error)] String appPath, [In, MarshalAs(UnmanagedType.Error)] String strUrlOfAppOrigin, [In, MarshalAs(UnmanagedType.I4)] int iZone); #endif // FEATURE_PAL } /// /// ///[To be supplied.] ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class AppDomainFactory : IAppDomainFactory { private AppManagerAppDomainFactory _realFactory; [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.Minimal)] public AppDomainFactory() { _realFactory = new AppManagerAppDomainFactory(); } /* * Creates an app domain with an object inside */ #if !FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Interface)] #endif // !FEATURE_PAL public Object Create(String module, String typeName, String appId, String appPath, String strUrlOfAppOrigin, int iZone) { return _realFactory.Create(appId, appPath); } } // // The new code -- IAppManagerAppDomainFactory / AppAppManagerDomainFactory // /// [ComImport, Guid("02998279-7175-4d59-aa5a-fb8e44d4ca9d"), System.Runtime.InteropServices.InterfaceTypeAttribute(System.Runtime.InteropServices.ComInterfaceType.InterfaceIsIUnknown)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IAppManagerAppDomainFactory { #if !FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Interface)] #else // !FEATURE_PAL Object Create(String appId, String appPath); #endif // !FEATURE_PAL Object Create([In, MarshalAs(UnmanagedType.BStr)] String appId, [In, MarshalAs(UnmanagedType.BStr)] String appPath); void Stop(); } /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class AppManagerAppDomainFactory : IAppManagerAppDomainFactory { private ApplicationManager _appManager; [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.Minimal)] public AppManagerAppDomainFactory() { _appManager = ApplicationManager.GetApplicationManager(); _appManager.Open(); } /* * Creates an app domain with an object inside */ #if FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Error)] #else // FEATURE_PAL [return: MarshalAs(UnmanagedType.Interface)] #endif // FEATURE_PAL public Object Create(String appId, String appPath) { try { // // Fill app a Dictionary with 'binding rules' -- name value string pairs // for app domain creation // // if (appPath[0] == '.') { System.IO.FileInfo file = new System.IO.FileInfo(appPath); appPath = file.FullName; } if (!StringUtil.StringEndsWith(appPath, '\\')) { appPath = appPath + "\\"; } // Create new app domain via App Manager #if FEATURE_PAL // FEATURE_PAL does not enable IIS-based hosting features throw new NotImplementedException("ROTORTODO"); #else // FEATURE_PAL ISAPIApplicationHost appHost = new ISAPIApplicationHost(appId, appPath, false /*validatePhysicalPath*/); ISAPIRuntime isapiRuntime = (ISAPIRuntime)_appManager.CreateObjectInternal(appId, typeof(ISAPIRuntime), appHost, false /*failIfExists*/, null /*hostingParameters*/); // store in the worker app domain the exact appId that is the key to unmananaged table isapiRuntime.SetThisAppDomainsIsapiAppId(appId); isapiRuntime.StartProcessing(); return new ObjectHandle(isapiRuntime); #endif // FEATURE_PAL } catch (Exception e) { Debug.Trace("internal", "AppDomainFactory::Create failed with " + e.GetType().FullName + ": " + e.Message + "\r\n" + e.StackTrace); throw; } } public void Stop() { // wait for all app domains to go away _appManager.Close(); } internal static String ConstructSimpleAppName(string virtPath) { if (virtPath.Length <= 1) // root? return "root"; else return virtPath.Substring(1).ToLower(CultureInfo.InvariantCulture).Replace('/', '_'); } } }
Link Menu
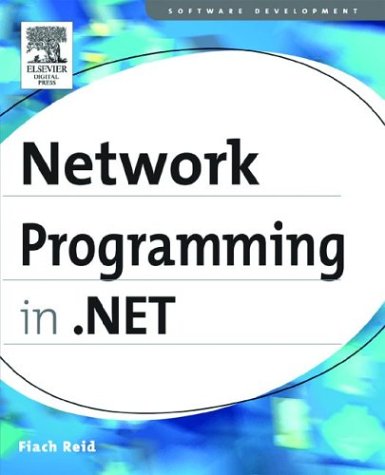
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SourceInterpreter.cs
- ConnectionManagementElement.cs
- Button.cs
- InstallerTypeAttribute.cs
- ObjectConverter.cs
- OleDbRowUpdatingEvent.cs
- XmlTextReaderImplHelpers.cs
- AuditLogLocation.cs
- LazyTextWriterCreator.cs
- oledbmetadatacolumnnames.cs
- ListSortDescriptionCollection.cs
- PerspectiveCamera.cs
- ProcessHostServerConfig.cs
- RegexCode.cs
- CornerRadiusConverter.cs
- DetailsViewInsertEventArgs.cs
- WebDisplayNameAttribute.cs
- XmlUnspecifiedAttribute.cs
- CodeSubDirectoriesCollection.cs
- MultipleViewProviderWrapper.cs
- DropShadowBitmapEffect.cs
- InputLangChangeEvent.cs
- DelegatedStream.cs
- TextRangeSerialization.cs
- RequestSecurityTokenSerializer.cs
- SQLBytes.cs
- Label.cs
- CorrelationExtension.cs
- ImageConverter.cs
- CharAnimationUsingKeyFrames.cs
- InvokeProviderWrapper.cs
- AbandonedMutexException.cs
- GeneratedView.cs
- ClusterSafeNativeMethods.cs
- ReadOnlyKeyedCollection.cs
- SmiEventSink_Default.cs
- VisualTransition.cs
- ProfileGroupSettingsCollection.cs
- ChannelManagerBase.cs
- UdpReplyToBehavior.cs
- PersistenceProviderElement.cs
- DataSetMappper.cs
- HierarchicalDataBoundControlAdapter.cs
- RoutedEventArgs.cs
- Vertex.cs
- XmlSchemaSubstitutionGroup.cs
- RightsManagementInformation.cs
- XPathDocumentNavigator.cs
- PropertyRecord.cs
- AuthenticationSection.cs
- Converter.cs
- SiteMapNodeItem.cs
- DayRenderEvent.cs
- OleDbTransaction.cs
- PenLineJoinValidation.cs
- SettingsProperty.cs
- Interlocked.cs
- XsltContext.cs
- ExecutionContext.cs
- PagesSection.cs
- ReferenceEqualityComparer.cs
- ButtonRenderer.cs
- DispatchChannelSink.cs
- InkSerializer.cs
- documentation.cs
- ValidatingReaderNodeData.cs
- TransformCollection.cs
- XmlToDatasetMap.cs
- RightsManagementInformation.cs
- BindingContext.cs
- DescriptionAttribute.cs
- CurrentTimeZone.cs
- EqualityComparer.cs
- ReflectPropertyDescriptor.cs
- HMACSHA256.cs
- SafeProcessHandle.cs
- ListItemConverter.cs
- ActivityBindForm.cs
- PlanCompilerUtil.cs
- DefaultBindingPropertyAttribute.cs
- ADMembershipUser.cs
- ErrorFormatterPage.cs
- GeometryGroup.cs
- AlternateView.cs
- UriParserTemplates.cs
- EtwTrace.cs
- XsltSettings.cs
- SelectorAutomationPeer.cs
- TabControlAutomationPeer.cs
- NameObjectCollectionBase.cs
- MultipartIdentifier.cs
- RtType.cs
- CalculatedColumn.cs
- EntityContainerEntitySetDefiningQuery.cs
- TripleDES.cs
- KeyMatchBuilder.cs
- TileModeValidation.cs
- DataList.cs
- CatalogZoneAutoFormat.cs
- RestHandlerFactory.cs