Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Effects / Generated / DropShadowBitmapEffect.cs / 1 / DropShadowBitmapEffect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class DropShadowBitmapEffect : BitmapEffect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DropShadowBitmapEffect Clone() { return (DropShadowBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DropShadowBitmapEffect CloneCurrentValue() { return (DropShadowBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ShadowDepthPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(ShadowDepthProperty); } private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(ColorProperty); } private static void DirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(DirectionProperty); } private static void NoisePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(NoiseProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(OpacityProperty); } private static void SoftnessPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(SoftnessProperty); } #region Public Properties ////// ShadowDepth - double. Default value is 5.0. /// public double ShadowDepth { get { return (double) GetValue(ShadowDepthProperty); } set { SetValueInternal(ShadowDepthProperty, value); } } ////// Color - Color. Default value is Colors.Black. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// Direction - double. Default value is 315.0. /// public double Direction { get { return (double) GetValue(DirectionProperty); } set { SetValueInternal(DirectionProperty, value); } } ////// Noise - double. Default value is 0.0. /// public double Noise { get { return (double) GetValue(NoiseProperty); } set { SetValueInternal(NoiseProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } ////// Softness - double. Default value is 0.5. /// public double Softness { get { return (double) GetValue(SoftnessProperty); } set { SetValueInternal(SoftnessProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DropShadowBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DropShadowBitmapEffect.ShadowDepth property. /// public static readonly DependencyProperty ShadowDepthProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Color property. /// public static readonly DependencyProperty ColorProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Direction property. /// public static readonly DependencyProperty DirectionProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Noise property. /// public static readonly DependencyProperty NoiseProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Softness property. /// public static readonly DependencyProperty SoftnessProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_ShadowDepth = 5.0; internal static Color s_Color = Colors.Black; internal const double c_Direction = 315.0; internal const double c_Noise = 0.0; internal const double c_Opacity = 1.0; internal const double c_Softness = 0.5; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static DropShadowBitmapEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(DropShadowBitmapEffect); ShadowDepthProperty = RegisterProperty("ShadowDepth", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(ShadowDepthPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.Black, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); DirectionProperty = RegisterProperty("Direction", typeof(double), typeofThis, 315.0, new PropertyChangedCallback(DirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); NoiseProperty = RegisterProperty("Noise", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(NoisePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); SoftnessProperty = RegisterProperty("Softness", typeof(double), typeofThis, 0.5, new PropertyChangedCallback(SoftnessPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class DropShadowBitmapEffect : BitmapEffect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DropShadowBitmapEffect Clone() { return (DropShadowBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DropShadowBitmapEffect CloneCurrentValue() { return (DropShadowBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ShadowDepthPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(ShadowDepthProperty); } private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(ColorProperty); } private static void DirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(DirectionProperty); } private static void NoisePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(NoiseProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(OpacityProperty); } private static void SoftnessPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowBitmapEffect target = ((DropShadowBitmapEffect) d); target.PropertyChanged(SoftnessProperty); } #region Public Properties ////// ShadowDepth - double. Default value is 5.0. /// public double ShadowDepth { get { return (double) GetValue(ShadowDepthProperty); } set { SetValueInternal(ShadowDepthProperty, value); } } ////// Color - Color. Default value is Colors.Black. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// Direction - double. Default value is 315.0. /// public double Direction { get { return (double) GetValue(DirectionProperty); } set { SetValueInternal(DirectionProperty, value); } } ////// Noise - double. Default value is 0.0. /// public double Noise { get { return (double) GetValue(NoiseProperty); } set { SetValueInternal(NoiseProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } ////// Softness - double. Default value is 0.5. /// public double Softness { get { return (double) GetValue(SoftnessProperty); } set { SetValueInternal(SoftnessProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DropShadowBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DropShadowBitmapEffect.ShadowDepth property. /// public static readonly DependencyProperty ShadowDepthProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Color property. /// public static readonly DependencyProperty ColorProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Direction property. /// public static readonly DependencyProperty DirectionProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Noise property. /// public static readonly DependencyProperty NoiseProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty; ////// The DependencyProperty for the DropShadowBitmapEffect.Softness property. /// public static readonly DependencyProperty SoftnessProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_ShadowDepth = 5.0; internal static Color s_Color = Colors.Black; internal const double c_Direction = 315.0; internal const double c_Noise = 0.0; internal const double c_Opacity = 1.0; internal const double c_Softness = 0.5; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static DropShadowBitmapEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(DropShadowBitmapEffect); ShadowDepthProperty = RegisterProperty("ShadowDepth", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(ShadowDepthPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.Black, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); DirectionProperty = RegisterProperty("Direction", typeof(double), typeofThis, 315.0, new PropertyChangedCallback(DirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); NoiseProperty = RegisterProperty("Noise", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(NoisePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); SoftnessProperty = RegisterProperty("Softness", typeof(double), typeofThis, 0.5, new PropertyChangedCallback(SoftnessPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
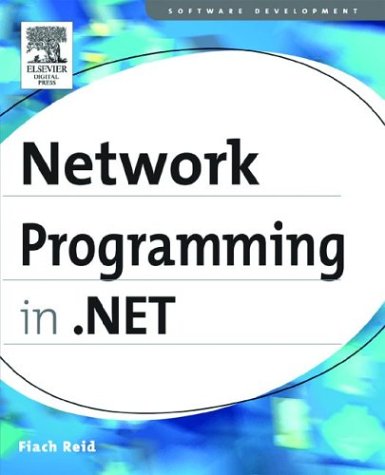
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakEventTable.cs
- TransformPattern.cs
- WindowVisualStateTracker.cs
- WmpBitmapDecoder.cs
- XmlTextWriter.cs
- XmlComplianceUtil.cs
- ObjectSelectorEditor.cs
- Win32.cs
- SQLInt16Storage.cs
- MenuItem.cs
- SafeEventLogReadHandle.cs
- SystemWebSectionGroup.cs
- SQLRoleProvider.cs
- InfoCardArgumentException.cs
- XmlUrlResolver.cs
- FormDocumentDesigner.cs
- RequiredArgumentAttribute.cs
- FormViewUpdatedEventArgs.cs
- HMACSHA384.cs
- XsdValidatingReader.cs
- Bits.cs
- XmlSchema.cs
- MtomMessageEncodingElement.cs
- SortExpressionBuilder.cs
- HMACSHA1.cs
- DbParameterHelper.cs
- LineProperties.cs
- AutoCompleteStringCollection.cs
- WebPartExportVerb.cs
- DiscoveryRequestHandler.cs
- HttpModule.cs
- ToolStripHighContrastRenderer.cs
- Color.cs
- ConnectionDemuxer.cs
- EntityDataSourceContextCreatingEventArgs.cs
- PointAnimationClockResource.cs
- ReflectionPermission.cs
- XmlSiteMapProvider.cs
- GroupAggregateExpr.cs
- MessageSmuggler.cs
- XmlWriterTraceListener.cs
- KeyValueInternalCollection.cs
- SynchronousReceiveElement.cs
- Schedule.cs
- TriggerBase.cs
- SortFieldComparer.cs
- CheckBoxFlatAdapter.cs
- ToolStripComboBox.cs
- DataGridCellItemAutomationPeer.cs
- KeyTime.cs
- ClientSettingsSection.cs
- LicenseContext.cs
- BitVec.cs
- SyndicationSerializer.cs
- MessageHeader.cs
- SoapMessage.cs
- ValidationErrorCollection.cs
- ScrollBar.cs
- designeractionbehavior.cs
- MediaContext.cs
- CaseInsensitiveOrdinalStringComparer.cs
- SourceLineInfo.cs
- xsdvalidator.cs
- SqlDataSourceQueryEditor.cs
- RecognitionResult.cs
- ResourceProperty.cs
- MasterPageCodeDomTreeGenerator.cs
- XmlSchemaAll.cs
- EmbeddedMailObjectsCollection.cs
- FunctionDetailsReader.cs
- Parser.cs
- IDReferencePropertyAttribute.cs
- ObjRef.cs
- ViewGenerator.cs
- MeasureData.cs
- HttpChannelBindingToken.cs
- AuthenticatedStream.cs
- ToolStripDropDownItemDesigner.cs
- WebBaseEventKeyComparer.cs
- OnOperation.cs
- VideoDrawing.cs
- DBCSCodePageEncoding.cs
- COM2TypeInfoProcessor.cs
- CommandValueSerializer.cs
- elementinformation.cs
- SqlFormatter.cs
- TextRangeEditTables.cs
- CacheEntry.cs
- WebPartVerbCollection.cs
- ActionItem.cs
- FormsAuthenticationModule.cs
- Vector.cs
- WebPartActionVerb.cs
- KeyConstraint.cs
- ExpressionBindingsDialog.cs
- ControlEvent.cs
- ToolStripRendererSwitcher.cs
- activationcontext.cs
- TreeViewImageIndexConverter.cs
- fixedPageContentExtractor.cs