Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / Paragraph.cs / 1305600 / Paragraph.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Paragraph element. // // History: // 05/05/2003 : [....] - created. // 10/28/2004 : [....] - ContentElements refactoring. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter, DesignerSerializationVisibility using System.Windows.Markup; // ContentProperty using MS.Internal; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Paragraph element /// [ContentProperty("Inlines")] public class Paragraph : Block { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Static ctor. Initializes property metadata. /// static Paragraph() { DefaultStyleKeyProperty.OverrideMetadata(typeof(Paragraph), new FrameworkPropertyMetadata(typeof(Paragraph))); } ////// Public constructor. /// public Paragraph() : base() { } ////// Paragraph constructor. /// public Paragraph(Inline inline) : base() { if (inline == null) { throw new ArgumentNullException("inline"); } this.Inlines.Add(inline); } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Collection of Inline items contained in this Paragraph. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public InlineCollection Inlines { get { return new InlineCollection(this, /*isOwnerParent*/true); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = Inline.TextDecorationsProperty.AddOwner( typeof(Paragraph), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextIndentProperty = DependencyProperty.Register( "TextIndent", typeof(double), typeof(Paragraph), new FrameworkPropertyMetadata( 0.0, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(IsValidTextIndent)); ///property. /// /// The TextIndent property specifies the indentation of the first line of a paragraph. /// [TypeConverter(typeof(LengthConverter))] public double TextIndent { get { return (double)GetValue(TextIndentProperty); } set { SetValue(TextIndentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty MinOrphanLinesProperty = DependencyProperty.Register( "MinOrphanLines", typeof(int), typeof(Paragraph), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidMinOrphanLines)); ///property. /// /// The MinOrphanLines is the minimum number of lines that /// can be left behind when a paragraph is broken on a page break /// or column break. /// public int MinOrphanLines { get { return (int)GetValue(MinOrphanLinesProperty); } set { SetValue(MinOrphanLinesProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty MinWidowLinesProperty = DependencyProperty.Register( "MinWidowLines", typeof(int), typeof(Paragraph), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidMinWidowLines)); ///property. /// /// The MinWidowLines is the minimum number of lines after a break /// to be put on the next page or column. /// public int MinWidowLines { get { return (int)GetValue(MinWidowLinesProperty); } set { SetValue(MinWidowLinesProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty KeepWithNextProperty = DependencyProperty.Register( "KeepWithNext", typeof(bool), typeof(Paragraph), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ///property. /// /// The KeepWithNext property indicates that this paragraph should be kept with /// the next paragraph in the track. (This also implies that the paragraph itself /// will not be broken.) /// public bool KeepWithNext { get { return (bool)GetValue(KeepWithNextProperty); } set { SetValue(KeepWithNextProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty KeepTogetherProperty = DependencyProperty.Register( "KeepTogether", typeof(bool), typeof(Paragraph), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ///property. /// /// The KeepTogether property indicates that all the text in the paragraph /// should be kept together. /// public bool KeepTogether { get { return (bool)GetValue(KeepTogetherProperty); } set { SetValue(KeepTogetherProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal void GetDefaultMarginValue(ref Thickness margin) { double lineHeight = this.LineHeight; if (IsLineHeightAuto(lineHeight)) { lineHeight = this.FontFamily.LineSpacing * this.FontSize; } margin = new Thickness(0, lineHeight, 0, lineHeight); } internal static bool IsMarginAuto(Thickness margin) { return (Double.IsNaN(margin.Left) && Double.IsNaN(margin.Right) && Double.IsNaN(margin.Top) && Double.IsNaN(margin.Bottom)); } internal static bool IsLineHeightAuto(double lineHeight) { return (Double.IsNaN(lineHeight)); } // Returns true if there is no text/embedded element content in passed paragraph, // it may have zero or more inline formatting tags with no text. internal static bool HasNoTextContent(Paragraph paragraph) { ITextPointer navigator = paragraph.ContentStart.CreatePointer(); ITextPointer end = paragraph.ContentEnd; while (navigator.CompareTo(end) < 0) { TextPointerContext symbolType = navigator.GetPointerContext(LogicalDirection.Forward); if (symbolType == TextPointerContext.Text || symbolType == TextPointerContext.EmbeddedElement || typeof(LineBreak).IsAssignableFrom(navigator.ParentType) || typeof(AnchoredBlock).IsAssignableFrom(navigator.ParentType)) { return false; } navigator.MoveToNextContextPosition(LogicalDirection.Forward); } return true; } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeInlines(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidMinOrphanLines(object o) { int value = (int)o; const int maxLines = PTS.Restrictions.tscLineInParaRestriction; return (value >= 0 && value <= maxLines); } private static bool IsValidMinWidowLines(object o) { int value = (int)o; const int maxLines = PTS.Restrictions.tscLineInParaRestriction; return (value >= 0 && value <= maxLines); } private static bool IsValidTextIndent(object o) { double indent = (double)o; double maxIndent = Math.Min(1000000, PTS.MaxPageSize); double minIndent = -maxIndent; if (Double.IsNaN(indent)) { return false; } if (indent < minIndent || indent > maxIndent) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
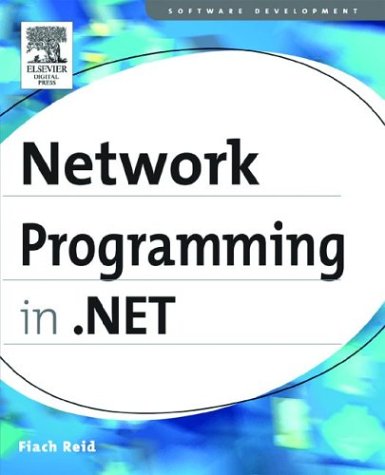
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebBrowserBase.cs
- EntityDataSourceSelectedEventArgs.cs
- ElementMarkupObject.cs
- TreeViewHitTestInfo.cs
- DbConvert.cs
- BaseTemplateCodeDomTreeGenerator.cs
- CompressedStack.cs
- returneventsaver.cs
- DependencyPropertyHelper.cs
- MethodResolver.cs
- OdbcDataReader.cs
- StreamReader.cs
- Matrix3D.cs
- EventMappingSettingsCollection.cs
- ClrPerspective.cs
- Attributes.cs
- SmiContext.cs
- CodeTypeDelegate.cs
- SocketException.cs
- Permission.cs
- UpdatePanelTrigger.cs
- AppSecurityManager.cs
- TraceRecord.cs
- TabControlCancelEvent.cs
- NativeMethods.cs
- SrgsElementFactory.cs
- CryptoKeySecurity.cs
- IntegerFacetDescriptionElement.cs
- Graph.cs
- StatusBarAutomationPeer.cs
- QilReference.cs
- ProcessHostServerConfig.cs
- MsdtcClusterUtils.cs
- XmlParserContext.cs
- WeakHashtable.cs
- DataGridTable.cs
- QueryableFilterUserControl.cs
- XmlILTrace.cs
- CapiHashAlgorithm.cs
- CodeTypeConstructor.cs
- ViewStateModeByIdAttribute.cs
- DataGridCommandEventArgs.cs
- ManagementBaseObject.cs
- SessionEndingEventArgs.cs
- SoapRpcMethodAttribute.cs
- Timeline.cs
- SymLanguageType.cs
- Token.cs
- CultureTable.cs
- EntryWrittenEventArgs.cs
- HighlightComponent.cs
- SqlNodeAnnotation.cs
- WpfPayload.cs
- Visual.cs
- ParserStack.cs
- ByteConverter.cs
- ItemDragEvent.cs
- DesignTimeVisibleAttribute.cs
- TransactionFilter.cs
- DetailsViewDesigner.cs
- shaperfactoryquerycachekey.cs
- diagnosticsswitches.cs
- BooleanFunctions.cs
- Ray3DHitTestResult.cs
- ToolStripPanelRenderEventArgs.cs
- ProxyAttribute.cs
- DataListItemEventArgs.cs
- X509Certificate.cs
- SmiEventStream.cs
- relpropertyhelper.cs
- _FtpDataStream.cs
- NodeLabelEditEvent.cs
- HttpServerVarsCollection.cs
- LocalizationCodeDomSerializer.cs
- EncodedStreamFactory.cs
- OleDbCommand.cs
- DataDocumentXPathNavigator.cs
- DomNameTable.cs
- XmlSchemaCollection.cs
- _AcceptOverlappedAsyncResult.cs
- DSASignatureDeformatter.cs
- IgnorePropertiesAttribute.cs
- Image.cs
- RoutedEvent.cs
- GetBrowserTokenRequest.cs
- BooleanProjectedSlot.cs
- DefaultValidator.cs
- DynamicMethod.cs
- UniqueID.cs
- DataControlCommands.cs
- ScriptModule.cs
- TextOnlyOutput.cs
- CatalogPartCollection.cs
- Rect3DConverter.cs
- WebPartEditVerb.cs
- ThicknessConverter.cs
- NamedPipeHostedTransportConfiguration.cs
- SessionSwitchEventArgs.cs
- StorageModelBuildProvider.cs
- Size3DConverter.cs