Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / _FtpDataStream.cs / 1 / _FtpDataStream.cs
// ------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // ----------------------------------------------------------------------------- // namespace System.Net { using System.IO; using System.Net.Sockets; using System.Security.Permissions; ////// internal class FtpDataStream : Stream, ICloseEx { private FtpWebRequest m_Request; private NetworkStream m_NetworkStream; private bool m_Writeable; private bool m_Readable; private bool m_IsFullyRead = false; bool m_Closing = false; internal FtpDataStream(NetworkStream networkStream, FtpWebRequest request, TriState writeOnly) { GlobalLog.Print("FtpDataStream#" + ValidationHelper.HashString(this) + "::FtpDataStream"); m_Readable = true; m_Writeable = true; if (writeOnly == TriState.True) { m_Readable = false; } else if (writeOnly == TriState.False) { m_Writeable = false; } m_NetworkStream = networkStream; m_Request = request; } protected override void Dispose(bool disposing) { try { if (disposing) ((ICloseEx)this).CloseEx(CloseExState.Normal); else ((ICloseEx)this).CloseEx(CloseExState.Abort | CloseExState.Silent); } finally { base.Dispose(disposing); } } void ICloseEx.CloseEx(CloseExState closeState) { GlobalLog.Print("FtpDataStream#" + ValidationHelper.HashString(this) + "::CloseEx, state = " + closeState.ToString()); lock (this) { if (m_Closing == true) return; m_Closing = true; m_Writeable = false; m_Readable = false; } try { try { if ((closeState & CloseExState.Abort) == 0) m_NetworkStream.Close(Socket.DefaultCloseTimeout); else m_NetworkStream.Close(0); } finally { m_Request.DataStreamClosed(closeState); } } catch (Exception exception) { bool doThrow = true; WebException webException = exception as WebException; if (webException != null) { FtpWebResponse response = webException.Response as FtpWebResponse; if (response != null) { if (!m_IsFullyRead && response.StatusCode == FtpStatusCode.ConnectionClosed) doThrow = false; } } if (doThrow) if ((closeState & CloseExState.Silent) == 0) throw; } } ////// The FtpDataStream class implements a data FTP connection, /// ////// private void CheckError() { if (m_Request.Aborted) { throw new WebException( NetRes.GetWebStatusString( "net_requestaborted", WebExceptionStatus.RequestCanceled), WebExceptionStatus.RequestCanceled); } } ///Rethrows the exception ////// public override bool CanRead { get { return m_Readable; } } ///Indicates that data can be read from the stream. /// /// public override bool CanSeek { get { return m_NetworkStream.CanSeek; } } ///Indicates that the stream is seekable ////// public override bool CanWrite { get { return m_Writeable; } } ///Indicates that the stream is writeable ////// public override long Length { get { return m_NetworkStream.Length; } } ///Indicates that the stream is writeable ////// public override long Position { get { return m_NetworkStream.Position; } set { m_NetworkStream.Position = value; } } ///Gets or sets the position in the stream. Always throws ///. /// public override long Seek(long offset, SeekOrigin origin) { CheckError(); try { return m_NetworkStream.Seek(offset, origin); } catch { CheckError(); throw; } } ///Seeks a specific position in the stream. ////// public override int Read(byte[] buffer, int offset, int size) { CheckError(); int readBytes; try { readBytes = m_NetworkStream.Read(buffer, offset, size); } catch { CheckError(); throw; } if (readBytes == 0) { m_IsFullyRead = true; Close(); } return readBytes; } ///Reads data from the stream. ////// public override void Write(byte[] buffer, int offset, int size) { CheckError(); try { m_NetworkStream.Write(buffer, offset, size); } catch { CheckError(); throw; } } private void AsyncReadCallback(IAsyncResult ar) { LazyAsyncResult userResult = (LazyAsyncResult) ar.AsyncState; try { try { int readBytes = m_NetworkStream.EndRead(ar); if (readBytes == 0) { m_IsFullyRead = true; Close(); // This should block for pipeline completion } userResult.InvokeCallback(readBytes); } catch (Exception exception) { // Complete with error. If already completed rethrow on the worker thread if (!userResult.IsCompleted) userResult.InvokeCallback(exception); } } catch {} } ///Writes data to the stream. ////// [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] buffer, int offset, int size, AsyncCallback callback, Object state) { CheckError(); LazyAsyncResult userResult = new LazyAsyncResult(this, state, callback); try { m_NetworkStream.BeginRead(buffer, offset, size, new AsyncCallback(AsyncReadCallback), userResult); } catch { CheckError(); throw; } return userResult; } ////// Begins an asychronous read from a stream. /// ////// public override int EndRead(IAsyncResult ar) { try { object result = ((LazyAsyncResult)ar).InternalWaitForCompletion(); if (result is Exception) throw (Exception) result; return (int)result; } finally { CheckError(); } } ////// Handle the end of an asynchronous read. /// ////// [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] buffer, int offset, int size, AsyncCallback callback, Object state) { CheckError(); try { return m_NetworkStream.BeginWrite(buffer, offset, size, callback, state); } catch { CheckError(); throw; } } ////// Begins an asynchronous write to a stream. /// ////// public override void EndWrite(IAsyncResult asyncResult) { try { m_NetworkStream.EndWrite(asyncResult); } finally { CheckError(); } } ////// Handle the end of an asynchronous write. /// ////// public override void Flush() { m_NetworkStream.Flush(); } ////// Flushes data from the stream. /// ////// public override void SetLength(long value) { m_NetworkStream.SetLength(value); } ////// Sets the length of the stream. Always throws ////// . /// /// public override bool CanTimeout { get { return m_NetworkStream.CanTimeout; } } ///Indicates whether we can timeout ////// public override int ReadTimeout { get { return m_NetworkStream.ReadTimeout; } set { m_NetworkStream.ReadTimeout = value; } } ///Set/Get ReadTimeout ////// public override int WriteTimeout { get { return m_NetworkStream.WriteTimeout; } set { m_NetworkStream.WriteTimeout = value; } } internal void SetSocketTimeoutOption(SocketShutdown mode, int timeout, bool silent) { m_NetworkStream.SetSocketTimeoutOption(mode, timeout, silent); } } }Set/Get WriteTimeout ///
Link Menu
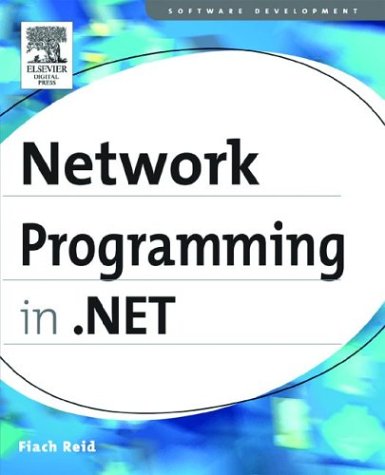
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripItemCollection.cs
- QuaternionKeyFrameCollection.cs
- EditingCoordinator.cs
- SafeLibraryHandle.cs
- DataGridCommandEventArgs.cs
- Line.cs
- CompressedStack.cs
- WebConfigurationManager.cs
- XmlSchemaCollection.cs
- Point3D.cs
- NumericExpr.cs
- CookieParameter.cs
- DateTimeFormat.cs
- PauseStoryboard.cs
- ForEachAction.cs
- BindingOperations.cs
- ComIntegrationManifestGenerator.cs
- FlowLayoutSettings.cs
- ObjectDataSourceDisposingEventArgs.cs
- HtmlEmptyTagControlBuilder.cs
- MachineSettingsSection.cs
- ToolStripItemImageRenderEventArgs.cs
- Vector.cs
- KeyConverter.cs
- PersonalizationStateInfoCollection.cs
- XPathNode.cs
- HTTPAPI_VERSION.cs
- InvokeMethodActivity.cs
- ObservableCollection.cs
- NativeMethods.cs
- Column.cs
- InfoCardKeyedHashAlgorithm.cs
- FactoryGenerator.cs
- SqlFunctionAttribute.cs
- VideoDrawing.cs
- QilPatternFactory.cs
- NonParentingControl.cs
- StrongNameIdentityPermission.cs
- DataGridCellInfo.cs
- NameValuePermission.cs
- MenuItemCollection.cs
- PersonalizationProviderCollection.cs
- QuadTree.cs
- DescriptionCreator.cs
- ImmutableDispatchRuntime.cs
- ExceptionHandlersDesigner.cs
- SystemKeyConverter.cs
- RegexStringValidatorAttribute.cs
- SspiWrapper.cs
- OutputCacheEntry.cs
- BinaryMethodMessage.cs
- MSHTMLHost.cs
- HtmlGenericControl.cs
- DockEditor.cs
- LiteralSubsegment.cs
- PropertyChangeTracker.cs
- TokenDescriptor.cs
- WS2007FederationHttpBindingCollectionElement.cs
- BooleanConverter.cs
- HttpServerVarsCollection.cs
- User.cs
- TypeTypeConverter.cs
- DataTemplateSelector.cs
- SignedXml.cs
- DataReceivedEventArgs.cs
- StorageComplexTypeMapping.cs
- DataSourceGroupCollection.cs
- RequestDescription.cs
- TextEffectResolver.cs
- DetailsViewRowCollection.cs
- QueryLifecycle.cs
- SafeCryptContextHandle.cs
- DebugView.cs
- securitymgrsite.cs
- COAUTHIDENTITY.cs
- AsyncOperationManager.cs
- RegistrationServices.cs
- DataControlReference.cs
- SvcMapFile.cs
- ToolboxBitmapAttribute.cs
- DataObjectFieldAttribute.cs
- DbTransaction.cs
- FactoryGenerator.cs
- CommandLibraryHelper.cs
- ClonableStack.cs
- TraceSwitch.cs
- ProxyGenerationError.cs
- ListParagraph.cs
- ThreadExceptionDialog.cs
- RegexCharClass.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- BaseValidator.cs
- AnimationLayer.cs
- CellPartitioner.cs
- LinkArea.cs
- SqlGenerator.cs
- COM2IPerPropertyBrowsingHandler.cs
- PropertyGridEditorPart.cs
- TextParaLineResult.cs
- ManagedWndProcTracker.cs