Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ImmutableDispatchRuntime.cs / 1 / ImmutableDispatchRuntime.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Channels; using System.Collections.Generic; using System.Collections.Specialized; using System.Runtime; using System.Threading; using System.Web.Hosting; using System.ServiceModel.Diagnostics; class ImmutableDispatchRuntime { // We mark TLS when making an async callout. The value of processMessage5IsOnTheStack is an object // whose identity is unique to this particular MessageRpc. [ThreadStatic] internal static ManualResetEvent processMessage5IsOnTheStack = null; readonly AuthorizationBehavior authorizationBehavior; readonly int correlationCount; readonly ConcurrencyBehavior concurrency; readonly IDemuxer demuxer; readonly ErrorBehavior error; readonly bool enableFaults; readonly bool ignoreTransactionFlow; readonly IInputSessionShutdown[] inputSessionShutdownHandlers; readonly InstanceBehavior instance; readonly bool isOnServer; readonly bool manualAddressing; readonly IDispatchMessageInspector[] messageInspectors; readonly int parameterInspectorCorrelationOffset; readonly IRequestReplyCorrelator requestReplyCorrelator; readonly SecurityImpersonationBehavior securityImpersonation; readonly TerminatingOperationBehavior terminate; readonly ThreadBehavior thread; readonly TransactionBehavior transaction; readonly bool validateMustUnderstand; readonly MessageRpcProcessor processMessage1; readonly MessageRpcProcessor processMessage2; readonly MessageRpcProcessor processMessage3; readonly MessageRpcProcessor processMessage4; readonly MessageRpcProcessor processMessage5; readonly MessageRpcProcessor processMessage6; readonly MessageRpcProcessor processMessage7; readonly MessageRpcProcessor processMessageCleanup; readonly MessageRpcProcessor processMessageCleanupError; bool didTraceProcessMessage2 = false; bool didTraceProcessMessage3 = false; bool didTraceProcessMessage4 = false; internal ImmutableDispatchRuntime(DispatchRuntime dispatch) { this.authorizationBehavior = AuthorizationBehavior.TryCreate(dispatch); this.concurrency = new ConcurrencyBehavior(dispatch); this.error = new ErrorBehavior(dispatch.ChannelDispatcher); this.enableFaults = dispatch.EnableFaults; this.inputSessionShutdownHandlers = EmptyArray.ToArray(dispatch.InputSessionShutdownHandlers); this.instance = new InstanceBehavior(dispatch, this); this.isOnServer = dispatch.IsOnServer; this.manualAddressing = dispatch.ManualAddressing; this.messageInspectors = EmptyArray .ToArray(dispatch.MessageInspectors); this.requestReplyCorrelator = new RequestReplyCorrelator(); this.securityImpersonation = SecurityImpersonationBehavior.CreateIfNecessary(dispatch); this.terminate = TerminatingOperationBehavior.CreateIfNecessary(dispatch); this.terminate = new TerminatingOperationBehavior(); this.thread = new ThreadBehavior(dispatch); this.validateMustUnderstand = dispatch.ValidateMustUnderstand; this.ignoreTransactionFlow = dispatch.IgnoreTransactionMessageProperty; this.transaction = TransactionBehavior.CreateIfNeeded(dispatch); this.parameterInspectorCorrelationOffset = (dispatch.MessageInspectors.Count + dispatch.MaxCallContextInitializers); this.correlationCount = this.parameterInspectorCorrelationOffset + dispatch.MaxParameterInspectors; DispatchOperationRuntime unhandled = new DispatchOperationRuntime(dispatch.UnhandledDispatchOperation, this); if (dispatch.OperationSelector == null) { ActionDemuxer demuxer = new ActionDemuxer(); for (int i=0; i 0) { AfterReceiveRequestCore(ref rpc); } } internal void AfterReceiveRequestCore(ref MessageRpc rpc) { int offset = this.MessageInspectorCorrelationOffset; try { for (int i = 0; i < this.messageInspectors.Length; i++) { rpc.Correlation[offset + i] = this.messageInspectors[i].AfterReceiveRequest(ref rpc.Request, (IClientChannel)rpc.Channel.Proxy, rpc.InstanceContext); } } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } if (ErrorBehavior.ShouldRethrowExceptionAsIs(e)) { throw; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperCallback(e); } } void BeforeSendReply(ref MessageRpc rpc, ref Exception exception, ref bool thereIsAnUnhandledException) { if (this.messageInspectors.Length > 0) { BeforeSendReplyCore(ref rpc, ref exception, ref thereIsAnUnhandledException); } } internal void BeforeSendReplyCore(ref MessageRpc rpc, ref Exception exception, ref bool thereIsAnUnhandledException) { int offset = this.MessageInspectorCorrelationOffset; for (int i = 0; i < this.messageInspectors.Length; i++) { try { Message originalReply = rpc.Reply; Message reply = originalReply; this.messageInspectors[i].BeforeSendReply(ref reply, rpc.Correlation[offset + i]); if ((reply == null) && (originalReply != null)) { string message = SR.GetString(SR.SFxNullReplyFromExtension2, this.messageInspectors[i].GetType().ToString(), (rpc.Operation.Name ?? "")); ErrorBehavior.ThrowAndCatch(new InvalidOperationException(message)); } rpc.Reply = reply; } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } if (!ErrorBehavior.ShouldRethrowExceptionAsIs(e)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperCallback(e); } if (exception == null) { exception = e; } thereIsAnUnhandledException = (!this.error.HandleError(e)) || thereIsAnUnhandledException; } } } internal bool Dispatch(ref MessageRpc rpc, bool isOperationContextSet) { rpc.ErrorProcessor = this.processMessageCleanup; rpc.NextProcessor = this.processMessage1; return rpc.Process(isOperationContextSet); } internal void InputSessionDoneReceiving(ServiceChannel channel) { if (this.inputSessionShutdownHandlers.Length > 0) { this.InputSessionDoneReceivingCore(channel); } } void InputSessionDoneReceivingCore(ServiceChannel channel) { IDuplexContextChannel proxy = channel.Proxy as IDuplexContextChannel; if (proxy != null) { IInputSessionShutdown[] handlers = this.inputSessionShutdownHandlers; try { for (int i=0; i 0) { this.InputSessionFaultedCore(channel); } } void InputSessionFaultedCore(ServiceChannel channel) { IDuplexContextChannel proxy = channel.Proxy as IDuplexContextChannel; if (proxy != null) { IInputSessionShutdown[] handlers = this.inputSessionShutdownHandlers; try { for (int i=0; i map; IDispatchOperationSelector selector; DispatchOperationRuntime unhandled; internal CustomDemuxer(IDispatchOperationSelector selector) { this.selector = selector; this.map = new Dictionary (); } internal void Add(string name, DispatchOperationRuntime operation) { this.map.Add(name, operation); } internal void SetUnhandled(DispatchOperationRuntime operation) { this.unhandled = operation; } public DispatchOperationRuntime GetOperation(ref Message request) { string operationName = this.selector.SelectOperation(ref request); DispatchOperationRuntime operation = null; if (this.map.TryGetValue(operationName, out operation)) return operation; else return this.unhandled; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
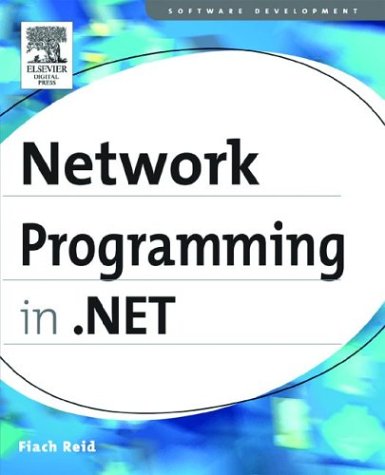
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ItemsControlAutomationPeer.cs
- ThreadPool.cs
- BitmapCodecInfo.cs
- XmlNode.cs
- Style.cs
- SymbolType.cs
- AccessViolationException.cs
- AggregationMinMaxHelpers.cs
- ConfigurationCollectionAttribute.cs
- DynamicEndpointElement.cs
- EpmSyndicationContentSerializer.cs
- EndpointAddressProcessor.cs
- ServiceAuthorizationBehavior.cs
- _DisconnectOverlappedAsyncResult.cs
- XamlDesignerSerializationManager.cs
- DataServiceConfiguration.cs
- MLangCodePageEncoding.cs
- HostingEnvironment.cs
- FileLevelControlBuilderAttribute.cs
- CursorEditor.cs
- ActivityDesigner.cs
- WindowVisualStateTracker.cs
- ToolStripInSituService.cs
- SmtpNtlmAuthenticationModule.cs
- MultilineStringEditor.cs
- SafeCryptHandles.cs
- VirtualDirectoryMappingCollection.cs
- MULTI_QI.cs
- DataServiceRequestOfT.cs
- AccessText.cs
- TableAdapterManagerMethodGenerator.cs
- DSASignatureFormatter.cs
- DuplicateWaitObjectException.cs
- PointAnimationBase.cs
- QilGeneratorEnv.cs
- RegistryPermission.cs
- EncodingTable.cs
- StorageRoot.cs
- Transform3D.cs
- UnsafeNativeMethods.cs
- View.cs
- QuotedPrintableStream.cs
- ReflectTypeDescriptionProvider.cs
- NavigateEvent.cs
- RawMouseInputReport.cs
- DBAsyncResult.cs
- Delegate.cs
- TextFormatter.cs
- InputReport.cs
- SemanticAnalyzer.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- Transform.cs
- iisPickupDirectory.cs
- ChtmlTextWriter.cs
- DiffuseMaterial.cs
- ProxyHwnd.cs
- ExceptionUtil.cs
- ColumnResult.cs
- SemaphoreSecurity.cs
- DataControlFieldCollection.cs
- Matrix3D.cs
- SqlTransaction.cs
- XPathScanner.cs
- sqlser.cs
- DataGridViewRowsRemovedEventArgs.cs
- GlobalProxySelection.cs
- DetailsView.cs
- DES.cs
- EmptyTextWriter.cs
- ProxyGenerator.cs
- EdmItemCollection.cs
- Panel.cs
- EventPrivateKey.cs
- AttributedMetaModel.cs
- WebRequestModuleElementCollection.cs
- OrderedDictionary.cs
- FormatSelectingMessageInspector.cs
- RepeaterItemCollection.cs
- Site.cs
- MULTI_QI.cs
- XPathParser.cs
- MailWriter.cs
- SettingsAttributes.cs
- Binding.cs
- DesignTimeXamlWriter.cs
- KeyGestureConverter.cs
- SqlTypesSchemaImporter.cs
- CodeDelegateCreateExpression.cs
- AssemblyCollection.cs
- CommandValueSerializer.cs
- MsmqAuthenticationMode.cs
- PartitionerStatic.cs
- PersonalizationDictionary.cs
- FormsAuthentication.cs
- ByteAnimationUsingKeyFrames.cs
- KeyMatchBuilder.cs
- WriteTimeStream.cs
- ContextMenuAutomationPeer.cs
- DataGridViewRowEventArgs.cs
- RC2.cs