Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Policy / Site.cs / 1 / Site.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Site.cs // // Site is an IIdentity representing internet sites. // namespace System.Security.Policy { using System.Security.Util; using System.Globalization; using SiteIdentityPermission = System.Security.Permissions.SiteIdentityPermission; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Site : IIdentityPermissionFactory, IBuiltInEvidence { private SiteString m_name; internal Site() { m_name = null; } public Site(String name) { if (name == null) throw new ArgumentNullException("name"); m_name = new SiteString( name ); } internal Site( byte[] id, String name ) { m_name = ParseSiteFromUrl( name ); } public static Site CreateFromUrl( String url ) { Site site = new Site(); site.m_name = ParseSiteFromUrl( url ); return site; } private static SiteString ParseSiteFromUrl( String name ) { URLString urlString = new URLString( name ); if (String.Compare( urlString.Scheme, "file", StringComparison.OrdinalIgnoreCase) == 0) throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidSite" ) ); return new SiteString( new URLString( name ).Host ); } public String Name { get { if (m_name != null) return m_name.ToString(); else return null; } } internal SiteString GetSiteString() { return m_name; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new SiteIdentityPermission( Name ); } public override bool Equals(Object o) { if (o is Site) { Site s = (Site) o; if (Name == null) return (s.Name == null); return String.Compare( Name, s.Name, StringComparison.OrdinalIgnoreCase) == 0; } return false; } public override int GetHashCode() { String name = this.Name; if (name == null) return 0; else return name.GetHashCode(); } public Object Copy() { return new Site(this.Name); } internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Site" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Site" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); if(m_name != null) elem.AddChild( new SecurityElement( "Name", m_name.ToString() ) ); return elem; } ///int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position++] = BuiltInEvidenceHelper.idSite; String name = this.Name; int length = name.Length; if (verbose) { BuiltInEvidenceHelper.CopyIntToCharArray(length, buffer, position); position += 2; } name.CopyTo( 0, buffer, position, length ); return length + position; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose) { if (verbose) return this.Name.Length + 3; else return this.Name.Length + 1; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position) { int length = BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); position += 2; m_name = new SiteString( new String(buffer, position, length )); return position + length; } public override String ToString() { return ToXml().ToString(); } // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_name.ToString().ToUpper(CultureInfo.InvariantCulture); } } }
Link Menu
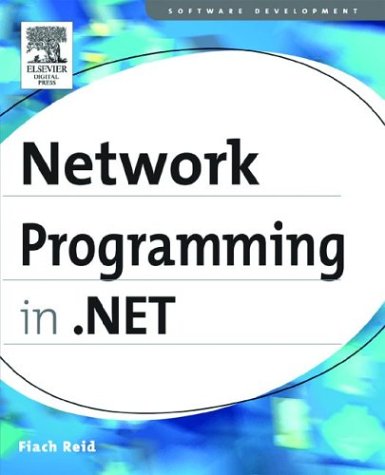
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SortableBindingList.cs
- RawUIStateInputReport.cs
- RelationshipEndCollection.cs
- ParameterCollection.cs
- PostBackOptions.cs
- CompileXomlTask.cs
- HostingEnvironmentWrapper.cs
- HandlerMappingMemo.cs
- _ProxyRegBlob.cs
- TemplateEditingVerb.cs
- HttpModulesSection.cs
- LinkUtilities.cs
- WindowsGrip.cs
- CssTextWriter.cs
- DataGridRow.cs
- MetaForeignKeyColumn.cs
- DBSqlParserColumnCollection.cs
- BufferedMessageData.cs
- RequestNavigateEventArgs.cs
- NamespaceCollection.cs
- RowToParametersTransformer.cs
- Inflater.cs
- BooleanSwitch.cs
- ScriptHandlerFactory.cs
- WebPartCancelEventArgs.cs
- StorageEntitySetMapping.cs
- BitArray.cs
- SqlProcedureAttribute.cs
- LogicalTreeHelper.cs
- dataobject.cs
- LinkTarget.cs
- EntityDataSourceState.cs
- DrawingVisualDrawingContext.cs
- HotCommands.cs
- IntMinMaxAggregationOperator.cs
- FamilyTypefaceCollection.cs
- SrgsElementFactory.cs
- SqlCaseSimplifier.cs
- SqlCharStream.cs
- MulticastOption.cs
- BindingSource.cs
- ItemContainerGenerator.cs
- IgnoreSection.cs
- SqlWriter.cs
- assemblycache.cs
- PreservationFileReader.cs
- ServicePointManagerElement.cs
- FixedTextContainer.cs
- SetterBase.cs
- AffineTransform3D.cs
- PrintPreviewGraphics.cs
- RuntimeHandles.cs
- EntityDataSourceContainerNameItem.cs
- MSHTMLHost.cs
- DigitShape.cs
- tooltip.cs
- WeakReferenceKey.cs
- WindowsEditBox.cs
- UserControlBuildProvider.cs
- AppSettingsExpressionBuilder.cs
- HorizontalAlignConverter.cs
- DbConnectionPoolGroupProviderInfo.cs
- DefaultTraceListener.cs
- DiagnosticsConfiguration.cs
- SystemUnicastIPAddressInformation.cs
- TableLayoutSettingsTypeConverter.cs
- Speller.cs
- SingleAnimationUsingKeyFrames.cs
- brushes.cs
- DynamicDataExtensions.cs
- DataServiceQuery.cs
- GlobalizationSection.cs
- _SSPISessionCache.cs
- CodeBinaryOperatorExpression.cs
- HttpCapabilitiesBase.cs
- DataGridViewBand.cs
- VSWCFServiceContractGenerator.cs
- dataprotectionpermission.cs
- DataGridViewLinkColumn.cs
- ExecutionContext.cs
- WebHeaderCollection.cs
- WindowsFormsHostPropertyMap.cs
- ContextProperty.cs
- ThemeableAttribute.cs
- CounterSetInstance.cs
- propertytag.cs
- AccessDataSourceDesigner.cs
- TimeZone.cs
- XsdBuildProvider.cs
- SingleObjectCollection.cs
- ConnectionOrientedTransportChannelListener.cs
- ServerType.cs
- Path.cs
- AssemblyAttributesGoHere.cs
- ComNativeDescriptor.cs
- EntityReference.cs
- DataGridViewDesigner.cs
- SchemaDeclBase.cs
- StateDesigner.LayoutSelectionGlyph.cs
- DataBindingExpressionBuilder.cs