Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Helper.cs / 1305376 / Helper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Linq; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Xml.XPath; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; namespace System.Data.Metadata.Edm { ////// Helper Class for EDM Metadata - this class contains all the helper methods /// which only accesses public methods/properties. The other partial class contains all /// helper methods which just uses internal methods/properties. The reason why we /// did this for allowing view gen to happen at compile time - all the helper /// methods that view gen or mapping uses are in this class. Rest of the /// methods are in this class /// internal static partial class Helper { #region Fields internal static readonly EdmMember[] EmptyArrayEdmProperty = new EdmMember[0]; #endregion #region Methods ////// The method wraps the GetAttribute method on XPathNavigator. /// The problem with using the method directly is that the /// Get Attribute method does not differentiate the absence of an attribute and /// having an attribute with Empty string value. In both cases the value returned is an empty string. /// So in case of optional attributes, it becomes hard to distinguish the case whether the /// xml contains the attribute with empty string or doesn't contain the attribute /// This method will return null if the attribute is not present and otherwise will return the /// attribute value. /// /// /// name of the attribute ///static internal string GetAttributeValue(XPathNavigator nav, string attributeName) { //Clone the navigator so that there wont be any sideeffects on the passed in Navigator nav = nav.Clone(); string attributeValue = null; if (nav.MoveToAttribute(attributeName, string.Empty)) { attributeValue = nav.Value; } return attributeValue; } /// /// The method returns typed attribute value of the specified xml attribute. /// The method does not do any specific casting but uses the methods on XPathNavigator. /// /// /// /// ///internal static object GetTypedAttributeValue(XPathNavigator nav, string attributeName, Type clrType) { //Clone the navigator so that there wont be any sideeffects on the passed in Navigator nav = nav.Clone(); object attributeValue = null; if (nav.MoveToAttribute(attributeName, string.Empty)) { attributeValue = nav.ValueAs(clrType); } return attributeValue; } /// /// Searches for Facet Description with the name specified. /// /// Collection of facet description /// name of the facet ///internal static FacetDescription GetFacet(IEnumerable facetCollection, string facetName) { foreach (FacetDescription facetDescription in facetCollection) { if (facetDescription.FacetName == facetName) { return facetDescription; } } return null; } // requires: firstType is not null // effects: Returns true iff firstType is assignable from secondType internal static bool IsAssignableFrom(EdmType firstType, EdmType secondType) { Debug.Assert(firstType != null, "firstType should not be not null"); if (secondType == null) { return false; } return firstType.Equals(secondType) || IsSubtypeOf(secondType, firstType); } // requires: firstType is not null // effects: if otherType is among the base types, return true, // otherwise returns false. // when othertype is same as the current type, return false. internal static bool IsSubtypeOf(EdmType firstType, EdmType secondType) { Debug.Assert(firstType != null, "firstType should not be not null"); if (secondType == null) { return false; } // walk up my type hierarchy list for (EdmType t = firstType.BaseType; t != null; t = t.BaseType) { if (t == secondType) return true; } return false; } internal static IList GetAllStructuralMembers(EdmType edmType) { switch (edmType.BuiltInTypeKind) { case BuiltInTypeKind.AssociationType: return ((AssociationType)edmType).AssociationEndMembers; case BuiltInTypeKind.ComplexType: return ((ComplexType)edmType).Properties; case BuiltInTypeKind.EntityType: return ((EntityType)edmType).Properties; case BuiltInTypeKind.RowType: return ((RowType)edmType).Properties; default: return EmptyArrayEdmProperty; } } internal static AssociationEndMember GetEndThatShouldBeMappedToKey(AssociationType associationType) { //For 1:* and 1:0..1 associations, the end other than 1 i.e. either * or 0..1 ends need to be //mapped to key columns if (associationType.AssociationEndMembers.Any( it => it.RelationshipMultiplicity.Equals(RelationshipMultiplicity.One))) { { return associationType.AssociationEndMembers.SingleOrDefault(it => ((it.RelationshipMultiplicity.Equals(RelationshipMultiplicity.Many)) || (it.RelationshipMultiplicity.Equals(RelationshipMultiplicity.ZeroOrOne)))); } } //For 0..1:* associations, * end must be mapped to key. else if (associationType.AssociationEndMembers.Any(it => (it.RelationshipMultiplicity.Equals(RelationshipMultiplicity.ZeroOrOne)))) { { return associationType.AssociationEndMembers.SingleOrDefault(it => ((it.RelationshipMultiplicity.Equals(RelationshipMultiplicity.Many)))); } } return null; } /// /// Creates a single comma delimited string given a list of strings /// /// ///internal static String GetCommaDelimitedString(IEnumerable stringList) { Debug.Assert(stringList != null , "Expecting a non null list"); StringBuilder sb = new StringBuilder(); bool first = true; foreach (string part in stringList) { if (!first) { sb.Append(", "); } else { first = false; } sb.Append(part); } return sb.ToString(); } // effects: concatenates all given enumerations internal static IEnumerable Concat (params IEnumerable [] sources) { foreach (IEnumerable source in sources) { if (null != source) { foreach (T element in source) { yield return element; } } } } internal static void DisposeXmlReaders(IEnumerable xmlReaders) { Debug.Assert(xmlReaders != null); foreach (XmlReader xmlReader in xmlReaders) { ((IDisposable)xmlReader).Dispose(); } } #region IsXXXType Methods internal static bool IsStructuralType(EdmType type) { return (IsComplexType(type) || IsEntityType(type) || IsRelationshipType(type) || IsRowType(type)); } internal static bool IsCollectionType(GlobalItem item) { return (BuiltInTypeKind.CollectionType == item.BuiltInTypeKind); } internal static bool IsEntityType(EdmType type) { return (BuiltInTypeKind.EntityType == type.BuiltInTypeKind); } internal static bool IsComplexType(EdmType type) { return (BuiltInTypeKind.ComplexType == type.BuiltInTypeKind); } internal static bool IsPrimitiveType(EdmType type) { return (BuiltInTypeKind.PrimitiveType == type.BuiltInTypeKind); } internal static bool IsRefType(GlobalItem item) { return (BuiltInTypeKind.RefType == item.BuiltInTypeKind); } internal static bool IsRowType(GlobalItem item) { return (BuiltInTypeKind.RowType == item.BuiltInTypeKind); } internal static bool IsAssociationType(EdmType type) { return (BuiltInTypeKind.AssociationType == type.BuiltInTypeKind); } internal static bool IsRelationshipType(EdmType type) { return (BuiltInTypeKind.AssociationType == type.BuiltInTypeKind); } internal static bool IsEdmProperty(EdmMember member) { return (BuiltInTypeKind.EdmProperty == member.BuiltInTypeKind); } internal static bool IsRelationshipEndMember(EdmMember member) { return (BuiltInTypeKind.AssociationEndMember == member.BuiltInTypeKind); } internal static bool IsAssociationEndMember(EdmMember member) { return (BuiltInTypeKind.AssociationEndMember == member.BuiltInTypeKind); } internal static bool IsNavigationProperty(EdmMember member) { return (BuiltInTypeKind.NavigationProperty == member.BuiltInTypeKind); } internal static bool IsEntityTypeBase(EdmType edmType) { return Helper.IsEntityType(edmType) || Helper.IsRelationshipType(edmType); } internal static bool IsTransientType(EdmType edmType) { return Helper.IsCollectionType(edmType) || Helper.IsRefType(edmType) || Helper.IsRowType(edmType); } internal static bool IsEntitySet(EntitySetBase entitySetBase) { return BuiltInTypeKind.EntitySet == entitySetBase.BuiltInTypeKind; } internal static bool IsRelationshipSet(EntitySetBase entitySetBase) { return BuiltInTypeKind.AssociationSet == entitySetBase.BuiltInTypeKind; } internal static bool IsEntityContainer(GlobalItem item) { return BuiltInTypeKind.EntityContainer == item.BuiltInTypeKind; } internal static bool IsEdmFunction(GlobalItem item) { return BuiltInTypeKind.EdmFunction == item.BuiltInTypeKind; } internal static string GetFileNameFromUri(Uri uri) { if ( uri == null ) throw new ArgumentNullException("uri"); if ( uri.IsFile ) return uri.LocalPath; if ( uri.IsAbsoluteUri ) return uri.AbsolutePath; throw new ArgumentException(System.Data.Entity.Strings.UnacceptableUri(uri),"uri"); } internal static bool IsEnumType(EdmType edmType) { return BuiltInTypeKind.EnumType == edmType.BuiltInTypeKind; } internal static bool IsUnboundedFacetValue(Facet facet) { return object.ReferenceEquals(facet.Value, EdmConstants.UnboundedValue); } #endregion /// IsXXXType region /// Performance of Enum.ToString() is slow and we use this value in building Identity internal static string ToString(System.Data.ParameterDirection value) { switch (value) { case ParameterDirection.Input: return "Input"; case ParameterDirection.Output: return "Output"; case ParameterDirection.InputOutput: return "InputOutput"; case ParameterDirection.ReturnValue: return "ReturnValue"; default: Debug.Assert(false, "which ParameterDirection.ToString() is missing?"); return value.ToString(); } } ///Performance of Enum.ToString() is slow and we use this value in building Identity internal static string ToString(System.Data.Metadata.Edm.ParameterMode value) { switch (value) { case ParameterMode.In: return EdmConstants.In; case ParameterMode.Out: return EdmConstants.Out; case ParameterMode.InOut: return EdmConstants.InOut; case ParameterMode.ReturnValue: return "ReturnValue"; default: Debug.Assert(false, "which ParameterMode.ToString() is missing?"); return value.ToString(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
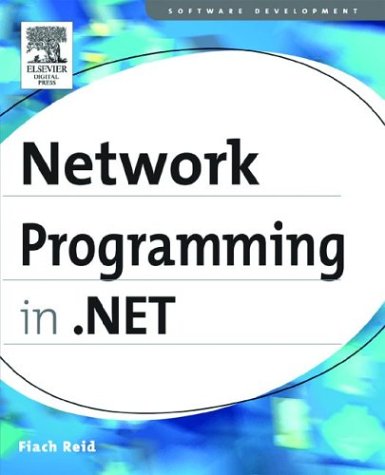
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RemotingClientProxy.cs
- HttpAsyncResult.cs
- Typography.cs
- CustomAttributeFormatException.cs
- MessageBodyDescription.cs
- CommonGetThemePartSize.cs
- ProcessHostServerConfig.cs
- BoundField.cs
- ItemDragEvent.cs
- SetterBase.cs
- ResXFileRef.cs
- HttpResponseWrapper.cs
- MatrixValueSerializer.cs
- DataGridViewDataErrorEventArgs.cs
- ProgressiveCrcCalculatingStream.cs
- UInt16Storage.cs
- ApplicationCommands.cs
- ServiceElementCollection.cs
- HttpInputStream.cs
- AssemblyResourceLoader.cs
- EntityDataSourceSelectingEventArgs.cs
- ParserExtension.cs
- XPathConvert.cs
- FixedLineResult.cs
- DataTable.cs
- MembershipSection.cs
- CLRBindingWorker.cs
- QueryIntervalOp.cs
- DynamicDiscoveryDocument.cs
- ModuleConfigurationInfo.cs
- SendKeys.cs
- OdbcDataAdapter.cs
- JsonDeserializer.cs
- ResourceExpression.cs
- ItemDragEvent.cs
- HTMLTextWriter.cs
- DetectEofStream.cs
- PixelFormatConverter.cs
- ThicknessAnimationBase.cs
- ViewManager.cs
- InkPresenterAutomationPeer.cs
- AddInActivator.cs
- BaseCodeDomTreeGenerator.cs
- SplitterCancelEvent.cs
- RawStylusActions.cs
- Int32RectConverter.cs
- DesignerView.xaml.cs
- TemplatePartAttribute.cs
- LinqToSqlWrapper.cs
- SelectionRange.cs
- DeploymentSection.cs
- XmlSchemaValidator.cs
- Zone.cs
- ServiceContractViewControl.cs
- StatusStrip.cs
- HtmlElementEventArgs.cs
- ButtonPopupAdapter.cs
- TableLayoutSettings.cs
- XmlSchemaImport.cs
- FixedSOMTableRow.cs
- SelectedDatesCollection.cs
- ToolStripMenuItemCodeDomSerializer.cs
- UpdateEventArgs.cs
- ControlAdapter.cs
- ClockGroup.cs
- CodeTypeDeclarationCollection.cs
- X509WindowsSecurityToken.cs
- DocumentPageHost.cs
- UrlPath.cs
- ControlPaint.cs
- DSASignatureDeformatter.cs
- HtmlInputCheckBox.cs
- SubMenuStyleCollection.cs
- DeviceSpecificDialogCachedState.cs
- JavaScriptSerializer.cs
- XmlWhitespace.cs
- XappLauncher.cs
- RegexNode.cs
- exports.cs
- SelectiveScrollingGrid.cs
- NetworkInformationException.cs
- SrgsGrammarCompiler.cs
- SystemEvents.cs
- GridPattern.cs
- VectorValueSerializer.cs
- ImageMap.cs
- WebAdminConfigurationHelper.cs
- XamlSerializationHelper.cs
- VoiceObjectToken.cs
- JsonReaderWriterFactory.cs
- QueryCacheEntry.cs
- ClientConvert.cs
- WebPartEditorApplyVerb.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- UDPClient.cs
- LockCookie.cs
- GridViewCommandEventArgs.cs
- NamedObject.cs
- ReadOnlyHierarchicalDataSource.cs
- IPCCacheManager.cs