Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / CombinedGeometry.cs / 1 / CombinedGeometry.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Implementation of CombinedGeometry // // History: // 2004/11/11-Michka // Created it // //--------------------------------------------------------------------------- using System; using MS.Internal; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Windows.Media.Composition; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Markup; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// CombinedGeometry /// public sealed partial class CombinedGeometry : Geometry { #region Constructors ////// Default constructor /// public CombinedGeometry() { } ////// Constructor from 2 operands /// /// /// First geometry to combine /// /// /// Second geometry to combine /// public CombinedGeometry( Geometry geometry1, Geometry geometry2 ) { Geometry1 = geometry1; Geometry2 = geometry2; } ////// Constructor from combine mode and 2 operands /// /// /// Combine mode - Union, Intersect, Exclude or Xor /// /// /// First geometry to combine /// /// /// Second geometry to combine /// public CombinedGeometry( GeometryCombineMode geometryCombineMode, Geometry geometry1, Geometry geometry2 ) { GeometryCombineMode = geometryCombineMode; Geometry1 = geometry1; Geometry2 = geometry2; } ////// Constructor from combine mode, 2 operands and a transformation /// /// /// Combine mode - Union, Intersect, Exclude or Xor /// /// /// First geometry to combine /// /// /// Second geometry to combine /// /// /// Transformation to apply to the result /// public CombinedGeometry( GeometryCombineMode geometryCombineMode, Geometry geometry1, Geometry geometry2, Transform transform) { GeometryCombineMode = geometryCombineMode; Geometry1 = geometry1; Geometry2 = geometry2; Transform = transform; } #endregion #region Bounds ////// Gets the bounds of this Geometry as an axis-aligned bounding box /// public override Rect Bounds { get { ReadPreamble(); // GetAsPathGeometry() checks if the geometry is valid return GetAsPathGeometry().Bounds; } } #endregion #region GetBoundsInternal ////// Gets the bounds of this Geometry as an axis-aligned bounding box given a Pen and/or Transform /// internal override Rect GetBoundsInternal(Pen pen, Matrix matrix, double tolerance, ToleranceType type) { if (IsObviouslyEmpty()) { return Rect.Empty; } return GetAsPathGeometry().GetBoundsInternal(pen, matrix, tolerance, type); } #endregion #region Hit Testing ////// Returns if point is inside the filled geometry. /// internal override bool ContainsInternal(Pen pen, Point hitPoint, double tolerance, ToleranceType type) { if (pen == null) { ReadPreamble(); // Hit the two operands bool hit1 = false; bool hit2 = false; Transform transform = Transform; if (transform != null && !transform.IsIdentity) { // Inverse-transform the hit point Matrix matrix = transform.Value; if (matrix.HasInverse) { matrix.Invert(); hitPoint *= matrix; } else { // The matrix will collapse the geometry to nothing, containing nothing return false; } } Geometry geometry1 = Geometry1; Geometry geometry2 = Geometry2; if (geometry1 != null) { hit1 = geometry1.ContainsInternal(pen, hitPoint, tolerance, type); } if (geometry2 != null) { hit2 = geometry2.ContainsInternal(pen, hitPoint, tolerance, type); } // Determine containment according to the theoretical definition switch (GeometryCombineMode) { case GeometryCombineMode.Union: return hit1 || hit2; case GeometryCombineMode.Intersect: return hit1 && hit2; case GeometryCombineMode.Exclude: return hit1 && !hit2; case GeometryCombineMode.Xor: return hit1 != hit2; } // We should have returned from one of the cases Debug.Assert(false); return false; } else { // pen != null return base.ContainsInternal(pen, hitPoint, tolerance, type); } } #endregion ////// Gets the area of this geometry /// /// The computational error tolerance /// The way the error tolerance will be interpreted - realtive or absolute public override double GetArea(double tolerance, ToleranceType type) { ReadPreamble(); // Potential speedup, to be done if proved important: As the result of a Combine // operation, the result of GetAsPathGeometry() is guaranteed to be organized into // flattened well oriented figures. Its area can therefore be computed much faster // without the heavy machinary of CArea. This will require writing an internal // CShapeBase::GetRawArea method, and a utility to invoke it. For now: return GetAsPathGeometry().GetArea(tolerance, type); } #region Internal internal override PathFigureCollection GetTransformedFigureCollection(Transform transform) { return GetAsPathGeometry().GetTransformedFigureCollection(transform); } ////// GetPathGeometryData - returns a struct which contains this Geometry represented /// as a path geometry's serialized format. /// internal override PathGeometryData GetPathGeometryData() { if (IsObviouslyEmpty()) { return Geometry.GetEmptyPathGeometryData(); } PathGeometry pathGeometry = GetAsPathGeometry(); return pathGeometry.GetPathGeometryData(); } internal override PathGeometry GetAsPathGeometry() { // Get the operands, interpreting null as empty PathGeometry Geometry g1 = Geometry1; Geometry g2 = Geometry2; PathGeometry geometry1 = (g1 == null) ? new PathGeometry() : g1.GetAsPathGeometry(); Geometry geometry2 = (g2 == null) ? new PathGeometry() : g2.GetAsPathGeometry(); // Combine them and return the result return Combine(geometry1, geometry2, GeometryCombineMode, Transform); } #endregion #region IsEmpty ////// Returns true if this geometry is empty /// public override bool IsEmpty() { return GetAsPathGeometry().IsEmpty(); } internal override bool IsObviouslyEmpty() { // See which operand is obviously empty Geometry geometry1 = Geometry1; Geometry geometry2 = Geometry2; bool empty1 = geometry1 == null || geometry1.IsObviouslyEmpty(); bool empty2 = geometry2 == null || geometry2.IsObviouslyEmpty(); // Depending on the operation -- if (GeometryCombineMode == GeometryCombineMode.Intersect) { return empty1 || empty2; } else if (GeometryCombineMode == GeometryCombineMode.Exclude) { return empty1; } else { // Union or Xor return empty1 && empty2; } } #endregion IsEmpty ////// Returns true if this geometry may have curved segments /// public override bool MayHaveCurves() { Geometry geometry1 = Geometry1; Geometry geometry2 = Geometry2; return ((geometry1 != null) && geometry1.MayHaveCurves()) || ((geometry2 != null) && geometry2.MayHaveCurves()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Implementation of CombinedGeometry // // History: // 2004/11/11-Michka // Created it // //--------------------------------------------------------------------------- using System; using MS.Internal; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Windows.Media.Composition; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Markup; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// CombinedGeometry /// public sealed partial class CombinedGeometry : Geometry { #region Constructors ////// Default constructor /// public CombinedGeometry() { } ////// Constructor from 2 operands /// /// /// First geometry to combine /// /// /// Second geometry to combine /// public CombinedGeometry( Geometry geometry1, Geometry geometry2 ) { Geometry1 = geometry1; Geometry2 = geometry2; } ////// Constructor from combine mode and 2 operands /// /// /// Combine mode - Union, Intersect, Exclude or Xor /// /// /// First geometry to combine /// /// /// Second geometry to combine /// public CombinedGeometry( GeometryCombineMode geometryCombineMode, Geometry geometry1, Geometry geometry2 ) { GeometryCombineMode = geometryCombineMode; Geometry1 = geometry1; Geometry2 = geometry2; } ////// Constructor from combine mode, 2 operands and a transformation /// /// /// Combine mode - Union, Intersect, Exclude or Xor /// /// /// First geometry to combine /// /// /// Second geometry to combine /// /// /// Transformation to apply to the result /// public CombinedGeometry( GeometryCombineMode geometryCombineMode, Geometry geometry1, Geometry geometry2, Transform transform) { GeometryCombineMode = geometryCombineMode; Geometry1 = geometry1; Geometry2 = geometry2; Transform = transform; } #endregion #region Bounds ////// Gets the bounds of this Geometry as an axis-aligned bounding box /// public override Rect Bounds { get { ReadPreamble(); // GetAsPathGeometry() checks if the geometry is valid return GetAsPathGeometry().Bounds; } } #endregion #region GetBoundsInternal ////// Gets the bounds of this Geometry as an axis-aligned bounding box given a Pen and/or Transform /// internal override Rect GetBoundsInternal(Pen pen, Matrix matrix, double tolerance, ToleranceType type) { if (IsObviouslyEmpty()) { return Rect.Empty; } return GetAsPathGeometry().GetBoundsInternal(pen, matrix, tolerance, type); } #endregion #region Hit Testing ////// Returns if point is inside the filled geometry. /// internal override bool ContainsInternal(Pen pen, Point hitPoint, double tolerance, ToleranceType type) { if (pen == null) { ReadPreamble(); // Hit the two operands bool hit1 = false; bool hit2 = false; Transform transform = Transform; if (transform != null && !transform.IsIdentity) { // Inverse-transform the hit point Matrix matrix = transform.Value; if (matrix.HasInverse) { matrix.Invert(); hitPoint *= matrix; } else { // The matrix will collapse the geometry to nothing, containing nothing return false; } } Geometry geometry1 = Geometry1; Geometry geometry2 = Geometry2; if (geometry1 != null) { hit1 = geometry1.ContainsInternal(pen, hitPoint, tolerance, type); } if (geometry2 != null) { hit2 = geometry2.ContainsInternal(pen, hitPoint, tolerance, type); } // Determine containment according to the theoretical definition switch (GeometryCombineMode) { case GeometryCombineMode.Union: return hit1 || hit2; case GeometryCombineMode.Intersect: return hit1 && hit2; case GeometryCombineMode.Exclude: return hit1 && !hit2; case GeometryCombineMode.Xor: return hit1 != hit2; } // We should have returned from one of the cases Debug.Assert(false); return false; } else { // pen != null return base.ContainsInternal(pen, hitPoint, tolerance, type); } } #endregion ////// Gets the area of this geometry /// /// The computational error tolerance /// The way the error tolerance will be interpreted - realtive or absolute public override double GetArea(double tolerance, ToleranceType type) { ReadPreamble(); // Potential speedup, to be done if proved important: As the result of a Combine // operation, the result of GetAsPathGeometry() is guaranteed to be organized into // flattened well oriented figures. Its area can therefore be computed much faster // without the heavy machinary of CArea. This will require writing an internal // CShapeBase::GetRawArea method, and a utility to invoke it. For now: return GetAsPathGeometry().GetArea(tolerance, type); } #region Internal internal override PathFigureCollection GetTransformedFigureCollection(Transform transform) { return GetAsPathGeometry().GetTransformedFigureCollection(transform); } ////// GetPathGeometryData - returns a struct which contains this Geometry represented /// as a path geometry's serialized format. /// internal override PathGeometryData GetPathGeometryData() { if (IsObviouslyEmpty()) { return Geometry.GetEmptyPathGeometryData(); } PathGeometry pathGeometry = GetAsPathGeometry(); return pathGeometry.GetPathGeometryData(); } internal override PathGeometry GetAsPathGeometry() { // Get the operands, interpreting null as empty PathGeometry Geometry g1 = Geometry1; Geometry g2 = Geometry2; PathGeometry geometry1 = (g1 == null) ? new PathGeometry() : g1.GetAsPathGeometry(); Geometry geometry2 = (g2 == null) ? new PathGeometry() : g2.GetAsPathGeometry(); // Combine them and return the result return Combine(geometry1, geometry2, GeometryCombineMode, Transform); } #endregion #region IsEmpty ////// Returns true if this geometry is empty /// public override bool IsEmpty() { return GetAsPathGeometry().IsEmpty(); } internal override bool IsObviouslyEmpty() { // See which operand is obviously empty Geometry geometry1 = Geometry1; Geometry geometry2 = Geometry2; bool empty1 = geometry1 == null || geometry1.IsObviouslyEmpty(); bool empty2 = geometry2 == null || geometry2.IsObviouslyEmpty(); // Depending on the operation -- if (GeometryCombineMode == GeometryCombineMode.Intersect) { return empty1 || empty2; } else if (GeometryCombineMode == GeometryCombineMode.Exclude) { return empty1; } else { // Union or Xor return empty1 && empty2; } } #endregion IsEmpty ////// Returns true if this geometry may have curved segments /// public override bool MayHaveCurves() { Geometry geometry1 = Geometry1; Geometry geometry2 = Geometry2; return ((geometry1 != null) && geometry1.MayHaveCurves()) || ((geometry2 != null) && geometry2.MayHaveCurves()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
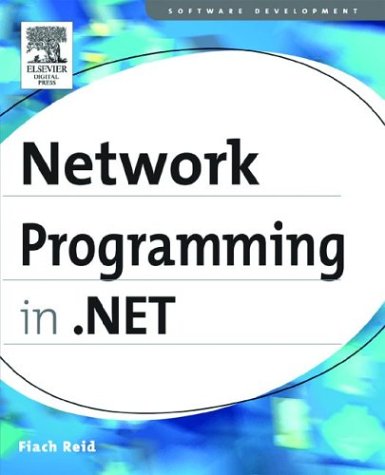
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PhonemeEventArgs.cs
- Relationship.cs
- CreateParams.cs
- ResourceIDHelper.cs
- FixUp.cs
- RunWorkerCompletedEventArgs.cs
- Boolean.cs
- securitycriticaldataClass.cs
- __Filters.cs
- Resources.Designer.cs
- EditorZone.cs
- VisualStyleRenderer.cs
- DataGridRowHeaderAutomationPeer.cs
- CultureMapper.cs
- RequestCacheValidator.cs
- DelegateTypeInfo.cs
- ZipPackage.cs
- SimpleRecyclingCache.cs
- TabControlAutomationPeer.cs
- CommentGlyph.cs
- ListViewPagedDataSource.cs
- WindowsToolbarAsMenu.cs
- SafeNativeMethodsOther.cs
- IntSecurity.cs
- ConfigurationPropertyCollection.cs
- PlainXmlSerializer.cs
- MatrixConverter.cs
- DashStyle.cs
- MachineKeySection.cs
- DependsOnAttribute.cs
- ComponentCache.cs
- DataRecordInternal.cs
- HealthMonitoringSection.cs
- DataGridViewLinkColumn.cs
- _ProxyChain.cs
- HyperLink.cs
- XmlSchemaValidationException.cs
- PageRanges.cs
- GridEntryCollection.cs
- BinaryReader.cs
- RegexMatchCollection.cs
- MiniParameterInfo.cs
- Container.cs
- ListViewGroupItemCollection.cs
- CdpEqualityComparer.cs
- WmlCalendarAdapter.cs
- StringConverter.cs
- StrokeDescriptor.cs
- StyleSheetRefUrlEditor.cs
- FaultFormatter.cs
- SystemDropShadowChrome.cs
- XmlSchemaComplexContentRestriction.cs
- ThemeableAttribute.cs
- CompositeCollection.cs
- DataGridViewBand.cs
- Zone.cs
- ImportFileRequest.cs
- Stream.cs
- JsonServiceDocumentSerializer.cs
- ToolStripLabel.cs
- DictionarySurrogate.cs
- EdmMember.cs
- SortKey.cs
- Validator.cs
- XmlQuerySequence.cs
- ZipIOLocalFileDataDescriptor.cs
- InternalDispatchObject.cs
- MemberRelationshipService.cs
- CharUnicodeInfo.cs
- WebPartDisplayModeCollection.cs
- UniformGrid.cs
- documentsequencetextview.cs
- OdbcConnectionHandle.cs
- MaskInputRejectedEventArgs.cs
- BinaryWriter.cs
- CurrentChangedEventManager.cs
- WebBrowserNavigatedEventHandler.cs
- SQLCharsStorage.cs
- DataSourceCache.cs
- TimelineGroup.cs
- ListSourceHelper.cs
- UIPermission.cs
- ThrowHelper.cs
- WindowsFont.cs
- RegisteredExpandoAttribute.cs
- MeasureItemEvent.cs
- TypeLoadException.cs
- XmlArrayItemAttributes.cs
- AudioFormatConverter.cs
- FontResourceCache.cs
- HttpProfileBase.cs
- DataGridViewRowPostPaintEventArgs.cs
- PageRequestManager.cs
- Html32TextWriter.cs
- ImmutableObjectAttribute.cs
- ArrayTypeMismatchException.cs
- CloseSequence.cs
- StyleTypedPropertyAttribute.cs
- FormsAuthenticationModule.cs
- XPathBuilder.cs