Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartMenuStyle.cs / 2 / WebPartMenuStyle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartMenuStyle : TableStyle, ICustomTypeDescriptor { private const int PROP_SHADOWCOLOR = 0x00200000; public WebPartMenuStyle() : this(null) { } public WebPartMenuStyle(StateBag bag) : base(bag) { CellPadding = 1; CellSpacing = 0; } ////// [ WebCategory("Appearance"), DefaultValue(typeof(Color), ""), TypeConverterAttribute(typeof(WebColorConverter)), WebSysDescription(SR.WebPartMenuStyle_ShadowColor) ] public Color ShadowColor { get { if (IsSet(PROP_SHADOWCOLOR)) { return (Color)(ViewState["ShadowColor"]); } return Color.Empty; } set { ViewState["ShadowColor"] = value; SetBit(PROP_SHADOWCOLOR); } } ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override HorizontalAlign HorizontalAlign { get { return base.HorizontalAlign; } set { } } ///protected override void FillStyleAttributes(CssStyleCollection attributes, IUrlResolutionService urlResolver) { base.FillStyleAttributes(attributes, urlResolver); Color shadowColor = ShadowColor; if (shadowColor.IsEmpty == false) { string colorValue = ColorTranslator.ToHtml(shadowColor); string filterValue = "progid:DXImageTransform.Microsoft.Shadow(color='" + colorValue + "', Direction=135, Strength=3)"; attributes.Add(HtmlTextWriterStyle.Filter, filterValue); } } /// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is WebPartMenuStyle) { WebPartMenuStyle ms = (WebPartMenuStyle)s; // Only copy the BackImageUrl if it isn't in the source Style's registered CSS class if (s.RegisteredCssClass.Length != 0) { if (ms.IsSet(PROP_SHADOWCOLOR)) { ViewState.Remove("ShadowColor"); ClearBit(PROP_SHADOWCOLOR); } } else { if (ms.IsSet(PROP_SHADOWCOLOR)) { this.ShadowColor = ms.ShadowColor; } } } } } /// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, // which is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is WebPartMenuStyle) { WebPartMenuStyle ms = (WebPartMenuStyle)s; // Since we're already copying the registered CSS class in base.MergeWith, we don't // need to any attributes that would be included in that class. if (s.RegisteredCssClass.Length == 0) { if (ms.IsSet(PROP_SHADOWCOLOR) && !this.IsSet(PROP_SHADOWCOLOR)) this.ShadowColor = ms.ShadowColor; } } } } /// public override void Reset() { if (IsSet(PROP_SHADOWCOLOR)) { ViewState.Remove("ShadowColor"); } base.Reset(); } #region ICustomTypeDesciptor implementation System.ComponentModel.AttributeCollection ICustomTypeDescriptor.GetAttributes() { return TypeDescriptor.GetAttributes(this, true); } string ICustomTypeDescriptor.GetClassName() { return TypeDescriptor.GetClassName(this, true); } string ICustomTypeDescriptor.GetComponentName() { return TypeDescriptor.GetComponentName(this, true); } TypeConverter ICustomTypeDescriptor.GetConverter() { return TypeDescriptor.GetConverter(this, true); } EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return TypeDescriptor.GetDefaultEvent(this, true); } PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return TypeDescriptor.GetDefaultProperty(this, true); } object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return TypeDescriptor.GetEditor(this, editorBaseType, true); } EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return TypeDescriptor.GetEvents(this, true); } EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return TypeDescriptor.GetEvents(this, attributes, true); } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return ((ICustomTypeDescriptor)this).GetProperties(null); } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { PropertyDescriptorCollection oldProperties = TypeDescriptor.GetProperties(GetType(), attributes); PropertyDescriptor[] newProperties = new PropertyDescriptor[oldProperties.Count]; PropertyDescriptor oldPaddingProperty = oldProperties["CellPadding"]; PropertyDescriptor newPaddingProperty = TypeDescriptor.CreateProperty(GetType(), oldPaddingProperty, new DefaultValueAttribute(1)); PropertyDescriptor oldSpacingProperty = oldProperties["CellSpacing"]; PropertyDescriptor newSpacingProperty = TypeDescriptor.CreateProperty(GetType(), oldSpacingProperty, new DefaultValueAttribute(0)); PropertyDescriptor oldFontProperty = oldProperties["Font"]; PropertyDescriptor newFontProperty = TypeDescriptor.CreateProperty(GetType(), oldFontProperty, new BrowsableAttribute(false), new ThemeableAttribute(false), new EditorBrowsableAttribute(EditorBrowsableState.Never)); PropertyDescriptor oldForeColorProperty = oldProperties["ForeColor"]; PropertyDescriptor newForeColorProperty = TypeDescriptor.CreateProperty(GetType(), oldForeColorProperty, new BrowsableAttribute(false), new ThemeableAttribute(false), new EditorBrowsableAttribute(EditorBrowsableState.Never)); for (int i = 0; i < oldProperties.Count; i++) { PropertyDescriptor property = oldProperties[i]; if (property == oldPaddingProperty) { newProperties[i] = newPaddingProperty; } else if (property == oldSpacingProperty) { newProperties[i] = newSpacingProperty; } else if (property == oldFontProperty) { newProperties[i] = newFontProperty; } else if (property == oldForeColorProperty) { newProperties[i] = newForeColorProperty; } else { newProperties[i] = property; } } return new PropertyDescriptorCollection(newProperties, true); } object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } #endregion //ICustomTypeDescriptor implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
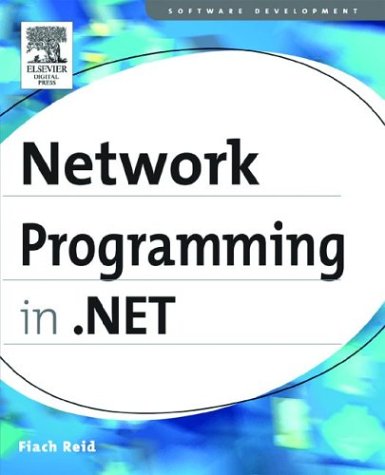
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScrollData.cs
- XmlSerializationGeneratedCode.cs
- DocumentSequence.cs
- ItemCheckEvent.cs
- DrawingGroup.cs
- RSAProtectedConfigurationProvider.cs
- AccessibleObject.cs
- SqlBinder.cs
- WebPartDisplayMode.cs
- ScrollData.cs
- ReadOnlyMetadataCollection.cs
- xmlglyphRunInfo.cs
- CollectionViewGroup.cs
- TemplateApplicationHelper.cs
- ToolStripSplitButton.cs
- EncoderBestFitFallback.cs
- ListChangedEventArgs.cs
- SyndicationDeserializer.cs
- TransformConverter.cs
- DrawingCollection.cs
- FromRequest.cs
- XmlReaderSettings.cs
- DependencyObjectPropertyDescriptor.cs
- CDSCollectionETWBCLProvider.cs
- BrowserCapabilitiesCompiler.cs
- WebBrowserHelper.cs
- DataGridViewComboBoxCell.cs
- HtmlInputReset.cs
- DataGridViewCellLinkedList.cs
- AssertFilter.cs
- RowUpdatingEventArgs.cs
- PropertyConverter.cs
- ReferenceEqualityComparer.cs
- DataGridViewUtilities.cs
- DateTimeFormat.cs
- OdbcConnection.cs
- EmbeddedMailObjectsCollection.cs
- Substitution.cs
- TextParaLineResult.cs
- DbTypeMap.cs
- NullReferenceException.cs
- DeferredTextReference.cs
- TextEndOfParagraph.cs
- SecurityTokenAuthenticator.cs
- DesignerProperties.cs
- StaticContext.cs
- TextBox.cs
- ScrollableControl.cs
- ProfessionalColors.cs
- ObjectViewListener.cs
- Viewport2DVisual3D.cs
- XmlILOptimizerVisitor.cs
- Identifier.cs
- CodeArrayIndexerExpression.cs
- TextWriterTraceListener.cs
- SizeChangedEventArgs.cs
- OdbcUtils.cs
- ImageMap.cs
- ProtocolsConfigurationEntry.cs
- PenThread.cs
- UrlMapping.cs
- COM2ICategorizePropertiesHandler.cs
- ChannelSettingsElement.cs
- ChooseAction.cs
- TreeViewImageKeyConverter.cs
- AtomServiceDocumentSerializer.cs
- SettingsPropertyIsReadOnlyException.cs
- Configuration.cs
- LiteralTextParser.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- GenerateScriptTypeAttribute.cs
- FolderBrowserDialogDesigner.cs
- WebConfigurationFileMap.cs
- NamespaceInfo.cs
- __Error.cs
- SecUtil.cs
- FontStyles.cs
- ContractSearchPattern.cs
- DataRecordObjectView.cs
- ObjectList.cs
- ConfigsHelper.cs
- RepeaterCommandEventArgs.cs
- GeneratedContractType.cs
- X509ChainElement.cs
- serverconfig.cs
- ByteStorage.cs
- OleStrCAMarshaler.cs
- DSACryptoServiceProvider.cs
- FixedPage.cs
- CompressStream.cs
- ObfuscationAttribute.cs
- Debug.cs
- ModelVisual3D.cs
- SiteOfOriginContainer.cs
- CapiSafeHandles.cs
- ResourceDefaultValueAttribute.cs
- WebPartManager.cs
- PrintEvent.cs
- DataBoundControl.cs
- XPathAncestorIterator.cs