Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / CornerRadius.cs / 1 / CornerRadius.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: CornerRadius.cs // // Description: Contains the CornerRadius (double x4) value type. // // History: // 07/19/2004 : t-jaredg - created. // //--------------------------------------------------------------------------- using MS.Internal; using System.ComponentModel; using System.Globalization; namespace System.Windows { ////// CornerRadius is a value type used to describe the radius of a rectangle's corners (controlled independently). /// It contains four double structs each corresponding to a corner: TopLeft, TopRight, BottomLeft, BottomRight. /// The corner radii cannot be negative. /// [TypeConverter(typeof(CornerRadiusConverter))] public struct CornerRadius : IEquatable{ //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors /// /// This constructor builds a CornerRadius with a specified uniform double radius value on every corner. /// /// The specified uniform radius. public CornerRadius(double uniformRadius) { _topLeft = _topRight = _bottomLeft = _bottomRight = uniformRadius; } ////// This constructor builds a CornerRadius with the specified doubles on each corner. /// /// The thickness for the top left corner. /// The thickness for the top right corner. /// The thickness for the bottom right corner. /// The thickness for the bottom left corner. public CornerRadius(double topLeft, double topRight, double bottomRight, double bottomLeft) { _topLeft = topLeft; _topRight = topRight; _bottomRight = bottomRight; _bottomLeft = bottomLeft; } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// This function compares to the provided object for type and value equality. /// /// Object to compare ///True if object is a CornerRadius and all sides of it are equal to this CornerRadius'. public override bool Equals(object obj) { if (obj is CornerRadius) { CornerRadius otherObj = (CornerRadius)obj; return (this == otherObj); } return (false); } ////// Compares this instance of CornerRadius with another instance. /// /// CornerRadius instance to compare. ///public bool Equals(CornerRadius cornerRadius) { return (this == cornerRadius); } /// true if this CornerRadius instance has the same value /// and unit type as cornerRadius./// This function returns a hash code. /// ///Hash code public override int GetHashCode() { return _topLeft.GetHashCode() ^ _topRight.GetHashCode() ^ _bottomLeft.GetHashCode() ^ _bottomRight.GetHashCode(); } ////// Converts this Thickness object to a string. /// ///String conversion. public override string ToString() { return CornerRadiusConverter.ToString(this, CultureInfo.InvariantCulture); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Operators // //-------------------------------------------------------------------- #region Public Operators ////// Overloaded operator to compare two CornerRadiuses for equality. /// /// First CornerRadius to compare /// Second CornerRadius to compare ///True if all sides of the CornerRadius are equal, false otherwise // SEEALSO public static bool operator==(CornerRadius cr1, CornerRadius cr2) { return ( (cr1._topLeft == cr2._topLeft || (DoubleUtil.IsNaN(cr1._topLeft) && DoubleUtil.IsNaN(cr2._topLeft))) && (cr1._topRight == cr2._topRight || (DoubleUtil.IsNaN(cr1._topRight) && DoubleUtil.IsNaN(cr2._topRight))) && (cr1._bottomRight == cr2._bottomRight || (DoubleUtil.IsNaN(cr1._bottomRight) && DoubleUtil.IsNaN(cr2._bottomRight))) && (cr1._bottomLeft == cr2._bottomLeft || (DoubleUtil.IsNaN(cr1._bottomLeft) && DoubleUtil.IsNaN(cr2._bottomLeft))) ); } ////// Overloaded operator to compare two CornerRadiuses for inequality. /// /// First CornerRadius to compare /// Second CornerRadius to compare ///False if all sides of the CornerRadius are equal, true otherwise // SEEALSO public static bool operator!=(CornerRadius cr1, CornerRadius cr2) { return (!(cr1 == cr2)); } #endregion Public Operators //------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ///This property is the Length on the thickness' top left corner public double TopLeft { get { return _topLeft; } set { _topLeft = value; } } ///This property is the Length on the thickness' top right corner public double TopRight { get { return _topRight; } set { _topRight = value; } } ///This property is the Length on the thickness' bottom right corner public double BottomRight { get { return _bottomRight; } set { _bottomRight = value; } } ///This property is the Length on the thickness' bottom left corner public double BottomLeft { get { return _bottomLeft; } set { _bottomLeft = value; } } #endregion Public Properties //------------------------------------------------------------------- // // Internal Methods Properties // //------------------------------------------------------------------- #region Internal Methods Properties internal bool IsValid(bool allowNegative, bool allowNaN, bool allowPositiveInfinity, bool allowNegativeInfinity) { if (!allowNegative) { if (_topLeft < 0d || _topRight < 0d || _bottomLeft < 0d || _bottomRight < 0d) { return (false); } } if (!allowNaN) { if (DoubleUtil.IsNaN(_topLeft) || DoubleUtil.IsNaN(_topRight) || DoubleUtil.IsNaN(_bottomLeft) || DoubleUtil.IsNaN(_bottomRight)) { return (false); } } if (!allowPositiveInfinity) { if (Double.IsPositiveInfinity(_topLeft) || Double.IsPositiveInfinity(_topRight) || Double.IsPositiveInfinity(_bottomLeft) || Double.IsPositiveInfinity(_bottomRight)) { return (false); } } if (!allowNegativeInfinity) { if (Double.IsNegativeInfinity(_topLeft) || Double.IsNegativeInfinity(_topRight) || Double.IsNegativeInfinity(_bottomLeft) || Double.IsNegativeInfinity(_bottomRight)) { return (false); } } return (true); } internal bool IsZero { get { return ( DoubleUtil.IsZero(_topLeft) && DoubleUtil.IsZero(_topRight) && DoubleUtil.IsZero(_bottomRight) && DoubleUtil.IsZero(_bottomLeft) ); } } #endregion Internal Methods Properties //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields private double _topLeft; private double _topRight; private double _bottomLeft; private double _bottomRight; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: CornerRadius.cs // // Description: Contains the CornerRadius (double x4) value type. // // History: // 07/19/2004 : t-jaredg - created. // //--------------------------------------------------------------------------- using MS.Internal; using System.ComponentModel; using System.Globalization; namespace System.Windows { ////// CornerRadius is a value type used to describe the radius of a rectangle's corners (controlled independently). /// It contains four double structs each corresponding to a corner: TopLeft, TopRight, BottomLeft, BottomRight. /// The corner radii cannot be negative. /// [TypeConverter(typeof(CornerRadiusConverter))] public struct CornerRadius : IEquatable{ //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors /// /// This constructor builds a CornerRadius with a specified uniform double radius value on every corner. /// /// The specified uniform radius. public CornerRadius(double uniformRadius) { _topLeft = _topRight = _bottomLeft = _bottomRight = uniformRadius; } ////// This constructor builds a CornerRadius with the specified doubles on each corner. /// /// The thickness for the top left corner. /// The thickness for the top right corner. /// The thickness for the bottom right corner. /// The thickness for the bottom left corner. public CornerRadius(double topLeft, double topRight, double bottomRight, double bottomLeft) { _topLeft = topLeft; _topRight = topRight; _bottomRight = bottomRight; _bottomLeft = bottomLeft; } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// This function compares to the provided object for type and value equality. /// /// Object to compare ///True if object is a CornerRadius and all sides of it are equal to this CornerRadius'. public override bool Equals(object obj) { if (obj is CornerRadius) { CornerRadius otherObj = (CornerRadius)obj; return (this == otherObj); } return (false); } ////// Compares this instance of CornerRadius with another instance. /// /// CornerRadius instance to compare. ///public bool Equals(CornerRadius cornerRadius) { return (this == cornerRadius); } /// true if this CornerRadius instance has the same value /// and unit type as cornerRadius./// This function returns a hash code. /// ///Hash code public override int GetHashCode() { return _topLeft.GetHashCode() ^ _topRight.GetHashCode() ^ _bottomLeft.GetHashCode() ^ _bottomRight.GetHashCode(); } ////// Converts this Thickness object to a string. /// ///String conversion. public override string ToString() { return CornerRadiusConverter.ToString(this, CultureInfo.InvariantCulture); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Operators // //-------------------------------------------------------------------- #region Public Operators ////// Overloaded operator to compare two CornerRadiuses for equality. /// /// First CornerRadius to compare /// Second CornerRadius to compare ///True if all sides of the CornerRadius are equal, false otherwise // SEEALSO public static bool operator==(CornerRadius cr1, CornerRadius cr2) { return ( (cr1._topLeft == cr2._topLeft || (DoubleUtil.IsNaN(cr1._topLeft) && DoubleUtil.IsNaN(cr2._topLeft))) && (cr1._topRight == cr2._topRight || (DoubleUtil.IsNaN(cr1._topRight) && DoubleUtil.IsNaN(cr2._topRight))) && (cr1._bottomRight == cr2._bottomRight || (DoubleUtil.IsNaN(cr1._bottomRight) && DoubleUtil.IsNaN(cr2._bottomRight))) && (cr1._bottomLeft == cr2._bottomLeft || (DoubleUtil.IsNaN(cr1._bottomLeft) && DoubleUtil.IsNaN(cr2._bottomLeft))) ); } ////// Overloaded operator to compare two CornerRadiuses for inequality. /// /// First CornerRadius to compare /// Second CornerRadius to compare ///False if all sides of the CornerRadius are equal, true otherwise // SEEALSO public static bool operator!=(CornerRadius cr1, CornerRadius cr2) { return (!(cr1 == cr2)); } #endregion Public Operators //------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ///This property is the Length on the thickness' top left corner public double TopLeft { get { return _topLeft; } set { _topLeft = value; } } ///This property is the Length on the thickness' top right corner public double TopRight { get { return _topRight; } set { _topRight = value; } } ///This property is the Length on the thickness' bottom right corner public double BottomRight { get { return _bottomRight; } set { _bottomRight = value; } } ///This property is the Length on the thickness' bottom left corner public double BottomLeft { get { return _bottomLeft; } set { _bottomLeft = value; } } #endregion Public Properties //------------------------------------------------------------------- // // Internal Methods Properties // //------------------------------------------------------------------- #region Internal Methods Properties internal bool IsValid(bool allowNegative, bool allowNaN, bool allowPositiveInfinity, bool allowNegativeInfinity) { if (!allowNegative) { if (_topLeft < 0d || _topRight < 0d || _bottomLeft < 0d || _bottomRight < 0d) { return (false); } } if (!allowNaN) { if (DoubleUtil.IsNaN(_topLeft) || DoubleUtil.IsNaN(_topRight) || DoubleUtil.IsNaN(_bottomLeft) || DoubleUtil.IsNaN(_bottomRight)) { return (false); } } if (!allowPositiveInfinity) { if (Double.IsPositiveInfinity(_topLeft) || Double.IsPositiveInfinity(_topRight) || Double.IsPositiveInfinity(_bottomLeft) || Double.IsPositiveInfinity(_bottomRight)) { return (false); } } if (!allowNegativeInfinity) { if (Double.IsNegativeInfinity(_topLeft) || Double.IsNegativeInfinity(_topRight) || Double.IsNegativeInfinity(_bottomLeft) || Double.IsNegativeInfinity(_bottomRight)) { return (false); } } return (true); } internal bool IsZero { get { return ( DoubleUtil.IsZero(_topLeft) && DoubleUtil.IsZero(_topRight) && DoubleUtil.IsZero(_bottomRight) && DoubleUtil.IsZero(_bottomLeft) ); } } #endregion Internal Methods Properties //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields private double _topLeft; private double _topRight; private double _bottomLeft; private double _bottomRight; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
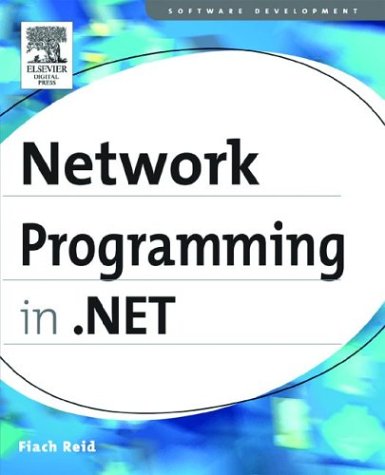
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamespaceEmitter.cs
- Registry.cs
- FontUnitConverter.cs
- WebServiceBindingAttribute.cs
- TableLayoutCellPaintEventArgs.cs
- AbstractExpressions.cs
- TextTrailingCharacterEllipsis.cs
- StorageComplexPropertyMapping.cs
- InputEventArgs.cs
- SettingsProviderCollection.cs
- LogEntryDeserializer.cs
- UIElement3D.cs
- AttachedAnnotation.cs
- RangeBaseAutomationPeer.cs
- WorkflowElementDialog.cs
- HttpCookiesSection.cs
- EventProvider.cs
- ClientApiGenerator.cs
- ReturnValue.cs
- DiscoveryClientReferences.cs
- EntityContainerAssociationSetEnd.cs
- HttpServerVarsCollection.cs
- XmlSerializer.cs
- ClientType.cs
- RotateTransform.cs
- String.cs
- BaseParagraph.cs
- SafeCoTaskMem.cs
- TextEditorCopyPaste.cs
- ResolveNameEventArgs.cs
- EntityDataSourceChangedEventArgs.cs
- ExeConfigurationFileMap.cs
- InputLanguageProfileNotifySink.cs
- PluralizationService.cs
- Function.cs
- StackBuilderSink.cs
- TaiwanCalendar.cs
- InvalidateEvent.cs
- TextRunCache.cs
- ArrangedElement.cs
- Transaction.cs
- CodeSnippetCompileUnit.cs
- WebExceptionStatus.cs
- Parser.cs
- ProgressBar.cs
- ObjectSet.cs
- CachedFontFamily.cs
- HtmlMeta.cs
- VisualBasicSettingsHandler.cs
- DecimalAnimationBase.cs
- SerializationException.cs
- SimpleWorkerRequest.cs
- TcpChannelHelper.cs
- CodeCatchClauseCollection.cs
- BrowserDefinitionCollection.cs
- ModelUIElement3D.cs
- DataPagerCommandEventArgs.cs
- CryptoStream.cs
- SqlDeflator.cs
- LinearGradientBrush.cs
- ComponentEditorPage.cs
- EventHandlersStore.cs
- ArgumentOutOfRangeException.cs
- RewritingProcessor.cs
- GradientStop.cs
- WrappedIUnknown.cs
- WebResourceAttribute.cs
- CngProvider.cs
- RectangleHotSpot.cs
- BridgeDataRecord.cs
- GridItemPattern.cs
- cookiecontainer.cs
- WizardPanel.cs
- ObjectDataSourceChooseTypePanel.cs
- RectangleGeometry.cs
- WsdlImporterElementCollection.cs
- HttpValueCollection.cs
- CompositeCollectionView.cs
- HoistedLocals.cs
- PropertyValueChangedEvent.cs
- SimpleTextLine.cs
- ObjectDataSourceMethodEventArgs.cs
- DispatcherHookEventArgs.cs
- TextEndOfLine.cs
- TextEffectResolver.cs
- Function.cs
- XsdDataContractImporter.cs
- CodeIterationStatement.cs
- WebRequest.cs
- LogicalTreeHelper.cs
- LinkAreaEditor.cs
- login.cs
- FloaterParaClient.cs
- AuthenticationService.cs
- CatalogZoneBase.cs
- SettingsBase.cs
- ScriptBehaviorDescriptor.cs
- WSHttpBindingCollectionElement.cs
- SqlClientWrapperSmiStream.cs
- ImageSourceConverter.cs