Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / documents / DocumentsTrace.cs / 1 / DocumentsTrace.cs
//#define TRACE //---------------------------------------------------------------------------- //// Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: DocumentsTrace is a tracing utility for Fixed and Flow documents // // History: // 02/03/2004 - Zhenbin Xu (ZhenbinX) - Created based on System.Windows.Media.Utility.MediaTrace // //--------------------------------------------------------------------------- namespace MS.Internal.Documents { using System; using System.Diagnostics; using System.Reflection; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationFramework; // SecurityHelper internal sealed class DocumentsTrace { internal static class FixedFormat { #if DEBUG public static DocumentsTrace FixedDocument = new DocumentsTrace("FixedFormat-FixedDocument"); public static DocumentsTrace PageContent= new DocumentsTrace("FixedFormat-PageContent"); public static DocumentsTrace IDF = new DocumentsTrace("FixedFormat-IDFAsync"); #else public static DocumentsTrace FixedDocument = null; public static DocumentsTrace PageContent = null; public static DocumentsTrace IDF = null; #endif #if DEBUG static FixedFormat() { // FixedFormat.IDF.Enable(); // FixedFormat.FixedDocument.Enable(); // FixedFormat.PageContent.Enable(); } #endif } internal static class FixedTextOM { #if DEBUG public static DocumentsTrace TextView = new DocumentsTrace("FixedTextOM-TextView"); public static DocumentsTrace TextContainer = new DocumentsTrace("FixedTextOM-TextContainer"); public static DocumentsTrace Map = new DocumentsTrace("FixedTextOM-FixedFlowMap"); public static DocumentsTrace Highlight = new DocumentsTrace("FixedTextOM-Highlight"); public static DocumentsTrace Builder = new DocumentsTrace("FixedTextOM-FixedTextBuilder"); public static DocumentsTrace FlowPosition = new DocumentsTrace("FixedTextOM-FlowPosition"); #else public static DocumentsTrace TextView = null; public static DocumentsTrace TextContainer = null; public static DocumentsTrace Map = null; public static DocumentsTrace Highlight = null; public static DocumentsTrace Builder = null; public static DocumentsTrace FlowPosition = null; #endif #if DEBUG // Note: // If you want to enable trace tags without recompiling. This is a good place to put a break point // during start-up. static FixedTextOM() { // FixedTextOM.TextView.Enable(); // FixedTextOM.TextContainer.Enable(); // FixedTextOM.Map.Enable(); // FixedTextOM.Highlight.Enable(); // FixedTextOM.Builder.Enable(); // FixedTextOM.FlowPosition.Enable(); } #endif } internal static class FixedDocumentSequence { #if DEBUG public static DocumentsTrace Content = new DocumentsTrace("FixedDocumentsSequence-Content"); public static DocumentsTrace IDF = new DocumentsTrace("FixedDocumentSequence-IDFAsync"); public static DocumentsTrace TextOM = new DocumentsTrace("FixedDocumentSequence-TextOM"); public static DocumentsTrace Highlights = new DocumentsTrace("FixedDocumentSequence-Highlights"); #else public static DocumentsTrace Content = null; public static DocumentsTrace IDF = null; public static DocumentsTrace TextOM = null; public static DocumentsTrace Highlights = null; #endif #if DEBUG static FixedDocumentSequence() { //FixedDocumentSequence.Content.Enable(); //FixedDocumentSequence.IDF.Enable(); //FixedDocumentSequence.TextOM.Enable(); //FixedDocumentSequence.Highlights.Enable(); } #endif } ////// Critical - it elevates to get trace listeners collection. /// TreatAsSafe - it doesn't disclose the information got through elevation. adding a well-known trace listener is considered safe. /// [SecurityCritical,SecurityTreatAsSafe] static DocumentsTrace() { #if DEBUG // we are adding console as a listener TextWriterTraceListener consoleListener = new TextWriterTraceListener( Console.Out ); // get the listeners collection TraceListenerCollection listeners = null; new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); try { listeners = System.Diagnostics.Trace.Listeners; } finally { SecurityPermission.RevertAssert(); } // add the console listener listeners.Add( consoleListener ); #endif } public DocumentsTrace(string switchName) { #if DEBUG string name = SafeSecurityHelper.GetAssemblyPartialName( Assembly.GetCallingAssembly() ); _switch = new BooleanSwitch(switchName, "[" + name + "]"); #endif } ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing, and this is debug only /// [SecurityCritical, SecurityTreatAsSafe] public DocumentsTrace(string switchName, bool initialState) : this(switchName) { #if DEBUG _switch.Enabled = initialState; #endif } [Conditional("DEBUG")] public void Trace(string message) { #if DEBUG if (_switch.Enabled) { System.Diagnostics.Trace.WriteLine(_switch.Description + " " + _switch.DisplayName + " : " + message); } #endif } [Conditional("DEBUG")] public void TraceCallers(int Depth) { #if DEBUG if (_switch.Enabled) { System.Diagnostics.StackTrace st = new System.Diagnostics.StackTrace(1); if (Depth < 0) Depth = st.FrameCount; Indent(); for (int i=0; i < st.FrameCount && i < Depth; i++) { System.Diagnostics.StackFrame sf = st.GetFrame(i); Trace(sf.GetMethod()+"+"+sf.GetILOffset().ToString()); } Unindent(); } #endif } [Conditional("DEBUG")] public void Indent() { #if DEBUG System.Diagnostics.Trace.Indent(); #endif } [Conditional("DEBUG")] public void Unindent() { #if DEBUG System.Diagnostics.Trace.Unindent(); #endif } ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing, and this is debug only /// [SecurityCritical, SecurityTreatAsSafe] [Conditional("DEBUG")] public void Enable() { #if DEBUG _switch.Enabled = true; #endif } ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to disable tracing, and this is debug only /// [SecurityCritical, SecurityTreatAsSafe] [Conditional("DEBUG")] public void Disable() { #if DEBUG _switch.Enabled = false; #endif } public bool IsEnabled { get { #if DEBUG return _switch.Enabled; #else return false; #endif } } #if DEBUG private System.Diagnostics.BooleanSwitch _switch; #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //#define TRACE //---------------------------------------------------------------------------- //// Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: DocumentsTrace is a tracing utility for Fixed and Flow documents // // History: // 02/03/2004 - Zhenbin Xu (ZhenbinX) - Created based on System.Windows.Media.Utility.MediaTrace // //--------------------------------------------------------------------------- namespace MS.Internal.Documents { using System; using System.Diagnostics; using System.Reflection; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationFramework; // SecurityHelper internal sealed class DocumentsTrace { internal static class FixedFormat { #if DEBUG public static DocumentsTrace FixedDocument = new DocumentsTrace("FixedFormat-FixedDocument"); public static DocumentsTrace PageContent= new DocumentsTrace("FixedFormat-PageContent"); public static DocumentsTrace IDF = new DocumentsTrace("FixedFormat-IDFAsync"); #else public static DocumentsTrace FixedDocument = null; public static DocumentsTrace PageContent = null; public static DocumentsTrace IDF = null; #endif #if DEBUG static FixedFormat() { // FixedFormat.IDF.Enable(); // FixedFormat.FixedDocument.Enable(); // FixedFormat.PageContent.Enable(); } #endif } internal static class FixedTextOM { #if DEBUG public static DocumentsTrace TextView = new DocumentsTrace("FixedTextOM-TextView"); public static DocumentsTrace TextContainer = new DocumentsTrace("FixedTextOM-TextContainer"); public static DocumentsTrace Map = new DocumentsTrace("FixedTextOM-FixedFlowMap"); public static DocumentsTrace Highlight = new DocumentsTrace("FixedTextOM-Highlight"); public static DocumentsTrace Builder = new DocumentsTrace("FixedTextOM-FixedTextBuilder"); public static DocumentsTrace FlowPosition = new DocumentsTrace("FixedTextOM-FlowPosition"); #else public static DocumentsTrace TextView = null; public static DocumentsTrace TextContainer = null; public static DocumentsTrace Map = null; public static DocumentsTrace Highlight = null; public static DocumentsTrace Builder = null; public static DocumentsTrace FlowPosition = null; #endif #if DEBUG // Note: // If you want to enable trace tags without recompiling. This is a good place to put a break point // during start-up. static FixedTextOM() { // FixedTextOM.TextView.Enable(); // FixedTextOM.TextContainer.Enable(); // FixedTextOM.Map.Enable(); // FixedTextOM.Highlight.Enable(); // FixedTextOM.Builder.Enable(); // FixedTextOM.FlowPosition.Enable(); } #endif } internal static class FixedDocumentSequence { #if DEBUG public static DocumentsTrace Content = new DocumentsTrace("FixedDocumentsSequence-Content"); public static DocumentsTrace IDF = new DocumentsTrace("FixedDocumentSequence-IDFAsync"); public static DocumentsTrace TextOM = new DocumentsTrace("FixedDocumentSequence-TextOM"); public static DocumentsTrace Highlights = new DocumentsTrace("FixedDocumentSequence-Highlights"); #else public static DocumentsTrace Content = null; public static DocumentsTrace IDF = null; public static DocumentsTrace TextOM = null; public static DocumentsTrace Highlights = null; #endif #if DEBUG static FixedDocumentSequence() { //FixedDocumentSequence.Content.Enable(); //FixedDocumentSequence.IDF.Enable(); //FixedDocumentSequence.TextOM.Enable(); //FixedDocumentSequence.Highlights.Enable(); } #endif } ////// Critical - it elevates to get trace listeners collection. /// TreatAsSafe - it doesn't disclose the information got through elevation. adding a well-known trace listener is considered safe. /// [SecurityCritical,SecurityTreatAsSafe] static DocumentsTrace() { #if DEBUG // we are adding console as a listener TextWriterTraceListener consoleListener = new TextWriterTraceListener( Console.Out ); // get the listeners collection TraceListenerCollection listeners = null; new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); try { listeners = System.Diagnostics.Trace.Listeners; } finally { SecurityPermission.RevertAssert(); } // add the console listener listeners.Add( consoleListener ); #endif } public DocumentsTrace(string switchName) { #if DEBUG string name = SafeSecurityHelper.GetAssemblyPartialName( Assembly.GetCallingAssembly() ); _switch = new BooleanSwitch(switchName, "[" + name + "]"); #endif } ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing, and this is debug only /// [SecurityCritical, SecurityTreatAsSafe] public DocumentsTrace(string switchName, bool initialState) : this(switchName) { #if DEBUG _switch.Enabled = initialState; #endif } [Conditional("DEBUG")] public void Trace(string message) { #if DEBUG if (_switch.Enabled) { System.Diagnostics.Trace.WriteLine(_switch.Description + " " + _switch.DisplayName + " : " + message); } #endif } [Conditional("DEBUG")] public void TraceCallers(int Depth) { #if DEBUG if (_switch.Enabled) { System.Diagnostics.StackTrace st = new System.Diagnostics.StackTrace(1); if (Depth < 0) Depth = st.FrameCount; Indent(); for (int i=0; i < st.FrameCount && i < Depth; i++) { System.Diagnostics.StackFrame sf = st.GetFrame(i); Trace(sf.GetMethod()+"+"+sf.GetILOffset().ToString()); } Unindent(); } #endif } [Conditional("DEBUG")] public void Indent() { #if DEBUG System.Diagnostics.Trace.Indent(); #endif } [Conditional("DEBUG")] public void Unindent() { #if DEBUG System.Diagnostics.Trace.Unindent(); #endif } ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing, and this is debug only /// [SecurityCritical, SecurityTreatAsSafe] [Conditional("DEBUG")] public void Enable() { #if DEBUG _switch.Enabled = true; #endif } ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to disable tracing, and this is debug only /// [SecurityCritical, SecurityTreatAsSafe] [Conditional("DEBUG")] public void Disable() { #if DEBUG _switch.Enabled = false; #endif } public bool IsEnabled { get { #if DEBUG return _switch.Enabled; #else return false; #endif } } #if DEBUG private System.Diagnostics.BooleanSwitch _switch; #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
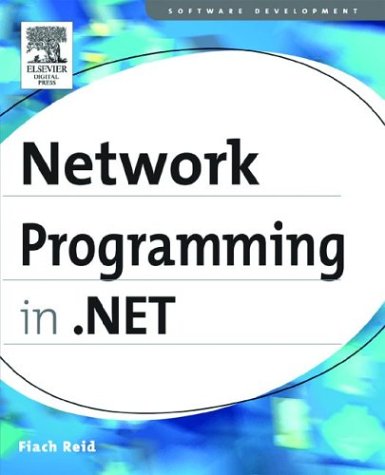
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FusionWrap.cs
- OrderedHashRepartitionStream.cs
- Symbol.cs
- DynamicMethod.cs
- ResourceReferenceExpressionConverter.cs
- PagedControl.cs
- CaseInsensitiveOrdinalStringComparer.cs
- MsmqActivation.cs
- FileRecordSequence.cs
- ElementsClipboardData.cs
- SettingsProperty.cs
- XMLDiffLoader.cs
- SoapHeaderAttribute.cs
- ClonableStack.cs
- CommentEmitter.cs
- ExceptionUtil.cs
- HierarchicalDataSourceConverter.cs
- TextEditorThreadLocalStore.cs
- WebBaseEventKeyComparer.cs
- EmptyCollection.cs
- ProxyWebPartManagerDesigner.cs
- TextEvent.cs
- TransformValueSerializer.cs
- CardSpaceShim.cs
- PixelFormat.cs
- BaseCodePageEncoding.cs
- CodeComment.cs
- ContainerCodeDomSerializer.cs
- IdentityModelStringsVersion1.cs
- TextClipboardData.cs
- WsiProfilesElementCollection.cs
- PackageFilter.cs
- CompilationRelaxations.cs
- XmlHelper.cs
- EventDescriptorCollection.cs
- TextEncodedRawTextWriter.cs
- _SecureChannel.cs
- SimpleExpression.cs
- ConfigXmlCDataSection.cs
- MimeObjectFactory.cs
- ScrollViewerAutomationPeer.cs
- SourceFileInfo.cs
- ApplicationFileCodeDomTreeGenerator.cs
- ErrorHandler.cs
- Knowncolors.cs
- Deserializer.cs
- _NestedSingleAsyncResult.cs
- dbdatarecord.cs
- ShapingEngine.cs
- MetadataArtifactLoaderResource.cs
- OdbcConnectionPoolProviderInfo.cs
- DialogDivider.cs
- ServerValidateEventArgs.cs
- UserNamePasswordServiceCredential.cs
- RenderOptions.cs
- MutableAssemblyCacheEntry.cs
- AuthenticationModuleElement.cs
- InternalCache.cs
- XmlTextWriter.cs
- ToolStripSystemRenderer.cs
- MergeFailedEvent.cs
- ValidationSummary.cs
- ServiceModelSecurityTokenRequirement.cs
- SqlPersonalizationProvider.cs
- TextTreePropertyUndoUnit.cs
- XsdCachingReader.cs
- ReadWriteObjectLock.cs
- RegexCompiler.cs
- PageCodeDomTreeGenerator.cs
- SoapParser.cs
- VirtualDirectoryMappingCollection.cs
- SmiEventStream.cs
- RemotingAttributes.cs
- EntryWrittenEventArgs.cs
- XPathNodeList.cs
- BitVector32.cs
- FormView.cs
- DrawingServices.cs
- MultipartContentParser.cs
- CodeTypeDeclaration.cs
- XmlSchemaAnnotation.cs
- FontUnit.cs
- MissingManifestResourceException.cs
- PolyBezierSegment.cs
- SQLChars.cs
- HttpApplication.cs
- ExpressionBuilder.cs
- TransformedBitmap.cs
- IsolationInterop.cs
- XmlComment.cs
- IHttpResponseInternal.cs
- TextEffectCollection.cs
- ControlCommandSet.cs
- Logging.cs
- VectorKeyFrameCollection.cs
- BuildProviderAppliesToAttribute.cs
- XsltSettings.cs
- TreeNode.cs
- StorageEndPropertyMapping.cs
- SessionStateUtil.cs